This content originally appeared on Level Up Coding - Medium and was authored by Sonika | Javascript Hungry | Working at Walmart
Topics
- Modal for your website just using HTML Elements
- Simpler way to control CSS Transform
- New viewport units that adapt to mobile user interface
- Deep Cloning Objects
- Accesibility :focus-visible Pseudo Class
- Transform Stream
- Import Maps
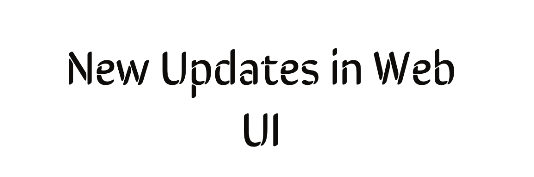
Dialog using HTML Elements
- The <dialog> element makes it easy to create popup dialogs and modals on a web page.
<dialog open>
<p>Greetings, one and all!</p>
<form method="dialog">
<button>OK</button>
</form>
</dialog>
Example, https://codepen.io/web-dot-dev/pen/BaOBLNy
CSS Transform
- The transform CSS property lets you rotate, scale, skew, or translate an element.
Earlier,

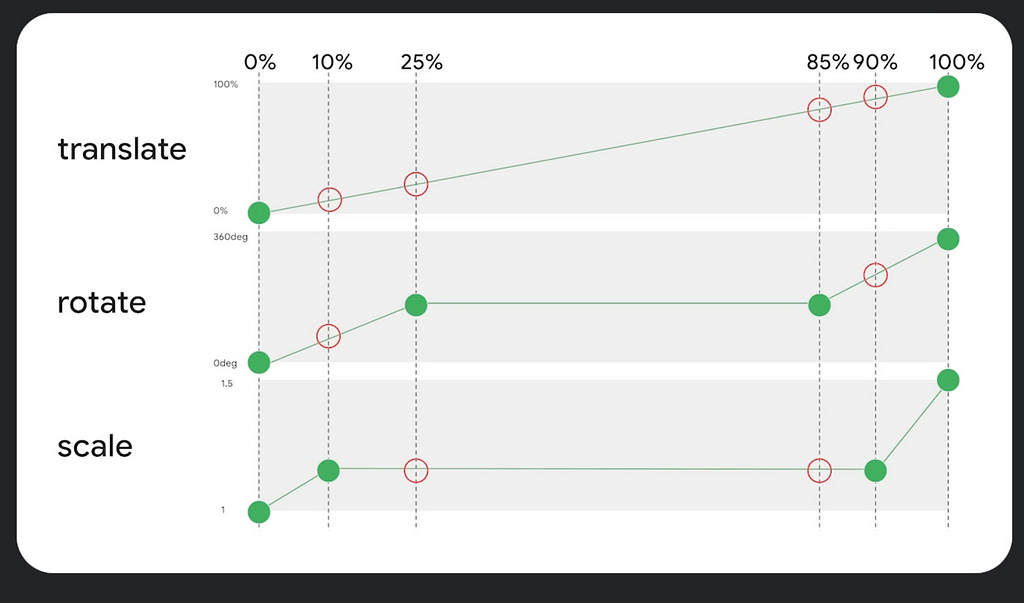
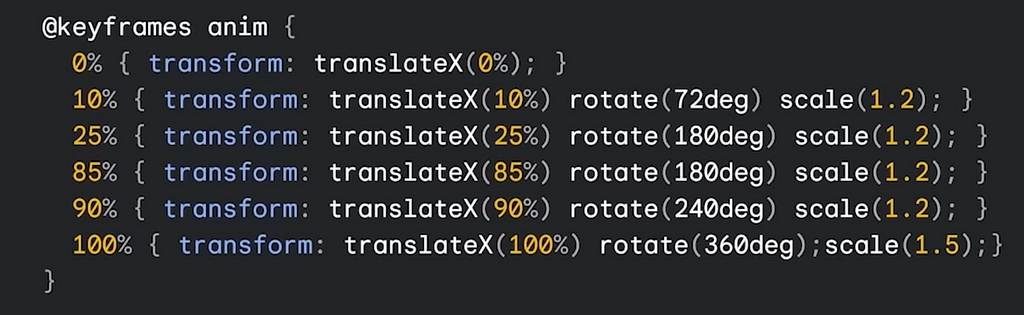
Now,
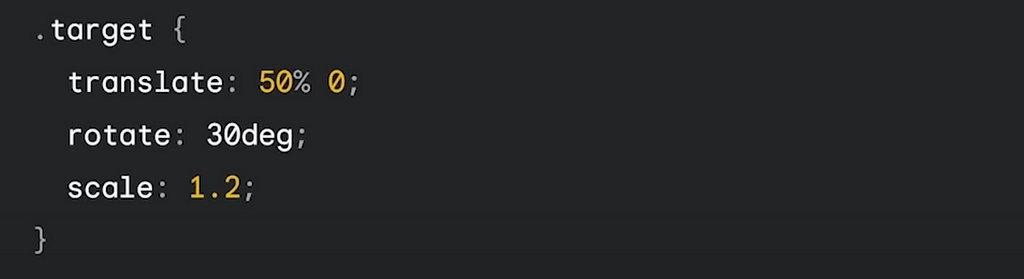
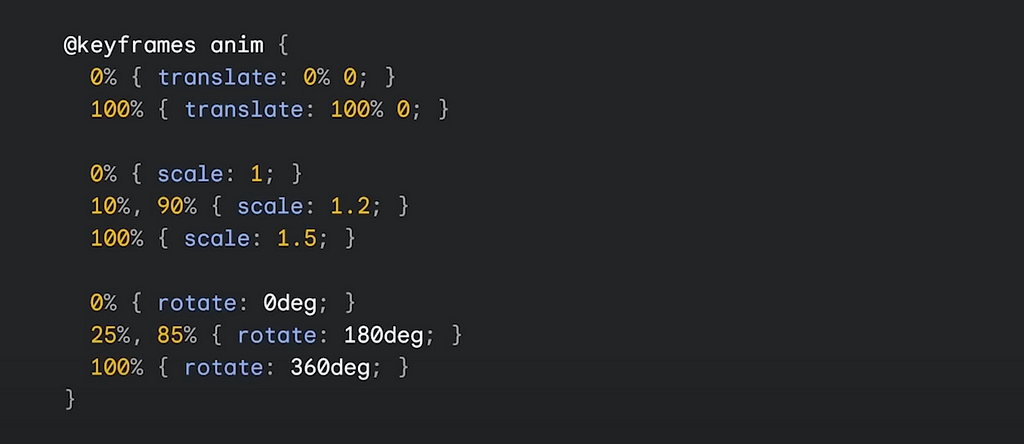
New ViewPort: When designing for mobile
- small view hright: svh
- large view height: lvh
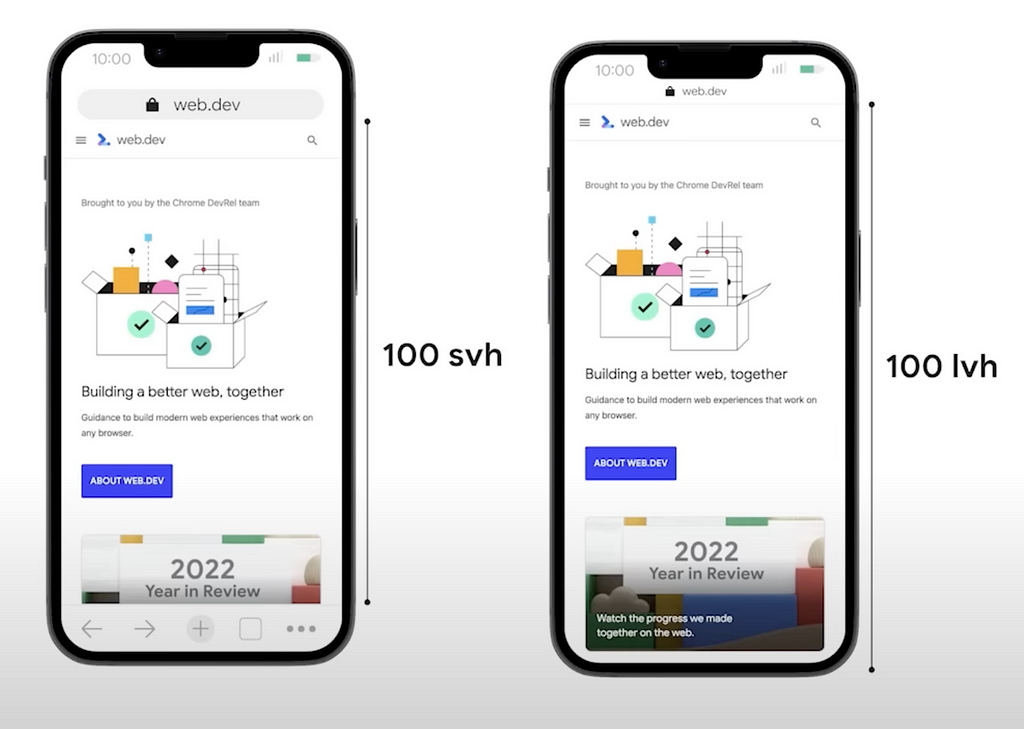
- min and max units as well
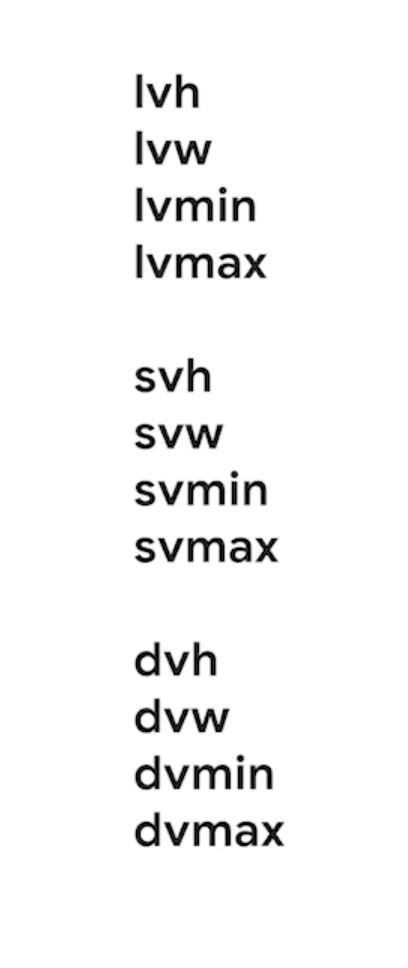
Deep Cloning Objects:
- Javascript objects are usually stored in memory and can only be copied by reference, meaning, that a variable does not store an object in itself, but rather an identifier that represents the memory location of the object. Therefore, objects cannot be copied in the same way as primitives.
- On the other hand, A deep copy creates a new object with its own set of data, separate from the original object. If the original object is modified, the copy will not be affected.
- Shallow Copies: A shallow copy is a copy of an object that only copies the reference to the object, not the actual data. If the original object is modified, the copy will also be modified.
