This content originally appeared on Bits and Pieces - Medium and was authored by FardaKarimov
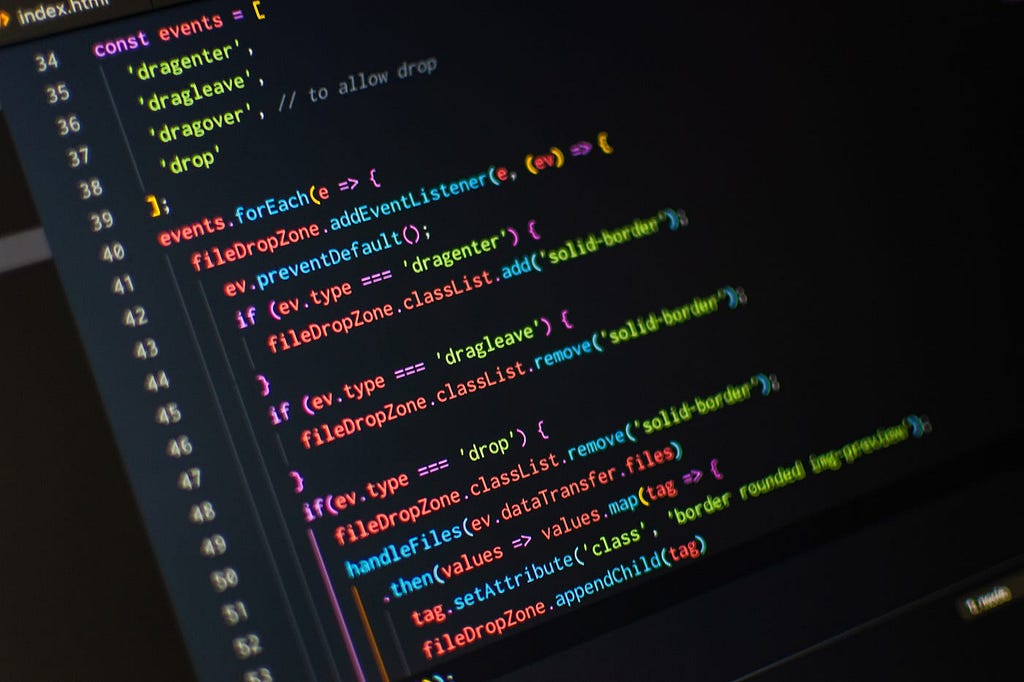
TypeScript offers numerous advantages such as type checking, better code completion, and easier debugging, among others. As a React developer, you might be familiar with the basics of TypeScript, but there are several advanced techniques that you can use to take your TypeScript skills to the next level. In this article, we’ll explore some of these advanced TypeScript techniques and provide code examples to help you understand how to implement them.
1. Type Guards
TypeScript provides a mechanism called “Type Guards” to help you narrow down the type of a variable based on its value. This technique can be useful when you have a union type that could have multiple possible types. For example, consider the following code:
type MyType = string | number;
function doSomething(value: MyType) {
if (typeof value === "string") {
console.log("Value is a string:", value);
} else {
console.log("Value is a number:", value);
}
}
In this example, we have a union type MyType that can be either a string or a number. Inside the doSomething function, we use a type guard to check the type of value. If it’s a string, we log a message saying that it’s a string. If it’s a number, we log a message saying that it’s a number. This technique can be particularly useful when working with React components that accept different types of props.
2. Conditional Types
Conditional types allow you to create a type that depends on a condition. You can use this technique to create more complex types that would be difficult to express otherwise. For example, consider the following code:
type MyType<T> = T extends string ? string : number;
const value1: MyType<string> = "hello";
const value2: MyType<number> = 123;
console.log(value1); // "hello"
console.log(value2); // 123
In this example, we define a generic type MyType that takes a type parameter T. We use a conditional type to check whether T extends string. If it does, the type is set to string. If it doesn’t, the type is set to number. We then create two variables value1 and value2 that use the MyType type with different type parameters. Finally, we log the values of value1 and value2 to the console.
3. Mapped Types
Mapped types allow you to create a new type based on an existing type by transforming each property in the existing type. You can use this technique to create new types that share properties with existing types, but with different values or types. For example, consider the following code:
interface MyInterface {
name: string;
age: number;
address: string;
}
type MyNewType = {
[K in keyof MyInterface]: string;
}
const myObject: MyNewType = {
name: "John",
age: "30",
address: "123 Main St."
