This content originally appeared on Level Up Coding - Medium and was authored by Anto Semeraro
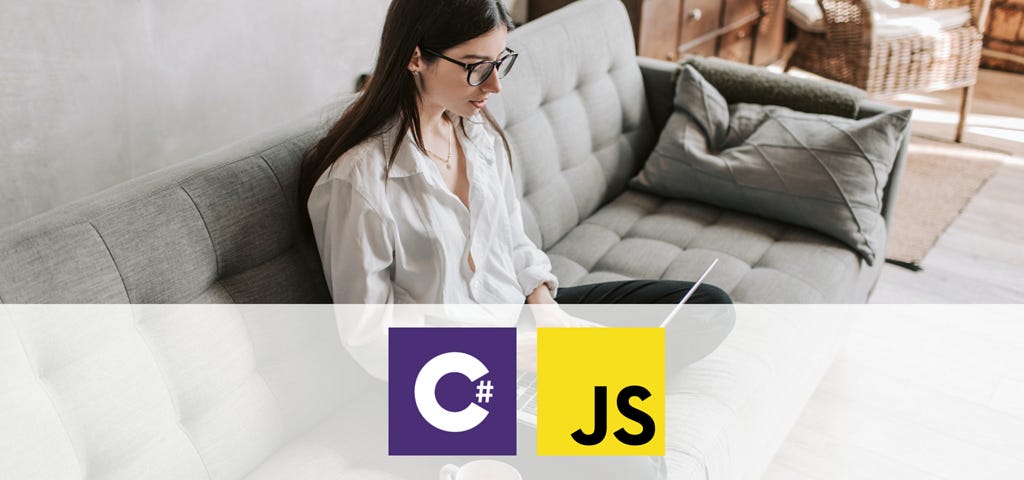
Software Development Principles
Unleash the power of techniques like a senior for managing errors in C# and JavaScript, and take your full-stack development skills to new heights.
Introduction
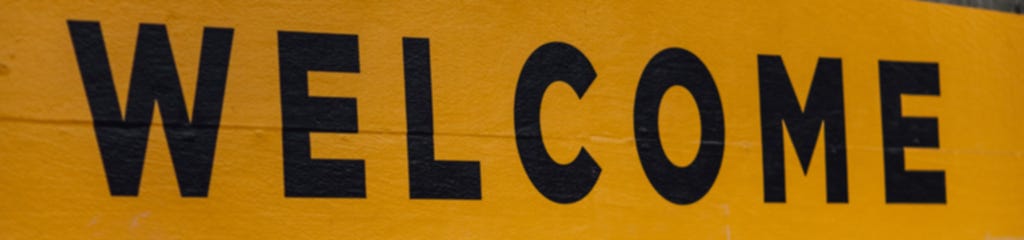
Embarking on the Error Management Adventure
Hello folks! I’m Anto, a passionate software engineer with almost 2 decades of experience in Microsoft platforms, including .NET Frameworks, .NET Core, SQL Server RDBMS, and frontend development, and today, I’ll share with you pro techniques to master error management in C# and JavaScript.
Error management is essential for creating efficient, stable, and user-friendly applications. Thus, let’s dive deep into this peculiar topic.
Overview: What We’ll Learn
- Understanding Error Management: Learn the fundamentals and importance of error management.
- Error Management in C#: Delve into C# error management, exploring syntax errors, logical errors, and exception handling and other techniques.
- Error Management in JavaScript: Discover error management in JavaScript, tackling syntax errors, logical errors, and advanced practices.
- Unified Strategies for Full-Stack Development: Apply best practices for both languages in full-stack development.
Embrace errors and bugs as opportunities to grow and hone your skills. Let’s get started!
Understanding Error Management
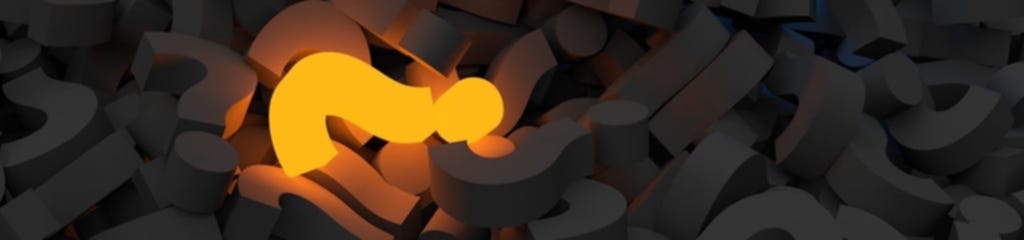
Why Error Management Matters
Before we delve into the nitty-gritty of error management techniques for C# and JavaScript, let’s pause to understand the importance of error management.