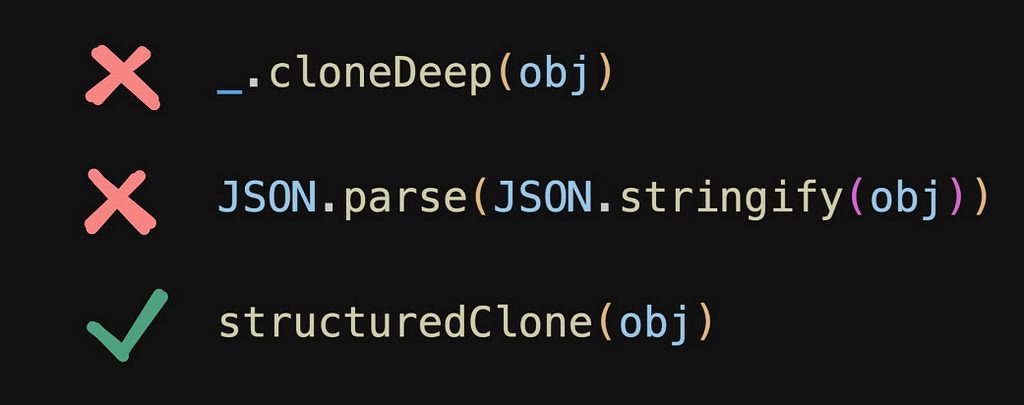
Now, we can do deep clone using structuredClone
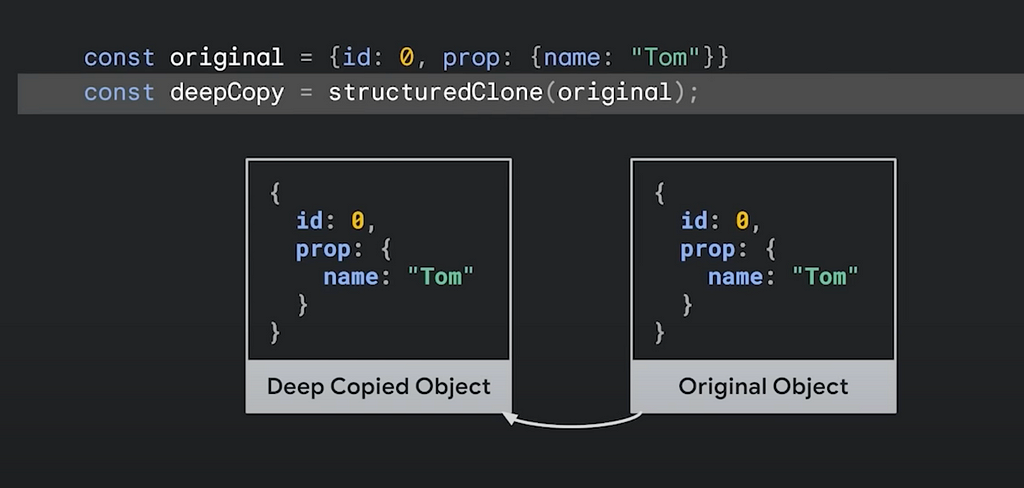
const mushrooms1 = {
amanita: ["muscaria", "virosa"],
};
const mushrooms2 = structuredClone(mushrooms1);
mushrooms2.amanita.push("pantherina");
mushrooms1.amanita.pop();
console.log(mushrooms2.amanita); // ["muscaria", "virosa", "pantherina"]
console.log(mushrooms1.amanita); // ["muscaria"]
structuredClone can not only do the above, but additionally:
- Clone infinitely nested objects and arrays
- Clone circular references
- Clone a wide variety of JavaScript types, such as Date, Set, Map, Error, RegExp, ArrayBuffer, Blob, File, ImageData, and many more
- Transfer any transferable objects
const kitchenSink = {
set: new Set([1, 3, 3]),
map: new Map([[1, 2]]),
regex: /foo/,
deep: { array: [ new File(someBlobData, 'file.txt') ] },
error: new Error('Hello!')
}
kitchenSink.circular = kitchenSink
// ✅ All good, fully and deeply copied!
const clonedSink = structuredClone(kitchenSink)
Why not just object spread ?
