This content originally appeared on Bits and Pieces - Medium and was authored by berastis
In the world of web development, JavaScript is a powerhouse programming language that can make your applications more dynamic and interactive. One of its many strengths is its powerful array methods, which provide a way to manipulate and iterate over arrays with ease.
In this post, we will cover seven of the most popular array methods in JavaScript: .find(), .findIndex(), .map(), .filter(), .fill(), .some(), and .every(). Let’s dive in!
.find()
The .find() method is used to search for the first element in an array that satisfies a given test function. If an element is found, it returns that element; otherwise, it returns undefined.
Syntax: array.find(callback(element, index, array), thisArg)
Example:
const numbers = [1, 3, 5, 7, 9];
const found = numbers.find(element => element > 3);
console.log(found); // Output: 5
.findIndex()
Similar to .find(), the .findIndex() method searches for the first element in an array that satisfies a test function. However, instead of returning the element itself, it returns the index of the found element (or -1 if no element satisfies the test).
Syntax: array.findIndex(callback(element, index, array), thisArg)
Example:
const numbers = [1, 3, 5, 7, 9];
const foundIndex = numbers.findIndex(element => element > 3);
console.log(foundIndex); // Output: 2
.map()
The .map() method creates a new array by applying a given function to every element of the original array.
Syntax: array.map(callback(element, index, array), thisArg)
Example:
const numbers = [1, 2, 3, 4, 5];
const doubled = numbers.map(element => element * 2);
console.log(doubled); // Output: [2, 4, 6, 8, 10]
.filter()
The .filter() method creates a new array containing elements from the original array that pass a given test function.
Syntax: array.filter(callback(element, index, array), thisArg)
Example:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(element => element % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
.fill()
The .fill() method fills all or part of an array with a specified value.
Syntax: array.fill(value, start, end)
Example:
const numbers = [1, 2, 3, 4, 5];
numbers.fill(0, 1, 4);
console.log(numbers); // Output: [1, 0, 0, 0, 5]
.some()
The .some() method tests whether at least one element in the array passes the test implemented by the provided function.
Syntax: array.some(callback(element, index, array), thisArg)
Example:
const numbers = [1, 2, 3, 4, 5];
const hasEven = numbers.some(element => element % 2 === 0);
console.log(hasEven); // Output: true
.every()
The .every() method tests whether all elements in the array pass the test implemented by the provided function.
Syntax: array.every(callback(element, index, array), thisArg)
Example:
const numbers = [2, 4, 6, 8, 10];
const areAllEven = numbers.every(element => element % 2 === 0);
console.log(areAllEven); // Output: true
Conclusion
JavaScript’s array methods provide a powerful and concise way to manipulate and iterate over arrays.
By understanding and mastering these seven array methods — .find(), .findIndex(), .map(), .filter(), .fill(), .some(), and .every() — you can greatly improve your productivity and code readability.
Keep practising and exploring these methods to harness their full potential!
