This content originally appeared on Bits and Pieces - Medium and was authored by Anto Semeraro
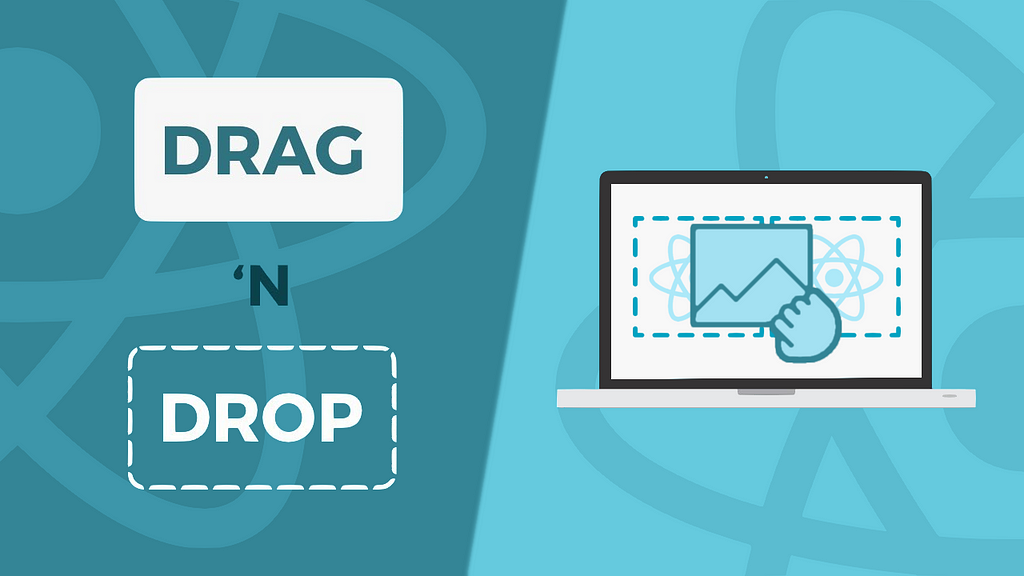
Front-End Development
Master the art of creating seamless and visually appealing drag-and-drop interactions in your React applications with the power of React Beautiful Dnd library
Introduction
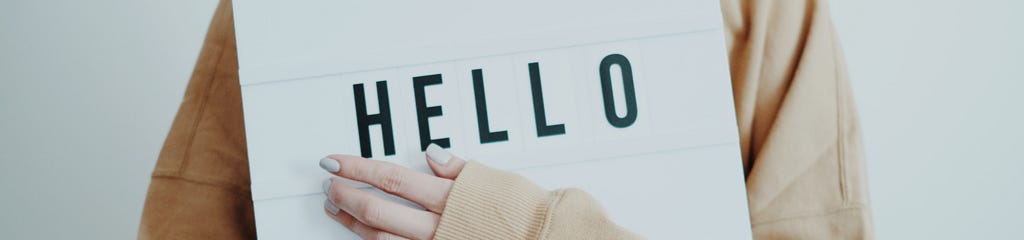
A World of Seamless Interactions: The Magic of Drag-And-Drop
Picture this: You’re using a project management app to organize your team’s tasks, effortlessly dragging and dropping tasks from one column to another as priorities shift. This smooth interaction feels almost magical, creating an intuitive and visually appealing user experience. But have you ever wondered how you can recreate this magic in your own React applications? Enter React Beautiful Dnd, a powerful library that makes implementing drag-and-drop functionality a breeze.
In this article, I’ll take a deep dive into the world of React Beautiful Dnd and explore how it can transform your web applications by adding seamless and visually appealing drag-and-drop interactions. Whether you’re a seasoned React developer or a newcomer eager to expand your skill set, this comprehensive guide will arm you with the knowledge and tools needed to create stunning and interactive web applications that delight your users.
Get ready to master the art of creating drag-and-drop interactions with the power of React Beautiful Dnd library! So, grab a cup of your favorite beverage, and let’s embark on this exciting journey together.
💡 It’s worth adding here that if you need to reuse the components/functionalities we are going to build in this article, you might want to consider using a component management hub such as Bit. With Bit you can easily share, discover, and reuse individual components across multiple projects.
