This content originally appeared on Level Up Coding - Medium and was authored by Anto Semeraro
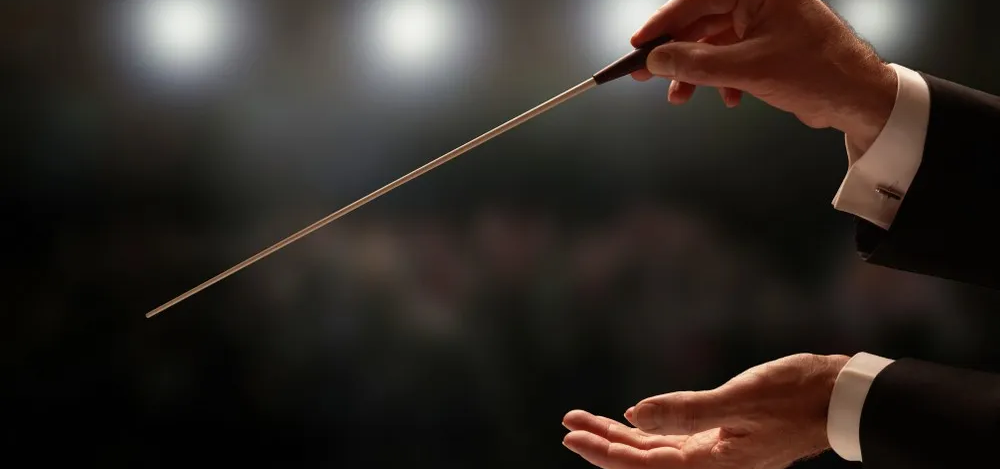
Software Architecture Patterns
Optimizing microservices communication and coordination through orchestration pattern with an example in C#
Welcome to the exciting world of microservices orchestration! As businesses rapidly move towards digitalization, there is an ever-increasing need for software architectures that can keep up with the changing demands of the market.
In this article, I will explore the benefits, challenges, and best practices of microservices orchestration, and why it’s become a crucial component of modern software development.
What are Microservices?
Microservices are a modern software development approach that breaks down complex applications into small, independent services that can be developed, deployed, and scaled independently. Unlike traditional monolithic applications, microservices allow for more agile development and easier maintenance, as well as faster deployment and scalability.
What is Microservices Orchestration?
However, with the advantages of microservices come new challenges in managing the complexity of a distributed system. That’s where microservices orchestration comes in.
Microservices orchestration is the process of managing and coordinating the interactions between multiple microservices to achieve a specific business goal.
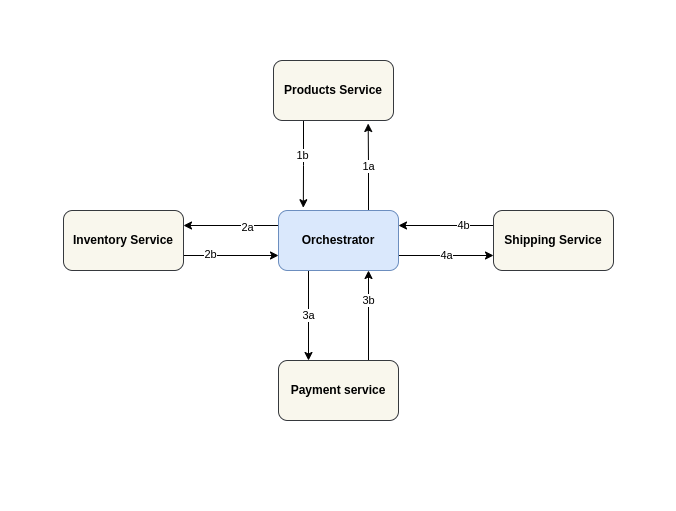
Microservices orchestration is important because it enables a more effective and efficient way to manage complex distributed systems. It provides a framework for managing the dependencies between microservices and ensuring that they work together seamlessly.
Without proper orchestration, microservices can become difficult to manage, and the system as a whole can become unreliable.
It’s worth to mention that an alternative approach is microservices choreography, a central component manages the flow of messages between services, in choreography, each service is responsible for its own message exchange, creating a decentralized architecture that can scale easily. But let’s dive into microservices orchestration here.
The Role of Orchestration in Microservices Architecture
Microservices architecture is a distributed system made up of multiple independent microservices that communicate with each other to fulfill a specific business goal.