As a software engineer, you’ll inevitably encounter various errors and bugs in your code, and mastering error management is crucial to creating robust, efficient, and user-friendly applications, not to mention saving yourself from countless hours of debugging.
Categories of Errors
To effectively manage errors, we must first understand the different types of errors that can occur during the software development process:
- Syntax Errors: These errors occur due to incorrect syntax, such as missing semicolons, braces, or parentheses. Syntax errors are usually caught during the compilation stage in C# and during interpretation in JavaScript.
- Compile-time Errors: Typically found in statically-typed languages like C#, these errors are detected during the compilation stage. Examples include type mismatches, missing references, and undeclared variables.
- Logical Errors: These errors result from incorrect logic or algorithms used in the code. Logical errors don’t cause the program to crash, but they lead to incorrect output or behavior.
- Runtime Errors: These errors occur while the application is running. Examples include null reference exceptions, divide-by-zero errors, and out-of-range index errors.
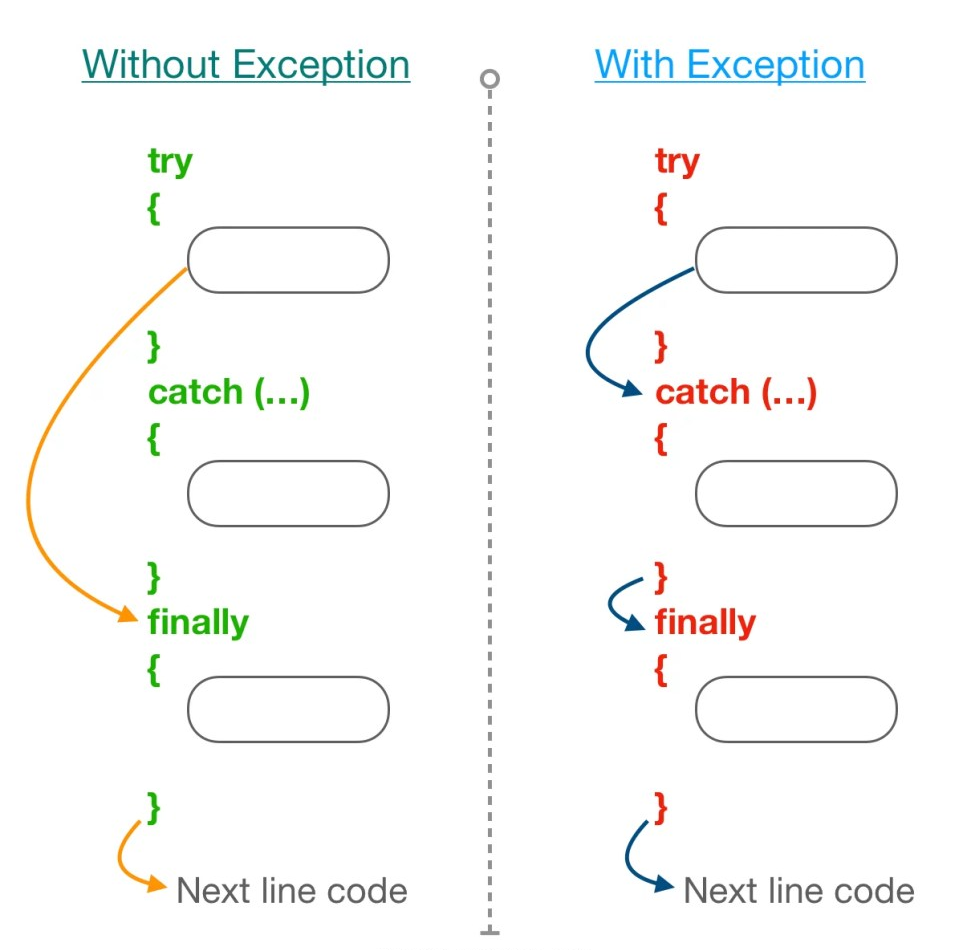
Exception Handling: A Key Error Management Technique
Exception handling is a powerful error management technique used to detect and resolve runtime errors, and so, if you implement exception handling mechanisms, you can gracefully handle errors, prevent application crashes, and provide users with informative error messages.