Learn more here:
How to reuse React components across your projects
Setting Up Your Environment
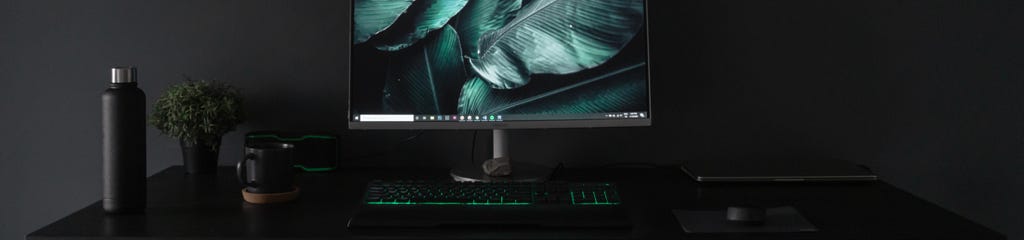
Laying the Foundation: Your Essential Toolkit
Before we dive into the world of React Beautiful Dnd, we need to ensure that your development environment is properly set up. Don’t worry, I’ll guide you through this process step by step, so you can start implementing drag-and-drop functionality in no time. By the end of this section, you’ll have all the necessary tools and software at your fingertips, ready to create something extraordinary!
Preparing Your Development Environment
First things first: let’s make sure you have the following software installed on your computer:
- Node.js (version 10 or higher): Node.js is a runtime environment that allows you to run JavaScript on the server side. You can download it from the official website: https://nodejs.org/
- A code editor of your choice: I recommend Visual Studio Code (https://code.visualstudio.com/), but feel free to use your favorite one.
Creating a New React Project
Now that your development environment is ready, it’s time to create a new React project. Open your terminal or command prompt and run the following command:
npx create-react-app my-dnd-app
This command will create a new folder called my-dnd-app containing a boilerplate React application. Feel free to replace "my-dnd-app" with your preferred project name.
Installing React Beautiful Dnd
With your React project set up, it’s time to install the React Beautiful Dnd library. Navigate to your project folder using the terminal or command prompt:
cd my-dnd-app
Then, run the following command to install the library:
npm install react-beautiful-dnd
Congratulations! You’ve successfully installed React Beautiful Dnd and are ready to start exploring its features.
Setting Up Your Code Editor
Before diving into the code, make sure your code editor is properly configured for a smooth development experience. If you’re using Visual Studio Code, I recommend installing the following extensions:
- ESLint: Helps you maintain a consistent code style and catches potential issues early on.
- Prettier: Automatically formats your code, keeping it clean and readable.
- Babel JavaScript: Enhances your JavaScript syntax highlighting and autocompletion.
Feel free to explore and install additional extensions that suit your preferences and workflow.
And that’s it! Your environment is now fully prepared for you to start implementing drag-and-drop functionality with React Beautiful Dnd. In the next section, I’ll introduce the key components of the library and explore how they interact with each other. Let the magic begin!
Understanding the Basics of React Beautiful Dnd
In this section, we’ll dive into the fundamentals of React Beautiful Dnd, a powerful library that makes it a breeze to create intuitive drag-and-drop interactions in your React applications.
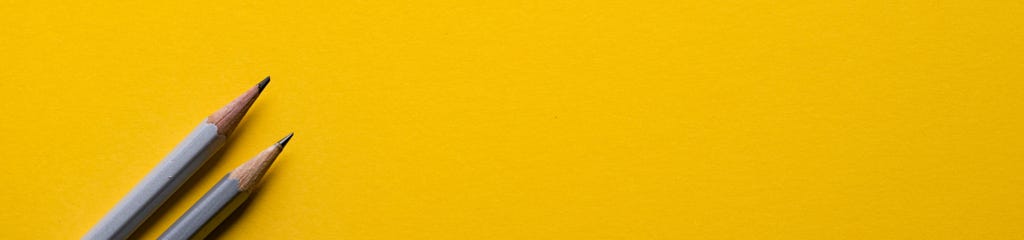
We’ll examine the key components that form the building blocks of the library: Draggable, Droppable, and DragDropContext, and explore how they work together to create a seamless user experience.
