This content originally appeared on Bits and Pieces - Medium and was authored by Dev Wanderer
JavaScript
A simple plugin allows for convenient switching between languages.
I recently built a static website for a family wedding. Because I’m a fan of Netlify and its price was right (free) I decided to build and host it there. It also gave me a chance to get more familiar with the Jamstack paradigm they promote.
I wanted a little more experience with static site generation and thought Gatsby would be a good fit. I was impressed with its plugin collection, especially when it became clear that my site would need to serve both English and Spanish-speaking guests.
I knew I wanted to research proven ways of handling internationalization — and didn’t want to just make two versions of the site. This is when I discovered gatsby-plugin-intl. This plugin allows you to parameterize your content and swap in values from language-specific JSON files. You can organize these and name properties however you see fit.
Here’s an excerpt from my en.json file for English content.
"menu" : {
"live": "Live Stream",
"gifts": "Gift Registry",
"story": "Our Story",
"guestbook": "Guestbook",
"list": "Email List"
}
Here’s the same excerpt from my es.json file for Spanish content.
"menu" : {
"live": "En Vivo",
"gifts": "Regalos",
"story": "La Historia",
"guestbook": "Saludos",
"list": "Noticias"
}
As you can see, these excerpts contain translations for the options used in the menu. We’ll look closer at that in a moment.
With the JSON files ready, I just needed to add the gatsby-plugin-int configuration to the plugins section of the gatsby-config.js file at the root of my project. That addition looked like this:
{
resolve: `gatsby-plugin-intl`,
options: {
// language JSON resource path
path: `${__dirname}/src/intl`,
// supported language
languages: [`en`, `es`],
// language file path
defaultLanguage: `en`,
// option to redirect to `/en` when connecting `/`
redirect: true,
},
}
The path property points to the folder where I stored the JSON files. The defaultLanguage property specifies which language file to start with.
With the plugin installed, I could now use its FormattedMessage component, with references to the relevant properties in the JSON files. The following code is for a Menu component I made, in which each menu option’s text is pulled from the JSON file for the selected language. You can see that the menu properties shown in the JSON excerpts earlier can now be referenced with id values formatted like JavaScript object property references.
import React from "react"
import { Link } from "gatsby"
import { FormattedMessage } from "gatsby-plugin-intl"
export default function Menu() {
return (
<ul id="nav">
<li><Link to="/live"><FormattedMessage id="menu.live" /></Link></li>
<li><Link to="/story"><FormattedMessage id="menu.story" /></Link></li>
<li><Link to="/registry"><FormattedMessage id="menu.gifts" /></Link></li>
<li><Link to="/guestbook"><FormattedMessage id="menu.guestbook" /></Link></li>
<li><Link to="/list"><FormattedMessage id="menu.list" /></Link></li>
</ul>
)
}
I was able to let users change languages by clicking flag icons in a footer component.
import React from "react"
import { Link } from "gatsby"
import { FormattedMessage, changeLocale } from "gatsby-plugin-intl"
export default function Footer() {
return (
<div id="footer">
<hr/>
<span><FormattedMessage id="content.civil" />: <Link to="/live">Provo, UT</Link></span>
<hr/>
<span id="flags">
<button className="icon-link" onClick={ () => changeLocale("en") }>
<img src="/images/usa.png" alt="en" /></button>|
<button className="icon-link" onClick={ () => changeLocale("es") }>
<img src="/images/argentina.png" alt="es-AR" />{" "}
<img src="/images/pr.png" alt="es-PR" />
</button>
</span>
</div>
)
}
Notice the onClick handlers which call the changeLocale function, provided by gatsby-plugin-intl. That function takes a parameter for the desired locale, which maps to the name of the JSON files to be used.
No server-side logic was needed anywhere on the site. Once the build was complete and the site deployed, it was all pushed out as separate HTML files — but Gatsby, and the plugin, handle all the switching between language versions for you. Once I had everything in my GitHub repository, I just pointed my Netlify account to it and the rest was magic. Simple automated deployments!
I was already impressed with Gatsby and how quickly I was able to get a site up and running in the Netlify/JamStack environment, but this speedy language switching was really a lifesaver. I recommend checking it out if you ever find yourself with a similar need.
