This content originally appeared on Level Up Coding - Medium and was authored by Arjun M S
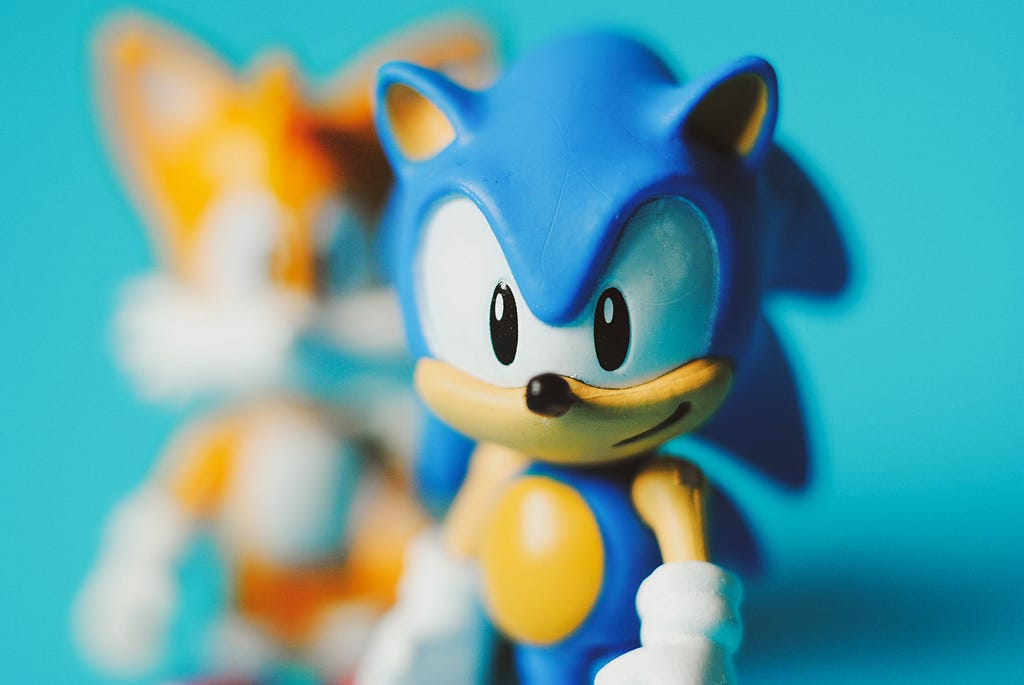
In recent years, Python has gained massive popularity as a high-level, general-purpose programming language, renowned for its user-friendly nature and uncomplicated structure. Nonetheless, as your codebase expands, so does the complexity, and your code may lose efficiency. Slow code can be responsible for an unpleasant user experience, wasted time, and reduced productivity. Thankfully, there are numerous techniques and strategies that you can implement to enhance the efficiency of your Python code. In this article, we’ll delve into some of the most effective methods for optimizing your Python code, thereby boosting its speed, dependability, and manageability.
Table of Contents
- Introduction
- Profiling Your Code
- Avoiding Inefficient Loops
- List Comprehensions
- Using Built-in Functions
- Lazy Evaluation
- Avoiding Global Variables
- The Power of NumPy
- Caching Results
- Multiprocessing and Threading
- Using Libraries
- Using a Compiler
- Testing Your Code
- Conclusion
Introduction
Python is an interpreted language, implying that it processes each line of code in a sequence. Consequently, the efficiency of each line is crucial to the overall speed of your code. As your code becomes more complex, it’s natural to overlook inefficient code, causing slower processing and decreased performance. However, there are several techniques and practices you can employ to optimize your Python code and improve its efficiency.
