This content originally appeared on Level Up Coding - Medium and was authored by Haseeba
Python is a powerful and versatile programming language that can be used for various tasks, such as web development, data analysis, machine learning, and more. One of the most common tasks that you can do with Python is reading and writing files. Whether it’s a simple text file, a complex server log, or even a binary file, Python can handle it with ease.
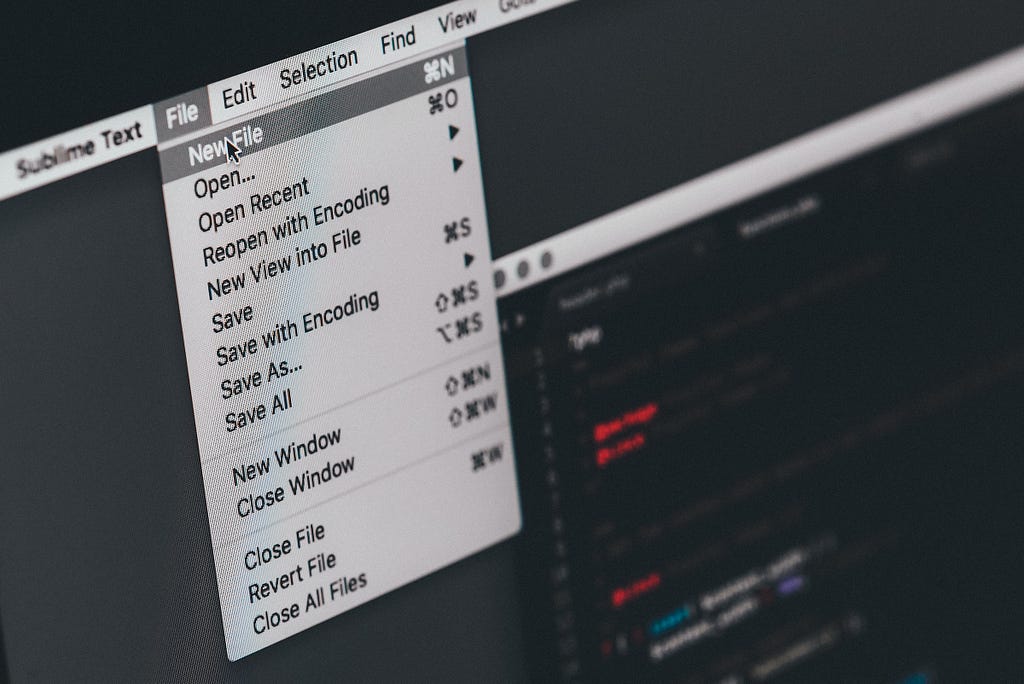
In this blog post, you will learn how to read and write a file in Python using the built-in “open()” function and the file object that it returns. You will also learn about the different access modes that you can use to open a file and the various methods that you can use to read from a file object.
What is Python File Handling?
Python file handling is the process of working with files in Python. It involves opening, reading, writing, and closing files using Python code. Python file handling allows you to manipulate the data stored in files and perform various operations on them.
Python file handling is based on the concept of a file object. A file object is an object that represents a file in Python. It has attributes and methods that allow you to access and modify the file’s contents. You can create a file object by using the “open()” function.
How to Open a File in Python?
The “open()” function is the main function that you use to open a file in Python. It takes two arguments: the filename and the access mode. The filename is the name of the file that you want to open, and the access mode is a string that specifies how you want to open the file.
The access mode determines what kind of operations you can perform on the file object. There are several access modes that you can use, but the most common ones are:
- “r”`: Read-only mode. You can only read from the file object. This is the default mode if you don’t specify any.
- “w”: Write-only mode. You can only write to the file object. If the file already exists, it will be overwritten. If the file does not exist, it will be created.
- “a”: Append mode. You can only write to the end of the file object. If the file does not exist, it will be created.
- “r+”: Read-write mode. You can read from and write to the file object.
- “w+”: Write-read mode. You can write to and read from the file object.
If the file already exists, it will be overwritten. If the file does not exist, it will be created. - “a+”: Append-read mode. You can write to the end of and read from the file object. If the file does not exist, it will be created.
