This content originally appeared on Level Up Coding - Medium and was authored by Okan Yenigün
An Introduction to Go Language
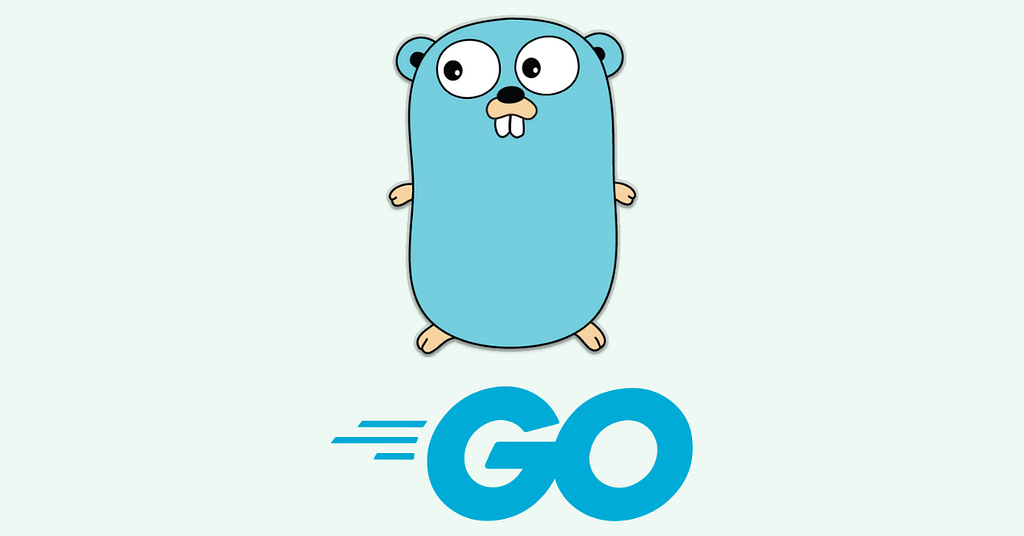
Go (or Golang) is a programming language developed by Google. It is designed in 2007, announced in 2009, and released in 2012. This language is officially called “Go,” but it is also commonly referred to as “Golang” to facilitate its discoverability in online search engines and research. It has a cute mascot called Gopher. At the same time, programmers who use Go are called gophers.
The official website of Go is here. We will frequently visit the documentation here throughout our series. In addition, Awesome Go does an awesome job and lists the packages, libraries, and frameworks of Go.
Legend has it that Go was born out of frustration with slow compilation times. The story goes that three Google engineers, Robert Griesemer, Rob Pike, and Ken Thompson, were working on a large C++ project at Google and grew tired of waiting for the slow C++ compiler to finish compiling their code.
During their wait, they began discussing what features they would like to see in a new language that would be more productive and efficient than C++. They wanted a language that would be easy to learn, with simpler syntax and faster compile times.
So, during their breaks from waiting for the C++ compiler, the three engineers started working on a new language that would meet their requirements. They drew inspiration from several existing languages, including C, Python, and others, and started experimenting with different features and syntaxes.
Over time, their experimentation and development efforts led to the creation of Go, a language that combines the efficiency and performance of C++ with the simplicity and readability of Python. And, as the legend goes, Go’s fast compilation times were a direct result of the team’s frustration with the slow compilation times of C++.
It depends on an individual’s prior experience with programming, but generally speaking, Go is considered to be a relatively easy language to learn. It has a clean syntax and a small set of keywords (25), which makes it easier to read and write compared to some other programming languages.
Go is a cross-platform language, which makes it a versatile language that can be used to develop applications for various platforms and devices.
It is statically typed. This means that variable types must be declared at compile time and cannot be changed at runtime.
Go has a strong commitment to backward compatibility, which means that programs written in earlier versions of the language should continue to compile and run without any issues on newer versions of the language. The Go team takes great care to ensure that new language features or changes to the standard library do not break existing code. This dedication to backward compatibility makes Go a reliable language choice for long-term projects.
It supports language-level concurrency. There is no framework in between for that.
Go has a garbage collector that automatically manages memory allocation and deallocation in a program. This means that programmers do not need to manually allocate or deallocate memory, which can help reduce the risk of memory leaks and other common memory-related errors.
In general, compiling a Go program is relatively fast compared to some other compiled languages. Go’s compiler is designed to be fast and efficient, and it can typically compile large programs in a matter of seconds, even on older hardware.