Build Apps with reusable components, just like Lego
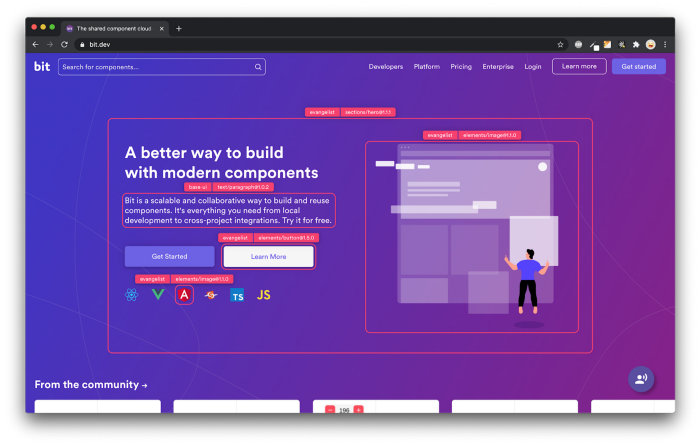
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Creating a Developer Website with Bit components
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
- How to Reuse and Share React Components in 2023: A Step-by-Step Guide
Exploring JavaScript Array Methods: A Guide for Modern Web Development was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by berastis
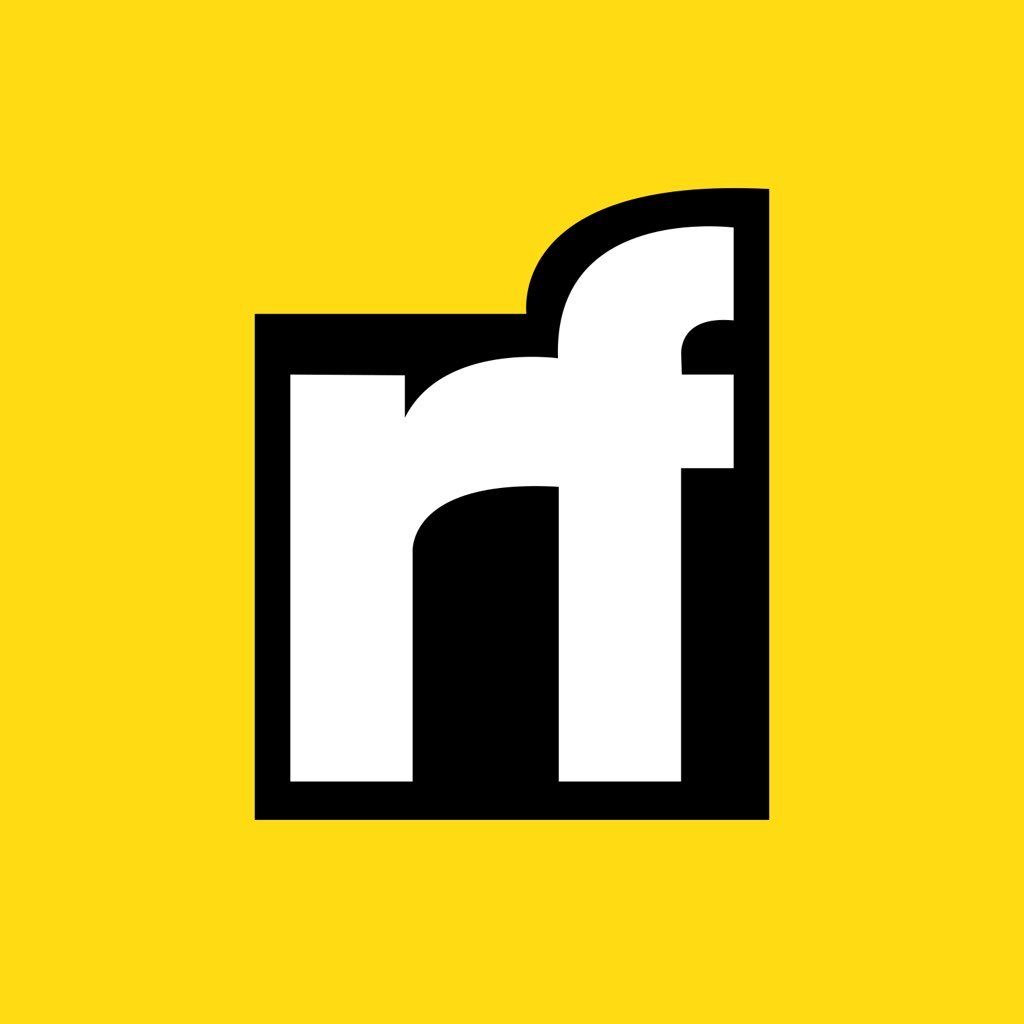
berastis | Sciencx (2023-04-17T08:45:24+00:00) Exploring JavaScript Array Methods: A Guide for Modern Web Development. Retrieved from https://www.scien.cx/2023/04/17/exploring-javascript-array-methods-a-guide-for-modern-web-development/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.