The Core Components
React Beautiful Dnd revolves around three primary components:
- Draggable: Represents the individual items that can be dragged around.
- Droppable: Defines the areas where Draggable items can be dropped.
- DragDropContext: The top-level component that manages the overall state and coordinates the communication between Draggable and Droppable components.
Let’s break down each component in more detail.
Draggable
The Draggable component is the heart of any drag-and-drop interaction. This component wraps around any element that you want to make draggable within your application. By giving it a unique id and an index, React Beautiful Dnd knows how to manage the item's position during the dragging process.
<Draggable draggableId="uniqueId" index={0}>
{(provided) => (
<div
ref={provided.innerRef}
{...provided.draggableProps}
{...provided.dragHandleProps}
>
Your draggable content here
</div>
)}
</Draggable>
Droppable
The Droppable component represents the drop zones, or the areas where users can drop Draggable items. Each Droppable requires a unique droppableId. You'll also need to provide a function that returns the appropriate markup for the drop zone.
<Droppable droppableId="uniqueId">
{(provided) => (
<div ref={provided.innerRef} {...provided.droppableProps}>
{/* The Draggable components will be inserted here */}
{provided.placeholder}
</div>
)}
</Droppable>
DragDropContext
The DragDropContext component acts as the top-level wrapper for your entire drag-and-drop system. It manages the global state and coordinates communication between Draggable and Droppable components. You’ll need to provide an onDragEnd callback function, which is called when a drag operation is completed. This is where you'll update your application state to reflect the new item order.
<DragDropContext onDragEnd={handleDragEnd}>
{/* Your Draggable and Droppable components go here */}
</DragDropContext>
How the Components Interact
Now that we’ve covered the individual components, let’s look at how they interact with each other. When a user starts dragging a Draggable item, the DragDropContext component listens for the drag event and updates its internal state accordingly. As the user moves the item over a Droppable area, the Droppable component communicates with the DragDropContext to determine if the item can be dropped there.
Once the user releases the item, the onDragEnd callback function is triggered. This is where you'll update your application's state to reflect the new order of the Draggable items. React Beautiful Dnd takes care of all the complex calculations and animations, allowing you to focus on implementing your desired functionality.
Building a Simple Example: Task Board
In this section, we’ll walk through the process of creating a basic task board using React Beautiful Dnd.
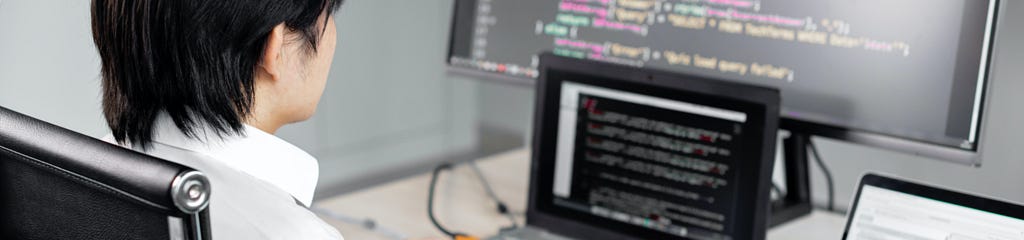
This step-by-step guide will help you understand how the library’s key components work together and provide tips for customization and styling.
