This content originally appeared on Level Up Coding - Medium and was authored by lance
Here are some tips to teach you how to name your code.
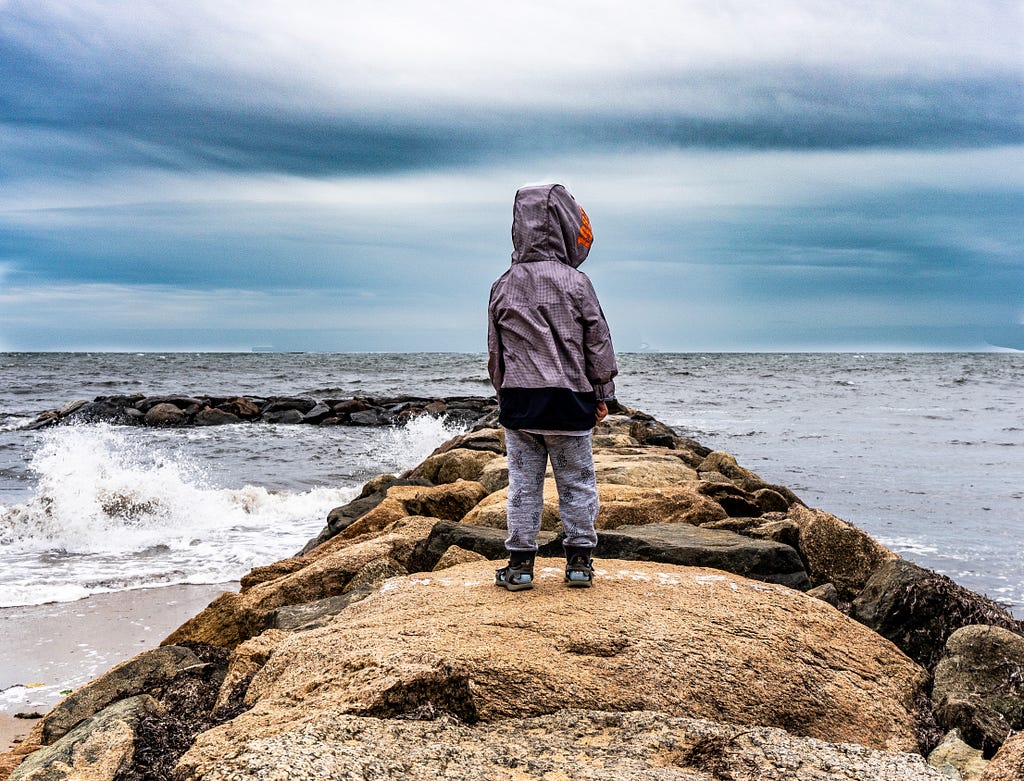
In our daily lives, we are constantly naming different things, giving names to newborns, giving names to cute pets, and giving different names to different flowers. Naming is very important, giving a meaningful name to a transaction can clarify what you are talking about. Naming is equally important in the code world, as it is needed everywhere in the code. As programmers, we need to name classes, variables, functions, and so on. But naming in the code cannot be the same as naming your pet for classes, methods, or variables just because it’s cute and kind. When writing code, if you name it as you please, it is very likely that after a while you will not be able to understand the code you have written yourself.
Why is it difficult to name correctly?
Many people believe that naming is the most difficult thing in programming and often rack their brains for a class name. In this case, unless your vocabulary is too small, it is likely that your code already has a lot of smells.
- A class does too many or few things.
- You don’t have enough understanding of the requirements, you need to obtain more information first.
- Use a lot of comments to explain your code.
So when you spend a lot of time on naming, my first suggestion is to take a look at the code again and see if it doesn’t follow the six design principles. Then I recommend you to read the book Clean Code and Refactoring.
Although code naming is more of a subjective operation, you can still improve your naming skills through some techniques.
- Naming basic tricks
- Class name. The class name should be a noun or noun phrase, such as User, PropertiesHandler, PriceCalculator, etc. It is best to indicate the properties of abstract classes, such as AbstractContext, baseExecutor, etc.
- Variable name. The variable name should also be a noun. They are attributes of the class.
- Method name. The method name should be a verb or verb phrase, such as invoiceApi(), apply(), bulkInsert(), etc.
