This content originally appeared on Level Up Coding - Medium and was authored by Mehran
Best Practices for 2023
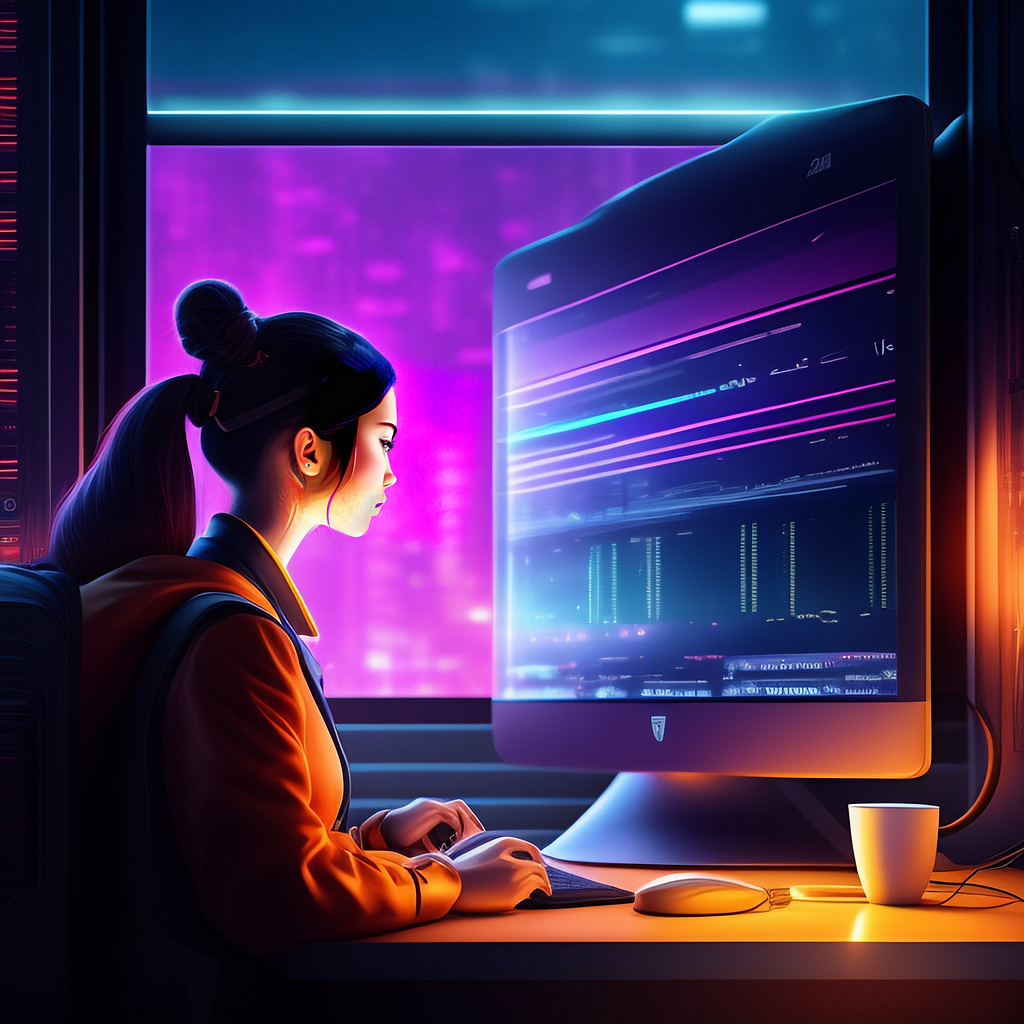
Introduction
In the digital age, software development places high importance on security. JavaScript remains a top choice for web development, and its server-side counterpart, Node.js, has gained immense popularity due to its flexibility and powerful features. However, when working with Node.js, it is crucial to prioritize security. This post guides the best security practices for Node.js in 2023.
Always Keep Your Environment Up-to-Date
The first line of defense in Node.js security is keeping your environment updated. Node.js and its packages are regularly updated to patch vulnerabilities, optimize performance, and add new features. Utilize npm's npm outdated command to identify outdated packages and npm update to upgrade them.
