This content originally appeared on CodeSource.io and was authored by Deven
Dev Bytes: The Validated DTO package for Laravel simplifies handling Data Transfer Objects (DTOs) with built-in validation and type-casting.
It allows centralized validation, similar to Laravel Request validation, and supports custom type casters. The package is easily integrated with existing projects and offers comprehensive documentation.
The validated DTO package for Laravel is an excellent tool for managing Data Transfer Objects (DTOs) with built-in validation and type-casting.
In situations where your application relies on DTOs for exchanging data between systems, this package allows for streamlined validation of incoming request data, much like how you would typically validate request data:
class UserDTO extends ValidatedDTO
{
/**
* @return array
*/
protected function rules(): array
{
return [
'name' => ['required', 'string'],
'email' => ['required', 'email'],
'password' => [
'required',
Password::min(8)
->mixedCase()
->letters()
->numbers()
->symbols()
->uncompromised(),
],
];
}
}
The Validated DTO package for Laravel offers:
- Easy integration into existing projects
- Data validation similar to Laravel Request validation
- Simplified custom validation messages
- Typed property support
- Built-in Type Casting for DTO properties
- Nested datacasting capabilities
- Custom Type Caster creation
One particularly useful feature is the ability to create a DTO instance directly from the request object, as demonstrated below:
public function store(Request $request): JsonResponse
{
$dto = UserDTO::fromRequest($request);
}
Refer to the detailed documentation for further information, setup instructions, and usage examples. The source code is available on GitHub at laravel-validated-dto.
This content originally appeared on CodeSource.io and was authored by Deven
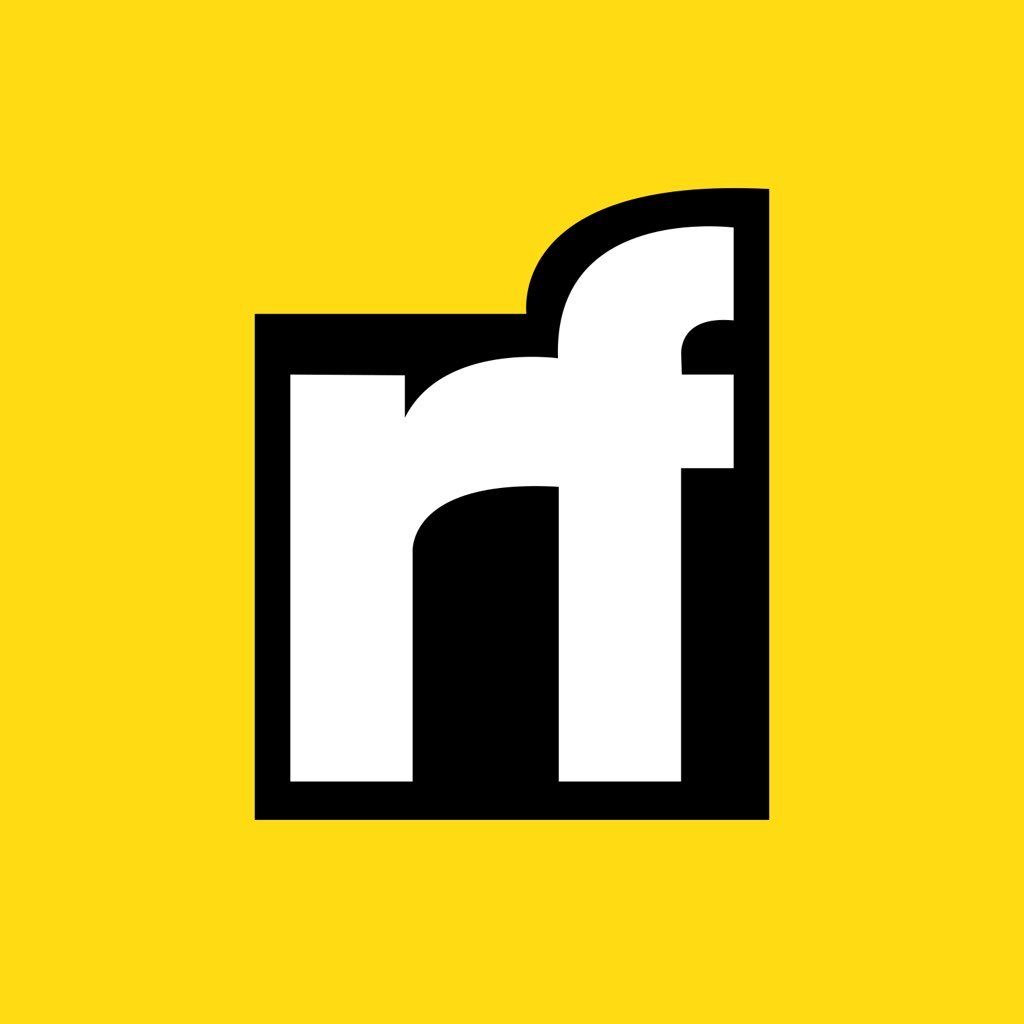
Deven | Sciencx (2023-04-12T07:21:07+00:00) Effortless DTO Validation for Supercharged Laravel Apps!. Retrieved from https://www.scien.cx/2023/04/12/effortless-dto-validation-for-supercharged-laravel-apps/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.