As always, thanks a lot for reading!
How to Build a Mobile Menu Microinteraction With CSS
Today, we’ll use the CSS checkbox hack technique to build an animated mobile menu; a useful microinteraction and an alternative UI to the main menu on mobile screens.
Without further ado, let’s preview the final demo:
What Are Microinteractions?
Microinteraction is a term heard often in UI and UX design these days. Microinteractions are trigger-feedback pairs, in other words where:
- something happens (by either a user doing something, or the system status changing)
- and then there’s feedback to show that something has happened.
The feedback is usually a small, highly contextual, visual indication in the UI. Today we’re going to build a mobile menu which, when clicked, makes menu items appear or disappear. We’ll use motion and color to give the user feedback.
CSS Animated Mobile Menu
1. Define the Page Markup
To kick things off we’ll define a nav
element where we’ll place:
- A checkbox followed by their associated label and
- the main menu
Each menu item will include the text node wrapped inside a span
element and a Font Awesome icon. For this reason, we’ve already loaded the Font Awesome library in our pen.
1 |
<nav class="nav"> |
2 |
<input id="menu" type="checkbox"> |
3 |
<label for="menu">Menu</label> |
4 |
<ul class="menu"> |
5 |
<li>
|
6 |
<a href="#0"> |
7 |
<span>About</span> |
8 |
<i class="fas fa-address-card" aria-hidden="true"></i> |
9 |
</a>
|
10 |
</li>
|
11 |
<li>
|
12 |
<a href="#0"> |
13 |
<span>Projects</span> |
14 |
<i class="fas fa-tasks" aria-hidden="true"></i> |
15 |
</a>
|
16 |
</li>
|
17 |
<li>
|
18 |
<a href="#0"> |
19 |
<span>Clients</span> |
20 |
<i class="fas fa-users" aria-hidden="true"></i> |
21 |
</a>
|
22 |
</li>
|
23 |
<li>
|
24 |
<a href="#0"> |
25 |
<span>Contact</span> |
26 |
<i class="fas fa-envelope-open-text" aria-hidden="true"></i> |
27 |
</a>
|
28 |
</li>
|
29 |
</ul>
|
30 |
</nav>
|
2. Specify the Main Styles
We’ll skip some introductory styles that you can examine by clicking on the CSS tab of the demo project and jump right into the main ones.
To begin with, we’ll give some styles to the nav that will make it look like this:



Next, as always, we’ll visually hide the checkbox. After that, we’ll place its related label, which will act as a button, at the center of the nav like this:



The corresponding styles are simple enough to go into more detail. Here they are:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.nav { |
4 |
position: relative; |
5 |
display: flex; |
6 |
justify-content: center; |
7 |
max-width: 400px; |
8 |
padding-bottom: 20px; |
9 |
border-radius: 5px 5px 25px 25px; |
10 |
margin: 300px auto 0; |
11 |
background: var(--white); |
12 |
box-shadow: rgb(50 50 93 / 10%) 0 30px 60px -12px, |
13 |
rgb(0 0 0 / 15%) 0 18px 36px -18px; |
14 |
}
|
15 |
|
16 |
.nav [type="checkbox"] { |
17 |
position: absolute; |
18 |
left: -9999px; |
19 |
}
|
20 |
|
21 |
.nav [type="checkbox"] + label { |
22 |
position: relative; |
23 |
width: 75px; |
24 |
height: 75px; |
25 |
display: flex; |
26 |
align-items: center; |
27 |
justify-content: center; |
28 |
font-size: 16px; |
29 |
cursor: pointer; |
30 |
z-index: 1; |
31 |
background: var(--violet); |
32 |
border-radius: 50%; |
33 |
transform: translateY(-50%); |
34 |
transition: all 0.2s; |
35 |
}
|
36 |
|
37 |
.nav [type="checkbox"] + label:hover { |
38 |
background: var(--dark-violet); |
39 |
}
|
3. The Menu Styles
Let’s now dive into the menu. Here are the important facts regarding its items’ styles:
- They will be absolutely positioned elements and initially sit underneath the label—we’ve given it
z-index: 1
. In such a way, they will look as being hidden.
- In terms of our microinteraction, we’ll use motion to indicate that the menu items have come from pressing the menu button. They’ll emerge from behind the button.
- We’ll assign them a different transition delay. Specifically, the fourth item will have the smallest one, then the third one, and so on. The bigger delay, the more time it’ll take for the associated item to disappear when the unchecked/closed state of the menu fires.
- They will be circular elements with the icon in their center and the text a few pixels above them. Initially, the text will be invisible.



