This content originally appeared on Level Up Coding - Medium and was authored by Rabi Siddique
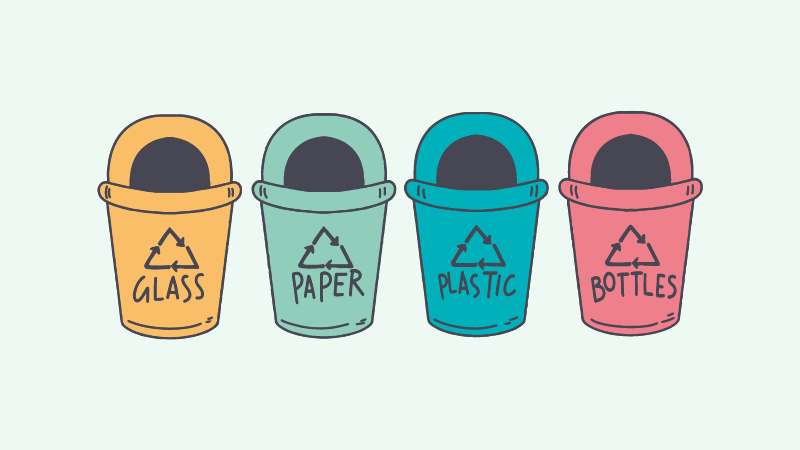
The Interface Segregation Principle (ISP) is a software design principle that suggests breaking down software interfaces into smaller, specialized ones that serve specific client needs. The goal of ISP is to prevent clients from being forced to implement methods they don’t use, thereby reducing interface pollution in the codebase.
In other words, it encourages software developers to create interfaces that are tailored to the specific needs of clients, rather than creating one big interface that tries to satisfy everyone. By doing so, it promotes a more modular and maintainable codebase, making it easier to make changes without affecting the entire system.
Violation
Let’s look at an example in Java. Suppose we have an interface called Vehicle that has two methods: drive() and fly(). We also have two classes called Car and Airplane that implement this interface.
public interface Vehicle {
public void drive();
public void fly();
}
public class Car implements Vehicle {
public void drive() {
// code to drive the car
}
public void fly() {
// OOPS
}
}
public class Airplane implements Vehicle {
public void drive() {
// code to drive the airplane
}
public void fly() {
// code to fly the airplane
}
}
In this code example, theCar class, does not have the capability to fly, yet it is still forced to implement the fly() method since it is part of the Vehicle interface. This violates the Interface Segregation Principle because the Vehicle interface is not tailored to the specific needs of each implementing class which can introduce the following problems:
- Inappropriate Behavior: If a class is forced to implement a method it doesn’t use, it might have to implement it with dummy code or throw an exception, which can lead to inappropriate behaviour in the system. For example, in the example where Car implements the fly() method with some dummy code, it might give the impression that the car can fly, which is not true.
- Reduced Flexibility: If the interface is polluted with unnecessary methods, it might make it harder to extend the system with new functionality. For example, if we want to add a new class that implements only the fly() method, we can't do that without also implementing the drive() method, which might be unnecessary and confusing.
- Misleading Design: An interface that is polluted with unrelated methods can be misleading to other developers who use the interface. It can give the impression that the implementing class supports functionality that it doesn’t actually have, leading to confusion and potentially incorrect assumptions.
Applying the Interface Segregation Principle
To apply the Interface Segregation Principle, a better approach would be to create separate interfaces for driving and flying, so that classes can implement only the methods they need, like this:
public interface Driveable {
public void drive();
}
public interface Flyable {
public void fly();
}
public class Car implements Driveable {
public void drive() {
// code to drive the car
}
}
public class Airplane implements Driveable, Flyable {
public void drive() {
// code to drive the airplane
}
public void fly() {
// code to fly the airplane
}
}
By separating the driving and flying functionality into separate interfaces, we can avoid interface pollution and create more modular and maintainable code.
Conclusion
In conclusion, the Interface Segregation Principle (ISP) is an essential software design principle that can make our code more modular and maintainable. However, applying ISP requires additional time and effort during the design phase and may increase code complexity. Therefore, we should apply it based on experience and common sense in identifying the areas where the extension of code is more likely to happen in the future.
