This content originally appeared on Level Up Coding - Medium and was authored by Gabriel Borges
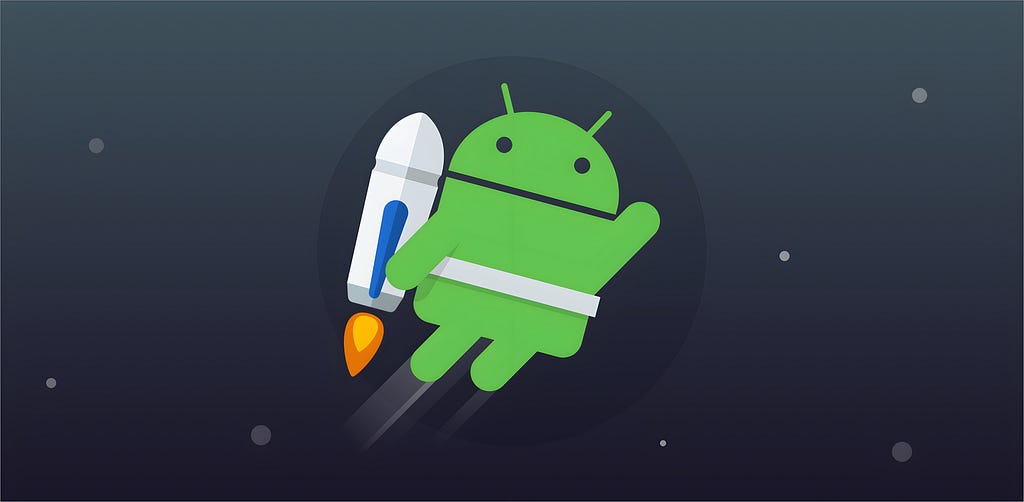
Simplify In-App Navigation and Enhance User Experience
The Android Jetpack Navigation Architecture is an essential component for modern Android applications, enabling developers to create seamless navigation experiences. This article aims to provide a simple yet comprehensive guide to understanding and implementing Jetpack Navigation in your Android projects.
If you’re interested in exploring more Android Jetpack libraries, check out our Android Jetpack Mastery list. In this list, we cover various Jetpack libraries in-depth, ensuring you have the knowledge to build amazing Android applications. Now, let’s dive into Jetpack Navigation!
1. Overview of Jetpack Navigation
Jetpack Navigation is composed of three main components:
- Navigation Graph: An XML resource that represents the navigation structure of your app
- NavController: A class responsible for managing app navigation within a NavHost
- NavHostFragment: A container for NavController that displays the destinations from your navigation graph
The benefits of using Jetpack Navigation include reduced boilerplate code, consistent navigation patterns, and enhanced deep linking support.
2. Setting Up Jetpack Navigation
To use Jetpack Navigation in your project, you’ll need to add the necessary dependencies to your app’s build.gradle file:
dependencies {
implementation "androidx.navigation:navigation-fragment-ktx:2.5.3"
implementation "androidx.navigation:navigation-ui-ktx:2.5.3"
}
After adding the dependencies, sync your project with Gradle.
3. Creating a Navigation Graph
The Navigation Graph is an XML resource that represents your app’s navigation structure. To create a Navigation Graph:
- Right click your res directory, selecte "New" > "Android Resource File".
- Name your file nav_graph.xml and select "navigation" as a resource type.
- Your navigation file will be created inside the res/navigation folder.
- Open nav_graph.xml in the Navigation Editor to visually manage your app's destinations and actions.
Destinations represent individual screens, while actions define the transitions between these screens.
4. Integrating NavController and NavHostFragment
To manage app navigation, integrate NavController and NavHostFragment into your activity or fragment.
In your activity’s XML layout file, add the NavHostFragment:
Then, in your activity’s file, you can retrieve the NavController using the findNavController(viewId) method.
5. Navigating Between Destinations
Before diving into navigation, let’s create two empty fragments called HomeFragment and PageFragment. These fragments will serve as the destinations for our example navigation scenario.