In the following sections, we’ll explore error management techniques and best practices for C# and JavaScript. We’ll study how to address syntax and compile-time errors, logical and runtime errors, and effectively use exception handling mechanisms.
So, let’s dive into the world of error management in C# and JavaScript, and learn how to harness the power of these pro techniques for full-stack development!
Error Management in C#: Techniques & Best Practices
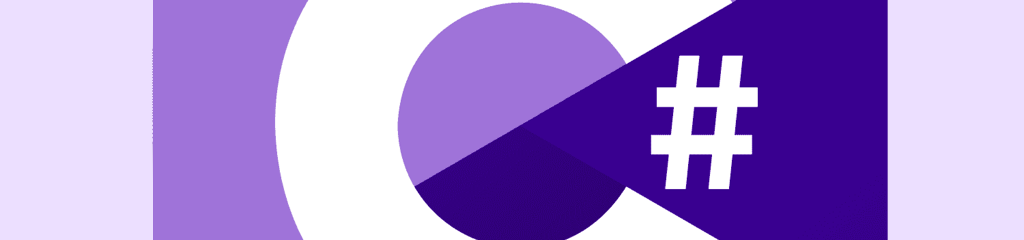
Syntax and Compile-Time Errors in C#
Oh right, let’s start by addressing syntax and compile-time errors in C#. These errors occur due to issues like missing semicolons, unmatched braces, or incorrect variable types, and thankfully, C#’s strong typing and Visual Studio’s IntelliSense make it easier to spot and fix these errors even before running an application.
Keep a close eye on your code as you write it, and leverage your IDE’s helpful tools to catch these errors before they cause problems.
Logical and Runtime Errors in C#
Logical and runtime errors in C# can be a bit more challenging to tackle, but with proper debugging techniques, you’ll overcome them in no time!
Example: Division by Zero
int x = 10;
int y = 0;
int result = x / y; // Throws a DivideByZeroException
In this example, we have one of the most common instnces: a division by zero error. To fix it, you could simply use a conditional statement to check if the denominator is zero before performing the division:
int x = 10;
int y = 0;
int result;
if (y != 0)
{
result = x / y;
}
else
{
Console.WriteLine("Cannot divide by zero.");
}
Remember to use a systematic approach while debugging logical errors, such as setting breakpoints, inspecting variable values, and carefully reviewing your code.
Exception Handling in C#
C# provides a robust exception handling mechanism with try, catch, and finally blocks, and using these constructs, you can gracefully handle runtime errors and maintain application stability.
Example: Catching and Handling Exceptions
int x = 10;
int y = 0;
int result;
try
{
result = x / y;
}
catch (DivideByZeroException ex)
{
Console.WriteLine($"Caught an exception: {ex.Message}");
result = -1; // Assigning a default value
}
finally
{
Console.WriteLine("Division operation completed.");
}
In this example, we use a try block to wrap the division operation, and a catch block to handle the DivideByZeroException. The finally block executes regardless of whether an exception occurs, ensuring that any necessary cleanup is performed.
Custom Exceptions
To provide more context for specific error scenarios, you can create custom exceptions by extending the base Exception class. Custom exceptions make it easier to catch and handle errors unique to your application.
Example: Creating a Custom Exception
public class InsufficientFundsException : Exception
{
public InsufficientFundsException() : base() { }
public InsufficientFundsException(string message) : base(message) { }
public InsufficientFundsException(string message, Exception inner) : base(message, inner) { }
}
To throw this custom exception, you might do something like this:
if (balance < withdrawalAmount)
{
throw new InsufficientFundsException("Insufficient funds for withdrawal.");
}
Exception Filters
C# 6 introduced exception filters, allowing you to catch exceptions based on specific conditions. Exception filters help reduce the need for complex nested catch blocks, and they maintain the original exception stack trace.
Example: Using an Exception Filter
try
{
// Some code that might throw an exception
}
catch (ArgumentException ex) when (ex.ParamName == "x")
{
Console.WriteLine("Exception occurred: Invalid argument 'x'.");
}
catch (ArgumentException ex) when (ex.ParamName == "y")
{
Console.WriteLine("Exception occurred: Invalid argument 'y'.");
}
AggregateException and Task Parallel Library
When working with parallel programming using the Task Parallel Library (TPL), multiple exceptions can occur simultaneously. The TPL wraps these exceptions in an AggregateException, allowing you to handle them in a single catch block.