# Check for outdated packages
npm outdated
# Update all packages
npm update
Limit the Use of Third-Party Packages
Node.js's vast ecosystem is one of its significant strengths, but it can also be a weakness if not properly managed. Third-party packages can introduce vulnerabilities into your application. Use them sparingly and only from trustworthy sources. Tools like the Node Security Platform or npm audit can help identify packages with known vulnerabilities.
npm audit
Use Appropriate HTTP Headers
Adding HTTP headers like Content Security Policy (CSP), HTTP Strict Transport Security (HSTS), and X-XSS-Protection can make your application more secure. To simplify things, you can use libraries like Helmet.js to help set these headers and protect your application from common web vulnerabilities.
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet());
Secure Your MongoDB Database
If you use MongoDB as your database, safeguard it against NoSQL injection attacks by verifying and cleansing user inputs. Keep MongoDB updated to the latest version, enable encryption while storing data, and employ MongoDB’s built-in RBAC (Role-Based Access Control) for precise access control.
const mongoose = require('mongoose');
const sanitize = require('mongo-sanitize');
// Clean the input to prevent injection attacks
const cleanInput = sanitize(userInput);
Secure Your Express Apps
When using the popular Node.js framework Express.js, it’s essential to utilize its built-in security measures. It’s crucial to avoid exposing errors to users, as this could potentially reveal sensitive information or app architecture details to attackers. To prevent brute-force attacks, implement the express-rate-limit middleware, and for protection against Cross-Site Request Forgery (CSRF), use the csurf middleware.
const rateLimit = require("express-rate-limit");
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // limit each IP to 100 requests per windowMs
});
app.use(limiter);
CSRF protection:
Cross-Site Request Forgery (CSRF) is a security vulnerability in web applications. It tricks the victim into submitting a malicious request. It uses the identity and privileges of the victim to perform an undesired function on their be
Let's consider a hypothetical situation. Imagine that you are currently signed into your bank's website, and a fraudulent website attempts to transfer funds from your account to the attacker's. If the bank's website lacks CSRF safeguards, it may accept the request as genuine since it originates from a logged-in user (you) and proceeds with the transaction.
To prevent a certain type of attack, CSRF protection is implemented. This protection involves the addition of an anti-CSRF token to forms and AJAX calls, which is a random value linked to the user’s session. To ensure that the request is legitimate, the server verifies that the correct token is included when the form is submitted. The request will be denied if the token is missing or incorrect.
To protect against CSRF attacks in Node.js, you can utilize the csurf middleware. This middleware incorporates a _csrf method into the request object, generating a fresh CSRF token. Below is an example of how to implement it.
const csrf = require('csurf');
app.use(csrf());
Implement Error Handling
Correct error handling helps with debugging and plays a crucial role in security. Unhandled errors can crash your application, making it a target for DoS attacks. Use ‘try-catch’ blocks and domain-specific error handlers to catch unhandled errors.
process.on('uncaughtException', (err) => {
console.error('There was an uncaught error', err);
process.exit(1); //mandatory (as per the Node.js docs)
});
Use TLS (Transport Layer Security)
Always use HTTPS instead of HTTP to encrypt data in transit between the client and server. This can be achieved by implementing TLS. Certificates can be acquired for free from Let’s Encrypt.
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('test/fixtures/keys/agent2-key.pem'),
cert: fs.readFileSync('test/fixtures/keys/agent2-cert.pem')
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end("Secure connection!\n");
}).listen(8000);
Use JWT for User Authentication
JSON Web Tokens (JWT) is a secure and stateless way to manage user authentication and can also be used for secure information exchange. However, remember to store tokens securely and set an expiration time.
