This content originally appeared on Bits and Pieces - Medium and was authored by Teslenko Oleh
Part 3: Understanding the Liskov Substitution Principle (LSP)
Hey there, fellow Angular developers! When it comes to crafting top-notch code, SOLID principles are our secret sauce. Today, we’re going to dive into one of those principles called the Liskov Substitution Principle (LSP). It’s all about designing classes and their relationships in a way that keeps our Angular projects flexible, extensible, and rock-solid. So, let’s explore LSP and see how it can level up our Angular game. We’ll walk through two examples — one without LSP and another with LSP — to see the difference it makes.
Understanding LSP:
So, LSP simply says that we should be able to replace objects of a base class with any of its subclasses without breaking stuff. In other words, if we have a base class and a bunch of derived classes, swapping them out should never lead to unexpected behavior or contract violations. It’s all about smooth substitution, my friends.
👉 Here are some Angular dev tools you could consider using.
Benefits of LSP in Angular:
Why should we care about LSP in Angular? Well, let me tell you:
Supercharged reusability: LSP unleashes the power of polymorphism, allowing us to interchange derived classes with their base class. This means we can reuse code components like a boss, reducing duplication and ramping up productivity.
Turbocharged extensibility: LSP ensures that adding new derived classes to our Angular project won’t cause us to tear our hair out modifying existing code. We can extend functionality without breaking things, keeping our codebase tidy and our sanity intact.
Effortless testing: LSP makes testing in Angular a piece of the cake. We can seamlessly swap derived classes for their base class during unit tests. This makes it a breeze to validate system behavior without creating an army of test cases or juggling code like a circus performer.
💡 If you’re sold on the benefits of LSP for your Angular projects, you might want to consider using an open-source tool like Bit. With Bit, you can harvest any reusable Angular component from your codebase and reuse it across all your projects. Moreover, using Bit’s Compositions feature, you can automatically create *.spec.* and recognize *.test.* files, and every component you publish and version on Bit will include tests as essentials, giving you confidence in your code.
Learn more:
Example 1: Without Liskov Substitution Principle in Angular
Picture this: we have a base class called Shape, and we’re all excited to create a couple of derived classes, Rectangle and Circle. But wait! Without sticking to LSP, we might end up with a messy situation. We implement a method in Shape called calculateArea() that returns the area of the shape. Now, in the Circle class, we need to pass additional parameters like radius to calculate its area. Uh-oh! We’ve just violated LSP. The Circle class expects something extra that the base class doesn’t, leading to inconsistency and potential bugs. Yikes!
Example 2: Applying Liskov Substitution Principle in Angular
No worries, we’ve got this! Let’s fix that LSP violation. Instead of adding parameters to calculateArea() in Circle, we can override the method and implement it using the radius property defined in the Circle class. That way, we maintain substitutability and ensure that replacing instances of the base class with Rectangle or Circle won't cause any unexpected surprises.
Before we wrap up, let’s quickly compare the Liskov Substitution Principle (LSP) with two other SOLID principles: the Single Responsibility Principle (SRP) and the Open-Closed Principle (OCP) which we learned in previous articles.
- Single Responsibility Principle (SRP): SRP states that a class should have only one reason to change, meaning it should have a single responsibility. It focuses on keeping classes focused and cohesive. While LSP deals with class hierarchies and substitutability, SRP ensures that each class has a well-defined purpose. Both principles work hand in hand to create maintainable and modular code.
Best Design Principles in Angular
- Open-Closed Principle (OCP): OCP states that classes should be open for extension but closed for modification. It emphasizes designing classes in a way that allows adding new functionality without modifying existing code. LSP complements OCP by ensuring that derived classes can seamlessly substitute their base classes, enabling extension without breaking the system’s behavior. Together, LSP and OCP promote code flexibility and extensibility.
Best Design Principles in Angular
Liskov Substitution Principle (LSP) is a crucial SOLID principle in Angular development. By adhering to LSP, we ensure that objects of derived classes can seamlessly replace objects of their base classes without introducing unexpected behavior. It works hand in hand with other SOLID principles such as the Single Responsibility Principle (SRP) and the Open-Closed Principle (OCP) to create maintainable, extensible, and modular code. Embrace LSP, SRP, and OCP in your Angular projects, and you’ll be on your way to building solid foundations and crafting top-notch applications. Happy coding!
Thanks for your attention, if you like this article you can 👏 , follow me and write a comment.