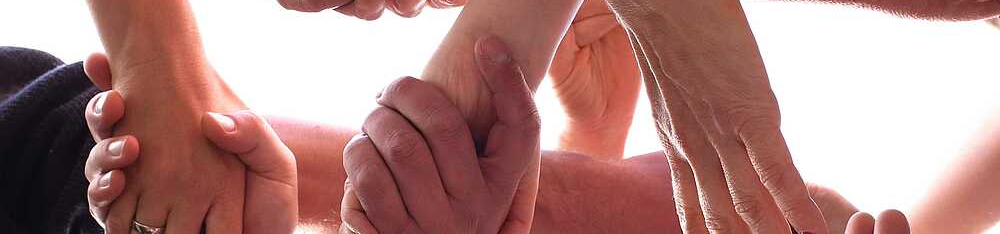
While this architecture provides many benefits, it also introduces complexity in managing the interactions between microservices. This is where microservices orchestration comes in, as it plays a crucial role in managing the interactions and dependencies between microservices.
Service Discovery and Communication
Microservices orchestration helps manage service discovery and communication. With many microservices running independently, it can become difficult to keep track of which services are available, their addresses, and how to communicate with them.
Orchestration tools provide a centralized service registry that tracks the available services and their network addresses. This enables the orchestration tool to route requests to the appropriate service and facilitate communication between services.
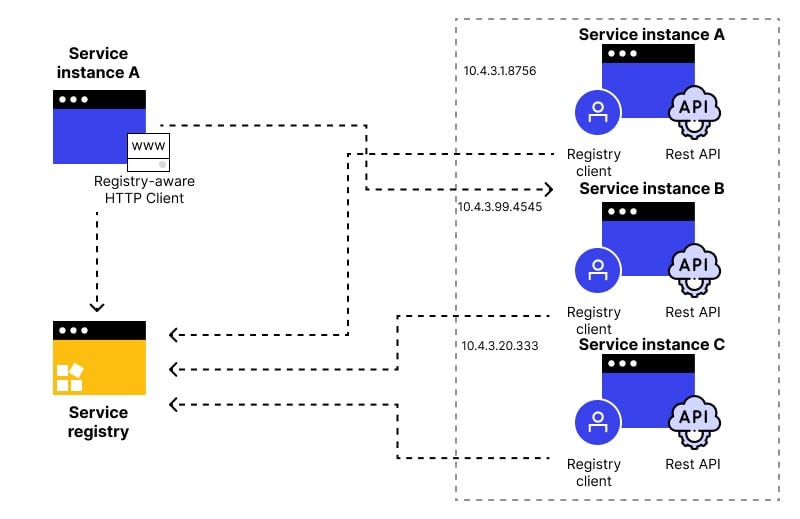
Fault Tolerance
Fault tolerance is another key feature of microservices orchestration. In a distributed system, it’s inevitable that failures will occur. Orchestration tools provide mechanisms for handling and recovering from these failures.
If a service becomes unavailable, the orchestration tool can automatically redirect requests to a backup service, ensuring that the system remains operational.
Scalability
Microservices orchestration also helps in scaling the system horizontally. As the demand for a service increases, orchestration tools can automatically spin up additional instances of that service to handle the increased load.
Orchestration tools can help balance the load across multiple instances of a service, ensuring that each instance is utilized optimally.
Tools for Microservices Orchestration
Microservices orchestration tools provide a way to manage the complexity of a microservices architecture. These tools help in service discovery, communication, fault tolerance, and scalability.
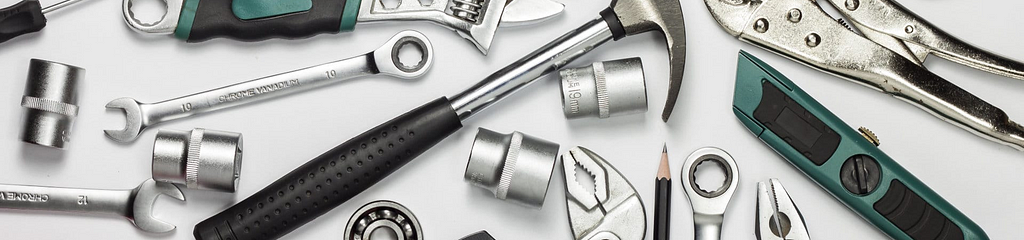
There are many tools available for microservices orchestration, each with its own strengths and weaknesses.
