This content originally appeared on Level Up Coding - Medium and was authored by Ulrik Thyge Pedersen
Risk of Rust — Part 1: Introduction and Getting Started
Introduction to Rust — A Beginner’s Guide to Installing and Setting Up Rust on Your System
Explore “Risk of Rust,” a series on the modern programming language Rust that combines speed and safety for writing efficient and resilient code. From syntax basics to advanced topics like concurrency and memory management, we’ll guide you through practical examples and real-world applications in game development, web development, and operating systems. Whether you’re new to programming or experienced, learn how Rust can help you write safe and fast code for complex systems.
Introduction
Welcome to the “Risk of Rust” article series, where we explore the modern programming language Rust that combines speed and safety for writing efficient and resilient code. In this first article, we’ll introduce you to Rust and guide you through the installation process on different operating systems. Whether you’re new to programming or an experienced developer, Rust is a language worth learning.
Rust is a relatively new programming language that was created with a focus on speed, safety, and concurrency. Since its release in 2010, Rust has gained popularity in the industry, and is now used by companies such as Mozilla, Microsoft, Dropbox, and Cloudflare. Rust is also used in a variety of open-source projects, including the popular web browser engine Servo, the file indexing system Tantivy, and the game engine Amethyst.
One of the reasons for Rust’s popularity is its ability to provide memory safety guarantees without sacrificing performance. This makes Rust a great choice for building systems software, web applications, and games, where both speed and reliability are critical. Additionally, Rust’s strong type system and pattern matching capabilities make it easier to write correct code, even in large and complex codebases.
In this article, we’ll cover the basics of Rust, including its syntax, data types, and fundamental concepts. We’ll also show you how to install Rust on your computer and set up your development environment. By the end of the article, you’ll have a good understanding of what Rust is and how to start using it to write safe and fast code. Let’s get started!
Getting Started with Rust
Rust has great documentation and an active community, making it easy to get started. Here are the steps to install Rust and set up your development environment:
Downloading Rust
You can download Rust from the official website: https://www.rust-lang.org/. The website provides installers for Windows, macOS, and Linux, and it also provides instructions for installing Rust using package managers.
Installing Rust on Windows
If you’re using Windows, you can download the Rust installer and run it. The installer will guide you through the installation process, and it will add Rust to your PATH environment variable so you can run Rust from anywhere in the terminal.
Installing Rust on macOS and Linux
If you’re using macOS or Linux, you can install Rust using the following command in the terminal:
This command will download the Rust installation script and run it, guiding you through the installation process.
Setting up Rust for development
After you’ve installed Rust, you’ll need to set up your development environment. Rust comes with a package manager called Cargo, which makes it easy to manage dependencies and build your project. You can create a new Rust project using the following command in the terminal:
This will create a new directory called “my_project” with a basic Rust project structure. You can then navigate into the directory and start editing the code.
Understanding Rust’s package manager — Cargo
Cargo is Rust’s package manager, which makes it easy to manage dependencies and build your project. You can use Cargo to install dependencies by adding them to your Cargo.toml file, and you can build your project using the cargo build command.
Here’s an example Cargo.toml file:
This file specifies the name, version, and dependencies of your project. You can install the dependencies by running cargo build, and Cargo will automatically download and install them for you.
Now that you have Rust installed and your development environment set up, let’s dive into the basics of Rust syntax and data types in the next section!
Rust Basics
Rust is a statically typed language that emphasizes safety and performance. Rust has a strong and expressive type system that prevents common programming errors such as null pointer dereferences and buffer overflows. In this section, we’ll cover the basics of Rust syntax and data types.
Hello, World!
Let’s start with the classic “Hello, World!” program in Rust:
This program defines a function called main, which is the entry point of a Rust program. The function calls the println! macro, which prints the string "Hello, world!" to the console.
Variables and Data Types
In Rust, you declare variables using the let keyword. Rust has several built-in data types, including integers, floating-point numbers, booleans, and characters. Here are some examples:
In Rust, you must specify the type of a variable when you declare it. Rust also has type inference, which means that the compiler can infer the type of a variable based on its usage.
Control Flow
Rust has several control flow constructs, including if expressions, for loops, and match expressions. Here are some examples:
The if expression checks whether x is negative, positive, or zero. The for loop iterates over the elements of an array. The match expression matches the value of x against several patterns.
References and Ownership
One of the unique features of Rust is its ownership system, which allows for safe and efficient memory management. In Rust, every value has an owner, and there can only be one owner at a time. If you want to borrow a value without taking ownership, you can use references. Here are some examples:
In the first example, we create a reference y to the value of x, and we use the * operator to dereference y and print the value of x. In the second example, we create a mutable reference y to the value of x, and we use *y to modify the value of x. In the third example, we create a vector v and use the iter() method to create an iterator over its elements. We then use a for loop to iterate over the elements and print them.
