This content originally appeared on Bits and Pieces - Medium and was authored by Brian Ridolce
Handling form data is a crucial aspect of building web applications. In Next.js, we can use the Formik library to handle form data in a more organized and efficient way, while also leveraging TypeScript’s type checking capabilities. In this article, we’ll walk through how to handle form data with Formik and TypeScript in a Next.js application.
Setting up Formik in Next.js
First, we need to install the necessary dependencies for Formik and Yup, a library for schema validation. We can do this by running the following command in our project directory:
npm install formik yup @types/yup
Now that we have Formik and Yup installed, we can set up a basic form with Formik. Let’s create a new component called ContactForm in our pages directory, and import Formik and Yup at the top of the file.
import { Formik, Form, Field } from 'formik';
import * as Yup from 'yup';
In the ContactForm component, we can define a schema for our form data using Yup's schema validation. This will ensure that the form data is valid and matches our expected data types.
const validationSchema = Yup.object().shape({
name: Yup.string().required('Please enter your name'),
email: Yup.string().email('Please enter a valid email address').required('Please enter your email'),
});
Next, we can create a new ContactForm component using Formik's Formik and Form components. The initialValues prop will initialize our form data with empty strings, and the onSubmit prop will handle the form submission.
function ContactForm() {
const initialValues = { name: '', email: '' };
const handleSubmit = (values: typeof initialValues) => {
console.log(values);
};
return (
<Formik initialValues={initialValues} onSubmit={handleSubmit} validationSchema={validationSchema}>
<Form>
<div>
<label htmlFor="name">Name:</label>
<Field type="text" id="name" name="name" />
</div>
<div>
<label htmlFor="email">Email:</label>
<Field type="email" id="email" name="email" />
</div>
<button type="submit">Submit</button>
</Form>
</Formik>
);
}
Using Formik’s Field component instead of a regular input element allows us to easily connect the input value to Formik's state management. The handleSubmit function will be called when the form is submitted, and the values argument will contain the form data.
💡 Note: Once you’ve built the form component, you can isolate and extract this component into packages, so you can use an open-source toolchain like Bit to publish, version, and reuse it across all of your projects with a simple npm i @bit/your-username/ContactForm. Find out more here, and here.
Handling Form Validation Errors
Now that we have our basic form set up, we can add some logic to display validation errors to the user if they enter invalid form data. We can do this by adding the ErrorMessage component from Formik to our form, which will display an error message if the form data does not match our validation schema.
import { Formik, Form, Field, ErrorMessage } from 'formik';
function ContactForm() {
// ...
return (
<Formik initialValues={initialValues} onSubmit={handleSubmit} validationSchema={validationSchema}>
<Form>
<div>
<label htmlFor="name">Name:</label>
<Field type="text" id="name" name="name" />
<ErrorMessage name="name" />
</div>
<div>
<label htmlFor="email">Email:</label>
<Field type="email" id="email" name="email" />
<ErrorMessage name="email" />
</div>
<button type="submit">Submit</button>
</Form>
</Formik>
);
}
The `ErrorMessage` component will automatically display an error message if the `name` or `email` field does not match the validation schema.
Handling Form Submission
When the user submits the form, the `handleSubmit` function will be called with the form data. We can then use this data to send a request to a server, or update our UI based on the user’s input. For example, we could use the `axios` library to send a POST request to a server with the form data.
import axios from 'axios';
function ContactForm() {
// ...
const handleSubmit = async (values: typeof initialValues) => {
try {
await axios.post('/api/contact', values);
alert('Message sent!');
} catch (error) {
console.error(error);
alert('An error occurred while sending your message. Please try again later.');
}
};
// ...
}
In this example, we’re sending a POST request to an API endpoint called `/api/contact` with the form data. If the request is successful, we’ll display an alert to the user saying “Message sent!”. If there’s an error, we’ll display an error message.
Using TypeScript with Formik
Now that we have our form set up with Formik and Yup, we can take advantage of TypeScript’s type-checking capabilities to ensure that our form data is properly typed. By default, Formik will infer the type of our form data based on the `initialValues` prop. However, we can also define an interface for our form data to provide additional type checking.
interface ContactFormData {
name: string;
email: string;
}
function ContactForm() {
const initialValues: ContactFormData = { name: '', email: '' };
const handleSubmit = async (values: ContactFormData) => {
// ...
};
// ...
}
In this example, we’ve defined an interface called `ContactFormData` that describes the shape of our form data. We’re also using the `ContactFormData` interface to type the `initialValues` prop and the `values` argument in the `handleSubmit` function.
Conclusion
In this article, we walked through how to handle form data with Formik and TypeScript in a Next.js application. We learned how to set up a basic form with Formik, handle form validation errors, and submit the form data to a server. We also learned how to use TypeScript to ensure that our form data is properly typed, which can help catch errors early in the development process.