The related styles:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.menu li { |
4 |
position: absolute; |
5 |
top: -25px; |
6 |
left: 50%; |
7 |
transform: translateX(-50%); |
8 |
transition: all 0.4s; |
9 |
}
|
10 |
|
11 |
.menu li:nth-child(1) { |
12 |
transition-delay: 0.2s; |
13 |
}
|
14 |
|
15 |
.menu li:nth-child(2) { |
16 |
transition-delay: 0.15s; |
17 |
}
|
18 |
|
19 |
.menu li:nth-child(3) { |
20 |
transition-delay: 0.1s; |
21 |
}
|
22 |
|
23 |
.menu li:nth-child(4) { |
24 |
transition-delay: 0.05s; |
25 |
}
|
26 |
|
27 |
.menu li a { |
28 |
width: 50px; |
29 |
height: 50px; |
30 |
border-radius: 50%; |
31 |
display: flex; |
32 |
align-items: center; |
33 |
justify-content: center; |
34 |
background: var(--violet); |
35 |
}
|
36 |
|
37 |
.menu li a span { |
38 |
position: absolute; |
39 |
top: 0; |
40 |
left: 0; |
41 |
transform: translateY(calc(-100% - 5px)); |
42 |
width: 100%; |
43 |
font-size: 13px; |
44 |
white-space: nowrap; |
45 |
pointer-events: none; |
46 |
opacity: 0; |
47 |
transition: opacity 0.3s; |
48 |
color: var(--black); |
49 |
font-weight: bold; |
50 |
}
|
4. Toggle Menu
Each time we click on the checkbox’s label, we’ll toggle the menu visibility. Once again, we’ll achieve this with the help of the :checked
pseudo-class, the adjacent sibling combinator (+
), and the general sibling combinator (~
).
We’ve already discussed the default closed menu state, so let’s now cover the sequence of actions during its opposite open state:
- We’ll give the label a different look to show it’s been pressed. This, again, is microinteraction feedback.



- The menu items will become visible smoothly by moving them upwards through the
top
andleft
offset values. But again, this will happen at different times. This time, the first item will appear first, then the second one, and so on.
- After all the elements come into view, the text nodes will appear simultaneously.