Build React Apps with reusable components, just like Lego
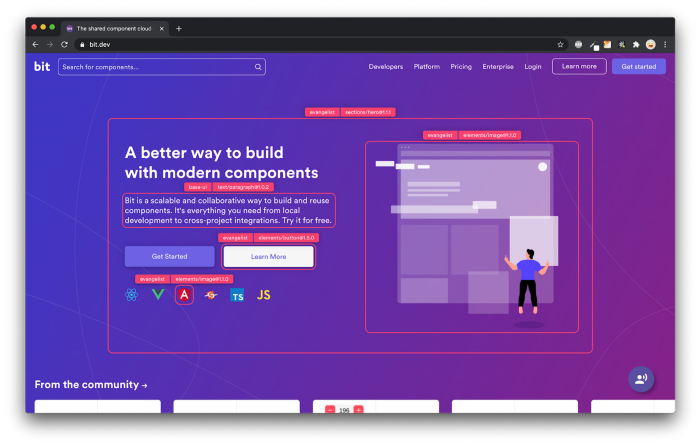
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
Easy Internationalization with Gatsby was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Dev Wanderer
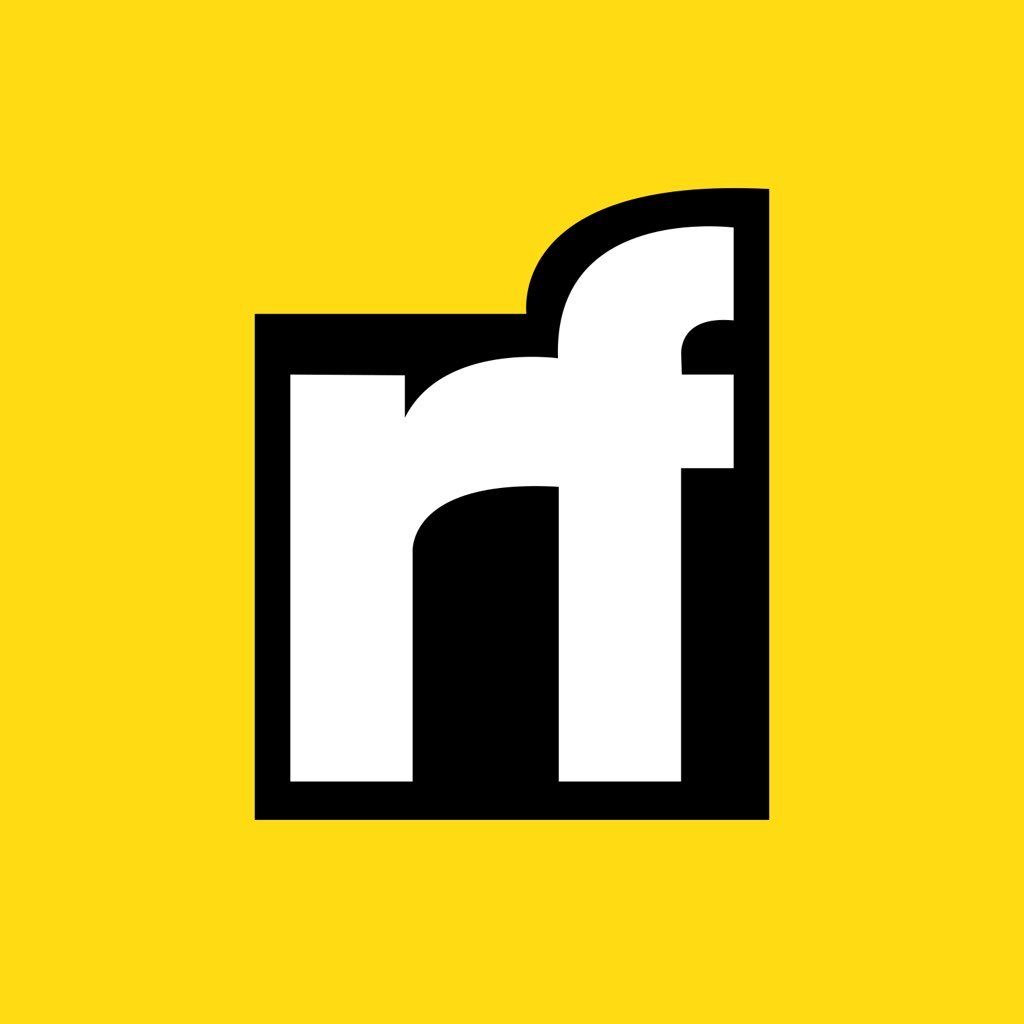
Dev Wanderer | Sciencx (2023-03-18T07:31:33+00:00) Easy Internationalization with Gatsby. Retrieved from https://www.scien.cx/2023/03/18/easy-internationalization-with-gatsby/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.