Example: Handling Multiple Exceptions with AggregateException
try
{
Task.WaitAll(task1, task2, task3);
}
catch (AggregateException ae)
{
ae.Handle((ex) =>
{
if (ex is InvalidOperationException)
{
Console.WriteLine("Caught an InvalidOperationException.");
return true;
}
if (ex is ArgumentNullException)
{
Console.WriteLine("Caught an ArgumentNullException.");
return true;
}
return false; // Any other exception will not be handled here
});
}
Global Error Handling
Centralizing your error handling logic can simplify your code and improve maintainability. In C#, you can achieve this with the AppDomain.CurrentDomain.UnhandledException event for console applications or Application.ThreadException event for Windows Forms applications.
Example: Global Error Handling in a Console Application
public static void Main()
{
AppDomain.CurrentDomain.UnhandledException += UnhandledExceptionHandler;
// Your application logic here
}
private static void UnhandledExceptionHandler(object sender, UnhandledExceptionEventArgs e)
{
Exception ex = (Exception)e.ExceptionObject;
Console.WriteLine($"Caught a global exception: {ex.Message}");
}
With the implementation of these advanced error management techniques in your C# applications, you’ll gain better control over the error handling process and enhance your application’s reliability.
Stay tuned for our next section, where we’ll explore error management techniques in JavaScript!
Error Management in JavaScript: Techniques & Best Practices
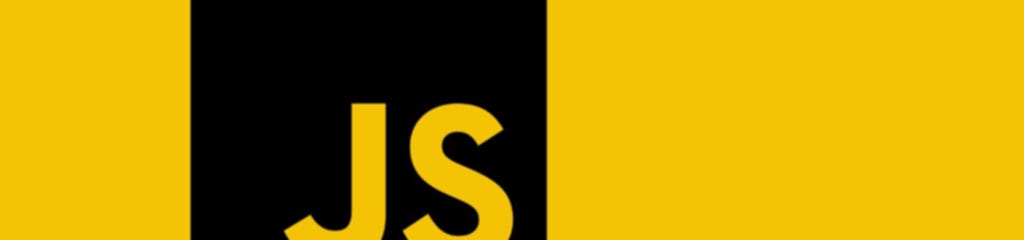
As a passionate full-stack developer, I’m excited to share my knowledge on managing errors in JavaScript as well, and, as you could guess, error management is vital for maintaining a seamless user experience and enhancing your application’s reliability.
Thus, let’s dive into JavaScript error management best practices!
Syntax and Compile-Time Errors
Syntax errors occur when the code structure does not adhere to JavaScript’s rules. Compile-time errors, on the other hand, arise from issues during the code compilation process. To avoid these errors, follow JavaScript syntax guidelines and employ linters like ESLint to validate your code.
Example: A syntax error in JavaScript
const user = {
name: 'John',
age: 30,
// Missing comma here
email: 'john@example.com'
};
Logical and Runtime Errors
Logical errors result from incorrect code logic, while runtime errors happen when a valid code encounters an issue during execution. Debugging tools like browser DevTools or console.log() can help identify and resolve these errors.
Example: A logical error in JavaScript
function calculateArea(width, height) {
// The logical error is using a plus operator instead of multiplication
return width + height;
}
console.log(calculateArea(10, 5)); // Output: 15 (should be 50)
Exception Handling
Likewise C# and many other programming languages, JavaScript also provides the try, catch, and finally statements for handling exceptions. They allow you to execute code that may throw exceptions and define how to handle errors gracefully.
Example: Basic exception handling in JavaScript
try {
// Code that might throw an exception
const result = riskyOperation();
} catch (error) {
console.error('An error occurred:', error.message);
} finally {
// This block will run regardless of success or failure
console.log('Risky operation completed.');
}
But, to level up your JavaScript error management skills, consider implementing these following pro techniques.
Custom Error Classes
Create custom error classes by extending the base Error class to handle application-specific errors.
