This content originally appeared on Level Up Coding - Medium and was authored by Tarek
In this article, I’ll share my personal experiences with daemon and non-daemon threads in Python, and provide practical advice on how to choose the right thread type for your application.
Understanding Daemon and Non-Daemon Threads
In Python threading, a daemon thread is a thread that runs in the background, and is not expected to complete its execution before the program exits. On the other hand, non-daemon threads are critical to the functioning of the program, and they prevent the main program from exiting until they have completed their execution.
Non-daemon Example:
from threading import Thread
import time
def run():
time.sleep(3)
if __name__ == "__main__":
t = Thread(target=run)
t.start()
Output:
> time python3 thread.py
python3 thread.py 0.04s user 0.01s system 1% cpu 3.062 total
The execution time is 3.062s which means that the program had to wait for the thread to finish.
Daemon Example:
from threading import Thread
import time
def run():
time.sleep(3)
if __name__ == "__main__":
t = Thread(target=run)
t.setDaemon(True)
t.start()
Output:
> time python3 thread.py
python3 thread.py 0.04s user 0.02s system 67% cpu 0.078 total
The execution time is 0.078s which means that the program didn’t wait for the thread to finish, instead it crashes the daemon thread.
Advantages and Disadvantages of Daemon Threads
One of the main advantages of daemon threads is that they can run in the background without blocking the main program. This is useful for tasks that are not critical to the functioning of the program, such as logging or monitoring.
Another advantage of daemon threads is that they can be used to perform periodic tasks, such as cleaning up temporary files or sending heartbeat signals, without the need for a separate scheduler.
However, there are also some disadvantages to using daemon threads. Since daemon threads are not critical to the functioning of the program, they may be terminated abruptly if the main program exits before they have completed their execution. This can lead to unexpected behavior and potential data loss.
How to Exit Python Daemon Thread Gracefully?
My advice would be not to use daemon in the first place, this way you can initiate a thread and be assured that it can clean-up its resources safely. However, a possible solution to use a daemon and gracefully terminate it is to use “threading.Event”, where you can track within the daemon.
Example:
import threading
import time
class MyDaemonThread(threading.Thread):
def __init__(self):
super().__init__()
self._stop_event = threading.Event()
def run(self):
while not self._stop_event.is_set():
# Do some work here...
print("Working...")
time.sleep(4)
# Clean up any resources used by the daemon thread here...
print("Daemon thread exiting gracefully")
def stop(self):
self._stop_event.set()
daemon_thread = MyDaemonThread()
daemon_thread.setDaemon(True)
daemon_thread.start()
time.sleep(1)
daemon_thread.stop()
daemon_thread.join()
# Program can exit normally here
Output:
> time python3 thread.py
Working...
Daemon thread exiting gracefully
python3 thread.py 0.04s user 0.02s system 1% cpu 4.069 total
Pitfalls to Avoid When Using Daemon Threads
Now that we have discussed some pros and cons of using daemon threads, it’s time to talk about some of the potential pitfalls to avoid when working with them.
Joining on Daemon Threads
As previously discussed, daemon threads are designed to automatically terminate when the main thread exits. Therefore, it is generally not advisable to use the join() method to explicitly wait for a daemon thread to complete its execution. This is because daemon threads are often implemented with infinite loops that are intended to keep running indefinitely.
Relying on Daemon Threads to Clean Up Resources
While daemon threads are useful for performing background tasks that don’t need to complete before the program exits, they should not be relied upon to clean up resources like file handles, database connections, or network sockets. The reason for this is that daemon threads are terminated abruptly when the program exits.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
Python Threading: When to Use Daemon Threads and When to Avoid Them was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Tarek
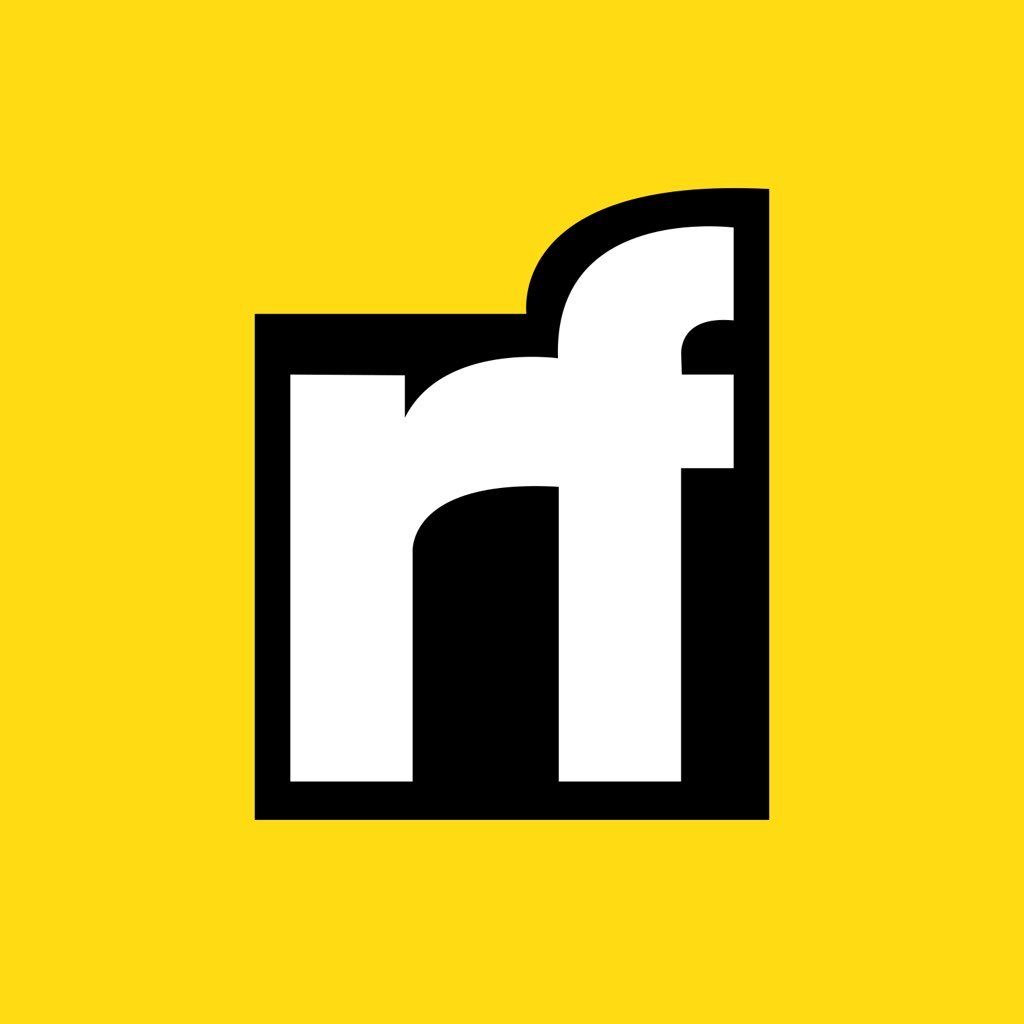
Tarek | Sciencx (2023-05-14T15:25:37+00:00) Python Threading: When to Use Daemon Threads and When to Avoid Them. Retrieved from https://www.scien.cx/2023/05/14/python-threading-when-to-use-daemon-threads-and-when-to-avoid-them/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.