It is important to note we are talking about a deep copy. If you just need to do a shallow copy, aka a copy that does not copy nested objects or arrays, then we can just do an object spread:
const simpleEvent = {
title: "Builder.io Conf",
}
// ✅ no problem, there are no nested objects or arrays
const shallowCopy = {...calendarEvent}
const shallowCopy = Object.assign({}, simpleEvent)
const shallowCopy = Object.create(simpleEvent)
But as soon as we have nested items, we run into trouble:
const calendarEvent = {
title: "Builder.io Conf",
date: new Date(123),
attendees: ["Steve"]
}
const shallowCopy = {...calendarEvent}
// 🚩 oops - we just added "Bob" to both the copy *and* the original event
shallowCopy.attendees.push("Bob")
// 🚩 oops - we just updated the date for the copy *and* original event
shallowCopy.date.setTime(456)
What can structuredClone not clone
- Functions cannot be cloned
// 🚩 Error!
structuredClone({ fn: () => { } })
- DOM nodes
// 🚩 Error!
structuredClone({ el: document.body })
Accessibility: CSS Pseudo Class →Focus Visible
- The :focus-visible pseudo-class applies while an element matches the :focus pseudo-class
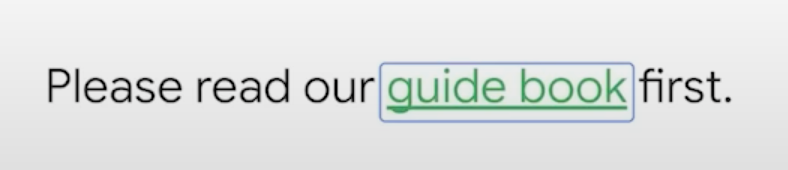
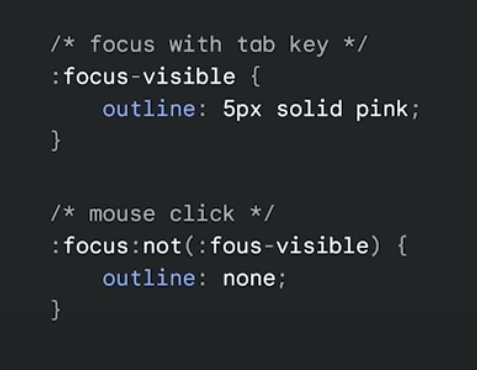
Transform Stream
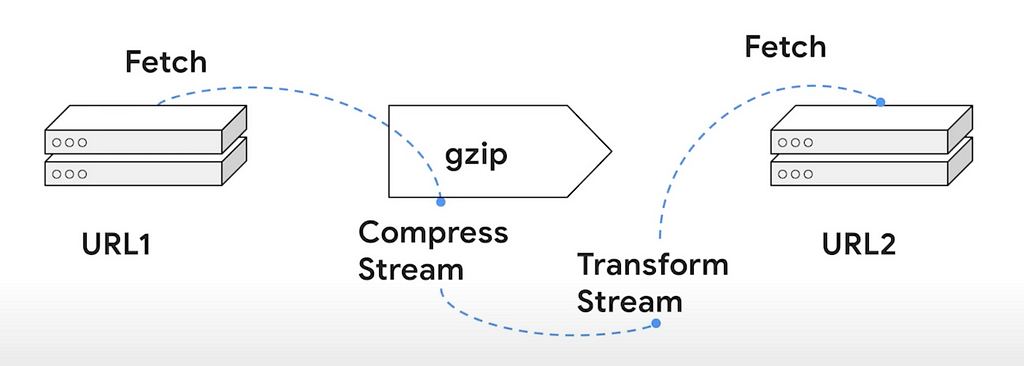
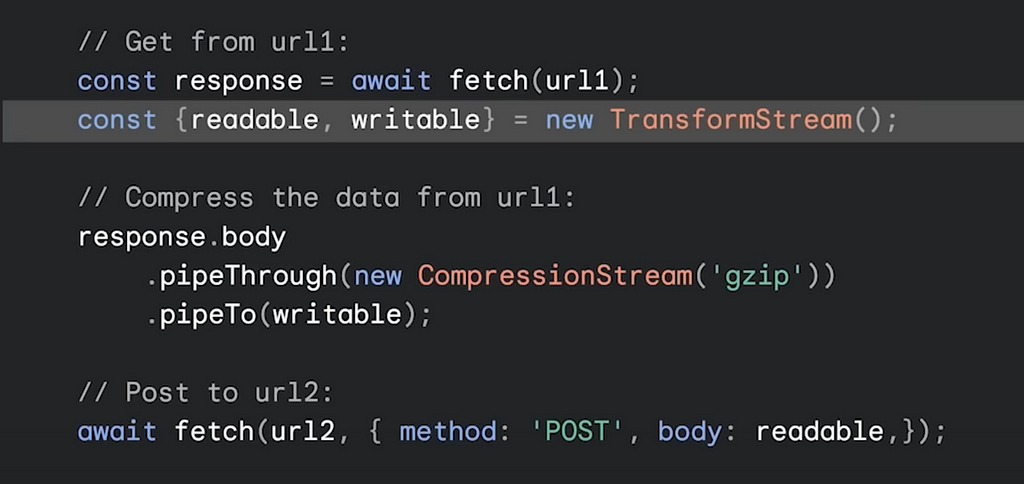
Import Maps / Module: ES6 Modules
<script type="importmap">
// JSON object defining import
</script>
<script type="module">
// JSON object defining import
</script>
- The importmap value of the type attribute of the <script> element indicates that the body of the element contains an import map.