Kubernetes
Kubernetes is a popular open-source container orchestration tool that provides a platform for managing containerized applications. It provides a powerful set of features for deploying, scaling, and managing microservices. Kubernetes is highly extensible, making it an attractive option for many organizations. However, its complexity can be a challenge for organizations without skilled personnel.
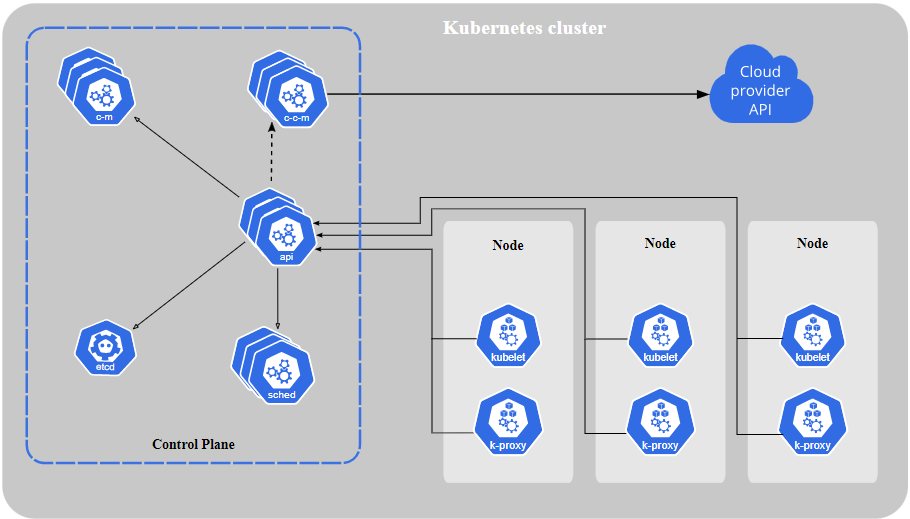
Docker Swarm
Docker Swarm is another container orchestration tool that is part of the Docker ecosystem. It’s simpler to set up and use than Kubernetes, making it a popular choice for smaller organizations. Docker Swarm provides a basic set of features for deploying and scaling microservices.
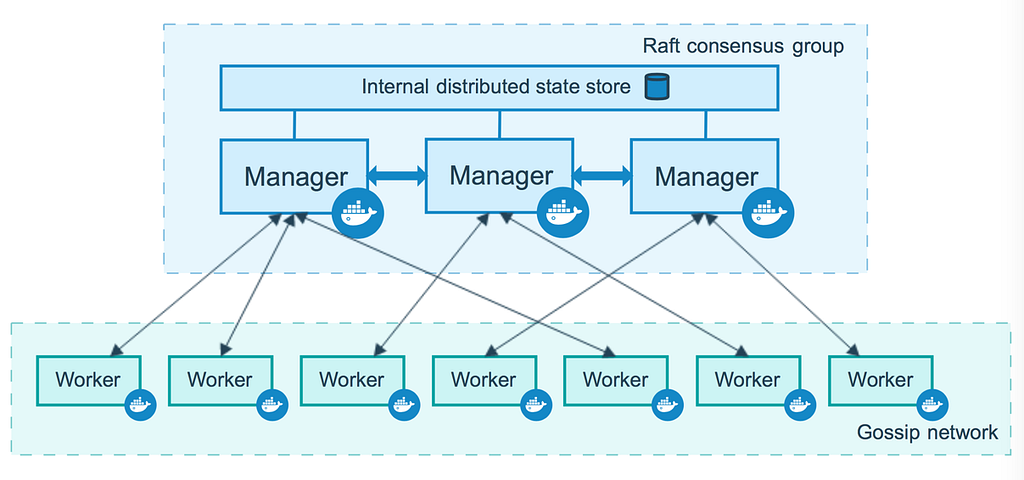
Apache Mesos
Apache Mesos is a popular open-source cluster manager that provides a platform for managing distributed systems. It provides a highly scalable and fault-tolerant platform for running microservices. Mesos is highly extensible and can integrate with many different technologies. However, its complexity can be a challenge for organizations without skilled personnel.