2. Use names that express intentions
A proper name can tell us what it is, what it wants to do, why it exists, and how it works.
Case:
// the class startup flag
private boolean flag = false;
public void run() {
if(!flag) {
// do something
}
}
What does flag mean? Looking at the comment, it is known that it is the startup flag of the class. The semantic meaning of this word is too large, and it may express different meanings in many scenarios. Therefore, it is necessary to rename a name that can express the intention.
Modification suggestions:
// the class startup flag
private boolean started = false;
public void run() {
if(!started) {
// do something
}
}
2. Refuse vague vocabulary and accurately express meaning
Case:
public void downloadExcel(HttpServletResponse response) {
List<File> files = listFile();
String fileName = System.currentTimeMillis()+".zip";
DownloadZip.downloadFiles(files,filePath);
DownlaodZip.fileDownload(response,filePath,fileName);
}
We may doubt, what is the difference between downloadFilesandfileDownload? Why call twice? Continuing to read the code will reveal that the function downloadFiles is to create files and package them into zip files while fileDownload outputting the specified files to the browser for download. So downloadFiles should be named n createZipFile to accurately express the meaning of the method.
3. Ensure parameter readability and avoid abbreviations
When the parameter name is too long, many people will abbreviate the parameter. If this abbreviation is an international convention, then there is no problem. If it is not, it will make people confused. I simply cannot understand what this is.
Case:
for(CustomerShippingAddress csa: customerShippingAddresses) {
if(csa!=null) {
allAddressIds.remove(csa.id);
}
}
Who can explain what csa is? If this abbreviation appears in the code, it will definitely need to be renamed. In the above example, it is okay to know that it is the acronym of customerShippingAddressbased on the type name. If the logic of this method is complex and the entire code logic is filled with abbreviations such as csa, you may forget the meaning of this parameter after reading the code and have to go back to the code line where the parameter is defined to read it again.
Modification suggestions:
for(CustomerShippingAddress customerShippingAddr: customerShippingAddresses) {
if(csa!=null) {
allAddressIds.remove(customerShippingAddr.id);
}
}
4. Avoid returning types that are not consistent with method name definitions
Case:
private List<CustomerShippingAddress> checkCustomerShippoingAddress(List<CustomerShippingAddress> addresses) {
if(Collections.isEmpty(addresses)) {
throw new Exception("the addresses is empty!");
}
return addresses.stream().filter(address->address!=null).collect(Collectors.toList());
}
Should the check method not have a boolean type if it has a return value? Why is the return value of a List type?
Continuing to read the code, the original method did non-null validation, and then a filtering operation was performed to filter out elements that did not meet the requirements. So the name of this method is not appropriate, which may cause ambiguity when viewed.
Modification suggestions: delete this method.
if(Collections.isEmpty(addresses)) {
throw new Exception("the addresses is empty!");
}
var validAddresses = addresses.stream().filter(address->address!=null).collect(Collectors.toList());
5. One concept, one word
Choose a word for an abstract concept and don’t change it. For example, get(), fetch(), and query(). Putting these methods into the same class can be confusing, as several words have similar meanings. To understand the differences, one can only read the code in detail. So it is recommended to select a word for an abstract concept in a project to avoid ambiguity and maintain consistent vocabulary, which is an important tool for programmers to navigate their code.
6. Add meaningful context
Most names themselves are meaningless and need to be placed in specific contexts in order for readers of the code to understand what they refer to.
Case:
@Component
public class DefaultProcessor implements Processor {
private static final Logger logger = LoggerFactory.getLogger(DefaultProcessor.class);
@Override
public BigDecimal processInputData(InsurancePolicy policy) throws Exception{
if(!checkPolicy(policy)) {
thow new Exception("the policy is illegal!");
}
var calInfo = generatePremiumCalculateInfo(policy);
var premium = calculatePremium(calInfo);
return premium;
}
The above class is used to calculate insurance policy premiums, but the class name uses a meaningless name of DefaultProcessor. The method name is named processInputData. This method name is very abstract, and we simply cannot understand the logic of this code through this method name. The best solution to this situation is to refactor this method.