Build Angular Apps with reusable components, just like Lego
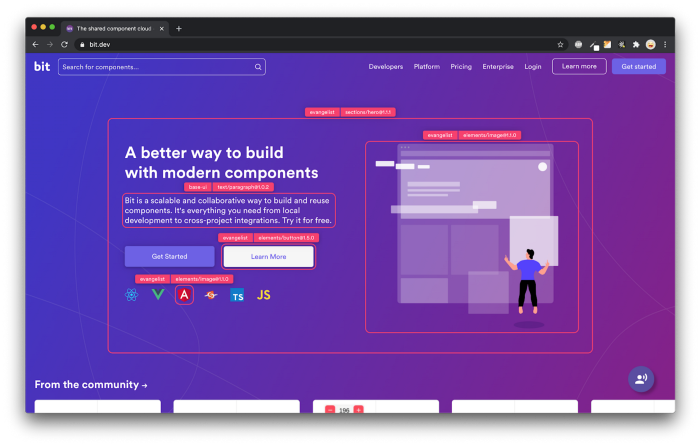
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Introducing Angular Component Development Environment
- 10 Useful Angular Features You’ve Probably Never Used
- Top 8 Tools for Angular Development in 2023
- Getting Started with a New Angular Project in 2023
- How We Build Micro Frontends
- How to Share Angular Components Between Projects and Apps
- How we Build a Component Design System
- Creating a Developer Website with Bit components
Best Design Principles in Angular was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Teslenko Oleh
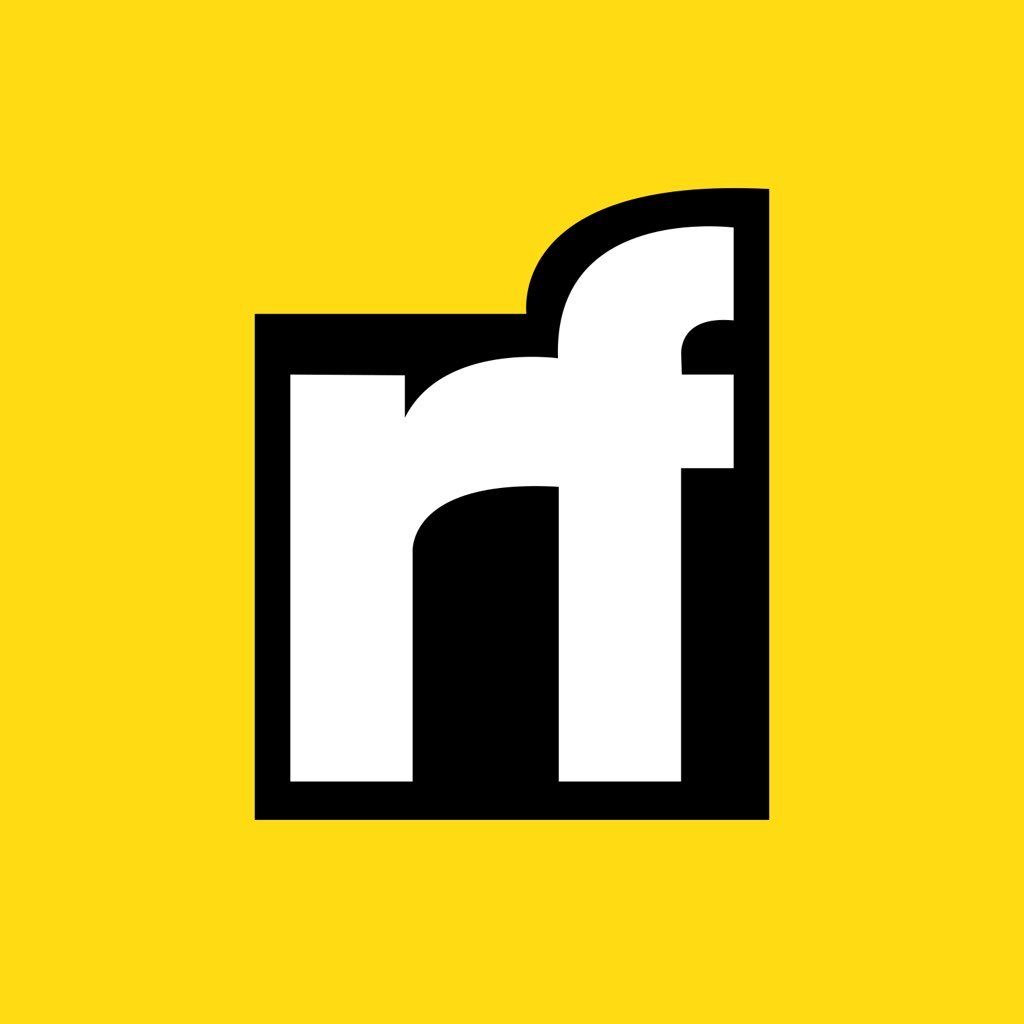
Teslenko Oleh | Sciencx (2023-05-22T06:02:07+00:00) Best Design Principles in Angular. Retrieved from https://www.scien.cx/2023/05/22/best-design-principles-in-angular-3/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.