- An import map is a JSON object that allows developers to control how the browser resolves module specifiers when importing JavaScript modules.

How Import Maps Work
<script type="importmap">
{
"imports": {
"dayjs": "https://cdn.skypack.dev/dayjs@1.10.7",
}
}
</script>
<script type="module">
import dayjs from 'dayjs';
console.log(dayjs('2019-01-25').format('YYYY-MM-DDTHH:mm:ssZ[Z]'));
</script>
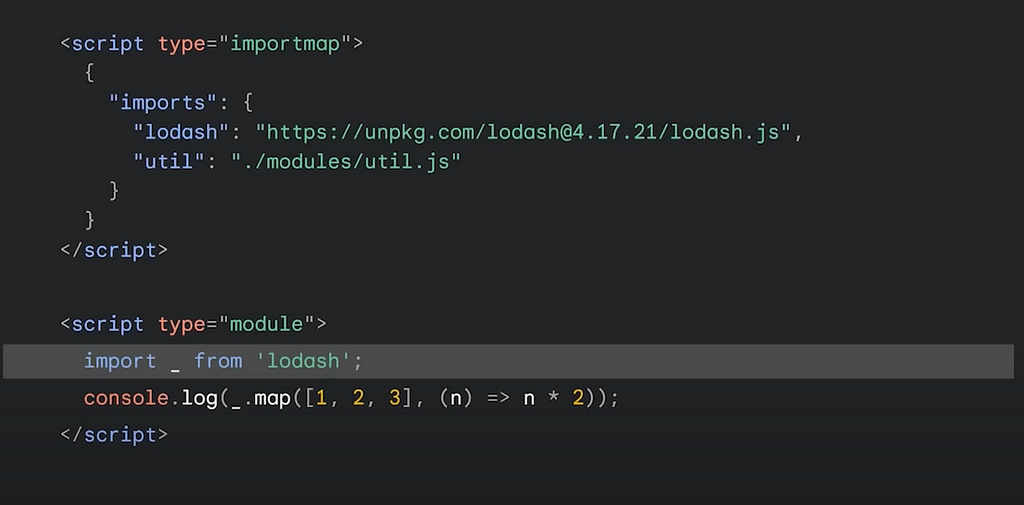
Define scopes,
<script type="importmap">
{
"imports": {
"lodash/": "https://unpkg.com/lodash-es@4.17.21/"
},
"scopes": {
"/static/js": {
"lodash/": "https://unpkg.com/lodash-es@3.10.1/"
}
}
}
</script>
Script type “module”,
<script type="module">
import { cloneDeep } from 'lodash';
const objects = [{ a: 1 }, { b: 2 }];
const deep = cloneDeep(objects);
console.log(deep[0] === objects[0]);
</script>
References,