Overall, integrating Formik into our Next.js applications can help us handle form data more efficiently, while also taking advantage of TypeScript’s type-checking capabilities.
Build Apps with reusable components, just like Lego
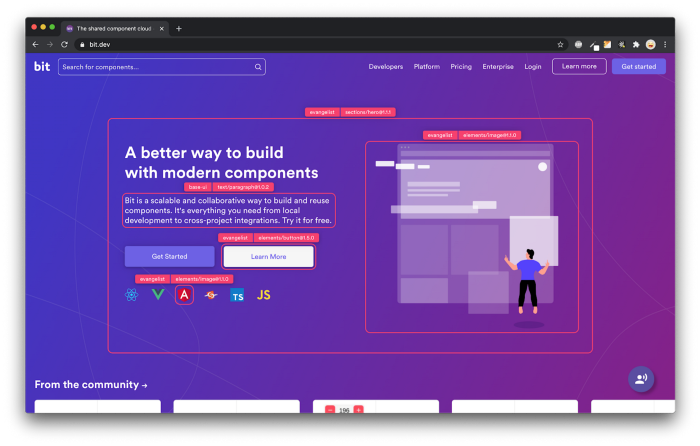
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Handling Form Data with TypeScript and Formik was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Brian Ridolce
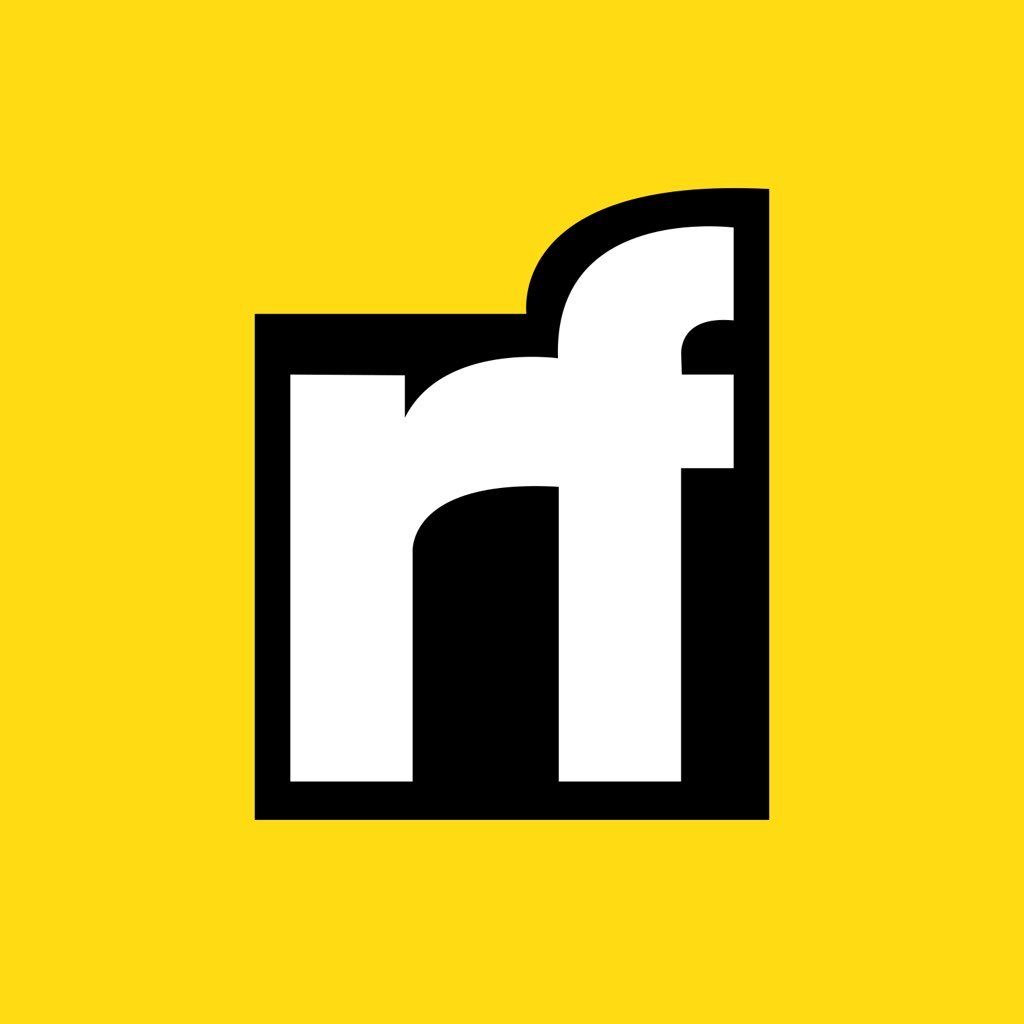
Brian Ridolce | Sciencx (2023-03-16T11:02:24+00:00) Handling Form Data with TypeScript and Formik. Retrieved from https://www.scien.cx/2023/03/16/handling-form-data-with-typescript-and-formik/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.