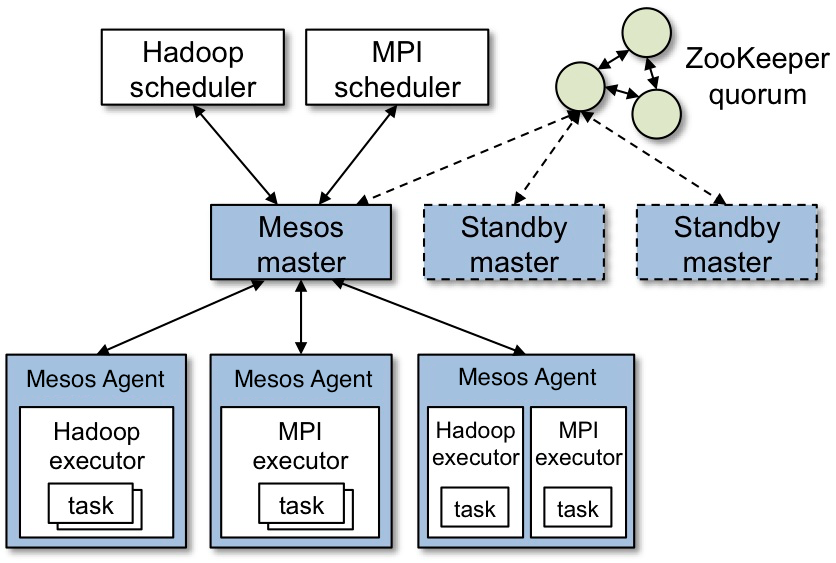
A Basic Implementation of Orchestration Pattern
If you want customize the orchestration process, here’s a very basic example of how you can implement microservices orchestration pattern with C# using a simple e-commerce application as instance.
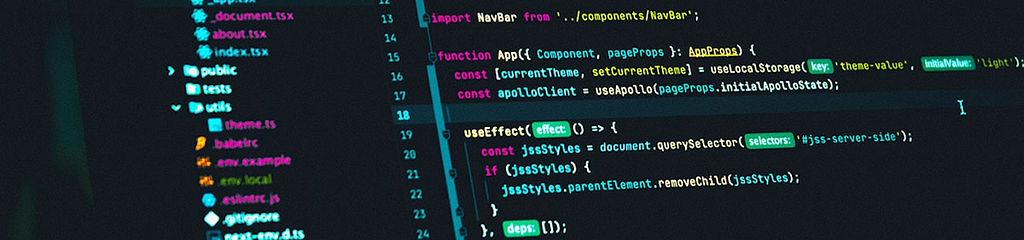
Let’s say we have three microservices:
- Product Service: Handles the management of products.
- Order Service: Handles the management of orders.
- User Service: Handles the management of users.
In this scenario, let’s use a central orchestrator service to manage communication between these microservices.
Define Contracts: first, let’s define the contracts for each microservice using an interface in a shared library. This interface specifies the methods and parameters for each microservice.
public interface IProductService
{
Task<Product> GetProductById(int productId);
Task<IEnumerable<Product>> GetProducts();
Task<bool> UpdateProduct(Product product);
Task<bool> DeleteProduct(int productId);
}
public interface IOrderService
{
Task<Order> GetOrderById(int orderId);
Task<IEnumerable<Order>> GetOrders();
Task<bool> UpdateOrder(Order order);
Task<bool> DeleteOrder(int orderId);
}
public interface IUserService
{
Task<User> GetUserById(int userId);
Task<IEnumerable<User>> GetUsers();
Task<bool> UpdateUser(User user);
Task<bool> DeleteUser(int userId);
}
Implement Microservices: next, implement each microservice using the defined interfaces. Each microservice will have its own database and business logic.
public class ProductService : IProductService
{
private readonly IDbContext _dbContext;
public ProductService(IDbContext dbContext)
{
_dbContext = dbContext;
}
public async Task<Product> GetProductById(int productId)
{
return await _dbContext.Products.FirstOrDefaultAsync(p => p.Id == productId);
}
// Other methods...
}
public class OrderService : IOrderService
{
private readonly IDbContext _dbContext;
public OrderService(IDbContext dbContext)
{
_dbContext = dbContext;
}
public async Task<Order> GetOrderById(int orderId)
{
return await _dbContext.Orders.FirstOrDefaultAsync(o => o.Id == orderId);
}
// Other methods...
}
public class UserService : IUserService
{
private readonly IDbContext _dbContext;
public UserService(IDbContext dbContext)
{
_dbContext = dbContext;
}
public async Task<User> GetUserById(int userId)
{
return await _dbContext.Users.FirstOrDefaultAsync(u => u.Id == userId);
}
// Other methods...