5.1 Adding destinations and actions
After creating the 2 empty fragments, define both in the navigation graph xml and set HomeFragment as the start destination:
<?xml version="1.0" encoding="utf-8"?>
<navigation xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/nav_graph"
app:startDestination="@id/homeFragment"> <!-- Add homeFragment -->
<!-- Add fragment in the navigation graph-->
<fragment
android:id="@+id/homeFragment"
android:name="com.gborges9.navigation_example.HomeFragment"
android:label="fragment_home"
tools:layout="@layout/fragment_home" />
<!-- Add fragment in the navigation graph-->
<fragment
android:id="@+id/pageFragment"
android:name="com.gborges9.navigation_example.PageFragment"
android:label="fragment_page"
tools:layout="@layout/fragment_page" />
</navigation>
You will also need to define an "Action" from HomeFragment to PageFragment:
<fragment
android:id="@+id/homeFragment"
android:name="com.gborges9.navigation_example.HomeFragment"
android:label="fragment_home"
tools:layout="@layout/fragment_home" >
<!-- Add an action -->
<action
android:id="@+id/action_homeFragment_to_pageFragment"
app:destination="@id/pageFragment" />
</fragment>
You can also do the same by using the "design" view of the navigation fragment.
5.2 Modifying HomeFragment layout
- In the HomeFragment layout file, add a Button that will be used to navigate to PageFragment:
2. In HomeFragment's Kotlin file, set up the navigation logic. First, set a click listener to the button, use findNavController() to get access to the NavController and then call navigate(id) with an destination id or an action id:
With these steps, you have set up a simple navigation scenario. When the button in HomeFragment is clicked, the app will navigate to PageFragment, if you press the back button, the app will handle the navigate back automatically.
6. Handling Deep Links
To set up deep linking:
- Add deep link information to the corresponding action in the Navigation Graph:
<action
android:id="@+id/action_destinationA_to_destinationB"
app:destination="@id/destinationB">
<deepLink
app:uri="example://destinationB/{data}" />
</action>
2. Retrieve the data from the deep link:
val data: String = args.data
3. Declare deep links in your AndroidManifest.xml:
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.VIEW" />
<category android:name="android.intent.category.DEFAULT" />
<category android:name="android.intent.category.BROWSABLE" />
<data android:scheme="example" />
</intent-filter>
</activity>
7. Customizing Navigation UI
To integrate Jetpack Navigation with Android UI components, such as BottomNavigationView, DrawerLayout, and Toolbar:
NavigationUI.setupActionBarWithNavController(activity, navController)
NavigationUI.setupWithNavController(activity, drawerLayout)
NavigationUI.setupWithNavController(activity, bottomNavBar)
Also, if you use a drawer, navigation is handled automatically if you set the id of a menu item the same as the action or destination id.
To customize navigation transitions and animations, define custom animations in your Navigation Graph:
<action
android:id="@+id/action_destinationA_to_destinationB"
app:destination="@id/destinationB"
app:enterAnim="@anim/slide_in_right"
app:exitAnim="@anim/slide_out_left"
app:popEnterAnim="@anim/slide_in_left"
app:popExitAnim="@anim/slide_out_right" />
8. Passing Data Between Destinations
One of the most common tasks in app development is passing data between destinations. Jetpack Navigation simplifies this process using Safe Args, a Gradle plugin that generates code for type-safe argument passing.
To use Safe Args, follow these steps:
- Add the Safe Args dependencies to your project level build.gradle file:
buildscript {
repositories {
google()
}
dependencies {
classpath("androidx.navigation:navigation-safe-args-gradle-plugin:2.5.3")
}
}
...
2. Add the Safe Args dependencies to your app level build.gradle file:
plugins {
id("androidx.navigation.safeargs.kotlin")
}
3. Define arguments in your Navigation Graph:
...
<fragment
android:id="@+id/pageFragment"
android:name="com.gborges9.navigation_example.PageFragment"
android:label="fragment_page"
tools:layout="@layout/fragment_page">
<argument
android:name="data"
app:argType="string" />
</fragment>
4. After building/making your project, Android Studio will generate a Directions class that you can use to easily create actions from any destination. In HomeFragment, modify your navigation code:
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
view.findViewById<Button>(R.id.navigate_to_page_btn).setOnClickListener {
val homeToPageAction = HomeFragmentDirections
.actionHomeFragmentToPageFragment("Hello world!")
findNavController().navigate(homeToPageAction)
}
}
5. In your target destination PageFragment, retrieve the passed data using the generated Args class:
val args: PageFragmentArgs by navArgs()
// Access any argument defined in the nav graph (e.g. args.data)
By following these steps, you can pass data between destinations in a type-safe and efficient manner using Jetpack Navigation’s Safe Args plugin.
9. Best Practices and Tips
- Modularize your navigation: Divide your Navigation Graph into smaller, feature-specific graphs to improve maintainability.
- Test your navigation: Use the androidx.navigation.testing library to test your navigation logic.
- Ensure accessibility: Implement accessible navigation patterns and provide descriptive labels for screen readers.
- If you want to do something when the destination changes, implement an NavController.OnDestinationChangedListener and add it to your controller with navController.addOnDestinationChangedListener(listener).
