This content originally appeared on Level Up Coding - Medium and was authored by Sarvsav Sharma
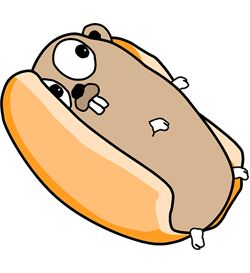
Business and IT don’t always understand each other. Godog’s executable specifications encourage closer collaboration, helping teams keep the business goal in mind at all times. ~ Godog Cucumber
How to write your first godogs behaviour driven development feature?
- Start initializing your project with go mod init , using below command:
$ go mod init github.com/sarvsav/godogs-example
2. The business team has features , and the best practice to define them is inside the features folder. Suppose, we have to design a feature for the bank, where the reservation to visit the office will be accepted only for working business hours. Monday — Friday: 7:30 AM to 5 PM. If someone tries to book outside office hours, it will display the message to book during working hours. And, the number of available slots available per day is 10.
3. Start describing the feature in plain text format first. The feature details will be present in features/book-a-meeting.feature file. This text file will follow the Gherkin syntax. And, more details can be found here — Gherkin Docs.
Gherkin serves multiple purposes:
- Unambiguous executable specification
- Automated testing using Cucumber
- Document how the system actually behaves
Feature: Book a meeting
In order to be able to book a meeting
As a bank customer is able to book a meeting with a bank employee
Scenario: Book a successful meeting
Given I am a bank customer
When I book a meeting
Then I should be told "booking is successful"
Starting with a very basic scenario, and without checks.
- The first line of the file, briefly tells about the Feature, and as a best practice, it would be good if it matches with the filename. It is like title or subject line.
- The second and third lines are brief description of the feature. Cucumber does not execute these lines because it’s documentation.
- The fifth line, Scenario: Book a successful meeting is a scenario, which is a concrete example illustrating how the software should behave.
- The last three lines starting with Given, When and Then are the steps of our scenario. This is what Cucumber will execute.
4. Add a test file as well as main file to test our feature, and it should be added at project package root level with same name as package. Hence the file names would be godogs-example.go & godogs-example_test.go . And, after adding this empty, try to run the tests. The output would be, no tests to run.
$ go test
testing: warning: no tests to run
PASS
ok github.com/sarvsav/godogs-example 0.192s
5. Start writing the Given , When , and Then steps in your test file with godog test suite and scenario.
File: godogs-example.go
package main
// MeetingSlots availablity
var MeetingSlots int
// UserRole is a employee or customer
var UserRole string
func main() { /* usual main func */ }
File: godogs-example_test.go
package main
import (
"testing"
"github.com/cucumber/godog"
)
func iAmABankCustomer() error {
return godog.ErrPending
}
func iBookAMeeting() error {
return godog.ErrPending
}
func iShouldBeTold() error {
return godog.ErrPending
}
func TestFeatures(t *testing.T) {
suite := godog.TestSuite{
ScenarioInitializer: InitializeScenario,
Options: &godog.Options{
Format: "pretty",
Paths: []string{"features"},
TestingT: t, // Testing instance that will run subtests.
},
}
if suite.Run() != 0 {
t.Fatal("non-zero status returned, failed to run feature tests")
}
}
func InitializeScenario(sc *godog.ScenarioContext) {
sc.Step(`^I am a bank customer$`, iAmABankCustomer)
sc.Step(`^I book a meeting$`, iBookAMeeting)
sc.Step(`^I should be told "([^"]*)"$`, iShouldBeTold)
}
On running the above codebase, it will give detailed information about the test.