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('test/fixtures/keys/agent2-key.pem'),
cert: fs.readFileSync('test/fixtures/keys/agent2-cert.pem')
};
https.createServer(options, (req, res) => {
res.writeHead(200);
res.end("Secure connection!\n");
}).listen(8000);
Use JWT for User Authentication
JSON Web Tokens (JWT) is a secure and stateless way to manage user authentication and can also be used for secure information exchange. However, remember to store tokens securely and set an expiration time.
const jwt = require('jsonwebtoken');
// sign with default (HMAC SHA256)
var token = jwt.sign({ data: 'userdata' }, 'secret', { expiresIn: '1h' });
Secure Against XSS and CSRF
Cross-site Scripting (XSS) and Cross-Site Request Forgery (CSRF) are common attack vectors in web applications. Use output encoding libraries like OWASP’s ESAPI to defend against XSS and anti-CSRF tokens for CSRF protection.
const escapeHtml = require('escape-html');
let userContent = '<script>alert("XSS")</script>';
let safeContent = escapeHtml(userContent);
Node.js Security was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Mehran
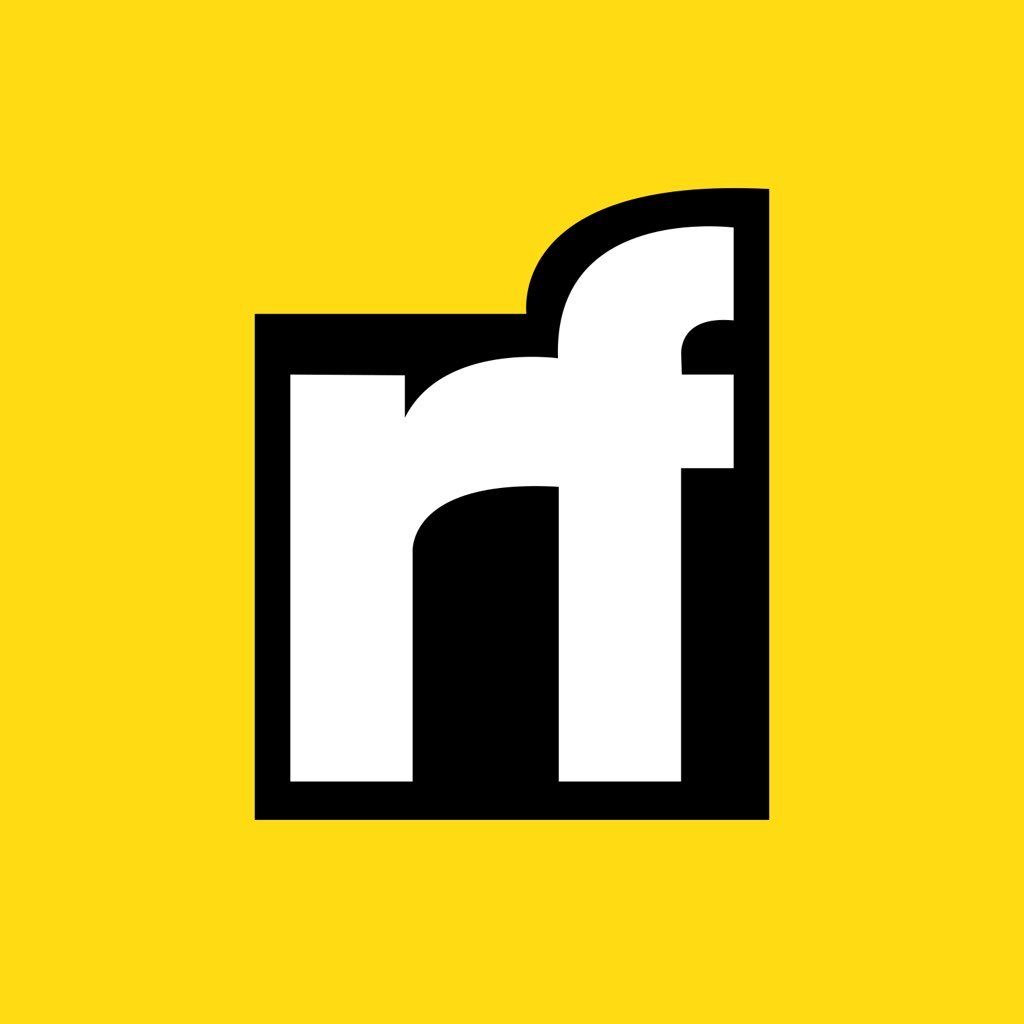
Mehran | Sciencx (2023-05-01T02:51:48+00:00) Node.js Security. Retrieved from https://www.scien.cx/2023/05/01/node-js-security/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.