}
console.log(myObject); // { name: "John", age: "30", address: "123 Main St." }
In this example, we have an interface MyInterface with three properties: name, age, and address. We then define a new type MyNewType using a mapped type. The keyof keyword is used to get the keys of the MyInterface type, and then we use a for...in loop to iterate over each property in MyInterface. For each property, we set its type to string. This creates a new type that has the same properties as MyInterface, but with their types set to string.
We then create an object myObject that uses the MyNewType type. Since the type of each property in MyNewType is set to string, we can assign string values to each property. Finally, we log the value of myObject to the console.
4. TypeScript Decorators
TypeScript decorators provide a way to add metadata to classes, properties, and methods. Decorators are functions that are executed at runtime and can modify the behavior of the target they are applied to. You can use decorators to add features to your code that would be difficult to implement otherwise. For example, consider the following code:
function log(target: any, key: string, descriptor: PropertyDescriptor) {
const originalMethod = descriptor.value;
descriptor.value = function (...args: any[]) {
console.log(Calling method ${ key } with args: ${ args });
return originalMethod.apply(this, args);
}
return descriptor;
}
class MyClass {
@log
myMethod(arg1: string, arg2: number) {
console.log(Inside myMethod with args: ${ arg1 }, ${ arg2 });
}
}
const myObject = new MyClass();
myObject.myMethod("hello", 123);
In this example, we define a decorator function log that takes three parameters: target, key, and descriptor. The target parameter refers to the class that the decorator is applied to, the key parameter refers to the name of the method that the decorator is applied to, and the descriptor parameter refers to the property descriptor of the method.
Inside the log decorator function, we save a reference to the original method using the PropertyDescriptor.value property. We then modify the descriptor.value property to add logging functionality before calling the original method with its arguments using Function.apply().
We then apply the log decorator to the myMethod method of the MyClass class using the @log syntax. This will modify the behavior of the myMethod method to log its arguments before calling the original method.
Finally, we create an instance of the MyClass class and call its myMethod method with some arguments. The log decorator will log the arguments before calling the original method.
5. TypeScript Mixins
TypeScript mixins allow you to create reusable code that can be mixed into different classes. Mixins are a way to share behavior between classes without using inheritance. You can use mixins to add functionality to your code that can be easily reused in different contexts. For example, consider the following code:
class MyMixin {
mixinMethod() {
console.log("Mixin method called");
}
}
class MyClass implements MyMixin {
mixinMethod: () => void;
constructor() {
const mixin = new MyMixin();
this.mixinMethod = mixin.mixinMethod.bind(this);
}
}
const myObject = new MyClass();
myObject.mixinMethod();
In this example, we define a mixin class MyMixin that has a mixinMethod method. We then define a MyClass class that implements the MyMixin class using the implements keyword.
Inside the constructor of the MyClass class, we create an instance of the MyMixin class and bind its mixinMethod method to the MyClass instance using Function.bind(). This makes the mixinMethod method available as a method of the MyClass instance.
Finally, we create an instance of the MyClass class and call its mixinMethod method. The mixinMethod method is called, and the message “Mixin method called” is logged to the console.
💡 Along with these TypeScript techniques you could also use an open-source toolchain such as Bit to further improve your React development workflow. With Bit you can easily share, discover, and reuse individual components across different projects, which can significantly reduce development time, and make your codebase modular, scalable and maintainable. This guide will show you how.
Find out more:
Sharing Types Between Your Frontend and Backend Applications
Conclusion
In this article, we’ve explored some advanced TypeScript techniques that can be useful for senior React developers. Type guards allow you to narrow down the type of a variable based on a condition, while decorators provide a way to add metadata and modify the behavior of classes, properties, and methods at runtime. Mixins allow you to create reusable code that can be mixed into different classes.
By leveraging these advanced techniques, you can write more flexible, maintainable, and scalable code in your React applications. Whether you’re working on a small personal project or a large enterprise application, these techniques can help you take your TypeScript skills to the next level and build better software.