Profiling Your Code
Profiling is the process of measuring the performance of your code. Python offers many profiling tools like cProfile and PyCharm that make profiling code a breeze. By utilizing these profiling tools, you can efficiently locate the bottlenecks in your code and optimize them to enhance its overall performance.
import cProfile
def my_function(param1,param2):
# ... some code ...
cProfile.run('my_function(param1,param2)')
Avoiding Inefficient Loops
Python, like many programming languages, relies heavily on loops in its operations. However, loops can also be a major source of inefficiency in your code. In particular, nested loops can quickly become a performance bottleneck. Python has numerous built-in functions, including map(), filter(), and reduce(), that can be employed to streamline loops and optimize them for better performance. These functions are often more efficient than manually iterating through a list or dictionary.
map()
The map() function applies a given function to each element of an iterable and returns an iterator of the results.
def square(x):
return x**2
my_list = [1, 2, 3, 4, 5]
squared = map(square, my_list)
print(list(squared)) # Output: [1, 4, 9, 16, 25]
filter()
The filter() function returns an iterator containing the elements from an iterable for which a given function returns True.
def is_even(x):
return x % 2 == 0
my_list = [1, 2, 3, 4, 5]
evens = filter(is_even, my_list)
print(list(evens)) # Output: [2, 4]
reduce()
The reduce() function applies a given function to the first two elements of an iterable, then to the result and the next element, and so on, until all elements have been processed.
def is_even(x):
return x % 2 == 0
my_list = [1, 2, 3, 4, 5]
evens = filter(is_even, my_list)
print(list(evens)) # Output: [2, 4]
List Comprehensions
List comprehensions provide a way to generate lists in Python, and are often more efficient than traditional loops as they can be executed in a single pass. They offer an elegant syntax that can simplify code and increase readability. For instance, instead of writing a for loop to create a list of even numbers, you can use a list comprehension: [x for x in range(10) if x % 2 == 0]. This makes the code more readable and easier to understand, especially when dealing with complex loops.
# Using a loop
squares = []
for i in range(10):
squares.append(i**2)
# Using a list comprehension
squares = [i**2 for i in range(10)]
Using Built-in Functions
Python comes with numerous built-in functions that can be employed to improve the efficiency of your code. These functions are designed to perform specific tasks and are optimized for performance, making them faster and more reliable than writing the code manually. For instance, instead of manually counting the elements in a list or dictionary, you can use the len() function, which is significantly faster. Similarly, instead of manually adding up the elements in a list, you can use the sum() function, which can process large lists much faster. Incorporating these pre-defined functions provided by Python can assist you in streamlining your code and enhancing its efficiency.
# Using a for loop to sum a list
my_list = [1, 2, 3, 4, 5]
total = 0
for i in my_list:
total += i
# Using the built-in sum() function
total = sum(my_list)
Lazy Evaluation
Lazy evaluation is a powerful programming concept that can help to improve the performance of your Python code. It involves delaying the evaluation of an expression until it is actually needed. This can be accomplished in Python through the use of generators and iterators. By taking advantage of lazy evaluation, you can reduce unnecessary computation and optimize your code for faster execution.
Lazy evaluation with Generators
Generators, a form of iterator, have the ability to produce values on-demand. Unlike lists, generators do not hold all values in memory simultaneously, rendering them more efficient in terms of memory usage. A generator can be created in a function using the yield keyword. By utilizing generators, you can effectively reduce memory consumption and optimize the performance of your code.
# Using a list to store all values
def my_function():
result = []
for i in range(10):
result.append(i**2)
return result
# Using a generator
def my_generator():
for i in range(10):
yield i**2
Generators in Python are powerful tools that can be used to create sequences of values on demand. This can be especially useful when working with large data sets that would be too memory-intensive to store in their entirety. By generating values on demand, generators allow you to work with these large data sets in a more efficient and memory-friendly way.
Lazy evaluation with Python’s itertools module
The itertools module in Python offers several functions that are useful for working with iterators and creating sequences of values. For example, the count() function generates an unending sequence of numbers.
from itertools import count
# create an iterator that generates an infinite sequence of numbers
it = count()
# take the first 10 numbers from the sequence
numbers = [next(it) for i in range(10)]
print(numbers) # Output: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
In this example, we use the count() function from the itertools module to generate an infinite sequence of numbers. We then use a list comprehension to take the first 10 numbers from the sequence.
