This content originally appeared on DEV Community and was authored by Artur Kędzior
I like to follow "Modern Microsoft" conventions and styles for C# and dotnet; these are the conventions typically found in modern Microsoft GitHub repos like .NET, EF, etc. They are generally also the defaults within Visual Studio.
I go for standard Microsoft conventions as they would be most popular in the community and would generally be more familiar to new developers joining the team.
1. Boolean Evaluations
😀 DO prefer expression operator short-hand syntax.
var success = true;
if (success)
{
// do if true
}
if (!success)
{
// do if not true
}
Why: Consistent with Microsoft’s .NET Framework and makes code simpler, more concise, and easier to read.
😡 DO NOT use long form operator syntax:
var success = true;
if (success == true)
{
// do if true
}
if (success == false)
{
// do if not true
}
2. Abbreviation Casing
😀 DO prefer PascalCase for a abbreviations of any length found within member names:
public DateTime CreatedUtc { get; set; }
public string SqlConnection { get; set; }
😡 DO NOT UPPERCASE abbreviations:
public DateTime CreatedUTC { get; set; }
public string SQLConnection { get; set; }
Why: Consistent with Microsoft’s .NET Framework and easier to read.
3. Conditional and Loop Brackets
😀 DO always include brackets around single-line conditional and loop statements:
if (true)
{
DoSomething():
}
😡 DO NOT omit brackets:
if (true)
DoSomething();
Why: It makes the scope of the statements clearer and avoids future issues if those statements are expanded.
4. Var Keyword
😀 DO always prefer using the var keyword instead of the
explicit type:
var option = new CookieOptions();
😡 DO NOT use the explicit type:
CookieOptions option = new CookieOptions();
Why: It simplifies the code and isn’t any less understandable.
5. LINQ Single vs Where
😀 DO always prefer using the Where function instead of the Single :
var order = await DbContext.Orders
.Where(x => x.Id == id)
.Where(x => x.Status == OrderStatus.Created)
.Where(x => x.Customer.Id == customerId)
.SingleAsync(cancellationToken);
😡 DO NOT use the explicit type:
var order = await DbContext.Orders
.SingleAsync(x => x.Id == id && x.Status == OrderStatus.Created && x.Status == OrderStatus.Created, cancellationToken);
Why: It improves readability of the code.
This content originally appeared on DEV Community and was authored by Artur Kędzior
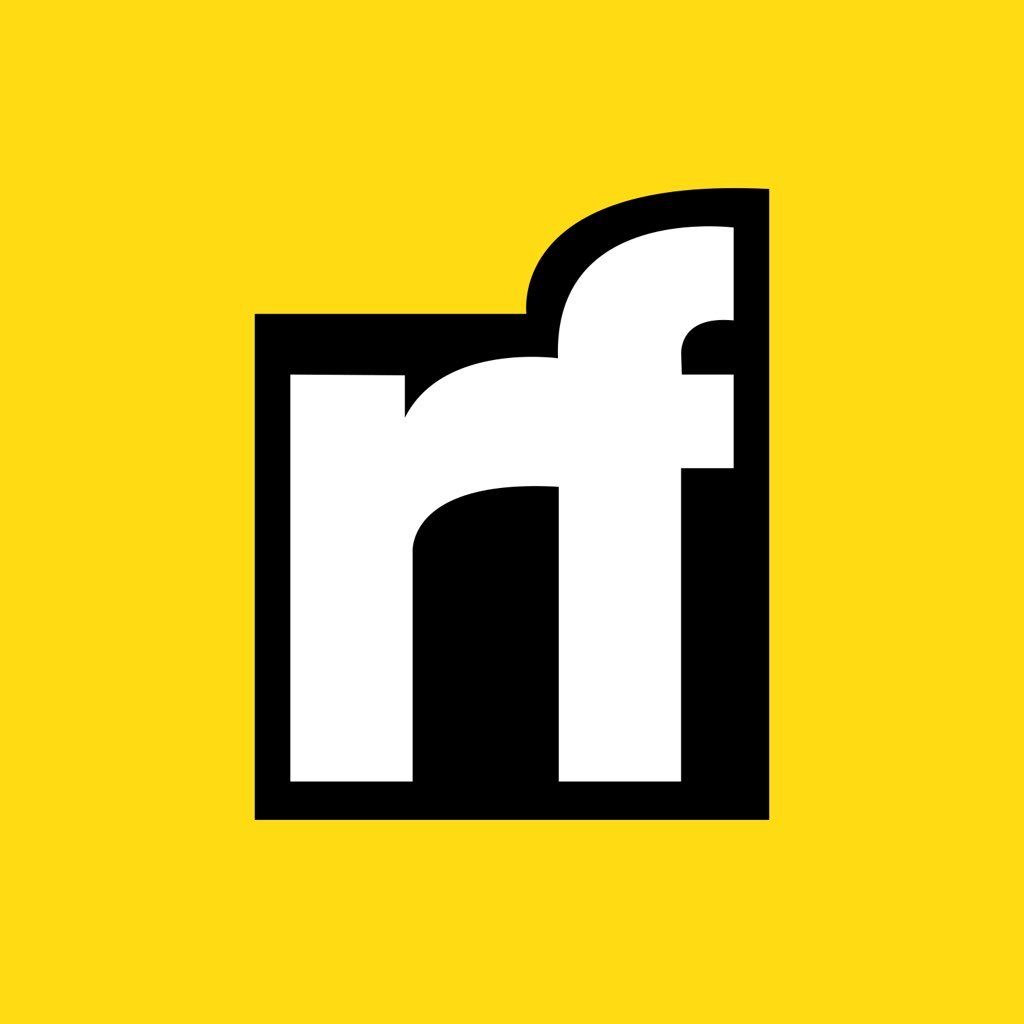
Artur Kędzior | Sciencx (2023-05-13T14:17:24+00:00) My C# Code Conventions and Style Guide. Retrieved from https://www.scien.cx/2023/05/13/my-c-code-conventions-and-style-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.