To open a file in Python, you use the following syntax:
file = open(filename, access_mode)
This will return a file object that you can use to read from or write to the file.
For example
- If you want to open a file called “Python.txt” in read-only mode, remember that the file exists we want to read it:
file=open("Python.txt", "r")
- If we try to open a file that doesn’t exist, a new file is created:
file_2=open("output", "w")
How to Read a File in Python?
Once you have opened a file in Python using the “open()” function and obtained a file object, you can use various methods to read from it.
- The most basic method is “read()”, which reads all the contents of the file object and returns them as a string. For example:
file=open("Python.txt", "r")
text=file.read()
print(text)
output:
Python is a high-level, general-purpose programming language.
Its design philosophy emphasizes code readability with the use of significant indentation via the off-side rule.
Python is dynamically typed and garbage-collected.
However, reading all the contents of a large file at once may not be very efficient or practical. You may want to read only a certain amount of bytes or characters from the file object at a time.
To do this, you can pass an argument to “read()” that specifies how many bytes or characters you want to read. For example:
file=open("Python_Wikipedia.txt", "r")
text=file.read(22)
print(text)
This will print only 22 bytes or characters from ”Python.txt”:
Python is a high-level
2. Another method that you can use to read from a file object is “readline()”, which reads one line at a time from the file object and returns it as a string. For example:
file=open("Python.txt", "r")
text=file.readline()
print(text)
This will print the first line of “Python.txt”:
Python is a high-level, general-purpose programming language.
You can call “readline()” again to read the next line, and so on.
file=open("Python.txt","r")
print(file.readline())
print(file.readline())
output:
Python is a high-level, general-purpose programming language.
Its design philosophy emphasizes code readability with the use of significant indentation via the off-side rule.
However, if you want to read all the lines of a file in a more convenient way, you can use the “readlines()” method, which reads all the lines of the file object and returns them as a list of strings. For example:
file=open("Python.txt", "r")
text=file.readlines()
print(text)
output:
['Python is a high-level, general-purpose programming language.\n', 'Its design philosophy emphasizes code readability with the use of significant indentation via the off-side rule.\n', 'Python is dynamically typed and garbage-collected. ']
Alternatively, you can use a for loop to iterate over the file object directly, without using “readline()” or “readlines()”. This is more memory-efficient and Pythonic. For example:
file=open("Python.txt", "r")
for line in file:
print(line)
output:
Python is a high-level, general-purpose programming language.
Its design philosophy emphasizes code readability with the use of significant indentation via the off-side rule.
Python is dynamically typed and garbage-collected.
3. You should always close the file object after you are done with it, using the “close()” method. This will free up any system resources used by the file. For example:
file=open("Python.txt", "r")
print(file.read())
file.close()
4. You can also use the “seek()” method to move the file pointer to a different position in the file object. This allows you to read from or write to a specific location in the file. The “seek()” method takes an offset (number of bytes) as an argument and moves the pointer to that position from the beginning of the file. For example:
file=open("Python.txt", "r")
file.seek(12) # Move the pointer 12 bytes from the beginning
print(file.read(10)) # Read 10 bytes from that position
output:
high-level
5. You can also use the “tell()” method to get the current position of the file pointer in bytes. This can be useful if you want to save or restore the pointer’s position. For example:
pos = file.tell() # Get the current position
print(pos)
file.seek(10) # Move the pointer 10 bytes from the beginning
pos = file.tell() # Get the new position
print(pos)
output:
0
10
6. counting lines in a file: we can use the for statement to iterate through a sequence(a file handle open for read can be treated as a sequence of strings where each line in the file is a string in the sequence). we used for loop to read each line, count the lines and print out the number of lines.
file=open("Python.txt", "r")
count=0
for line in file:
count=count+1
print('line count:', count)
output:
line count: 3
7. searching through a file: we use the if statement in for loop to only print lines that meet some criteria.
file=open("Python.txt", "r")
for line in file:
if line.startswith('Its'):
print(line)
output:
Its design philosophy emphasizes code readability with the use of significant indentation via the off-side rule.