Example: Customizing validation error in JavaScript
class ValidationError extends Error {
constructor(message) {
super(message);
this.name = 'ValidationError';
}
}
// Usage
if (!isValid(user)) {
throw new ValidationError('Invalid user data.');
}
Promise Error Handling
When working with promises, use the catch() method to handle errors. Alternatively, employ async and await with try-catch blocks to simplify error management in asynchronous code.
Example: Catching an error in an async function and with catch() in JavaScript
// With catch()
fetchData()
.then((data) => processData(data))
.catch((error) => console.error('An error occurred:', error.message));
// With async/await
async function fetchDataAndProcess() {
try {
const data = await fetchData();
const processedData = await processData(data);
} catch (error) {
console.error('An error occurred:', error.message);
}
}
Global Error Handlers
Use global error handlers like window.onerror and window.addEventListener('unhandledrejection', ...) to catch unhandled errors and promise rejections.
Example: Global error handling in JavaScript
window.onerror = function (message, source, lineno, colno, error) {
console.error('A global error occurred:', error.message);
};
window.addEventListener('unhandledrejection', function (event) {
console.error('A promise rejection occurred:', event.reason.message);
});
Remember, errors are an inevitable part of the software development journey, and handling them effectively and gracefully in JavaScript, you’ll not only deliver a robust and user-friendly application but also gain the confidence to tackle even more challenging full-stack projects.
In the next section, we’ll explore unified strategies for full-stack development, integrating the C# and JavaScript error management techniques we’ve discussed so far. This will empower you to leverage your newfound skills across both the backend and frontend aspects of your applications.
Unified Strategies for Full-Stack Development
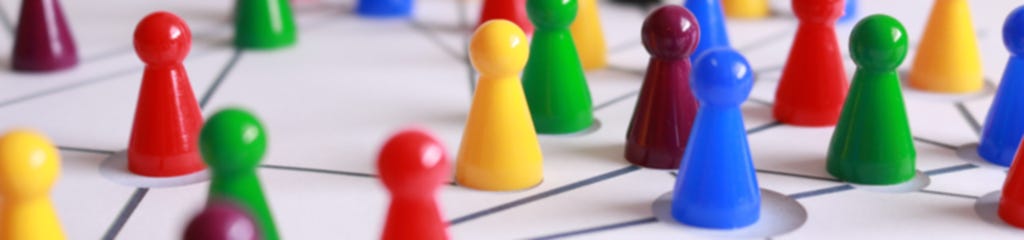
Throughout this article, we’ve delved deep into the realm of error management in both C# and JavaScript, exploring a myriad of techniques and best practices for handling errors like a pro.
Now it’s time to bring it all together with unified strategies for full-stack development, and with these strategies, you’ll enhance your full-stack expertise, ensuring your applications remain robust, performant, and user-friendly.
Embracing Cross-Languages Synergies
It’s essential to understand that, as a full-stack developer, you’re in a unique position to find synergies between the languages you work with daily. As you become more comfortable with error management in C# and JavaScript, you’ll start to notice patterns and parallels between these two languages that can be applied in a complementary manner.
For example, the concepts of try-catch blocks, custom error types, and logging best practices are applicable in both C# and JavaScript. With these practices across your entire application, you can create a unified error management strategy that simplifies debugging and maintenance, ultimately improving overall application quality.
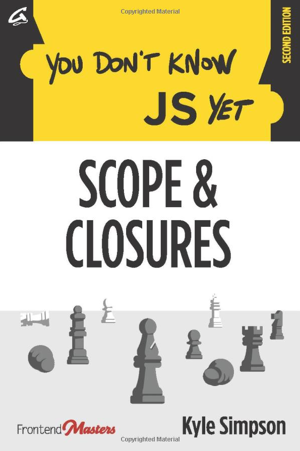
📣For your information: Buying a book through links in this article gives me a small commission at no additional cost to you. This helps me continue producing content you enjoy. Thank you for your support!
Create a Centralized Logging System
A centralized logging system is an invaluable asset when managing errors across both the frontend and backend of your applications, and collecting all log entries in a single, accessible location, you can efficiently analyze and correlate issues that might span across multiple layers of your application stack.
Implement a logging system that captures essential information like timestamps, error types, stack traces, and any additional context that might be helpful for debugging. Thus, consider utilizing log aggregation tools like Elasticsearch and Kibana or third-party logging services like Loggly or Sumo Logic to manage and analyze your log data effectively.