Step 1: Create Task Board Components
First, let’s create a few components that will represent our task board, columns, and tasks. In your src directory, create a new folder called components and add three new files: TaskBoard.js, Column.js, and Task.js. We'll start by creating the Task component in Task.js:
import React from 'react';
const Task = ({ task }) => {
return (
<div className="task">
{task.content}
</div>
);
};
export default Task;
In Column.js, create a simple column component that renders its child tasks:
import React from 'react';
import Task from './Task';
const Column = ({ column, tasks }) => {
return (
<div className="column">
<h3>{column.title}</h3>
<div className="task-list">
{tasks.map(task => <Task key={task.id} task={task} />)}
</div>
</div>
);
};
export default Column;
Lastly, create the TaskBoard component in TaskBoard.js, which will render multiple columns:
import React from 'react';
import Column from './Column';
const TaskBoard = ({ data }) => {
return (
<div className="task-board">
{data.columnOrder.map(columnId => {
const column = data.columns[columnId];
const tasks = column.taskIds.map(taskId => data.tasks[taskId]);
return <Column key={column.id} column={column} tasks={tasks} />;
})}
</div>
);
};
export default TaskBoard;
Step 2: Integrate React Beautiful Dnd
Now that we have our basic components, let’s integrate React Beautiful Dnd to enable drag-and-drop functionality. First, import the necessary components at the top of TaskBoard.js:
import { DragDropContext, Droppable } from 'react-beautiful-dnd';
Next, wrap the entire task board in a DragDropContext component, which will manage the overall drag-and-drop state:
const TaskBoard = ({ data }) => {
return (
<DragDropContext>
{/* ... */}
</DragDropContext>
);
};
In Column.js, wrap the task list in a Droppable component, which defines the areas where tasks can be dropped:
import { Droppable } from 'react-beautiful-dnd';
// ...
const Column = ({ column, tasks }) => {
return (
<div className="column">
<h3>{column.title}</h3>
<Droppable droppableId={column.id}>
{(provided) => (
<div
className="task-list"
ref={provided.innerRef}
{...provided.droppableProps}
>
{tasks.map(task => <Task key={task.id} task={task} />)}
{provided.placeholder}
</div>
)}
</Droppable>
</div>
);
};
Finally, update Task.js to wrap the task content in a Draggable component, which allows tasks to be picked up and moved:
import { Draggable } from 'react-beautiful-dnd';
// ...
const Task = ({ task, index }) => {
return (
<Draggable draggableId={task.id} index={index}>
{(provided) => (
<div
className="task"
ref={provided.innerRef}
{...provided.draggableProps}
{...provided.dragHandleProps}
>
{task.content}
</div>
)}
</Draggable>
);
};
Don’t forget to pass the index prop from the Column component to the Task component, as it is required by the Draggable component:
// Column.js
{tasks.map((task, index) => (
<Task key={task.id} task={task} index={index} />
))}
Step 3: Implement Drag-and-Drop Event Handlers
To handle drag-and-drop events, we’ll need to define a function that handles the onDragEnd event in our TaskBoard component. This function will be responsible for updating the task order when a drag-and-drop operation is completed:
import { useState } from 'react';
// ...
const TaskBoard = ({ data }) => {
const [boardData, setBoardData] = useState(data);
const onDragEnd = (result) => {
// Implement the logic for updating task order
};
return (
<DragDropContext onDragEnd={onDragEnd}>
{/* ... */}
</DragDropContext>
);
};
Inside the onDragEnd function, check if the drag operation was successful, and update the task order accordingly:
const onDragEnd = (result) => {
const { destination, source, draggableId } = result;
if (!destination) {
return;
}
if (
destination.droppableId === source.droppableId &&
destination.index === source.index
) {
return;
}
const column = boardData.columns[source.droppableId];
const newTaskIds = Array.from(column.taskIds);
newTaskIds.splice(source.index, 1);
newTaskIds.splice(destination.index, 0, draggableId);
const newColumn = {
...column,
taskIds: newTaskIds,
};
const newBoardData = {
...boardData,
columns: {
...boardData.columns,
[newColumn.id]: newColumn,
},
};
setBoardData(newBoardData);
};
Step 4: Customize and Style
Now that our task board has drag-and-drop functionality, you can further customize and style it according to your preferences. Use CSS to style the components and make them visually appealing. For example, add smooth transitions during dragging, customize the colors and fonts, or add hover effects to enhance the user experience.
With your task board complete, you can now explore additional features of React Beautiful Dnd, such as dragging between multiple columns or incorporating more complex layouts. Don’t hesitate to experiment and apply this knowledge to your own projects!
Advanced Features and Use Cases
In this section, we’ll dive into some advanced features of React Beautiful Dnd and explore potential use cases to take your drag-and-drop interactions to the next level.