}
Implement Orchestrator: finally, the orchestrator service will manage communication between the microservices. The orchestrator service will use the interfaces defined in the contracts to communicate with the microservices.
public class OrchestratorService
{
private readonly IProductService _productService;
private readonly IOrderService _orderService;
private readonly IUserService _userService;
public OrchestratorService(IProductService productService, IOrderService orderService, IUserService userService)
{
_productService = productService;
_orderService = orderService;
_userService = userService;
}
public async Task<Product> GetProductById(int productId)
{
return await _productService.GetProductById(productId);
}
public async Task<IEnumerable<Product>> GetProducts()
{
return await _productService.GetProducts();
}
public async Task<bool> UpdateProduct(Product product)
{
return await _productService.UpdateProduct(product);
}
public async Task<bool> DeleteProduct(int productId)
{
return await _productService.DeleteProduct(productId);
}
// Repeat for OrderService and UserService...
}
With this implementation, the orchestrator service can now be used to manage communication between the microservices. For example, if a client wants to get the details of an order, the orchestrator service can use the GetOrderById method in the OrderService microservice to retrieve the necessary data.
This approach allows us to achieve the benefits of microservices, such as scalability and fault tolerance, while maintaining a level of abstraction that makes it easier to manage the complexity of the system.
Best Practices for Microservices Orchestration
Microservices architecture offers numerous benefits, including scalability, fault tolerance, and flexibility. H
Orchestrating these services can be complex and challenging, particularly as the number of services increases.
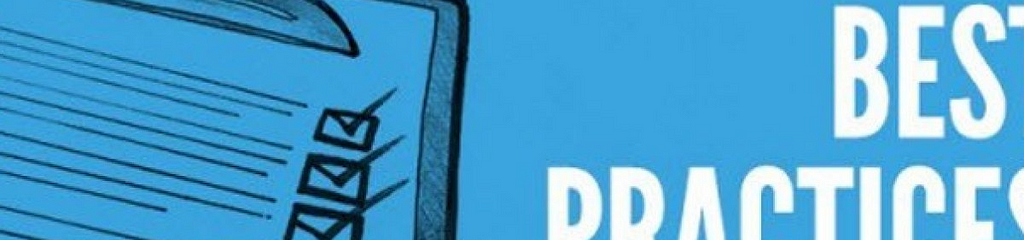
Deployment Strategies
One of the most important decisions in microservices orchestration is how to deploy and manage services. Some common deployment strategies include blue-green deployments, canary releases, and rolling updates. It’s essential to select the right deployment strategy based on your requirements, architecture, and organizational culture.
Service Discovery
Service discovery is a critical aspect of microservices orchestration, as it enables services to locate and communicate with each other. Some popular service discovery tools include ZooKeeper, Consul, and etcd. It’s essential to carefully consider the performance, scalability, and fault tolerance of your service discovery solution.
Load Balancing
Load balancing helps distribute traffic across multiple instances of a service, ensuring high availability and scalability. There are several load balancing strategies, including round-robin, least connections, and IP hash. It’s important to choose the right load balancing strategy for your application based on factors such as traffic patterns, service availability, and performance requirements.
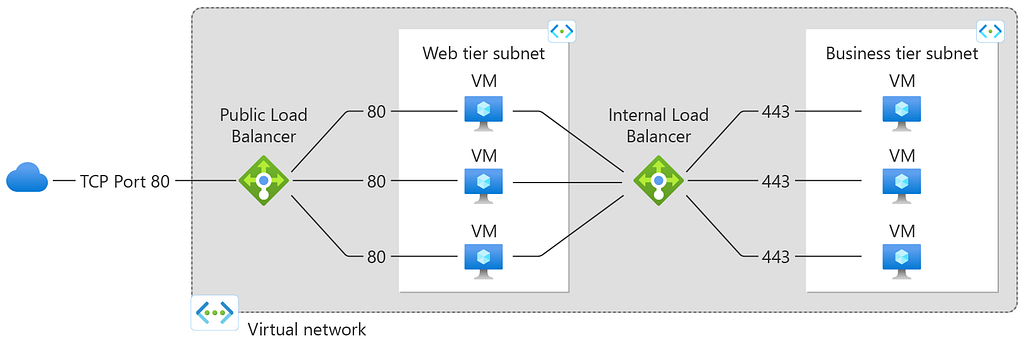
Monitoring
Effective monitoring is essential for detecting and diagnosing issues in your microservices architecture. There are several tools for monitoring microservices, including Prometheus, Grafana, and Zipkin. It’s important to choose a monitoring solution that provides real-time visibility into the performance and health of your services and enables proactive issue resolution.