Modification suggestions:
@Component
public class InsurancePolicyProcessor implements Processor {
private static final Logger logger = LoggerFactory.getLogger(InsurancePolicyProcessor.class);
@Override
public BigDecimal calculatePremium(InsurancePolicy policy) throws Exception{
if(!checkPolicy(policy)) {
thow new Exception("the policy is illegal!");
}
var calInfo = generatePremiumCalculateInfo(policy);
var premium = doCalculatePremium(calInfo);
return premium;
}
Conclusion
The purpose of naming is to facilitate a dialogue between code and engineers, enhance the readability and maintainability of the code, and excellent code can often be understood by words. This article explains the importance of naming and some suggestions for developing good naming habits through case studies, hoping to be helpful to everyone. It is also recommended that everyone be strict with themselves and have the willingness to create meaningful names for each parameter when writing code.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
How To Name Your Code? was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by lance
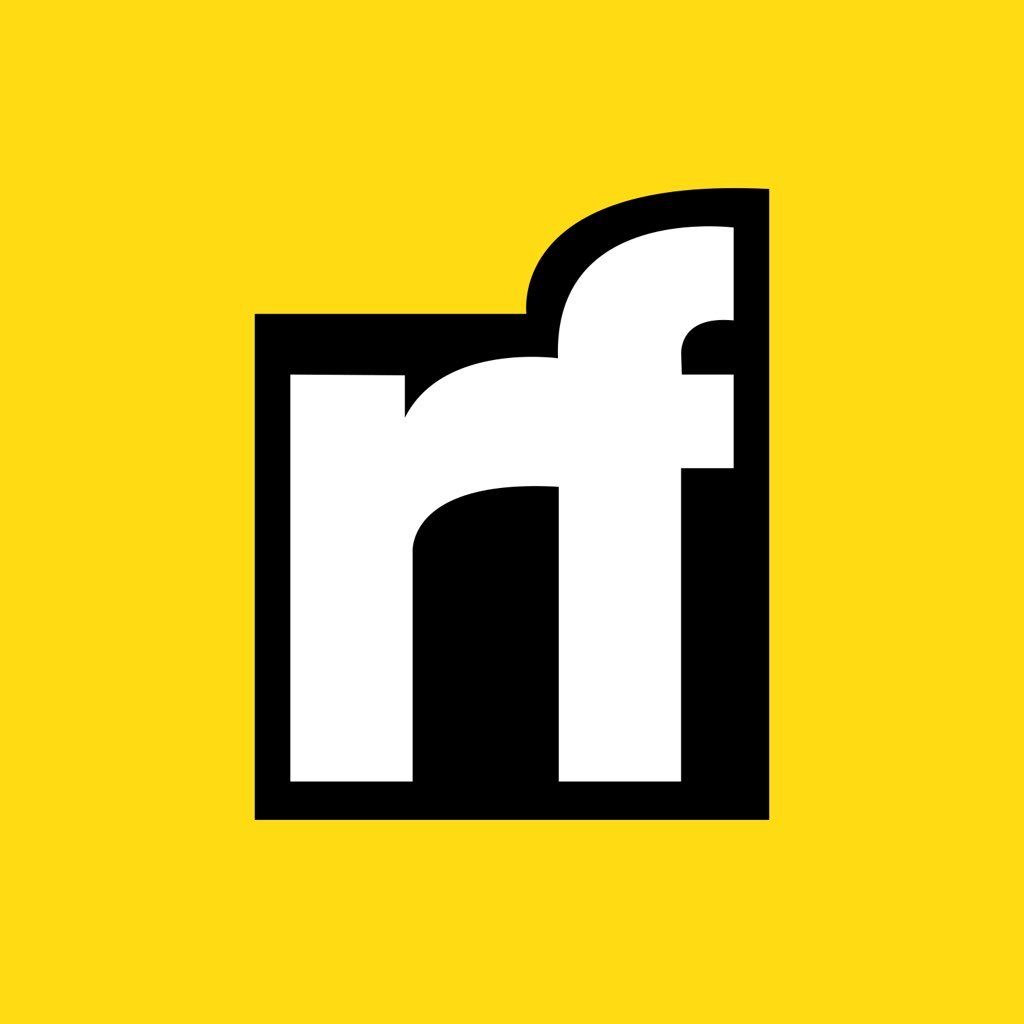
lance | Sciencx (2023-05-08T03:23:44+00:00) How To Name Your Code?. Retrieved from https://www.scien.cx/2023/05/08/how-to-name-your-code/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.