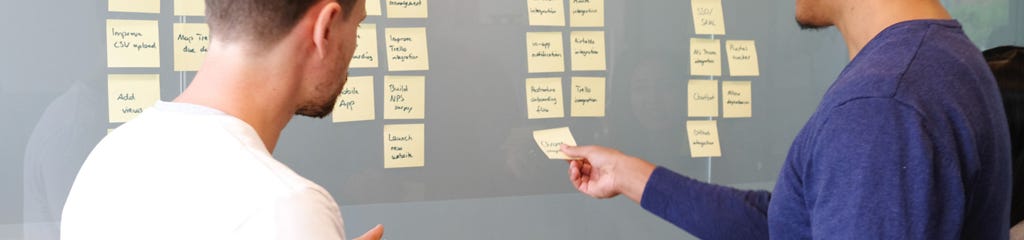
Keep in mind that code examples below are simplified to give you a general idea of how to implement each feature. You’ll need to adjust and expand the code based on your specific use case and application requirements.
Multiple Droppable Areas
React Beautiful Dnd makes it easy to support multiple droppable areas in your application. You can achieve this by creating additional Droppable components and ensuring they have unique droppableId values.
This feature can be particularly useful when you want to implement a Kanban board or move items between lists, like transferring tasks from a to-do list to a done list.
/* Example of Multiple Droppable Areas */
<Droppable droppableId="droppable1">
{(provided) => (
<div ref={provided.innerRef} {...provided.droppableProps}>
{/* Render draggable items here */}
{provided.placeholder}
</div>
)}
</Droppable>
<Droppable droppableId="droppable2">
{(provided) => (
<div ref={provided.innerRef} {...provided.droppableProps}>
{/* Render draggable items here */}
{provided.placeholder}
</div>
)}
</Droppable>
Conditional Dropping
Sometimes, you may want to restrict the items that can be dropped into specific areas. With React Beautiful Dnd, you can implement conditional dropping by using the isDropDisabled prop in the Droppable component. You can set this prop to a boolean value or pass a function that returns a boolean value based on certain conditions.
/* Example of Conditional Dropping */
<Droppable droppableId="droppable1" isDropDisabled={isDropDisabled}>
{/* ... */}
</Droppable>
Custom Drag Handles
React Beautiful Dnd allows you to create custom drag handles to enhance the user experience. By default, the entire draggable area acts as the drag handle, but you can choose a specific part of the component to be the drag handle using the dragHandleProps provided by the Draggable component.
/* Example of Custom Drag Handles */
<Draggable draggableId="draggable1" index={0}>
{(provided) => (
<div ref={provided.innerRef} {...provided.draggableProps}>
{/* Render the drag handle */}
<div {...provided.dragHandleProps}>Drag Handle</div>
{/* Rest of the content */}
</div>
)}
</Draggable>
Dragging Between Lists with Different Types
If you want to implement a drag-and-drop interaction between lists with different types, React Beautiful Dnd has you covered. You can set the type prop on the Droppable component to differentiate lists and prevent items from being dropped in the wrong list.
/* Example of Dragging Between Lists with Different Types */
<Droppable droppableId="droppable1" type="TYPE_A">
{/* ... */}
</Droppable>
<Droppable droppableId="droppable2" type="TYPE_B">
{/* ... */}
</Droppable>
Performance Optimizations
For large lists, performance can be a concern, but React Beautiful Dnd provides options for optimization. You can use the windowing technique, which involves rendering only a subset of items in the list based on the user's scroll position. There are third-party libraries, such as react-window and react-virtualized, that can help you implement windowing in your application.
/* Example of Performance Optimizations (using react-window) */
import { FixedSizeList as List } from 'react-window';
<List
height={500}
itemCount={items.length}
itemSize={75}
width={300}
>
{({ index, style }) => (
<Draggable key={items[index].id} draggableId={items[index].id} index={index}>
{(provided) => (
<div
ref={provided.innerRef}
{...provided.draggableProps}
{...provided.dragHandleProps}
style={{ ...provided.draggableProps.style, ...style }}
>
{/* Render item content */}
</div>
)}
</Draggable>
)}
</List>
Accessibility
React Beautiful Dnd comes with built-in keyboard support, making your drag-and-drop interactions accessible to users who prefer or require keyboard navigation. It also supports screen readers to enhance the experience for users with visual impairments.