Security and Compliance
Microservices orchestration can create new security and compliance challenges, particularly as services are distributed across multiple nodes and environments. It’s important to carefully consider security and compliance requirements and implement appropriate measures such as network segmentation, encryption, and access control.
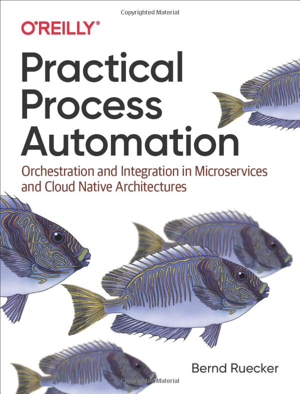
Hey there, just a quick heads up! When you purchase a book through the links I’ve shared in this post, I receive a small commission at no extra cost to you. It’s a great way to support my work while discovering awesome reads. Thanks for your support, and happy reading!
Challenges of Microservices Orchestration
While microservices offer significant benefits, such as scalability, agility, and flexibility, implementing and managing microservices orchestration can also pose several challenges.
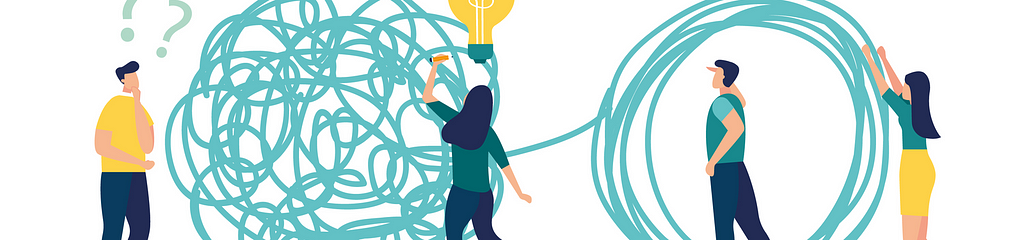
Next section will explore some of the common challenges associated with microservices orchestration.
Skilled Personnel
Microservices orchestration requires specialized skills in areas such as service discovery, load balancing, and container orchestration. Hiring, training, and retaining personnel with these skills can be a significant challenge for organizations.
Maintaining Consistency
In a distributed system, ensuring consistency across services can be challenging. Services may have different versions, dependencies, and configurations, leading to issues such as incompatible APIs and inconsistent data. Organizations must implement strategies such as versioning and compatibility checks to maintain consistency across services.
Ensuring Security and Compliance
Microservices orchestration can create new security and compliance challenges, particularly as services are distributed across multiple nodes and environments. Organizations must carefully consider security and compliance requirements and implement appropriate measures such as network segmentation, encryption, and access control.
Debugging and Troubleshooting
In a microservices architecture, issues can arise at any layer, from the infrastructure to the application code. Debugging and troubleshooting can be challenging, particularly as services are distributed across multiple nodes and environments. Organizations must implement effective monitoring and logging strategies to diagnose and resolve issues quickly.
Managing Complexity
Microservices orchestration can significantly increase the complexity of an application, particularly as the number of services and nodes increases. Organizations must implement effective governance and management practices, such as service cataloging, versioning, and monitoring, to manage the complexity of their microservices architecture.
While microservices orchestration offers significant benefits, implementing and managing it can be complex and challenging. Organizations must carefully consider the challenges associated with microservices orchestration and implement appropriate measures to address them. By doing so, they can effectively leverage microservices architecture to deliver scalable, resilient, and flexible applications.
Future Directions of Microservices Orchestration
Microservices orchestration has rapidly evolved over the years, driven by the need for scalable, resilient, and flexible applications.
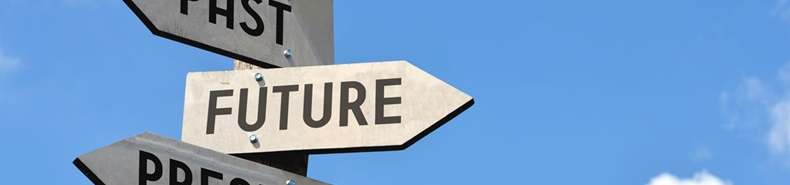
Microservices orchestration is evolving rapidly, driven by emerging trends such as serverless architectures, edge computing, artificial intelligence, hybrid cloud, and standardization.
