This content originally appeared on DEV Community and was authored by nozumaz
So I'm working on a project using PokeAPI (https://pokeapi.co/), and still trying to wrap my head around fetching json objects. Let's try to use this blog as a way to practice and document some of the data you can pull with pokeapi.
For this, I will be using mainly Javascript and HTML, with maybe some CSS for styling.
To start off, here is a basic Javascript fetchPokemon() method to get the pokemon data and create a pokemon object with the name, id, and image for the first pokemon, bulbasaur, which I arrived at by following the Build a Pokedex with Vanilla HTML, CSS, and Javascript Youtube video by James Q Quick.
const fetchPokemon = () => {
const url = `https://pokeapi.co/api/v2/pokemon/1`;
fetch(url)
.then((res) => {
return res.json();
})
.then((data) => {
console.log(data);
const pokemon = {
name: data.name,
id: data.id,
image: data.sprites['front_default'],
type: data.types.map((type) => type.type.name).join (', ')
};
});
}
The above Javascript code generates the following JSON data in the browser console window (which you can get from right-clicking and selecting 'Inspect', or F12 to open DevTools in the browser):
Now, trying to pull the pokemon by ID number, you can use the following Javascript code (using ID 2 for Ivysaur so we can tell that the output is different:
let pokemonID = 2;
const fetchPokemon = (id) => {
const url = `https://pokeapi.co/api/v2/pokemon/${id}`;
fetch(url)
.then((res) => {
return res.json();
})
.then((data) => {
console.log(data);
const pokemon = {
name: data.name,
id: data.id,
image: data.sprites['front_default'],
type: data.types.map((type) => type.type.name).join (', ')
};
});
}
fetchPokemon(pokemonID);
This generates the following JSON output, which we can see has the information for Ivysaur:
Next, I want to make an array of six pokemon, so I made the array:
let pokemonIDs = [1,2,3,4,5,6];
and added a for loop to iterate through the array, like this:
let pokemonIDs = [1,2,3,4,5,6];
const fetchPokemon = (ids) => {
for (let i = 0; i < 6; i++) {
const url = `https://pokeapi.co/api/v2/pokemon/${ids[i]}`;
fetch(url)
.then((res) => {
return res.json();
})
.then((data) => {
console.log(data);
const pokemon = {
name: data.name,
id: data.id,
image: data.sprites['front_default'],
type: data.types.map((type) => type.type.name).join (', ')
};
});
}
}
fetchPokemon(pokemonIDs);
which gives the JSON output for the first six pokemon:
Continuing to follow the video, we use a Promise object to fetch all of the pokemon at the same time, to make it easier to deal with multiple asynchronous calls.
let pokemonIDs = [1,2,3,4,5,6];
const fetchPokemon = (ids) => {
const promises = [];
for (let i = 0; i < 6; i++) {
const url = `https://pokeapi.co/api/v2/pokemon/${ids[i]}`;
promises.push(fetch(url).then((res) => res.json()));
}
Promise.all(promises).then((results) => {
const pokemon = results.map((data) => ({
name: data.name,
id: data.id,
image: data.sprites['front_default'],
type: data.types.map((type) => type.type.name).join(', ')
}));
console.log(pokemon);
});
};
fetchPokemon(pokemonIDs);
This gives us a JSON output with all six pokemon data objects conveniently together:
Next we are adding this displayPokemon function to create HTML elements to show the first 6 pokemon:
const displayPokemon = (pokemon) => {
console.log(pokemon);
const pokemonHTMLString = pokemon
.map(
(pokemonDisplay) =>
`
<div class="card">
<img class="card-image" src="${pokemonDisplay.image}"/>
<h2 class="card-title">${pokemonDisplay.id}. ${pokemonDisplay.name}</h2>
<p class="card-subtitle">Type: ${pokemonDisplay.type}</p>
</div>
`
).join('');
pokedex.innerHTML = pokemonHTMLString;
}
Here is the very basic HTML so far:
<!DOCTYPE html>
<html lang = "en">
<head>
<link rel="stylesheet" type="text/css" href="style.css"/>
<title></title>
</head>
<body>
<h1>test</h1>
<script type="text/javascript" src = "index.js"></script>
<p id="pokedex"></p>
</body>
</html>
Here is the CSS from the video so far, tweaked a bit:
body {
background-color:rgb(181, 219, 255);
}
.card {
list-style: none;
padding: 40px;
background-color: #f4f4f4;
color: #222;
}
#pokedex {
padding-inline-start: 0;
display: grid;
grid-gap: 30px;
grid-template-columns: repeat(auto-fit, minmax(150px,1fr));
}
which gives us a webpage looking like this:
For now this seems like a good stopping point for this first blog entry. This was helpful for going through the Pokemon API, and hopefully it'll get easier from here on out!