Now that you’re familiar with some advanced features of React Beautiful Dnd, I encourage you to explore further customization and apply these techniques to your own projects. Let your imagination run wild and create innovative, visually stunning, and highly functional drag-and-drop experiences!
Unlocking Drag and Drop Potential and Frontend Best Practices
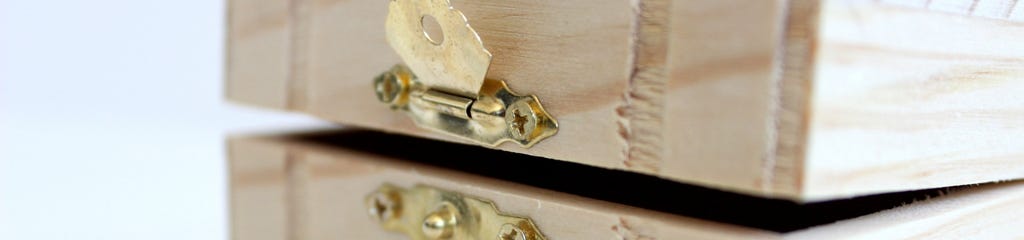
Congratulations! You’ve now mastered the basics of React Beautiful Dnd and built a task board. You’ve learned about the key components: Draggable, Droppable, and DragDropContext, empowering you to create seamless drag-and-drop interactions for your React applications.
However, this is just the beginning. Explore further features and use cases to tailor your application’s drag-and-drop experience. And to keep your React applications top-notch, stay informed on frontend best practices. Check out these articles for additional insights.
- Mastering UI Design: A Complete Guide to Best Practices
- JavaScript Optimization Techniques for Faster Website Load Times: An In-Depth Guide
Don’t forget to share your experiences with the community and learn from others. Now, go ahead and enhance your React applications with engaging drag-and-drop interactions. Happy coding!