Here are the required styles:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.nav input:checked + label { |
4 |
background: var(--black); |
5 |
transform: translateY(calc(-50% + 4px)); |
6 |
}
|
7 |
|
8 |
.nav input:checked ~ .menu li:nth-child(1) { |
9 |
top: -210px; |
10 |
transition-delay: 0.1s; |
11 |
}
|
12 |
|
13 |
.nav input:checked ~ .menu li:nth-child(2) { |
14 |
top: -160px; |
15 |
left: calc(50% - 75px); |
16 |
transition-delay: 0.2s; |
17 |
}
|
18 |
|
19 |
.nav input:checked ~ .menu li:nth-child(3) { |
20 |
top: -160px; |
21 |
left: calc(50% + 75px); |
22 |
transition-delay: 0.3s; |
23 |
}
|
24 |
|
25 |
.nav input:checked ~ .menu li:nth-child(4) { |
26 |
top: -110px; |
27 |
transition-delay: 0.4s; |
28 |
}
|
29 |
|
30 |
.nav input:checked ~ .menu li a span { |
31 |
opacity: 1; |
32 |
transition-delay: 0.9s; |
33 |
}
|
Conclusion
Done! With just a few CSS rules and some straightforward markup, we managed to build a CSS-only animated menu microinteraction. This pattern might be beneficial if you need inspiration for implementing floating menus for secondary navigation or mobile screens.
Before closing, here’s a reminder of what we built:
This content originally appeared on Envato Tuts+ Tutorials and was authored by George Martsoukos
Today, we'll use the CSS checkbox hack technique to build an animated mobile menu; a useful microinteraction and an alternative UI to the main menu on mobile screens.
Without further ado, let’s preview the final demo:
What Are Microinteractions?
Microinteraction is a term heard often in UI and UX design these days. Microinteractions are trigger-feedback pairs, in other words where:
- something happens (by either a user doing something, or the system status changing)
- and then there’s feedback to show that something has happened.
The feedback is usually a small, highly contextual, visual indication in the UI. Today we’re going to build a mobile menu which, when clicked, makes menu items appear or disappear. We’ll use motion and color to give the user feedback.
CSS Animated Mobile Menu
1. Define the Page Markup
To kick things off we’ll define a nav
element where we'll place:
- A checkbox followed by their associated label and
- the main menu
Each menu item will include the text node wrapped inside a span
element and a Font Awesome icon. For this reason, we’ve already loaded the Font Awesome library in our pen.
1 |
<nav class="nav"> |
2 |
<input id="menu" type="checkbox"> |
3 |
<label for="menu">Menu</label> |
4 |
<ul class="menu"> |
5 |
<li>
|
6 |
<a href="#0"> |
7 |
<span>About</span> |
8 |
<i class="fas fa-address-card" aria-hidden="true"></i> |
9 |
</a>
|
10 |
</li>
|
11 |
<li>
|
12 |
<a href="#0"> |
13 |
<span>Projects</span> |
14 |
<i class="fas fa-tasks" aria-hidden="true"></i> |
15 |
</a>
|
16 |
</li>
|
17 |
<li>
|
18 |
<a href="#0"> |
19 |
<span>Clients</span> |
20 |
<i class="fas fa-users" aria-hidden="true"></i> |
21 |
</a>
|
22 |
</li>
|
23 |
<li>
|
24 |
<a href="#0"> |
25 |
<span>Contact</span> |
26 |
<i class="fas fa-envelope-open-text" aria-hidden="true"></i> |
27 |
</a>
|
28 |
</li>
|
29 |
</ul>
|
30 |
</nav>
|
2. Specify the Main Styles
We’ll skip some introductory styles that you can examine by clicking on the CSS tab of the demo project and jump right into the main ones.
To begin with, we’ll give some styles to the nav that will make it look like this:



Next, as always, we’ll visually hide the checkbox. After that, we’ll place its related label, which will act as a button, at the center of the nav like this:



The corresponding styles are simple enough to go into more detail. Here they are:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.nav { |
4 |
position: relative; |
5 |
display: flex; |
6 |
justify-content: center; |
7 |
max-width: 400px; |
8 |
padding-bottom: 20px; |
9 |
border-radius: 5px 5px 25px 25px; |
10 |
margin: 300px auto 0; |
11 |
background: var(--white); |
12 |
box-shadow: rgb(50 50 93 / 10%) 0 30px 60px -12px, |
13 |
rgb(0 0 0 / 15%) 0 18px 36px -18px; |
14 |
}
|
15 |
|
16 |
.nav [type="checkbox"] { |
17 |
position: absolute; |
18 |
left: -9999px; |
19 |
}
|
20 |
|
21 |
.nav [type="checkbox"] + label { |
22 |
position: relative; |
23 |
width: 75px; |
24 |
height: 75px; |
25 |
display: flex; |
26 |
align-items: center; |
27 |
justify-content: center; |
28 |
font-size: 16px; |
29 |
cursor: pointer; |
30 |
z-index: 1; |
31 |
background: var(--violet); |
32 |
border-radius: 50%; |
33 |
transform: translateY(-50%); |
34 |
transition: all 0.2s; |
35 |
}
|
36 |
|
37 |
.nav [type="checkbox"] + label:hover { |
38 |
background: var(--dark-violet); |
39 |
}
|
3. The Menu Styles
Let’s now dive into the menu. Here are the important facts regarding its items’ styles:
- They will be absolutely positioned elements and initially sit underneath the label—we’ve given it
z-index: 1
. In such a way, they will look as being hidden.
- In terms of our microinteraction, we’ll use motion to indicate that the menu items have come from pressing the menu button. They’ll emerge from behind the button.
- We'll assign them a different transition delay. Specifically, the fourth item will have the smallest one, then the third one, and so on. The bigger delay, the more time it'll take for the associated item to disappear when the unchecked/closed state of the menu fires.
- They will be circular elements with the icon in their center and the text a few pixels above them. Initially, the text will be invisible.



