This content originally appeared on DEV Community and was authored by Bentil Shadrack
React.js is a powerful and versatile library that has become one of the most popular choices for building user interfaces. With its modular architecture, performance optimizations, and extensive community support, React has revolutionized the way we create web applications. However, for beginners, React can be quite overwhelming, and it's easy to get lost in the sea of concepts, tools, and best practices.
As a beginner in React.js, it's important to understand the fundamental principles and best practices that can help you become more productive and efficient in your development process. Whether you're building a simple todo app or a complex e-commerce platform, following these best practices can help you write clean, maintainable, and scalable code, and avoid common pitfalls and mistakes.
- ## Keep your components small and reusable A good rule of thumb is to follow the Single Responsibility Principle (SRP) and ensure your components are responsible for only one thing. For example, you could create a separate component for each input field, such as username, password, and email address. By keeping components small and reusable, you can easily test them, debug them, and maintain them in the long run. Example snippet
import React from 'react';
function InputField({ type, name, placeholder }) {
return (
<div>
<label htmlFor={name}>{name}</label>
<input type={type} id={name} name={name} placeholder={placeholder} />
</div>
);
}
export default InputField;
- ## Use JSX correctly JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. When using JSX, make sure you use curly braces to embed JavaScript expressions within JSX code, and use camelCase naming conventions for HTML attributes. For example, instead of using "class" as an attribute, use "className" instead. Avoid using if-else statements in your JSX code and use conditional rendering instead to make it easier to read and maintain your code. Example snippet
import React from 'react';
function Button({ text, onClick }) {
return <button onClick={onClick}>{text}</button>;
}
function App() {
const handleClick = () => {
console.log('Button clicked');
};
return (
<div>
<h1>Hello World</h1>
<Button text="Click me" onClick={handleClick} />
</div>
);
}
export default App;
- ## Follow the one-way data flow The one-way data flow is a fundamental concept in React, where data flows in one direction from the parent component to its children. Avoid modifying the state of a child component from its parent and use props to pass data down from the parent to its children. For example, if you have a shopping cart component, the parent component can pass the items to the cart component as a prop. Example snippet
import React, { useState } from 'react';
function ParentComponent() {
const [items, setItems] = useState(['item 1', 'item 2']);
const handleAddItem = () => {
const newItem = `item ${items.length + 1}`;
setItems([...items, newItem]);
};
return (
<div>
<button onClick={handleAddItem}>Add item</button>
<ChildComponent items={items} />
</div>
);
}
function ChildComponent({ items }) {
return (
<ul>
{items.map((item) => (
<li key={item}>{item}</li>
))}
</ul>
);
}
export default ParentComponent;
- ## Use React DevTools React DevTools is a browser extension that allows you to inspect and debug React components. You can see the hierarchy of your components, inspect their props and state, and even modify them in real-time. For example, if you have a bug in your code, React DevTools can help you identify the source of the problem and make it easier to fix it.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
const handleDecrement = () => {
setCount(count - 1);
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={handleIncrement}>Increment</button>
<button onClick={handleDecrement}>Decrement</button>
</div>
);
}
export default Counter;
- ## Use a CSS-in-JS library Styling components in React can be a challenging task, especially for beginners. Using a CSS-in-JS library like styled-components or emotion can help you write cleaner and more maintainable code. For example, you can use a CSS-in-JS library to style a button component directly in your JavaScript file without having to create a separate CSS file. Example code snippet
import styled from 'styled-components';
const Button = styled.button`
background-color: #4caf50;
border: none;
color: white;
padding: 10px 20px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
border-radius: 5px;
`;
function App() {
return (
<div>
<h1>Hello World</h1>
<Button>Click me</Button>
</div>
);
}
export default App;
- ## Use React hooks React hooks are a set of functions that allow you to use state and other React features in functional components. Hooks can simplify your code, reduce the number of class components you need to write, and make it easier to share logic across different components. For example, you can use the useState hook to manage the state of your components and make it easier to update them based on user interactions.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
const handleIncrement = () => {
setCount(count + 1);
};
const handleDecrement = () => {
setCount(count - 1);
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={handleIncrement}>Increment</button>
<button onClick={handleDecrement}>Decrement</button>
</div>
);
}
export default Counter;
- ## Use PropTypes or TypeScript React allows you to define the type of props that a component should receive using PropTypes or TypeScript. Using these tools can help you catch errors early and ensure that your code is robust and maintainable. For example, if you have a component that requires a specific prop type, using PropTypes or TypeScript can help you avoid type-related bugs and provide better documentation for your components. Example snippet on PropTypes
import React from 'react';
import PropTypes from 'prop-types';
function Person({ name, age, email }) {
return (
<div>
<h1>{name}</h1>
<p>Age: {age}</p>
<p>Email: {email}</p>
</div>
);
}
Person.propTypes = {
name: PropTypes.string.isRequired,
age: PropTypes.number.isRequired,
email: PropTypes.string.isRequired,
};
export default Person;
Example snippet on TypeScript
import React, { useState } from 'react';
interface CounterProps {
initialValue: number;
}
function Counter({ initialValue }: CounterProps) {
const [count, setCount] = useState(initialValue);
const handleIncrement = () => {
setCount(count + 1);
};
const handleDecrement = () => {
setCount(count - 1);
};
return (
<div>
<h1>Count: {count}</h1>
<button onClick={handleIncrement}>Increment</button>
<button onClick={handleDecrement}>Decrement</button>
</div>
);
}
export default Counter;
By the end of this article, you'll have a better understanding of how to structure your components, use JSX correctly, manage state and props, debug and inspect your code, and leverage the latest tools and techniques to create high-quality applications. Whether you're a beginner or an experienced developer, these best practices can help you become a better React developer and take your skills to the next level. So let's dive in and explore the world of React best practices!