There are no warnings during compilation. The code either compiles successfully or it doesn’t. If there are any unused variables or imports in the code, Go will throw an error.
In Go, there is usually a recommended way to perform a task or accomplish a goal. If a feature or approach is not commonly used, it may be viewed as unnecessary and therefore not added to the language. This is in contrast to languages like Python, where there may be multiple ways to achieve the same outcome. In Go, the preferred and most effective approach is typically implemented and used. This focus on standardization and optimization helps ensure that Go programs are reliable, efficient, and easy to maintain.
When learning Go, it is important to approach it with an open mind and not rely too heavily on previous experience with other programming languages. Go is designed with a distinct philosophy and may lack some features that are common in other languages. Therefore, it is essential to understand Go’s unique mindset and learn how to work within its design.
Go can be compiled into a single binary file, making it easy to distribute and share.
We don’t have classes in Go, we have structs instead. It is not a pure object-oriented programming language. While Go does support some object-oriented programming concepts, such as struct types, methods, and interfaces, it does not include all the features commonly associated with object-oriented languages, such as inheritance and class-based polymorphism. It does not have any kind of overloading or overriding, too. And to your surprise, it does not have try and catch mechanism.
Based on the findings of the 2019 Go Developer Survey, the three most common fields where Go is utilized are web development, databases, and networks.
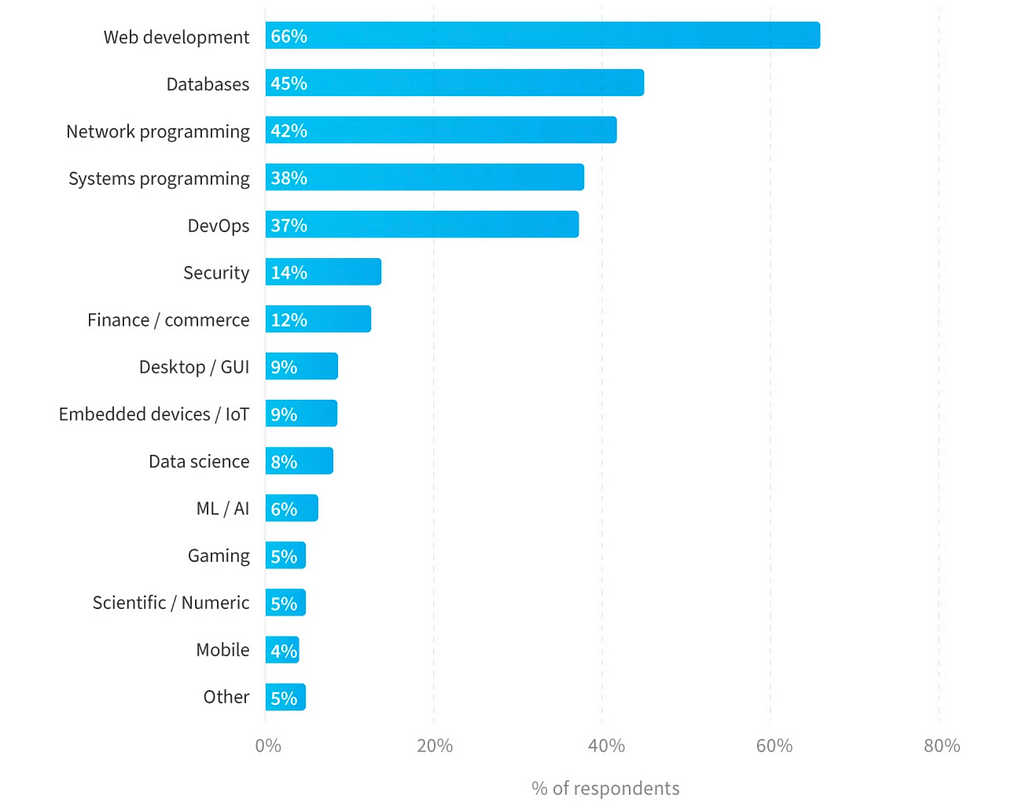
It is used worldwide and in all the famous companies and projects you can think of.