Centralizing JavaScript and C# Logging with a Logger Aggregator API
One essential aspect of unified error management across full-stack development is effective logging, so let’s explore how to centralize logs from both JavaScript and C# by using a Logger Aggregator API implemented in C#. This API will save logs in ElasticSearch, providing an efficient, centralized, and scalable solution for monitoring and analyzing error data.
In our full-stack application, the frontend JavaScript code will communicate with the backend C# Logger Aggregator API to send error logs, which will then be processed and stored in ElasticSearch.
Example Frontend: Sending Logs from JavaScript
To send logs from JavaScript, you can use the fetch function or any preferred AJAX library to make HTTP requests to the Logger Aggregator API.
function sendLogToAPI(level, message) {
fetch('/api/logger', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ level, message }),
})
.catch(error => console.error('Error sending log to API:', error));
}
// Usage
sendLogToAPI('error', 'An error occurred in the frontend.');
Example Backend: Implementing the Logger Aggregator API in C#
The Logger Aggregator API receives logs from the frontend and stores them in ElasticSearch using a C# logger. This API can be implemented as an ASP.NET Core Web API with a simple POST action to accept logs.
[Route("api/[controller]")]
[ApiController]
public class LoggerController : ControllerBase
{
private readonly ILogger<LoggerController> _logger;
public LoggerController(ILogger<LoggerController> logger)
{
_logger = logger;
}
[HttpPost]
public IActionResult Post(LogEntry logEntry)
{
_logger.Log(logEntry.Level, logEntry.Message);
return Ok();
}
}
Leveraging ElasticSearch for Powerful Log Analysis
Storing logs in ElasticSearch allows you to take advantage of its powerful search and analytics capabilities. To integrate ElasticSearch into your .NET solution, check out the article ElasticSearch in .NET for a comprehensive guide to master the art of this integration.
ElasticSearch in .NET: A Guide to Building Fast and Scalable Search Experiences
Uplifting Your Full-Stack Development Game
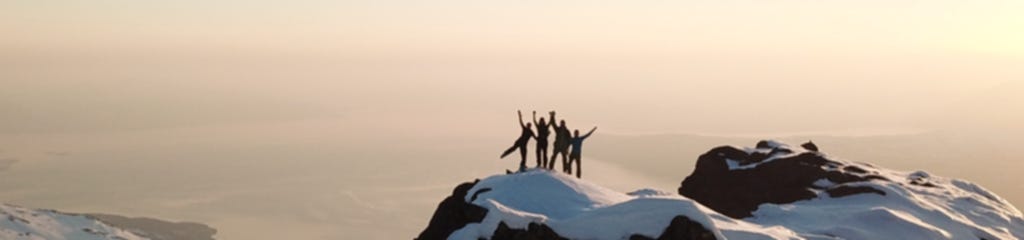
As we come to the end of our exploration of mastering error management in C# and JavaScript, it’s clear that adopting these pro techniques is essential for enhancing your full-stack development prowess.
The strategies we’ve discussed, such as understanding different error types, utilizing exception handling mechanisms, and applying unified practices across both languages, can immensely improve the reliability and maintainability of your software solutions.
Remember: if you focus on best practices, nurture cross-language synergies, and embrace the spirit of continuous improvement, you can create a powerful and unified full-stack development experience.
Let’s seize this opportunity to learn from our mistakes, refine our skills, and contribute to building better software solutions for a brighter, more connected future.
Bibliography
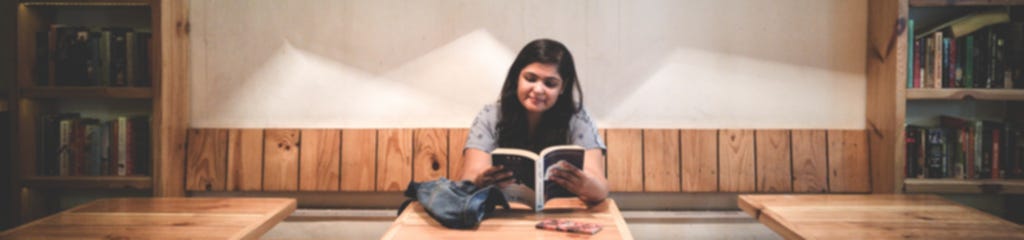
- “Effective C#: 50 Specific Ways to Improve Your C#”, Bill Wagner, 2016, Addison-Wesley Professional.