$ go test -v
=== RUN TestFeatures
Feature: Book a meeting
In order to be able to book a meeting
As a bank customer is able to book a meeting with a bank employee
=== RUN TestFeatures/Book_a_successful_meeting
Scenario: Book a successful meeting # features\book-a-meeting.feature:5
Given I am a bank customer # godogs-example_test.go:10 -> github.com/sarvsav/godogs-example.iAmABankCustomer
TODO: write pending definition
When I book a meeting # godogs-example_test.go:14 -> github.com/sarvsav/godogs-example.iBookAMeeting
Then I should be told "booking is successful" # godogs-example_test.go:18 -> github.com/sarvsav/godogs-example.iShouldBeTold
suite.go:451: step implementation is pending
1 scenarios (1 pending)
3 steps (1 pending, 2 skipped)
12.464ms
--- FAIL: TestFeatures (0.04s)
--- FAIL: TestFeatures/Book_a_successful_meeting (0.01s)
FAIL
exit status 1
FAIL github.com/sarvsav/godogs-example 0.224s
6. For all the above functions, we just added godog.ErrPending that defines the implementation is yet to be done.
7. Start adding the functional logic to the test file.
package main
import (
"context"
"errors"
"testing"
"time"
"github.com/cucumber/godog"
)
// godogsCtxKey is the key used to store the available godogs in the context.Context
type godogsCtxKey struct{}
func iAmABankCustomer(ctx context.Context, isBankCustomer string) (context.Context, error) {
// isBankCustomer is a boolean value that verifies if the customer is a bank customer or not
return context.WithValue(ctx, godogsCtxKey{}, isBankCustomer), nil
}
func iBookAMeeting(ctx context.Context) (context.Context, error) {
// Here we check for conditions the day and time of the meeting
// and availablity of the slots
weekday := time.Now().Weekday()
if weekday == time.Saturday || weekday == time.Sunday {
return ctx, errors.New("Weekend meetings are not allowed")
}
// Just for demo, we are setting the available slots to 10
availableSlots := 10
if availableSlots <= 0 {
return ctx, errors.New("No slots available")
}
return ctx, nil
}
func iShouldBeTold(ctx context.Context) error {
userIsBankCustomer := ctx.Value(godogsCtxKey{}).(string)
if userIsBankCustomer == "customer" {
return nil
}
return errors.New("User is not a bank customer")
}
func TestFeatures(t *testing.T) {
suite := godog.TestSuite{
ScenarioInitializer: InitializeScenario,
Options: &godog.Options{
Format: "pretty",
Paths: []string{"features"},
TestingT: t, // Testing instance that will run subtests.
},
}
if suite.Run() != 0 {
t.Fatal("non-zero status returned, failed to run feature tests")
}
}
func InitializeScenario(sc *godog.ScenarioContext) {
sc.Step(`^I am a bank (\w+)$`, iAmABankCustomer)
sc.Step(`^I book a meeting$`, iBookAMeeting)
sc.Step(`^I should be told "([^"]*)"$`, iShouldBeTold)
}
Running the tests:
$ go test -v
=== RUN TestFeatures
Feature: Book a meeting
In order to be able to book a meeting
As a bank customer is able to book a meeting with a bank employee
=== RUN TestFeatures/Book_a_successful_meeting
Scenario: Book a successful meeting # features\book-a-meeting.feature:5
Given I am a bank customer # godogs-example_test.go:15 -> github.com/sarvsav/godogs-example.iAmABankCustomer
When I book a meeting # godogs-example_test.go:20 -> github.com/sarvsav/godogs-example.iBookAMeeting
Then I should be told "booking is successful" # godogs-example_test.go:37 -> github.com/sarvsav/godogs-example.iShouldBeTold
1 scenarios (1 passed)
3 steps (3 passed)
16.0478ms
--- PASS: TestFeatures (0.02s)
--- PASS: TestFeatures/Book_a_successful_meeting (0.01s)
PASS
ok github.com/sarvsav/godogs-example 0.640s
Yay!! All test cases passed. 🎉
Source code can be downloaded from below repository.
GitHub - sarvsav/godogs-example
Spread the love ❤ by sharing the post. Thank you for reading.