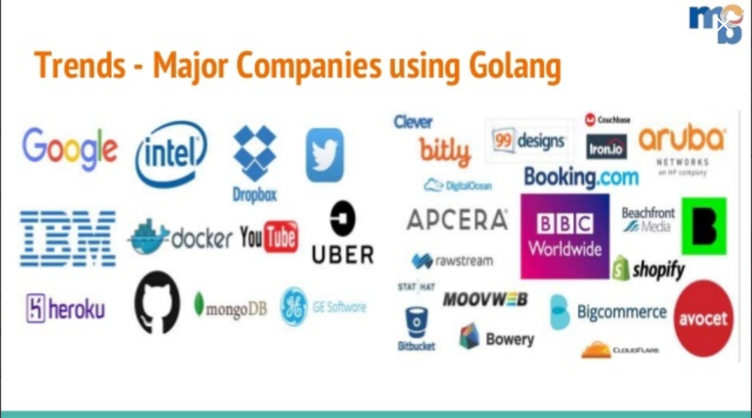
It is also one of the most demanded programming languages.
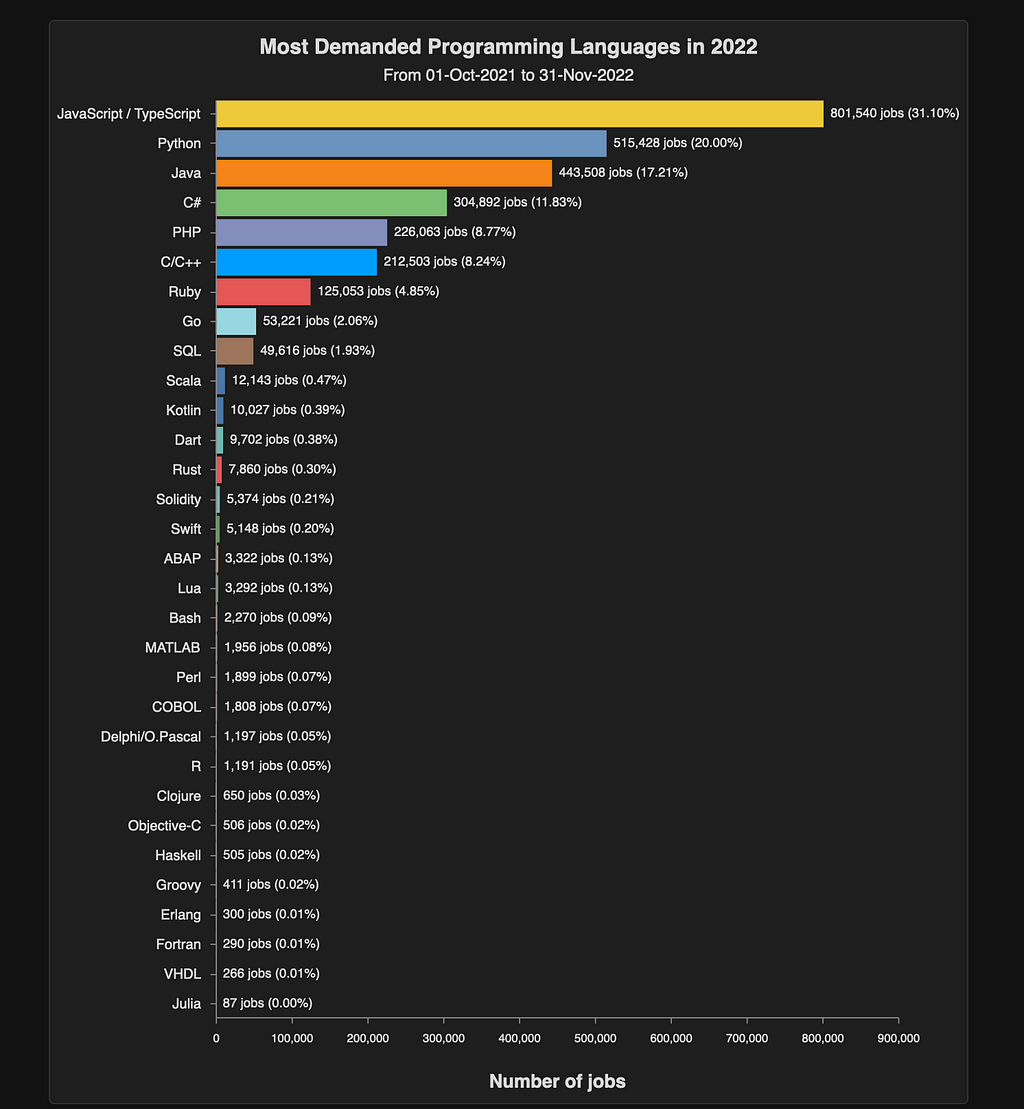
You can follow here to install Go into your OS. Plus, Go has an online IDE. You can play around with Go using it.