- “JavaScript: The Definitive Guide”, David Flanagan, 2020, O’Reilly Media.
- “You Don’t Know JS: Scope & Closures”, Kyle Simpson, 2014, O’Reilly Media.
- “C# in Depth”, Jon Skeet, 2019, Manning Publications.
- “JavaScript Patterns: Build Better Applications with Coding and Design Patterns”, Stoyan Stefanov, 2010, O’Reilly Media.
Mastering Error Management in C# & JavaScript: Pro Techniques for Full-Stack Development was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anto Semeraro
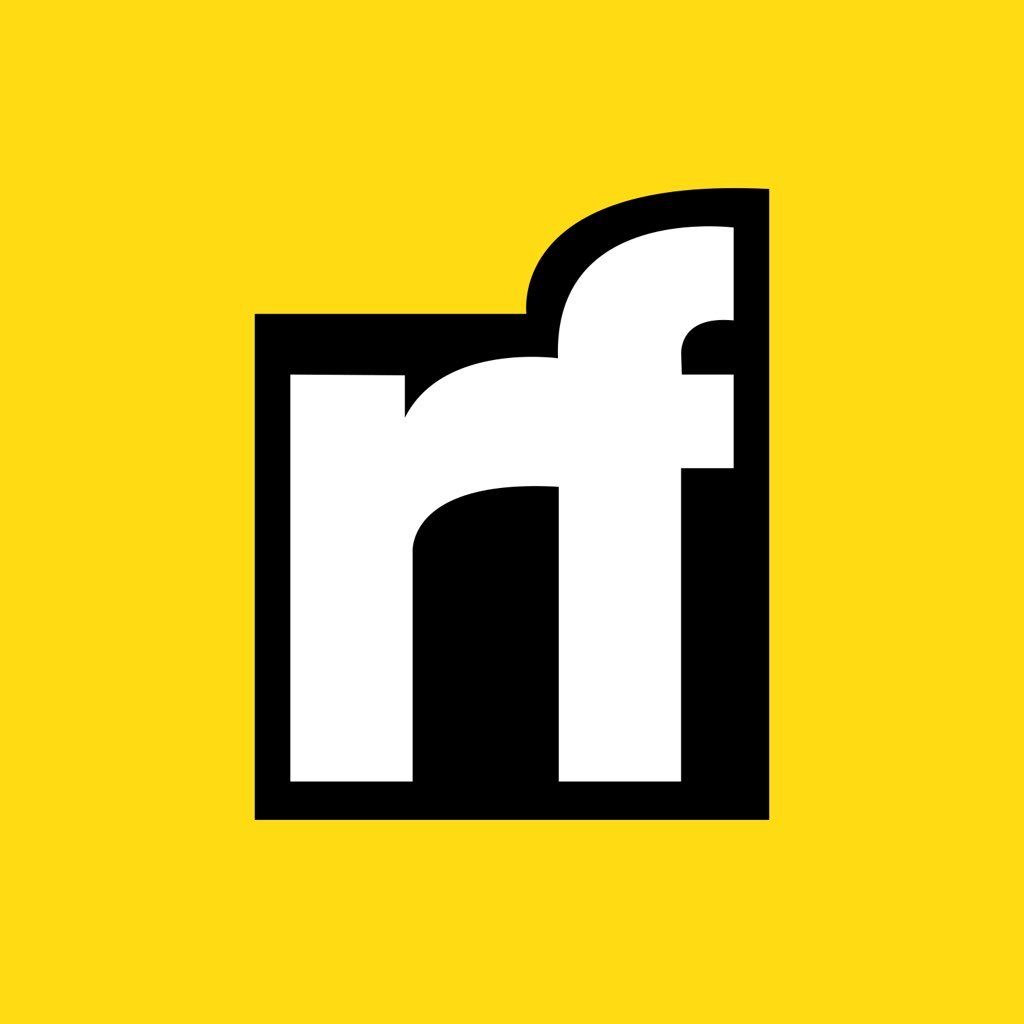
Anto Semeraro | Sciencx (2023-05-09T02:49:27+00:00) Mastering Error Management in C# & JavaScript: Pro Techniques for Full-Stack Development. Retrieved from https://www.scien.cx/2023/05/09/mastering-error-management-in-c-javascript-pro-techniques-for-full-stack-development/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.