In my next blog post, we discuss Dependency Inversion Principle(DIP). Here is the link.
Thank you for reading. I hope this post is helpful to you. If you have any further questions, don’t hesitate to reach out. I’m always happy to help.
Interface Segregation Principle in Software Engineering 🔍 was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Rabi Siddique
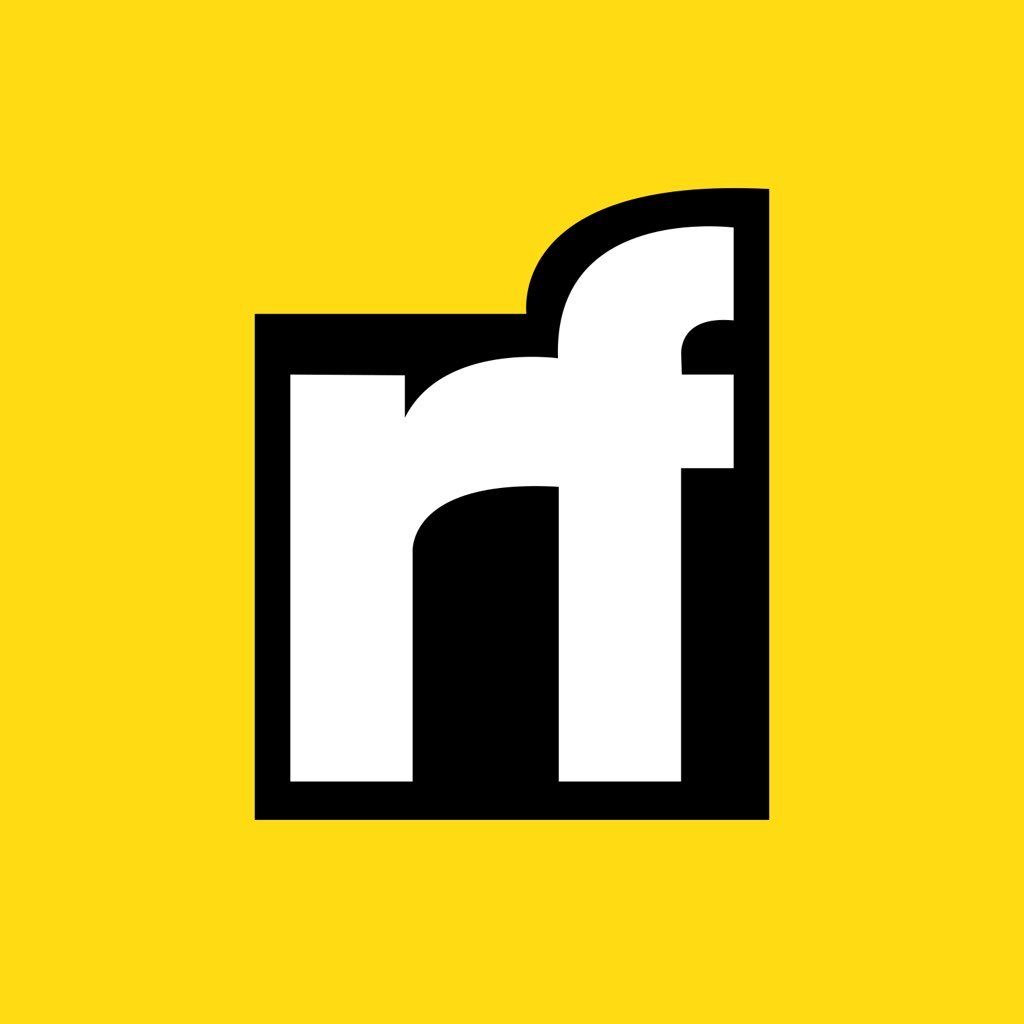
Rabi Siddique | Sciencx (2023-05-02T17:24:47+00:00) Interface Segregation Principle in Software Engineering. Retrieved from https://www.scien.cx/2023/05/02/interface-segregation-principle-in-software-engineering/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.