As you can see, Rust’s ownership and borrowing system can take some getting used to, but it is one of the key features that make Rust a safe and efficient language. In the next section, we will cover Rust’s data types and how they can be used in your code.
These are just some of the basic concepts in Rust that you will need to know as you start coding in the language. For more information on these concepts, and others not mentioned here, you can refer to the Rust documentation, which is an excellent resource for learning the language.
Conclusion
In this article, we covered the basics of Rust and how to install the language on different operating systems. We also went over some of the key concepts of Rust such as ownership, borrowing and control flow.
Now that you have a solid understanding of the basics, you are ready to move on to the next article in our series where we will cover Rust’s syntax, data types, and control flow in more detail. This will provide you with a deeper understanding of the language, and allow you to start writing Rust code on your own.
Stay tuned for the next article, where we will dive deeper into Rust’s syntax and explore more advanced concepts in the language. In the meantime, feel free to explore Rust’s documentation and try writing some code on your own.
Thank you for reading my story!
Subscribe for free to get notified when I published a new story!
Find me on LinkedIn and Kaggle!
…and I would love your feedback!
Risk of Rust: Introduction and Getting Started was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Ulrik Thyge Pedersen
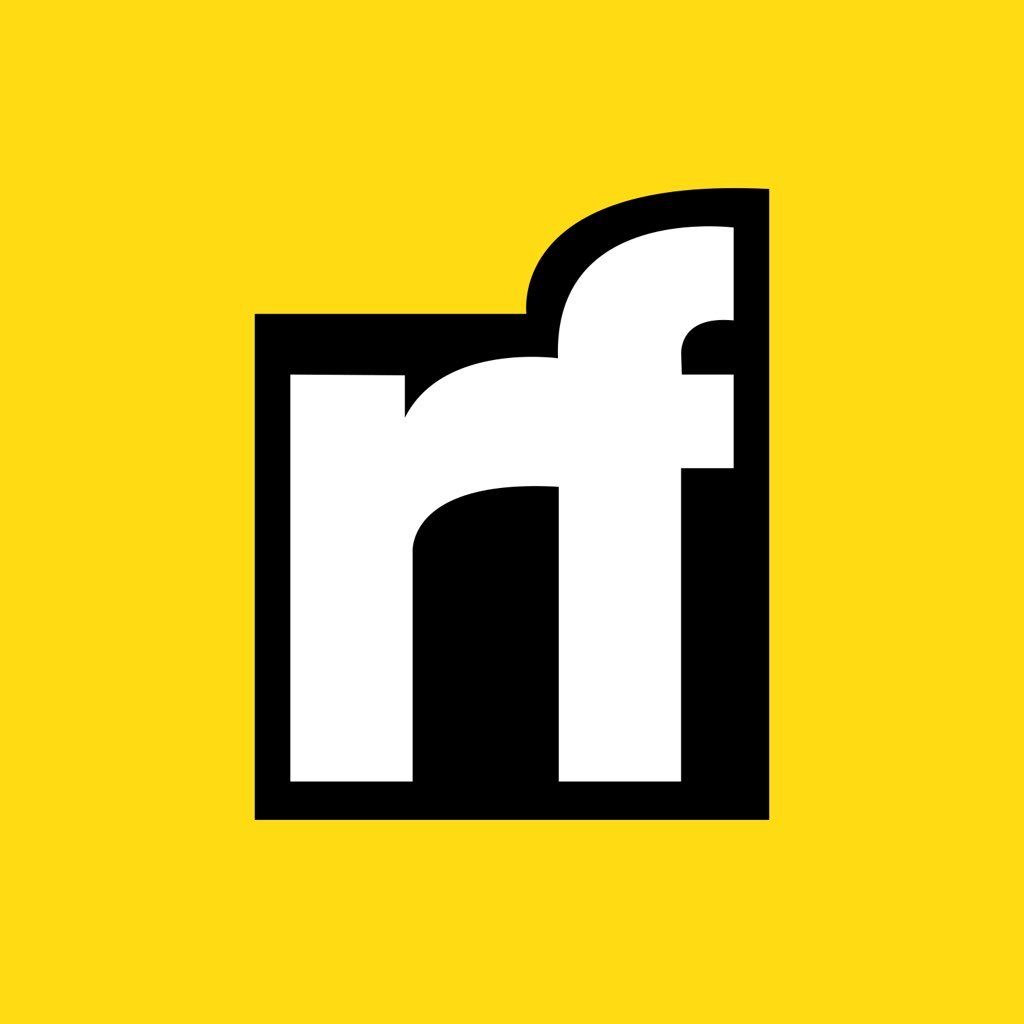
Ulrik Thyge Pedersen | Sciencx (2023-04-06T02:46:07+00:00) Risk of Rust: Introduction and Getting Started. Retrieved from https://www.scien.cx/2023/04/06/risk-of-rust-introduction-and-getting-started/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.