Conclusion
Jetpack Navigation is a powerful tool for creating consistent, seamless navigation experiences in Android applications. By understanding the core components and following best practices, you can improve your app’s usability and maintainability. We encourage you to experiment with Jetpack Navigation in your projects and share your experiences with the Android development community.
If you want to take a look at the full source code of the app we built during this tutorial, check out our repository on GitHub, where we implement everything discussed in the article. Feel free to clone, explore, and learn from this project as you follow along with the tutorial.
GitHub - gborges9/android_jetpack_navigation
If you found this article helpful, be sure to check out our comprehensive list of Jetpack library articles on Medium. We cover a wide range of topics, including LiveData, DataStore and more. Our goal is to provide you with a deep understanding of Android Jetpack libraries and help you create robust, high-quality apps. Don’t miss out on these valuable resources!
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Mastering Android Jetpack Navigation: A Comprehensive Guide was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Gabriel Borges
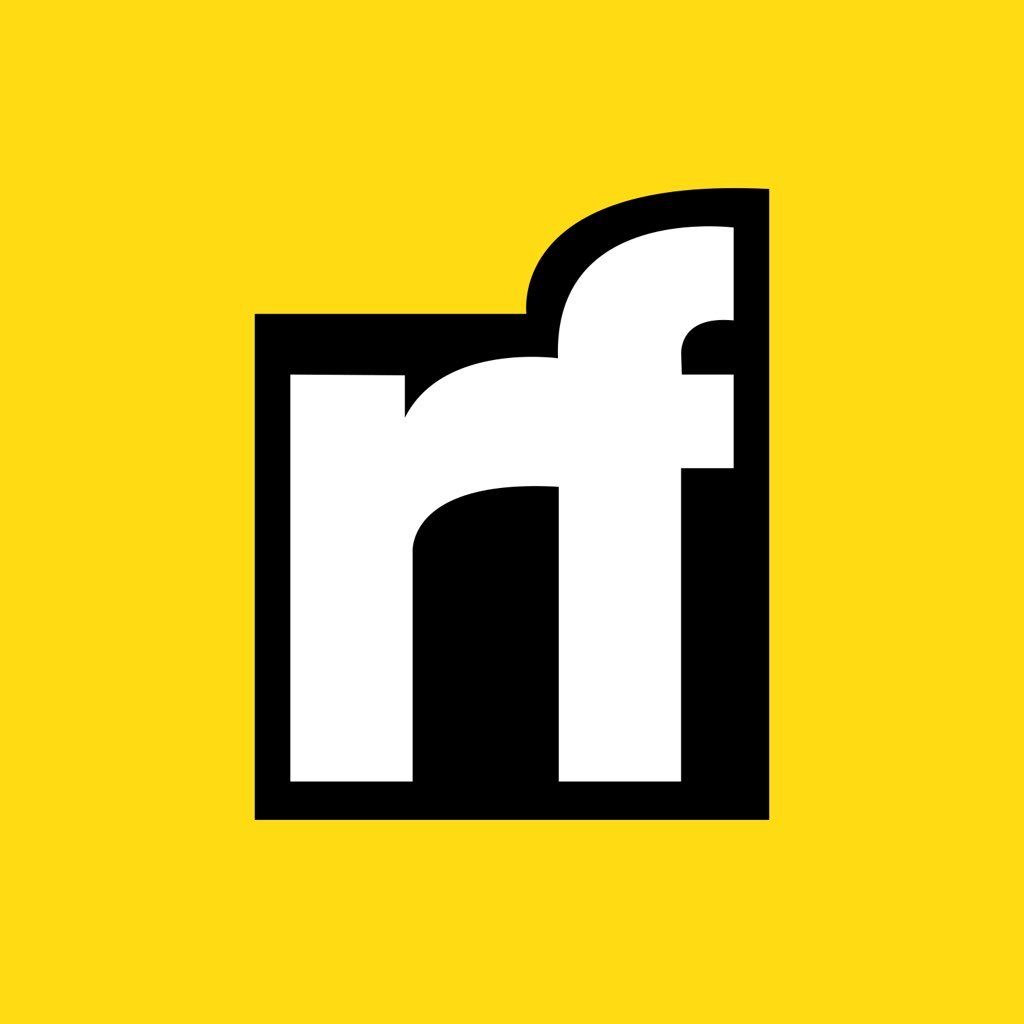
Gabriel Borges | Sciencx (2023-03-27T00:45:20+00:00) Mastering Android Jetpack Navigation: A Comprehensive Guide. Retrieved from https://www.scien.cx/2023/03/27/mastering-android-jetpack-navigation-a-comprehensive-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.