Conclusion
In this article, we have explored some of the best practices that every beginner should follow to increase productivity and build efficient React applications. From keeping your components small and reusable to using React DevTools, CSS-in-JS libraries, and TypeScript, we have cover a range of topics that can help you improve your coding skills and build better applications.
Following these best practices can help you become a more efficient and effective React developer. By keeping your components small and reusable, using JSX correctly, following the one-way data flow, using React DevTools, using a CSS-in-JS library, using React hooks, and using PropTypes or TypeScript, you can write clean, maintainable, and scalable React applications.
Bentil here🚀
Are you a ReactJS developer? What tips can you add to help beginners about to speed their React.js journey?
Kindly leave them in the comment section. It might be helpful for others.
If you like this article, Kindly Like, Share and follow us for more.
This content originally appeared on DEV Community and was authored by Bentil Shadrack
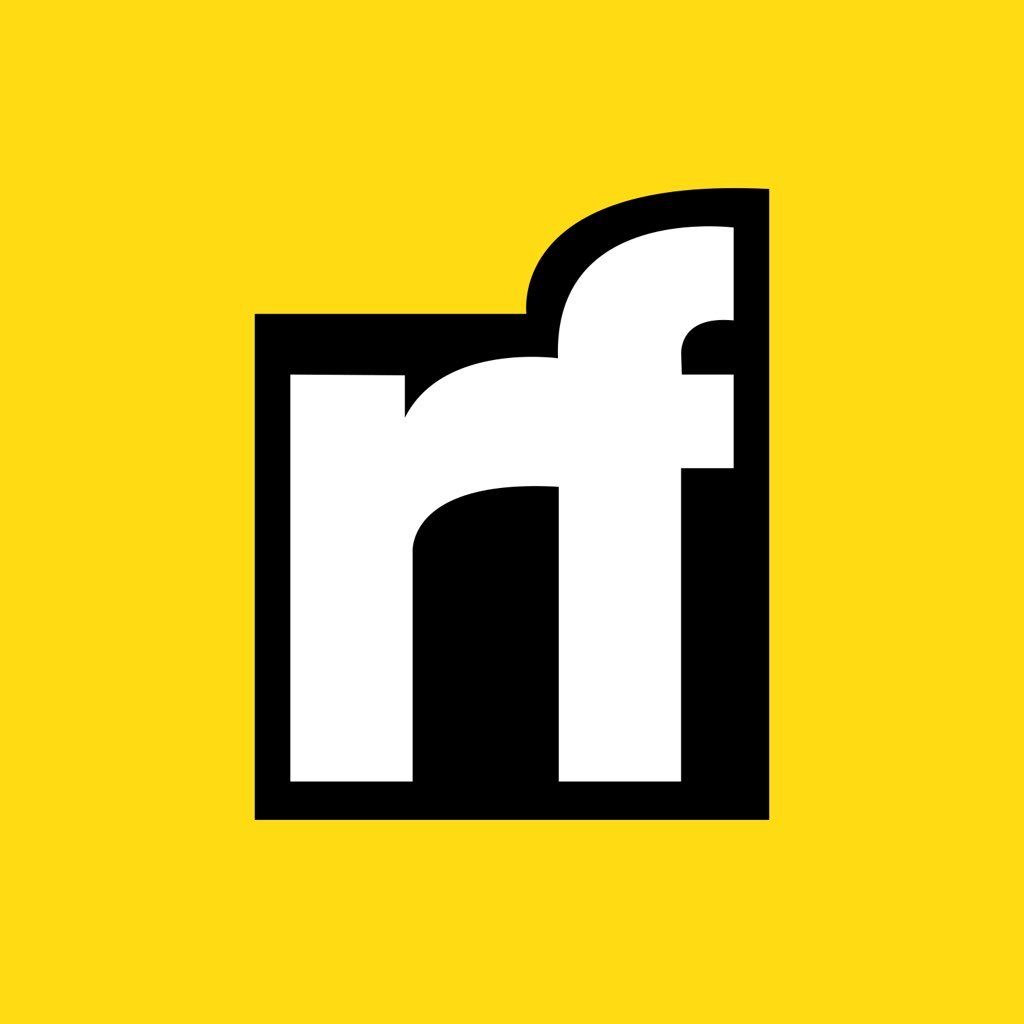
Bentil Shadrack | Sciencx (2023-04-21T12:55:37+00:00) Best practices every beginner in React.js must follow to increase productivity. Retrieved from https://www.scien.cx/2023/04/21/best-practices-every-beginner-in-react-js-must-follow-to-increase-productivity/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.