Build React Apps with reusable components, just like Lego
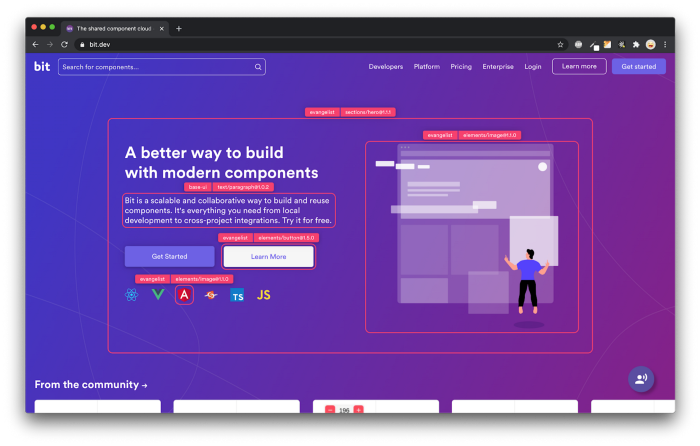
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Creating a Developer Website with Bit components
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
- How to Reuse and Share React Components in 2023: A Step-by-Step Guide
Bibliography
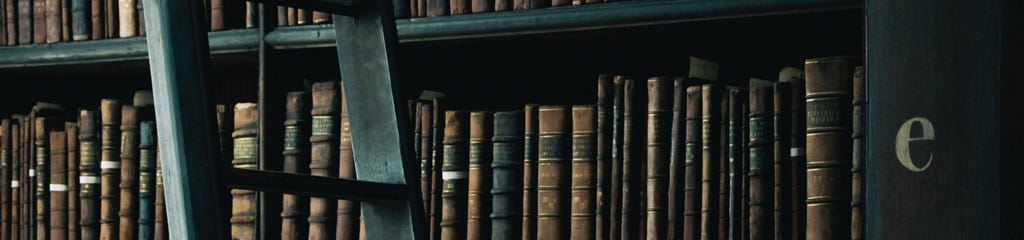
- “React: Up & Running”, Stoyan Stefanov, 2021, O’Reilly Media
- “Learning React: A Hands-On Guide to Building Web Applications”, Kirupa Chinnathambi, 2018, Addison-Wesley
- “React Explained: Your Step-by-Step Guide to React”, Zac Gordon, 2019, Kindle Scribe
- “The Road to React: The React.js with Hooks in JavaScript Book”, Robin Wieruch, 2017, Kindle Scribe
How to Implement Drag and Drop Functionality in React with React Beautiful Dnd was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Anto Semeraro
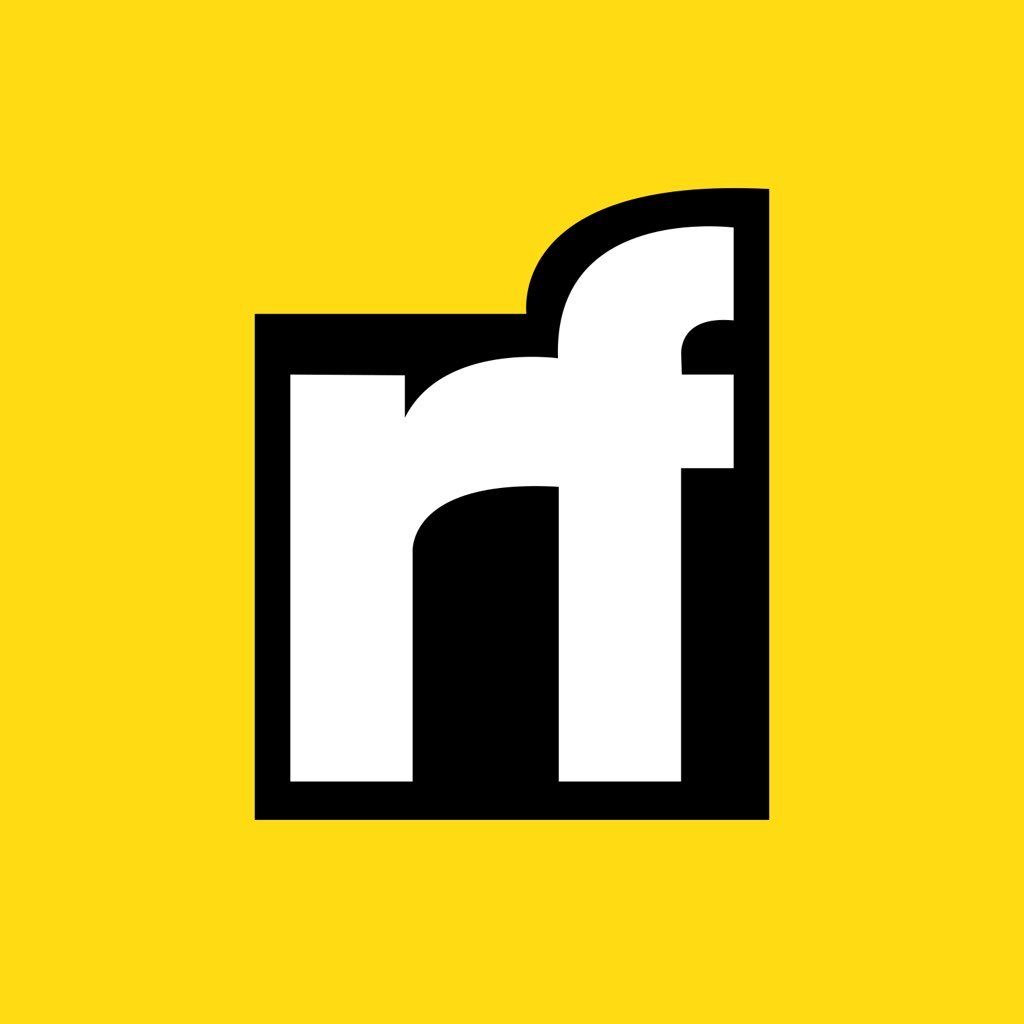
Anto Semeraro | Sciencx (2023-04-29T06:02:09+00:00) How to Implement Drag and Drop Functionality in React with React Beautiful Dnd. Retrieved from https://www.scien.cx/2023/04/29/how-to-implement-drag-and-drop-functionality-in-react-with-react-beautiful-dnd/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.