- https://www.builder.io/blog/structured-clone
- https://matiashernandez.dev/blog/post/deep-cloning-in-javascript-the-modern-way.-use-%60structuredclone%60
- https://developer.mozilla.org/en-US/docs/Web/API/TransformStream/TransformStream
- https://io.google/2023/program/cafbe05a-c19e-4fe5-9e25-61c5c0c2f6cf/
- https://developer.mozilla.org/en-US/docs/Web/HTML/Element/script/type/importmap
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
New Updates in Web UI was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Sonika | Javascript Hungry | Working at Walmart
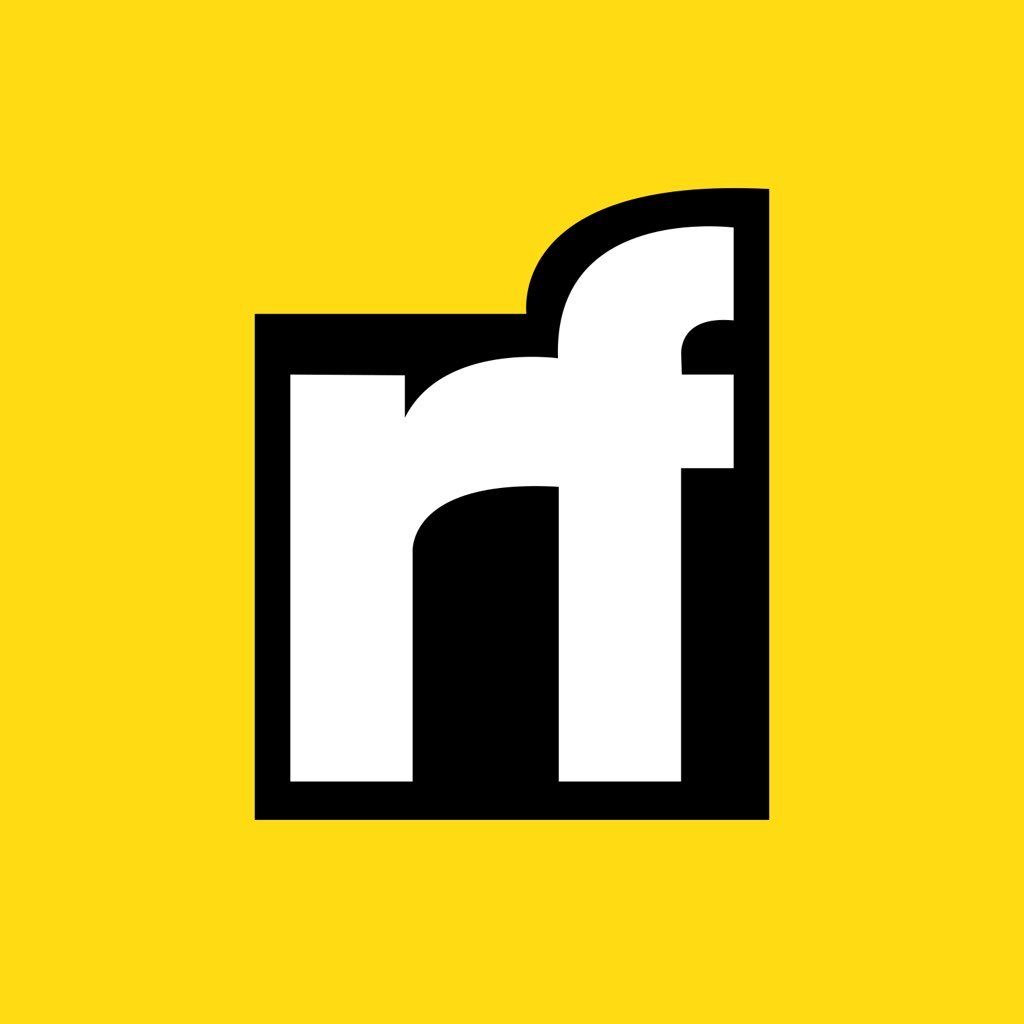
Sonika | Javascript Hungry | Working at Walmart | Sciencx (2023-05-12T17:19:30+00:00) New Updates in Web UI. Retrieved from https://www.scien.cx/2023/05/12/new-updates-in-web-ui/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.