After installing it, open up VS Code. Check if you have the Go extension. If not, install it. Then, create a file named main.go.
package main
import "fmt"
func main() {
fmt.Println("Hello World")
}
package main - This line specifies that this file is part of the main package, which is a special package that can be executed as a program. Every Go program must have a main package.
import "fmt" - This line imports the fmt package, which provides functions for formatting and printing strings to the console.
func main() { - This line defines the main function, which is the entry point of the program. The main function is automatically called when the program is executed.
Open up an integrated terminal in the directory of the main.go file and type go run main.go. Ta daa! You have run your first program in Go!
Read More
- How Does Python Work?
- Scala #1: An Introduction
- Multiprocessing in Python
- Harnessing the Power of Stochasticity for Better Predictions
- ANOVA Test in Python
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Learning Go: #1 Getting Started was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Okan Yenigün
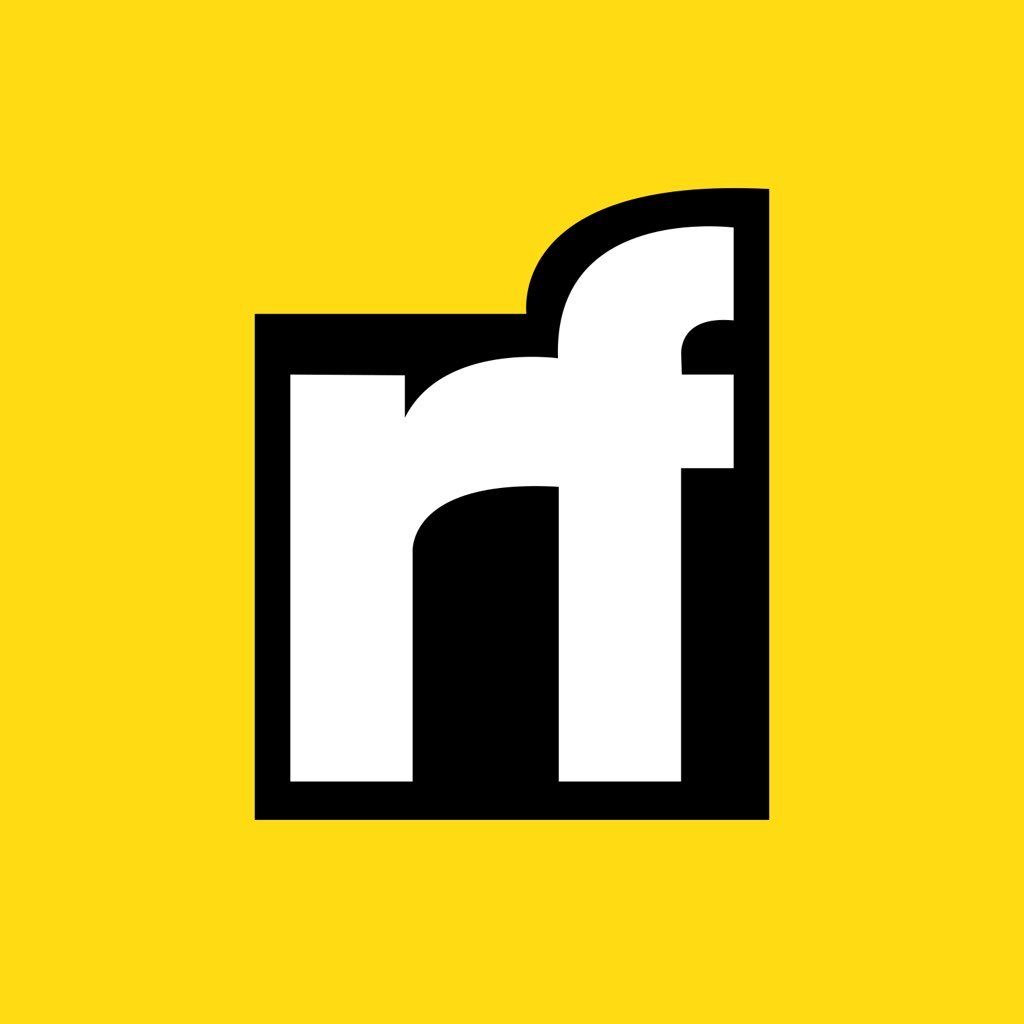
Okan Yenigün | Sciencx (2023-05-07T21:33:16+00:00) Learning Go: #1 Getting Started. Retrieved from https://www.scien.cx/2023/05/07/learning-go-1-getting-started/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.