This content originally appeared on Bits and Pieces - Medium and was authored by Shamaz Saeed
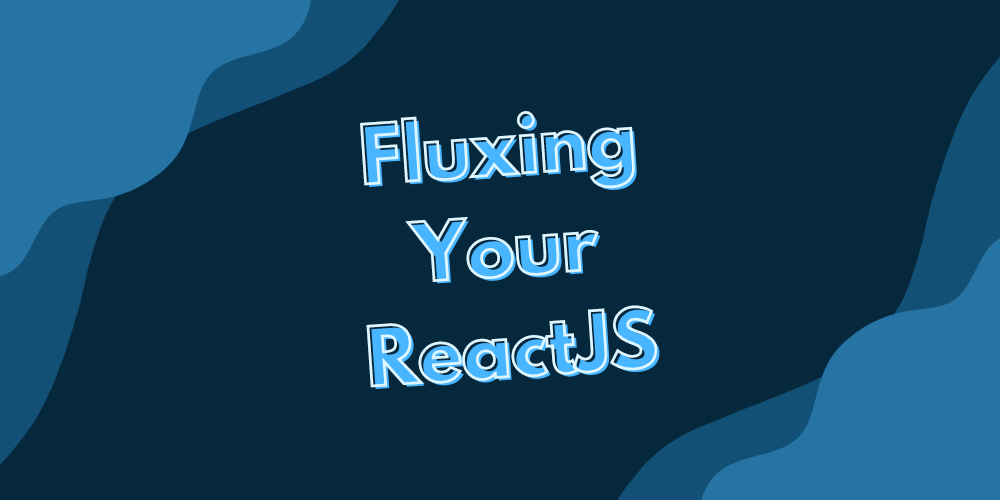
As a front-end developer, managing state in a large-scale ReactJS application can be challenging. As an application grows, the state management can become more complex and harder to maintain. This is where the Flux architecture comes in. Flux is a pattern that helps manage data flow in an application and is particularly useful for ReactJS applications.
In this article, we’ll take a look at what the Flux architecture is, how it works, and how you can use it to manage state in your ReactJS applications.
What is the Flux Architecture?
Flux is an architecture for building client-side web applications that was created by Facebook. It is a pattern for managing data flow and is particularly useful for ReactJS applications. Flux is based on three core principles:
- Unidirectional Data Flow: The data in a Flux application flows in one direction, from the view to the actions, to the dispatcher, to the stores, and back to the view. This helps keep the data flow organized and makes it easier to reason about the state of the application.
- Centralized Control: The control of the application state is centralized in the dispatcher. The dispatcher receives actions from the view and sends them to the appropriate store. This helps keep the state management consistent and prevents race conditions.
- Immutable Data: The data in a Flux application is immutable. This means that the state of the application cannot be changed directly. Instead, when a change is needed, a new state is created and the old state is replaced. This makes it easier to track changes and maintain the integrity of the data.
How Does Flux Work?
Flux is composed of four main parts: the view, the action, the dispatcher, and the store. Let’s take a closer look at each of these parts.
1. View: The view is responsible for rendering the user interface and capturing user actions. When a user interacts with the view, it triggers an action.
2. Action: An action is a plain JavaScript object that describes an event in the system. When an action is triggered, it is sent to the dispatcher.
3. Dispatcher: The dispatcher is the central hub of a Flux application. It receives actions from the view and sends them to the appropriate store. It ensures that the data flow is unidirectional and that there is no direct coupling between the view and the store.
4. Store: The store is responsible for managing the state of the application. It receives actions from the dispatcher, updates its state accordingly, and emits a change event to notify the view that the state has changed. The view then re-renders with the updated state.
How to Use Flux in ReactJS Applications?
To use Flux in a ReactJS application, you need to implement the four parts of the architecture described above. Here’s how you can do it:
1. View: In a ReactJS application, the view is implemented using components. When a user interacts with a component, it triggers an action. Here’s an example of a component that triggers an action when a button is clicked:
import React from 'react';
import { myAction } from './actions';
class MyComponent extends React.Component {
handleClick() {
myAction({ data: 'some data' });
}
render() {
return (
<button onClick={this.handleClick}>Click me</button>
);
}
}
2. Action: An action is a plain JavaScript object that describes an event in the system. Actions are defined in a separate file, usually called actions.js. Here's an example of an action that sends data to the dispatcher:
import dispatcher from './dispatcher';
export function myAction(data) {
dispatcher.dispatch({
type: 'MY_ACTION',
data,
});
}
3. Dispatcher: The dispatcher is responsible for receiving actions from the view and sending them to the appropriate store. The dispatcher is implemented using the `flux` package. Here’s an example of a dispatcher that receives actions from the view and sends them to the store:
import { Dispatcher } from 'flux';
const dispatcher = new Dispatcher();
export default dispatcher;
4. Store: The store is responsible for managing the state of the application. The store is implemented using the EventEmitter module, which allows it to emit change events when the state changes. Here's an example of a store that receives actions from the dispatcher, updates its state accordingly, and emits a change event:
import { EventEmitter } from 'events';
import dispatcher from './dispatcher';
const myStore = new EventEmitter();
let myData = [];
function handleAction(action) {
switch (action.type) {
case 'MY_ACTION':
myData.push(action.data);
myStore.emit('change');
break;
default:
// Do nothing
}
}
dispatcher.register(handleAction);
export function getData() {
return myData;
}
export function addChangeListener(callback) {
myStore.on('change', callback);
}
export function removeChangeListener(callback) {
myStore.removeListener('change', callback);
}
Once you’ve implemented the view, action, dispatcher, and store, you can use them together in your ReactJS application to manage state. Here’s an example of a component that uses the store to display data:
import React from 'react';
import { getData, addChangeListener, removeChangeListener } from './myStore';
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
data: getData(),
};
}
componentDidMount() {
addChangeListener(this.handleChange);
}
componentWillUnmount() {
removeChangeListener(this.handleChange);
}
handleChange = () => {
this.setState({ data: getData() });
}
render() {
const { data } = this.state;
return (
<ul>
{data.map(item => (
<li key={item.id}>{item.name}</li>
))}
</ul>
);
}
}
In this example, the MyComponent component gets the initial data from the myStore store and subscribes to change events using the addChangeListener method. When the store emits a change event, the handleChange method is called, which updates the state of the component with the new data. The component then re-renders with the updated data.