The related styles:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.menu li { |
4 |
position: absolute; |
5 |
top: -25px; |
6 |
left: 50%; |
7 |
transform: translateX(-50%); |
8 |
transition: all 0.4s; |
9 |
}
|
10 |
|
11 |
.menu li:nth-child(1) { |
12 |
transition-delay: 0.2s; |
13 |
}
|
14 |
|
15 |
.menu li:nth-child(2) { |
16 |
transition-delay: 0.15s; |
17 |
}
|
18 |
|
19 |
.menu li:nth-child(3) { |
20 |
transition-delay: 0.1s; |
21 |
}
|
22 |
|
23 |
.menu li:nth-child(4) { |
24 |
transition-delay: 0.05s; |
25 |
}
|
26 |
|
27 |
.menu li a { |
28 |
width: 50px; |
29 |
height: 50px; |
30 |
border-radius: 50%; |
31 |
display: flex; |
32 |
align-items: center; |
33 |
justify-content: center; |
34 |
background: var(--violet); |
35 |
}
|
36 |
|
37 |
.menu li a span { |
38 |
position: absolute; |
39 |
top: 0; |
40 |
left: 0; |
41 |
transform: translateY(calc(-100% - 5px)); |
42 |
width: 100%; |
43 |
font-size: 13px; |
44 |
white-space: nowrap; |
45 |
pointer-events: none; |
46 |
opacity: 0; |
47 |
transition: opacity 0.3s; |
48 |
color: var(--black); |
49 |
font-weight: bold; |
50 |
}
|
4. Toggle Menu
Each time we click on the checkbox’s label, we'll toggle the menu visibility. Once again, we’ll achieve this with the help of the :checked
pseudo-class, the adjacent sibling combinator (+
), and the general sibling combinator (~
).
We’ve already discussed the default closed menu state, so let’s now cover the sequence of actions during its opposite open state:
- We’ll give the label a different look to show it’s been pressed. This, again, is microinteraction feedback.



- The menu items will become visible smoothly by moving them upwards through the
top
andleft
offset values. But again, this will happen at different times. This time, the first item will appear first, then the second one, and so on.
- After all the elements come into view, the text nodes will appear simultaneously.