8. Selecting the lines: if you want to select the line in which the word “python” is contained, we use an if statement with “not” which means that if the line which not contain the “Python” word will be skipped.
file=open("Python.txt", "r")
for line in file:
if not "Python" in line:
continue
print(line)
output:
Python is a high-level, general-purpose programming language.
Python is dynamically typed and garbage-collected.
How to write a file in Python?
If you want to write to a file in Python, you need to open the file in write mode or append mode. Write mode will overwrite the existing file if it exists, or create a new file if it does not. Append mode will write to the end of the file without deleting any existing data.
- To write to a file in Python, you can use the “write()” method of the file object. The “write()” method takes a string as an argument and writes it to the file. For example:
file = open('file_1.txt', 'w')
file.write('I like python programming')
file.close()
You should always close the file object after you are done with it, using the “close()” method. This will ensure that all the data is written to the file and prevent any data loss or corruption
output:
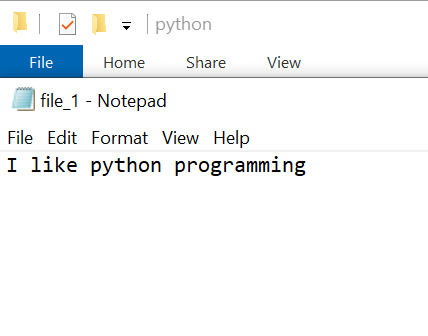
This will create a file called ‘file_1.txt’ and write ‘I like Python programming’ to it.
2. writing line to the existing file we use append mode:
file = open('file_1.txt', 'a')
file.write('I am learning python programming')
file.close()
output:
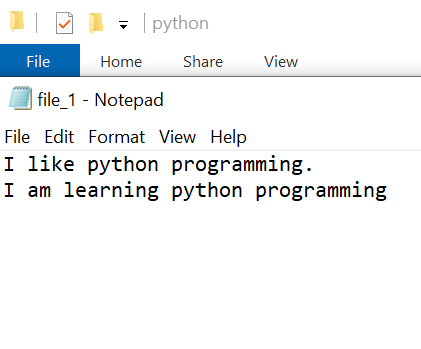
3. You can also use the “writelines()” method to write a list of strings to the file. For example:
file = open('file_6.txt', 'w')
lines = ['I am learning python programming\n', 'Python is a popular programming language\n']
file.writelines(lines)
file.close()
This will create a file called ’file_1.txt’ and write the lines in the list to it.
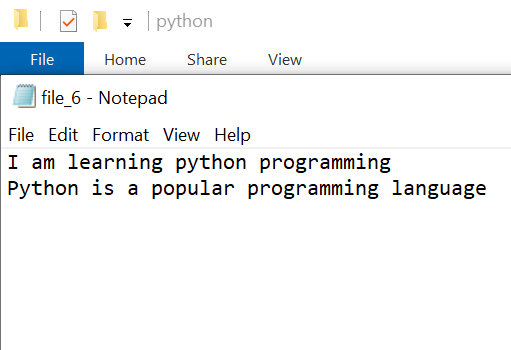
Note that you need to add the newline character (‘\n’) at the end of each line if you want them to appear on separate lines in the file.
4. You can also use string formatting or f-strings to create dynamic strings that can be written to the file. For example:
profession = 'programmer'
experience = 5
file = open('file_3.txt', 'w')
file.write(f'I am a {profession} and my {experience} is years.')
file.close()
output:
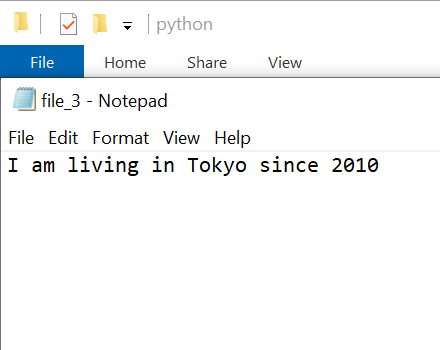
Conclusion
In this blog post, you learned how to read and write a file in Python using the built-in “open()” function and the file object. You also learned about the different access modes that you can use to open a file and the various methods that you can use to read from a file object.
