This content originally appeared on Level Up Coding - Medium and was authored by Shamaz Saeed
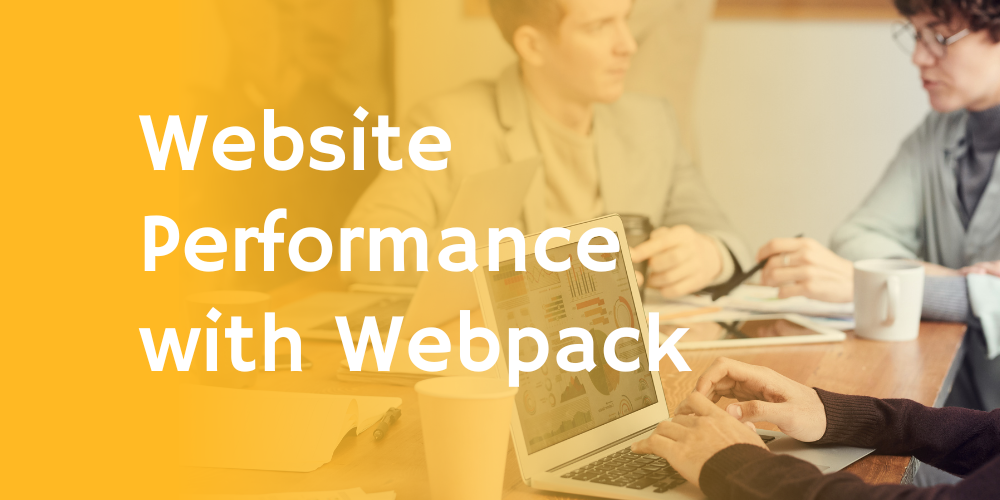
Website performance is critical for providing a good user experience, and Webpack is a powerful tool for optimizing website performance. In this article, we will explore some advanced techniques for using Webpack to optimize website performance, including code splitting, tree shaking, and dynamic imports. We will also cover some best practices for configuring Webpack for optimal performance.
Code Splitting
Code splitting is a technique for dividing your code into smaller, more manageable chunks that can be loaded on demand. This can help improve website performance by reducing the amount of code that needs to be loaded when a user visits your site.
To use code splitting in Webpack, you can use the splitChunks configuration option. This option allows you to specify which modules should be split into separate chunks. You can also use dynamic imports to load modules on demand, further reducing the amount of code that needs to be loaded upfront.
Here’s an example configuration for code splitting in Webpack:
module.exports = {
optimization: {
splitChunks: {
chunks: 'all',
cacheGroups: {
defaultVendors: {
test: /[\\/]node_modules[\\/]/,
name: 'vendors',
chunks: 'all',
},
},
},
},
};
In this example, we are using the splitChunks configuration option to split all chunks, and we are using the defaultVendors cache group to specify that any modules in the node_modules directory should be split into a separate chunk named vendors.
Tree Shaking
Tree shaking is a technique for removing unused code from your application. This can help reduce the amount of code that needs to be loaded and executed, improving website performance.
To use tree shaking in Webpack, you can use the optimization.usedExports configuration option. This option tells Webpack to only include the code that is actually used in your application, rather than including the entire module.
Here’s an example configuration for tree shaking in Webpack:
module.exports = {
optimization: {
usedExports: true,
},
};
In this example, we are using the usedExports configuration option to enable tree shaking.
Dynamic Imports
Dynamic imports are a technique for loading modules on demand, which can help reduce the amount of code that needs to be loaded upfront. This can be particularly useful for modules that are only needed in certain parts of your application.
To use dynamic imports in Webpack, you can use the import() function. This function returns a Promise that resolves to the module when it is loaded. You can then use this module in your application.
Here’s an example of using dynamic imports in a React component:
import React, { useState } from 'react';
const MyComponent = () => {
const [Module, setModule] = useState(null);
const handleClick = async () => {
const module = await import('./my-module.js');
setModule(module);
};
return (
<div>
<button onClick={handleClick}>Load Module</button>
{Module && <Module />}
</div>
);
};
export default MyComponent;
In this example, we are using the import() function to load a module on demand when a button is clicked. The module is then rendered using JSX.