Here are the required styles:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.nav input:checked + label { |
4 |
background: var(--black); |
5 |
transform: translateY(calc(-50% + 4px)); |
6 |
}
|
7 |
|
8 |
.nav input:checked ~ .menu li:nth-child(1) { |
9 |
top: -210px; |
10 |
transition-delay: 0.1s; |
11 |
}
|
12 |
|
13 |
.nav input:checked ~ .menu li:nth-child(2) { |
14 |
top: -160px; |
15 |
left: calc(50% - 75px); |
16 |
transition-delay: 0.2s; |
17 |
}
|
18 |
|
19 |
.nav input:checked ~ .menu li:nth-child(3) { |
20 |
top: -160px; |
21 |
left: calc(50% + 75px); |
22 |
transition-delay: 0.3s; |
23 |
}
|
24 |
|
25 |
.nav input:checked ~ .menu li:nth-child(4) { |
26 |
top: -110px; |
27 |
transition-delay: 0.4s; |
28 |
}
|
29 |
|
30 |
.nav input:checked ~ .menu li a span { |
31 |
opacity: 1; |
32 |
transition-delay: 0.9s; |
33 |
}
|
Conclusion
Done! With just a few CSS rules and some straightforward markup, we managed to build a CSS-only animated menu microinteraction. This pattern might be beneficial if you need inspiration for implementing floating menus for secondary navigation or mobile screens.
Before closing, here’s a reminder of what we built:
As always, thanks a lot for reading!
This content originally appeared on Envato Tuts+ Tutorials and was authored by George Martsoukos
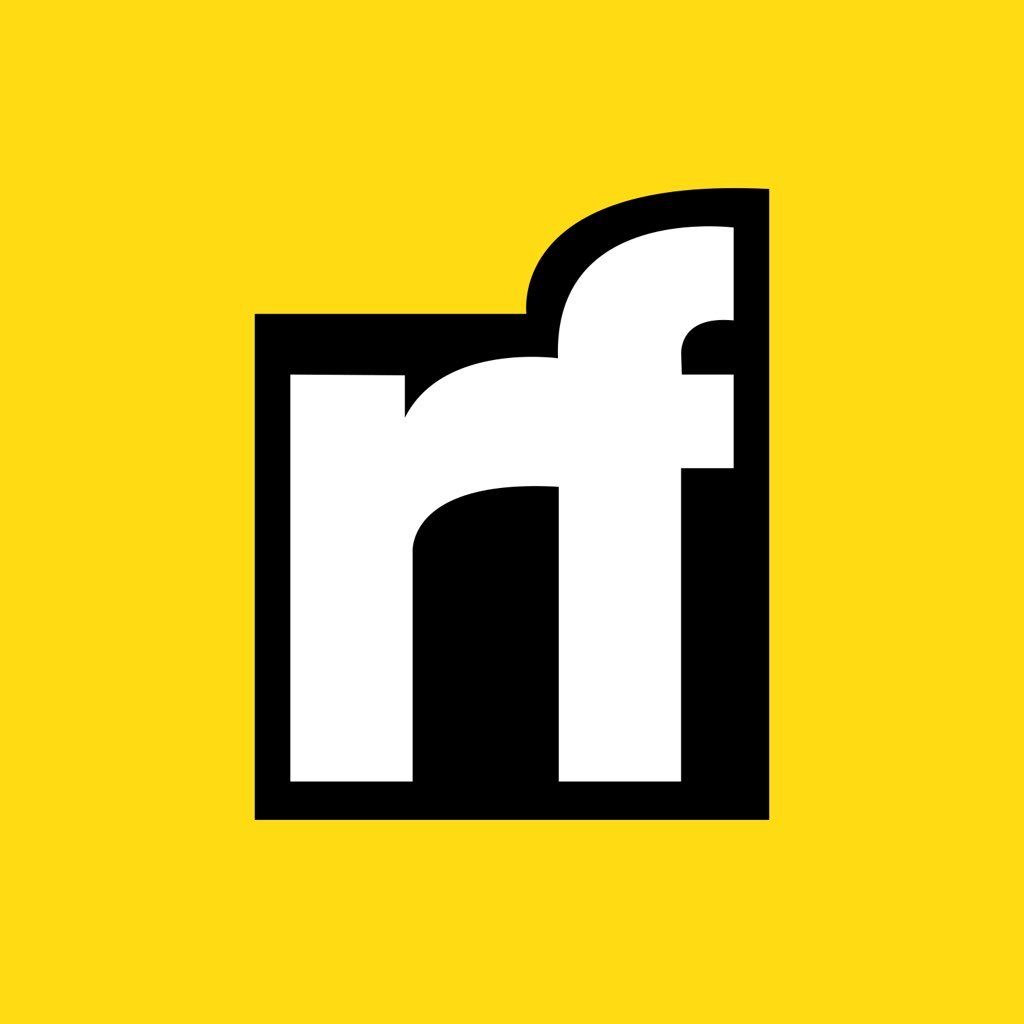
George Martsoukos | Sciencx (2023-03-19T13:42:04+00:00) How to Build a Mobile Menu Microinteraction With CSS. Retrieved from https://www.scien.cx/2023/03/19/how-to-build-a-mobile-menu-microinteraction-with-css/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.