Again, since the sequence is generated lazily, we can use it to generate large or infinite sequences of data without running out of memory.
Avoiding Global Variables
Global variables can be a source of inefficiency in your code. This is because global variables are accessed differently than local variables, which can slow down the execution of your code. Instead of using global variables, consider passing variables as arguments to functions or using class variables.
# Using a global variable
my_var = 0
def my_function():
global my_var
my_var += 1
# ... some code ...
# Using a function argument
def my_function(my_var):
my_var += 1
# ... some code ...
The Power of NumPy
NumPy is a powerful library for scientific computing in Python. It provides fast, efficient data structures and functions for manipulating large arrays and matrices. By using NumPy, you can perform complex computations on large datasets much faster than using traditional Python lists. NumPy is also highly optimized for multi-core CPUs, making it a great choice for parallel computing.
import numpy as np
# Using a list to perform a calculation
my_list = [1, 2, 3, 4, 5]
squared = []
for i in my_list:
squared.append(i**2)
result = sum(squared)
# Using NumPy to perform the same calculation
my_array = np.array([1, 2, 3, 4, 5])
result = np.sum(my_array**2)
Caching Results
Caching is the process of storing the results of a computation so that they can be reused later. By caching results, you can avoid unnecessary computations and improve the performance of your code. There are many caching libraries available for Python, such as functools.lru_cache(), which can automatically cache the results of a function.
from functools import lru_cache
@lru_cache(maxsize=None)
def my_function(x):
# ... some calculation ...
return result
Multiprocessing and Threading
Python provides built-in support for multiprocessing and threading, allowing you to execute multiple tasks in parallel. Multiprocessing is ideal for CPU-bound tasks, while threading is best for I/O-bound tasks. By using multiprocessing and threading, you can take advantage of multi-core CPUs and improve the performance of your code.
from multiprocessing import Pool
import threading
# Using multiprocessing
def my_function(x):
# ... some calculation ...
return result
with Pool(4) as p:
results = p.map(my_function, [1, 2, 3, 4, 5])
# Using threading
def my_function(x):
# ... some calculation ...
return result
threads = []
for i in range(5):
t = threading.Thread(target=my_function, args=[i])
threads.append(t)
t.start()
for t in threads:
t.join()
Using Libraries
Python has a vast ecosystem of libraries that can be used to optimize your code. For example, the pandas library provides fast, efficient data structures for working with tabular data, while the scikit-learn library provides machine learning algorithms optimized for large datasets. By using these libraries, you can avoid reinventing the wheel and improve the performance of your code.
import pandas as pd
from sklearn.linear_model import LinearRegression
# Using traditional Python code to analyze data
my_data = [
[1, 2],
[2, 4],
[3, 6],
[4, 8],
[5, 10]
]
x = [row[0] for row in my_data]
y = [row[1] for row in my_data]
total = 0
for i in
Using a Compiler
Python is an interpreted language, meaning that each line of code is executed one after another. However, you can use a compiler such as Cython to compile your Python code into machine code. By using a compiler, you can significantly improve the performance of your code, especially for CPU-bound tasks.
Testing Your Code
Testing is an essential part of software development, and it’s especially important for optimizing your code. By writing automated tests, you can ensure that your optimizations do not introduce new bugs or regressions. Python provides many testing frameworks, including unittest and pytest, that can be used to write automated tests for your code.
Conclusion
Optimizing your Python code can be a challenging task, but it’s essential for improving the performance and reliability of your software. By using the tips and tricks outlined in this article, you can make your code faster, more efficient, and easier to maintain. Remember to profile your code, avoid inefficient loops, use built-in functions, and take advantage of libraries and tools such as NumPy, caching, multiprocessing, and threading. By following these best practices, you can ensure that your Python code is running as efficiently as possible.