Best Practices for Webpack Configuration
In addition to these advanced techniques, there are some best practices that you should follow when configuring Webpack for optimal performance:
- Minimize the number of plugins and loaders
- Use production mode in your Webpack configuration to enable optimizations
- Use source maps to help with debugging
- Use cache-loader to cache expensive operations
- Use babel-loader to transpile your code for maximum browser compatibility
- Use HtmlWebpackPlugin to generate a production index.html file
Conclusion:
In this article, we have covered some advanced techniques for optimizing website performance with Webpack. We explored code splitting, tree shaking, and dynamic imports, as well as some best practices for configuring Webpack for optimal performance. By following these techniques and best practices, you can improve website performance and provide a better user experience for your users.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Advanced Techniques for Optimizing Website Performance with Webpack was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Shamaz Saeed
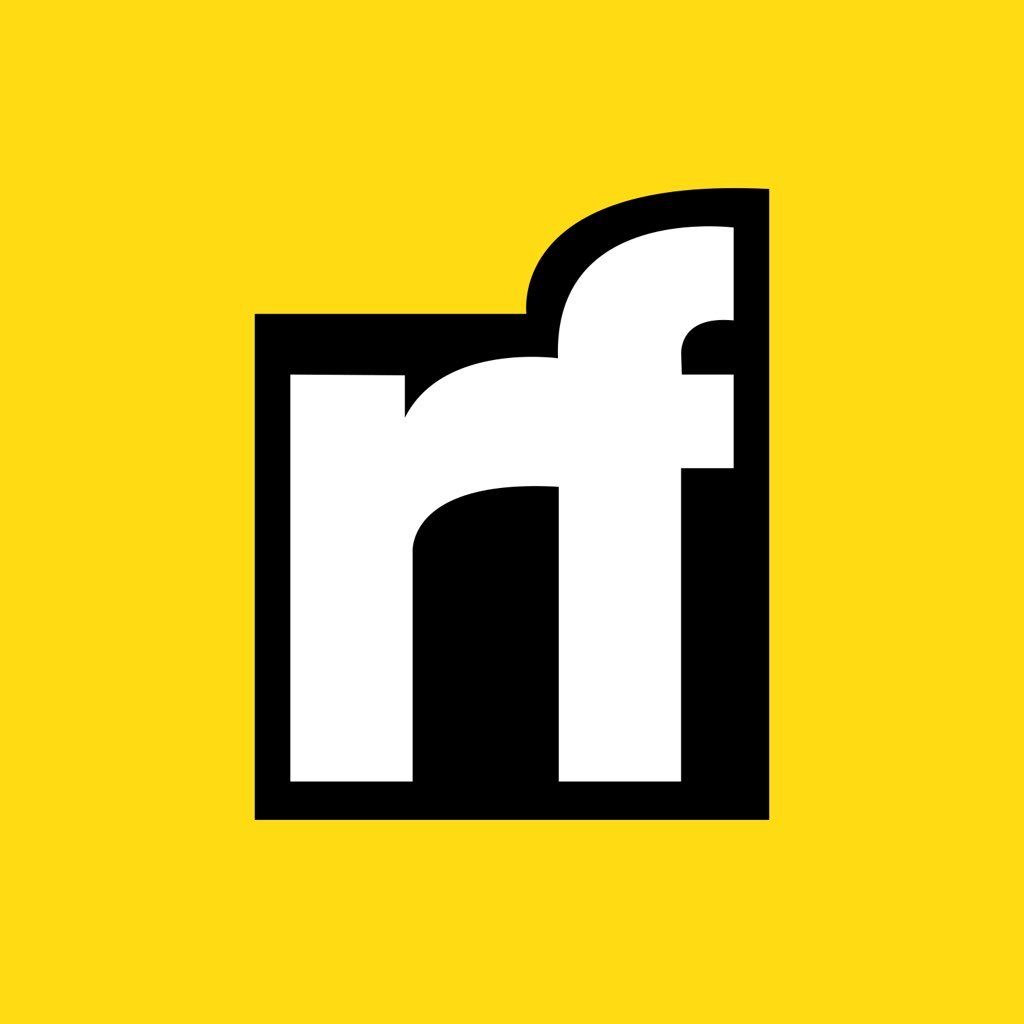
Shamaz Saeed | Sciencx (2023-03-14T15:22:13+00:00) Advanced Techniques for Optimizing Website Performance with Webpack. Retrieved from https://www.scien.cx/2023/03/14/advanced-techniques-for-optimizing-website-performance-with-webpack/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.