Organizations must carefully consider these trends and incorporate them into their microservices orchestration strategies to stay ahead of the curve and deliver innovative, scalable, and resilient applications.
Here are some of the emerging trends and future directions in microservices orchestration.
Serverless Architectures
Serverless architectures are gaining popularity in the microservices ecosystem, as they enable software engineers to focus on application logic without worrying about infrastructure management. Serverless platforms such as AWS Lambda and Azure Functions provide a scalable and cost-effective approach to microservices orchestration.
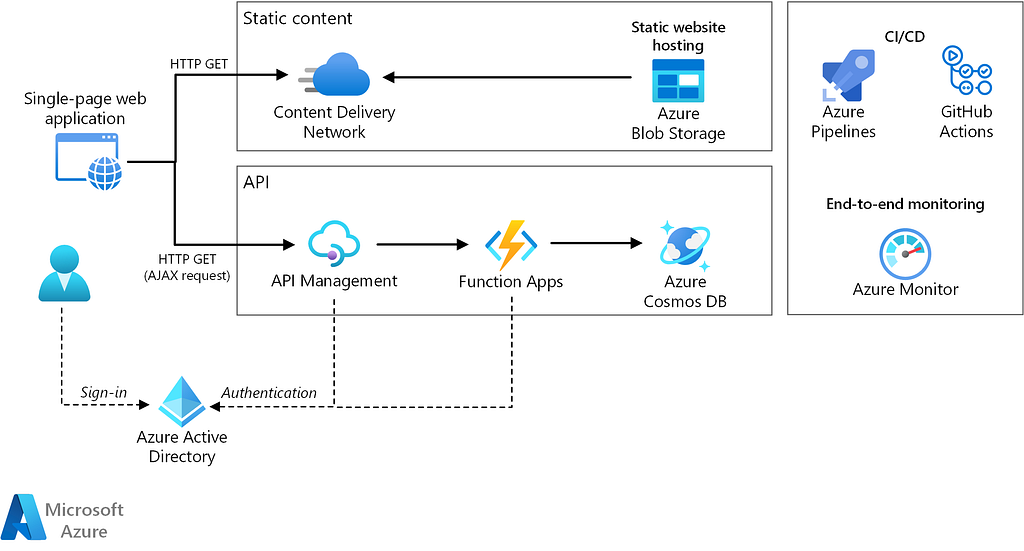
Edge Computing
Edge computing brings computing resources closer to the edge of the network, reducing latency and improving application performance. Microservices orchestration can leverage edge computing to improve scalability, fault tolerance, and data processing capabilities.
Artificial Intelligence
Artificial intelligence and machine learning can enhance microservices orchestration by providing intelligent automation, predictive analytics, and proactive issue resolution. Tools such as AI-driven monitoring and decision-making systems can help organizations optimize their microservices architecture.
Hybrid Cloud
With the rise of hybrid cloud adoption, microservices orchestration must support applications deployed across multiple clouds and on-premise environments. Tools such as Istio and Linkerd can provide seamless orchestration across heterogeneous environments.
Standardization
As microservices continue to proliferate, standardization becomes increasingly important. The Cloud Native Computing Foundation (CNCF) is leading efforts to standardize microservices orchestration tools such as Kubernetes, enabling interoperability and ease of use across different platforms.
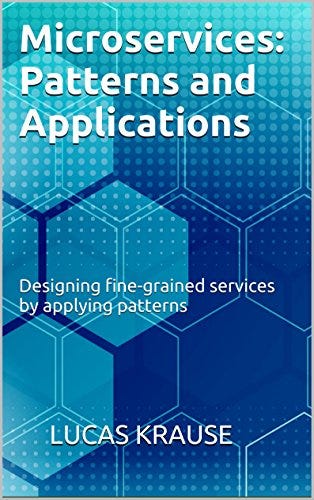
Microservices orchestration offers significant benefits for organizations looking to develop scalable, resilient, and flexible applications.