Level Up Coding
Thanks for being a part of our community! Before you go:
- 👏 Clap for the story and follow the author 👉
- 📰 View more content in the Level Up Coding publication
- 💰 Free coding interview course ⇒ View Course
- 🔔 Follow us: Twitter | LinkedIn | Newsletter
🚀👉 Join the Level Up talent collective and find an amazing job
I am new to Behaviour Driven Development using Go was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Sarvsav Sharma
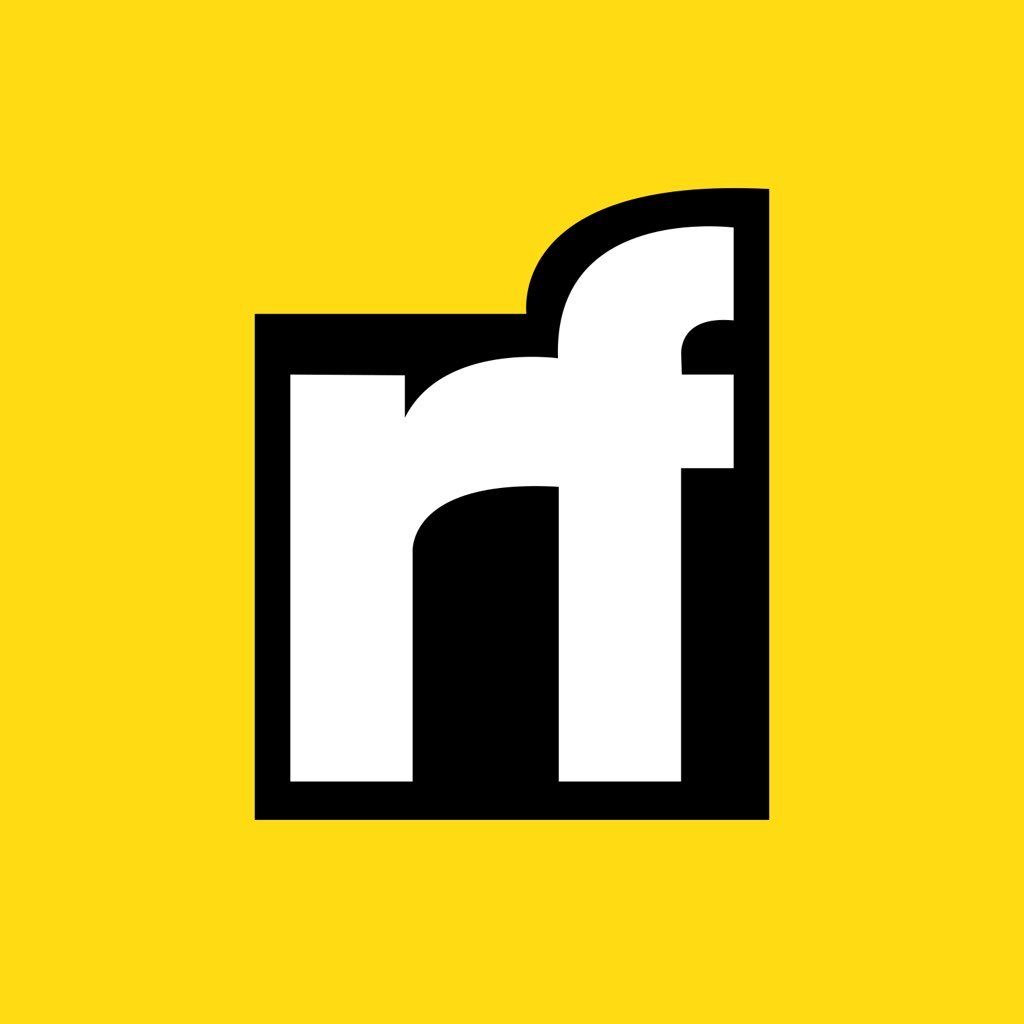
Sarvsav Sharma | Sciencx (2023-05-19T16:17:19+00:00) I am new to Behaviour Driven Development using Go. Retrieved from https://www.scien.cx/2023/05/19/i-am-new-to-behaviour-driven-development-using-go/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.