Conclusion
Flux is a powerful architecture for managing state in ReactJS applications. By using a unidirectional data flow, centralized control, and immutable data, Flux helps keep your application’s state management organized and maintainable. Implementing Flux in your ReactJS application may require some upfront work, but the benefits are well worth it in the long run.
Build Apps with reusable components, just like Lego
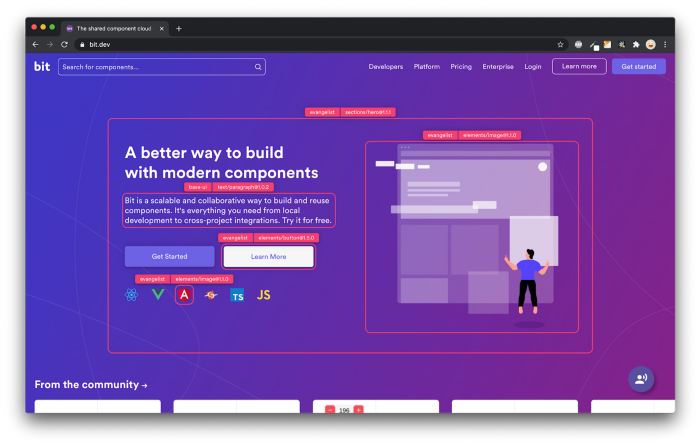
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
A Step-by-Step Guide to Flux Architecture with React was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Shamaz Saeed
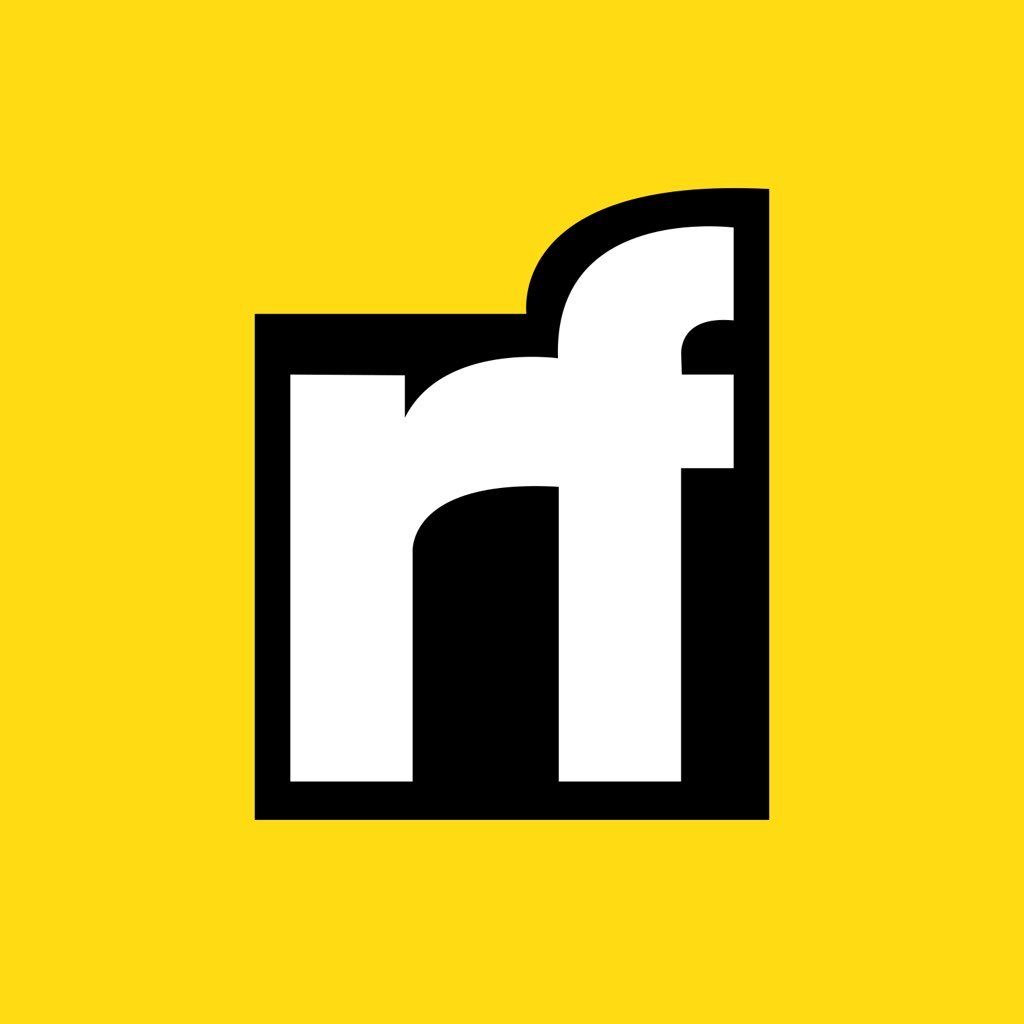
Shamaz Saeed | Sciencx (2023-03-12T08:31:28+00:00) A Step-by-Step Guide to Flux Architecture with React. Retrieved from https://www.scien.cx/2023/03/12/a-step-by-step-guide-to-flux-architecture-with-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.