If you want to learn more about Microservices, I highly recommend reading this article to dig into an architectural alternative, Microservices Choreography:
Microservices Choreography Best Practices and Tools
And, please if you liked this article, don’t forget to follow me for more rocky content like this! 😎🚀 #Medium #FollowMe
Bibliographic References
- “Microservices: Patterns and Applications: Designing fine-grained services by applying patterns”, Lucas Krause, 2015
- “Microservices: Up and Running: A Step-by-Step Guide to Building a Microservices Architecture”, Ronnie Mitra, Irakli Nadareishvili, 2020, O’Reilly Media
- “Practical Process Automation: Orchestration and Integration in Microservices and Cloud Native Architectures”, Bernd Ruecker, 2021, O’Reilly Media
- “Microservices Architecture — A Game Changer: A Guide to Understand Designing, Developing, and Deploying Scalable and Resilient Distributed Systems”, Badal Tripathy, 2023
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Microservices Orchestration Best Practices and Tools was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anto Semeraro
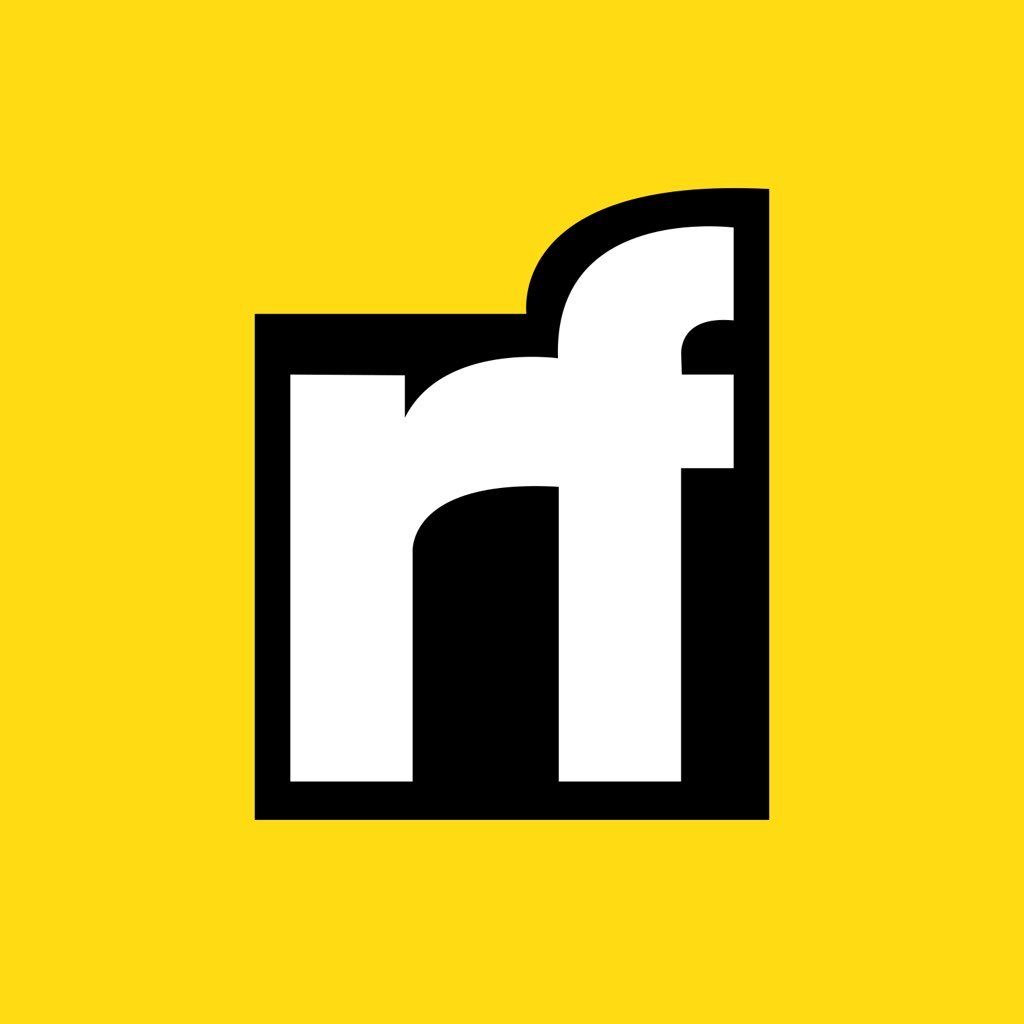
Anto Semeraro | Sciencx (2023-05-07T21:26:58+00:00) Microservices Orchestration Best Practices and Tools. Retrieved from https://www.scien.cx/2023/05/07/microservices-orchestration-best-practices-and-tools/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.