Build React Apps with reusable components, just like Lego
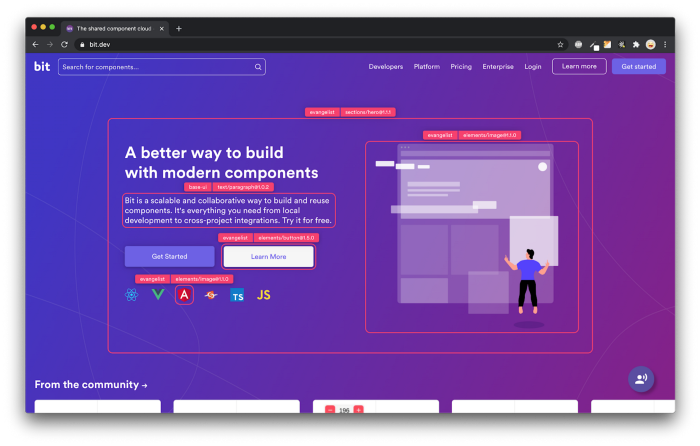
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Creating a Developer Website with Bit components
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
TypeScript Techniques for Optimal React Development was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by FardaKarimov
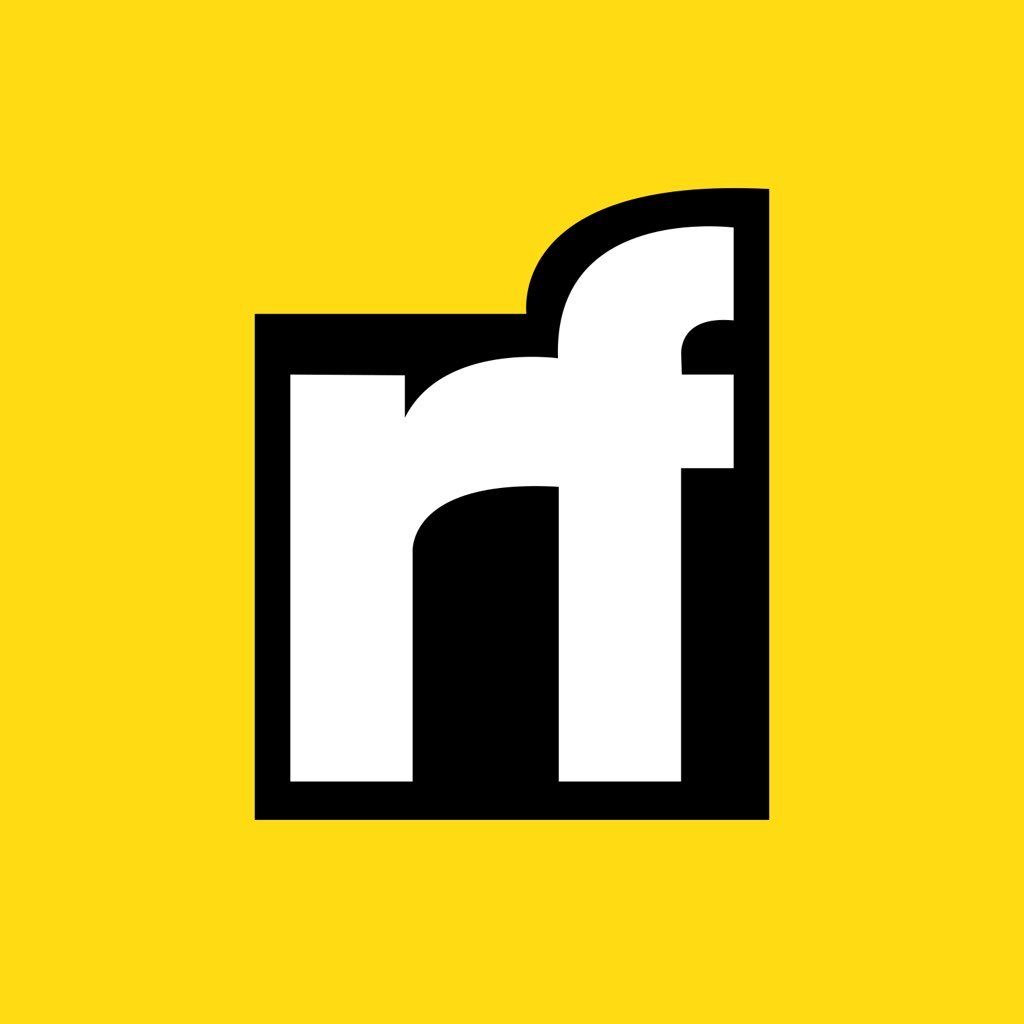
FardaKarimov | Sciencx (2023-03-29T06:02:28+00:00) TypeScript Techniques for Optimal React Development. Retrieved from https://www.scien.cx/2023/03/29/typescript-techniques-for-optimal-react-development/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.