This content originally appeared on DEV Community and was authored by Uyai-Abasi
Hello and welcome to my first article! As a tech newbie myself, I understand how daunting it can be to dive into the world of technology. But fear not! This article is designed to provide you with a basic introduction to the topic at hand, and I hope it will help you gain a better understanding of the subject.
So sit back, relax, and get ready to learn something new. Let's dive into the fascinating world of technology together!
In programming, string manipulation is a common task that is required to achieve various objectives. One such task is to reverse a string. A string reversal means to change the order of the characters in a string. For instance, reversing the string "Hello" will give "olleH". In this article, we will discuss how to reverse a string in JavaScript.
There are several ways to reverse a string in JavaScript. We will discuss some of the most commonly used methods below.
- Using the split(), reverse(), and join() methods This is the most commonly used method to reverse a string in JavaScript. It involves splitting the string into an array of characters, reversing the array, and then joining the array back into a string. Here's how to do it:
const str = "Hello";
const reversedStr = str.split("").reverse().join("");
console.log(reversedStr);
The split() method splits the string into an array of characters, the reverse() method reverses the order of the elements in the array, and the join() method joins the elements of the array back into a string.
- Using a for loop
Another way to reverse a string is to use a for loop. This method involves iterating through the string from the last character to the first character and concatenating the characters into a new string.
Here's how to do it:
const str = "Hello";
let reversedStr = "";
for (let i = str.length - 1; i >= 0; i--) {
reversedStr += str[i];
}
console.log(reversedStr);
This method initializes an empty string and then iterates through the original string from the last character to the first character using a for loop. In each iteration, the current character is concatenated to the new string.
- Using recursion
Another way to reverse a string is to use recursion. This method involves calling a function recursively to reverse the string.
Here's how to do it:
function reverseString(str) {
if (str === "") {
return "";
} else {
return reverseString(str.substr(1)) + str.charAt(0);
}
}
console.log(reverseString("Hello"));
This method checks if the string is empty. If it is, it returns an empty string. If it is not empty, it calls the function recursively with the substring starting from the second character and concatenates the first character to the end.
In conclusion, there are several ways to reverse a string in JavaScript. The most commonly used method involves using the split(), reverse(), and join() methods. Other methods include using a for loop and recursion. Each method has its advantages and disadvantages, and the best method to use depends on the specific requirements of the project.
Thank you for reading to this point, I hope this was helpful in some way.
This content originally appeared on DEV Community and was authored by Uyai-Abasi
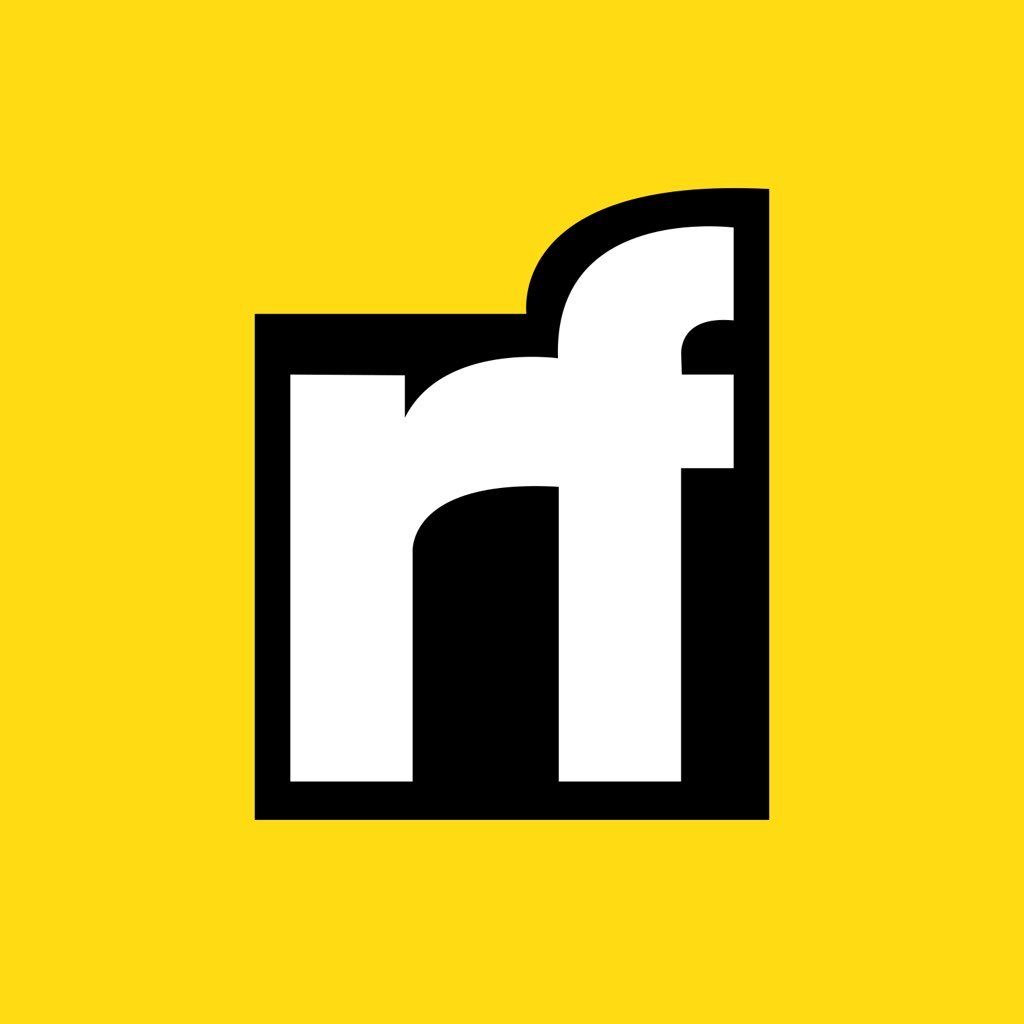
Uyai-Abasi | Sciencx (2023-03-27T11:07:25+00:00) How to Reverse string in JavaScript. Retrieved from https://www.scien.cx/2023/03/27/how-to-reverse-string-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.