This content originally appeared on DEV Community and was authored by Kauna Hassan
React and Redux are two popular web development libraries. React is a JavaScript library for creating simple and effective user interfaces. Redux is a JavaScript library for managing an application's state. Integrating React and Redux can be extremely beneficial when developing large-scale applications. This tutorial will walk you through the process of integrating React with Redux step by step.
Step 1: Create a new project.
To begin, you must create a new React project. To start a new project, use create-react-app. Enter the following command into your command line.
npx create-react-app my-app
Step 2: Install Redux
In the next step, you need to install Redux. Type the following command in the command line.
npm install redux react-redux
Step 3: Create a Redux store
Create a store that contains the application's state using the createStore method from the Redux library. This store will hold the entire state of the application.
import { createStore } from 'redux';
const store = createStore(reducer);
Step 4: Create a reducer
A reducer is a pure function that receives the current state and an action and returns a new state. You use reducers to update the state in your store.
const initialState = {
//initial state
};
function reducer(state = initialState, action) {
switch (action.type) {
//switch cases for different actions
default:
return state;
}
}
export default reducer;
Step 5: Create actions
Actions are objects that describe what should happen in the application. An action is a function that creates an object with a type property and optional payload.
const incrementCount = () => ({
type: 'INCREMENT_COUNT',
payload: 1
});
export { incrementCount }
Step 6: Connect a component to the store
Finally, you need to connect a component to the store. Use the connect method from the react-redux library to connect a component to the store.
import { connect } from 'react-redux';
const Count = ({ count, incrementCount }) => {
return (
<div>
<h1>Count: {count}</h1>
<button onClick={incrementCount}>Increment Count</button>
</div>
);
}
const mapStateToProps = (state) => ({
count: state.count
});
const mapDispatchToProps = {
incrementCount: incrementCount
};
export default connect(mapStateToProps, mapDispatchToProps)(Count);
Conclusion
In conclusion, integrating React with Redux is a powerful technique for building large-scale applications. By following the above steps, you can connect your React component with the Redux store seamlessly. This tutorial has provided a step-by-step guide to help you integrate React with Redux.
This content originally appeared on DEV Community and was authored by Kauna Hassan
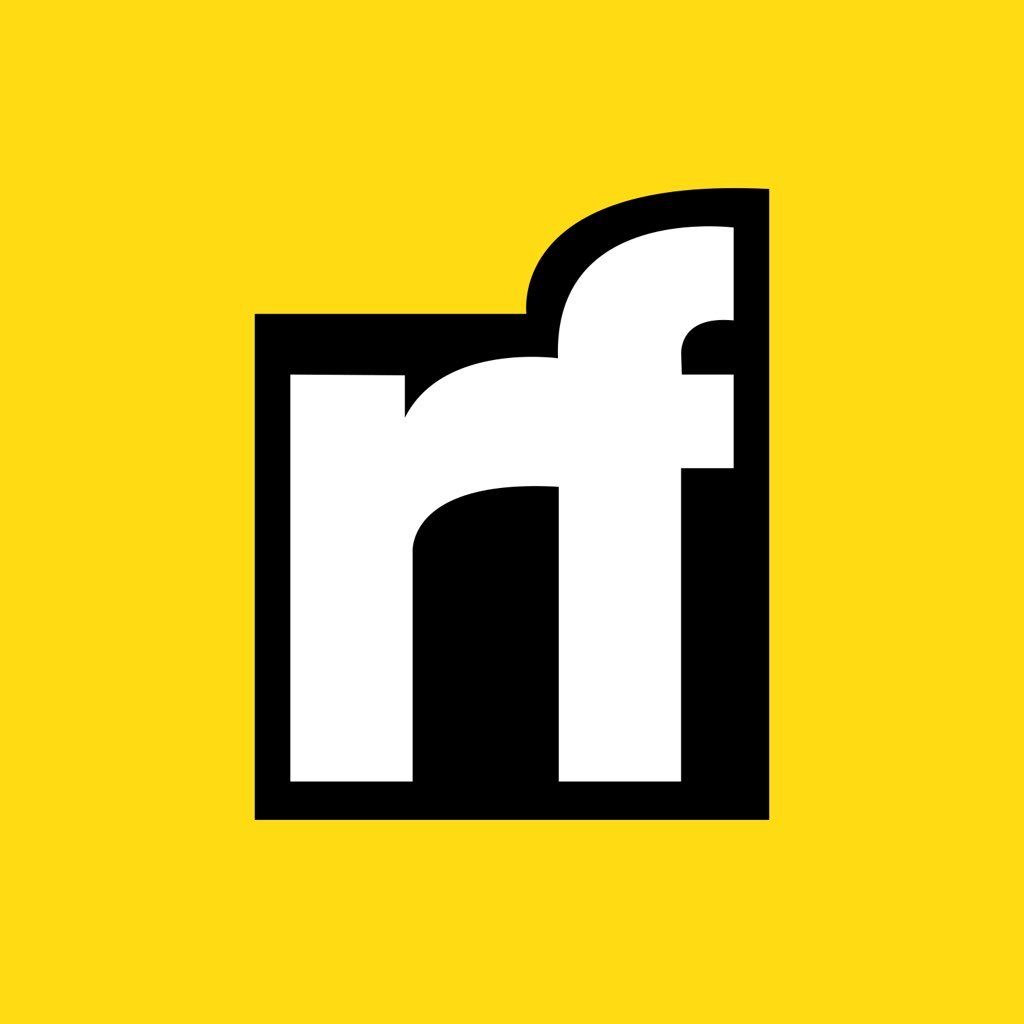
Kauna Hassan | Sciencx (2023-03-11T15:18:53+00:00) How to Integrate React with Redux: Step-by-Step Tutorial. Retrieved from https://www.scien.cx/2023/03/11/how-to-integrate-react-with-redux-step-by-step-tutorial/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.