This content originally appeared on DEV Community and was authored by nozumaz
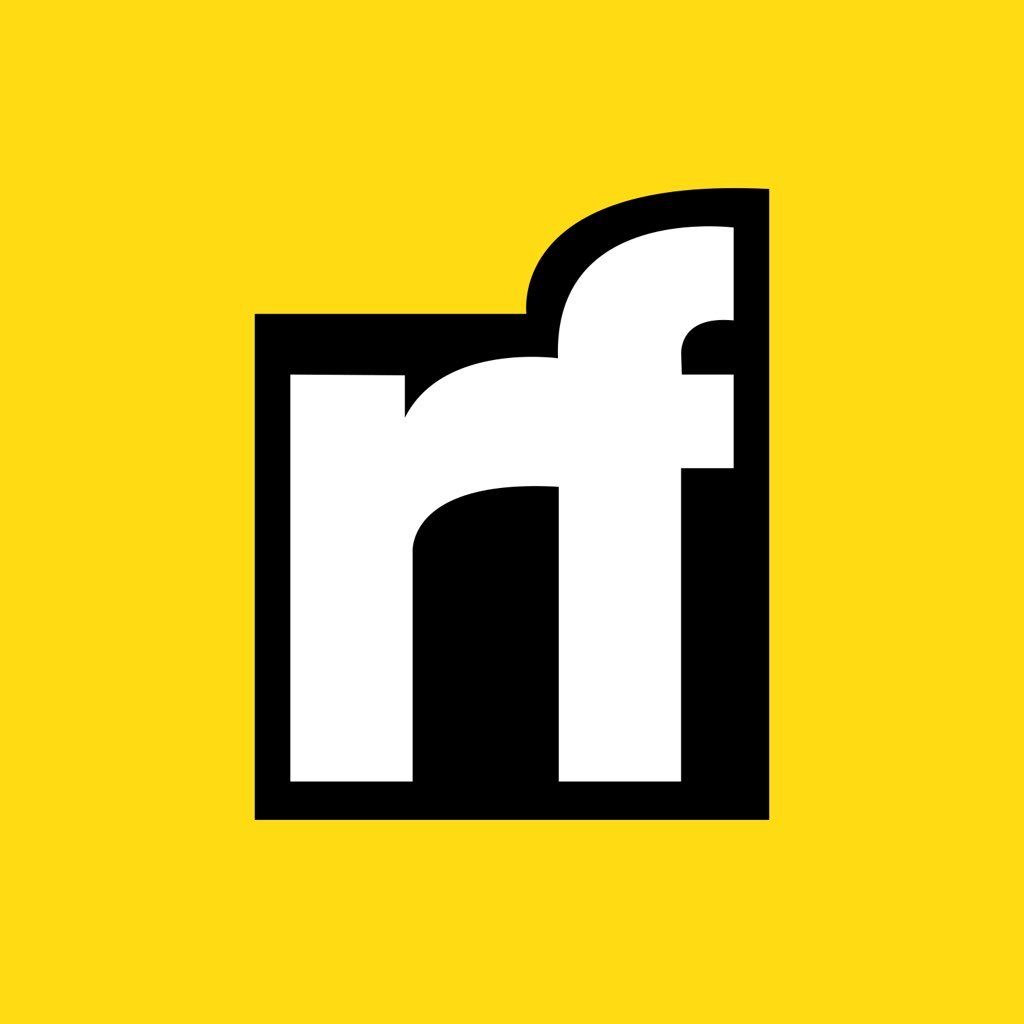
nozumaz | Sciencx (2023-05-14T02:42:05+00:00) First PokeAPI Project. Retrieved from https://www.scien.cx/2023/05/14/first-pokeapi-project/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.