Python file handling is a very useful skill that can help you with various tasks, such as data analysis, web development, automation, and more. By mastering Python file handling, you can take your Python skills to the next level.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
How to Read and Write a File in Python: A Guide to Python File Handling was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Haseeba
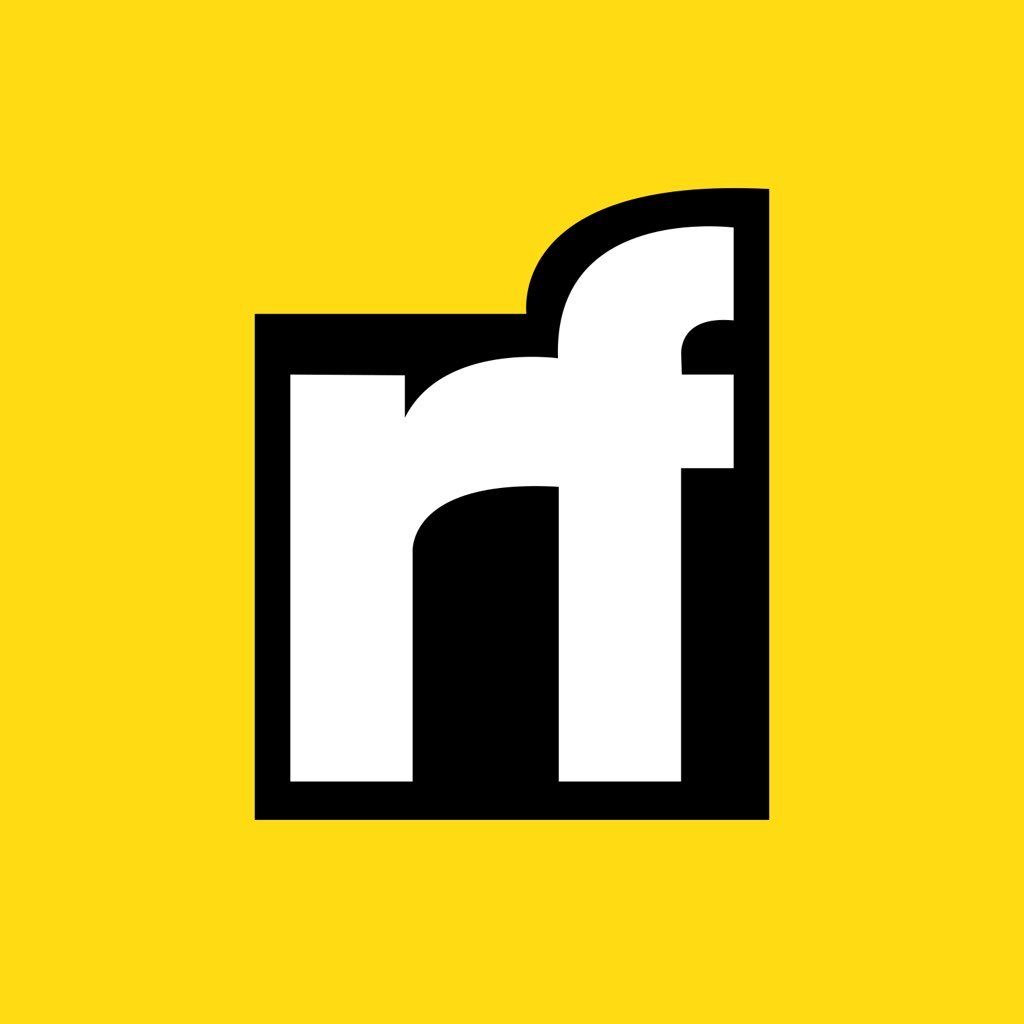
Haseeba | Sciencx (2023-05-19T16:17:14+00:00) How to Read and Write a File in Python: A Guide to Python File Handling. Retrieved from https://www.scien.cx/2023/05/19/how-to-read-and-write-a-file-in-python-a-guide-to-python-file-handling/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.