If you enjoyed this article and want to read more like it, be sure to follow me on Medium! I regularly publish new content on Python,AI and ML ,so you won’t want to miss out ❤️✨
From Slow to Speedy: Tips for Making Your Python Code More Efficient was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Arjun M S
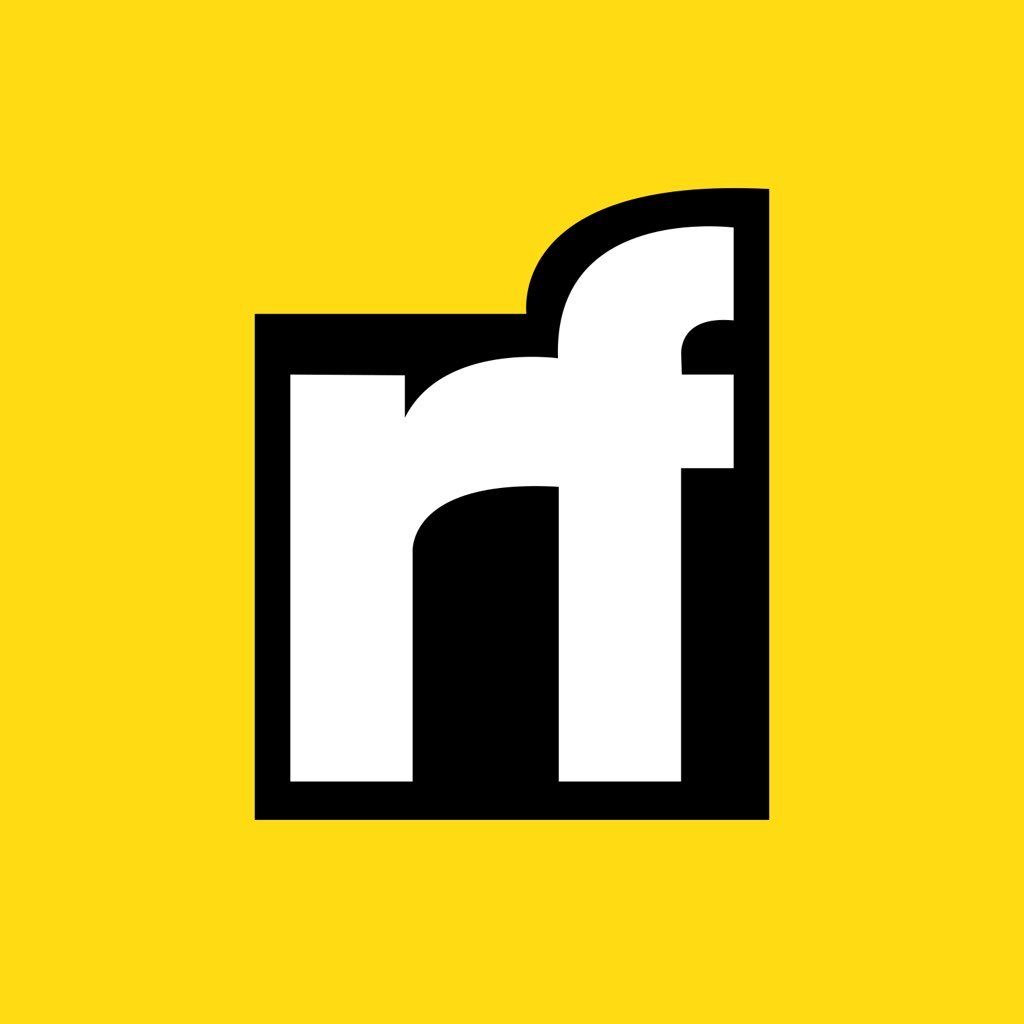
Arjun M S | Sciencx (2023-05-03T02:37:37+00:00) From Slow to Speedy: Tips for Making Your Python Code More Efficient. Retrieved from https://www.scien.cx/2023/05/03/from-slow-to-speedy-tips-for-making-your-python-code-more-efficient/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.