This content originally appeared on Bits and Pieces - Medium and was authored by Theodore John.S
Explore the useMemo hook in detail, understand its purpose, and examine real-time examples to demonstrate its effectiveness.
In React, optimizing performance is a crucial aspect of building high-performing web applications. One powerful tool in a React developer’s arsenal is the useMemo hook. This hook allows for the memoization of expensive computations, resulting in improved performance and responsiveness. In this article, we will explore the useMemo hook in detail, understand its purpose, and examine real-time examples to demonstrate its effectiveness.
Understanding the useMemo Hook:
The useMemo hook is designed to memoize the result of a function and cache it for future use. By memoizing expensive computations, we can avoid unnecessary recalculations, leading to significant performance enhancements. The hook takes two arguments: a function and an array of dependencies. When any of the dependencies change, the function is re-executed, and the result is memoized. If the dependencies remain the same, the cached value is returned, reducing the computational overhead.
How useMemo Works Behind the Scenes:
Behind the scenes, useMemo employs a technique known as memoization. It stores the result of the computation and checks for any changes in the dependencies array. If there are no changes, it returns the cached result. This optimization technique eliminates redundant calculations, leading to a more efficient rendering process.
Benefits of Using useMemo for Performance Optimization:
By utilizing the useMemo hook, developers can achieve several performance benefits:
- Avoiding Expensive Recalculations: Expensive computations can be time-consuming and resource-intensive. With useMemo, we can calculate a value only when its dependencies change, avoiding unnecessary recalculations and improving overall performance.
- Smoother User Experience: By optimizing computations, we can reduce the time spent on rendering, resulting in a smoother user experience. React components will update more efficiently, leading to faster response times and improved interactivity.
- Efficient Resource Utilization: Memoization optimizes resource utilization by preventing wasteful computations. By caching results, we can reduce the load on the CPU and memory, making our application more efficient.
- Memoizing Expensive Computations: One of the primary use cases for the useMemo hook is to memoize expensive computations. Let’s explore an example where we calculate the factorial of a given number.
Example : Memoizing Factorial Calculation
import React, { useMemo } from 'react';
const ExpensiveComputation = ({ number }) => {
const factorial = useMemo(() => {
let result = 1;
for (let i = 2; i <= number; i++) {
result *= i;
}
return result;
}, [number]);
return (
<div>
Factorial of {number} is: {factorial}
</div>
);
};
const App = () => {
return <ExpensiveComputation number={5} />;
};
export default App;
Explanation: In the code snippet above, we have a functional component called ExpensiveComputation that receives a number prop. Inside the component, we define a memoized variable factorial using the useMemo hook. The provided function calculates the factorial of the number prop. The dependencies array [number] ensures that the memoized value is updated whenever the number prop changes.
The code flow in this example is as follows:
- The App component renders the ExpensiveComputation component with the number prop set to 5.
- The useMemo hook is called, and the factorial calculation function is executed to calculate the factorial of 5.
- The result of the factorial calculation is memoized and stored in the factorial variable.
- The memoized value is returned and displayed in the component’s JSX.
By memoizing the factorial calculation, we avoid redundant computations when the number prop remains the same, enhancing the performance of our application.
Preventing Unnecessary Re-renders:
In certain scenarios, preventing unnecessary re-renders can significantly impact performance. The useMemo hook can help optimize such situations by memoizing values that don’t depend on changing dependencies. Let’s consider an example where we display a timestamp that shouldn’t update on state changes.
Example: Preventing Unnecessary Re-renders
import React, { useState, useMemo } from 'react';
const Timestamp = () => {
const [count, setCount] = useState(0);
const currentTime = useMemo(() => new Date().toLocaleTimeString(), []);
return (
<div>
<p>Count: {count}</p>
<p>Current Time: {currentTime}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
const App = () => {
return <Timestamp />;
};
export default App;
Explanation: In the above code snippet, we have a functional component called Timestamp that maintains a count state using the useState hook. We also have a memoized value currentTime, which is calculated using the useMemo hook. The dependencies array [] ensures that the currentTime value is memoized and doesn't change on subsequent re-renders caused by state updates.
The code flow in this example is as follows:
- The component Timestamp is rendered initially.
- The currentTime variable is memoized using useMemo and stores the initial value of the current time.
- When the setCount function is called on button click, the component re-renders, but the currentTime value remains the same since it doesn't depend on any state or prop changes.
- Only the count value gets updated and displayed.
By memoizing the current time calculation, we prevent unnecessary re-renders of the component when state updates occur.
Memoizing Callback Functions:
In addition to memoizing values, the useMemo hook can also be used to memoize callback functions. This optimization is particularly useful for event handlers or prop callbacks where we want to avoid re-creating the same function instance on each render. By combining useMemo with useCallback, we can achieve optimal performance in such scenarios.
Example: Memoizing an Event Handler
import React, { useCallback, useMemo } from 'react';
const Form = () => {
const handleSubmit = useCallback(() => {
// Perform form submission logic
}, []);
const memoizedHandleSubmit = useMemo(() => handleSubmit, [handleSubmit]);
return (
<form onSubmit={memoizedHandleSubmit}>
{/* Form inputs */}
<button type="submit">Submit</button>
</form>
);
};
const App = () => {
return <Form />;
};
export default App;
Explanation: In the above example, we have a component called Form that includes a form with an event handler handleSubmit. To optimize performance, we memoize the handleSubmit function using the useCallback hook. We then further memoize it using the useMemo hook to create a memoized instance of the callback function.
The code flow in this example is as follows:
- The Form component renders initially.
- The handleSubmit function is created using the useCallback hook and memoized.
- The memoized callback memoizedHandleSubmit is created using the useMemo hook and depends on the handleSubmit function.
- The memoizedHandleSubmit is passed as the event handler to the form's onSubmit prop.
By memoizing the event handler, we ensure that the same function instance is used across re-renders, preventing unnecessary re-renders of child components that depend on this callback.
💡 As an aside, if you wanted to create custom React Hooks using other React Hooks, you could reuse them across multiple projects using an open-source tool like Bit.
Learn more:
How to reuse React components across your projects
Caveats and Considerations:
While useMemo is a powerful optimization tool, it’s essential to understand its limitations and use it appropriately. Some key considerations include:
- Avoid Overusing Memoization: Memoization should be used for computations that are genuinely expensive and can benefit from caching. Applying memoization to every computation can lead to unnecessary complexity and potential performance degradation.
- Evaluate Dependencies Carefully: The dependencies array passed to useMemo determines when the memoized value is recalculated. Ensure that all relevant dependencies are included to avoid stale data. Incorrect or missing dependencies can lead to bugs or incorrect behavior.
- Performance Trade-offs: While memoization can improve performance, it comes with a trade-off in terms of memory usage. Memoizing large or frequently changing data can increase memory consumption. Evaluate the performance impact against the memory usage in your specific use cases.
Best Practices for Using useMemo:
To effectively utilize the useMemo hook and maximize performance benefits, consider the following best practices:
- Identify Expensive Computations: Analyze your components and identify computations that are computationally expensive or have a high probability of being repeated frequently.
- Measure Performance Impact: Benchmark and measure the performance gain achieved by applying memoization. This helps you make informed decisions about where to apply memoization and evaluate its effectiveness.
- Fine-tune Dependencies: Carefully review and choose the dependencies array for useMemo. Include only the necessary dependencies that affect the computation result. Avoid excessive dependencies to prevent unnecessary recalculations.
- Use Memoization Strategically: Apply memoization to computations and functions that truly benefit from caching. Consider the trade-offs and ensure that the performance gain outweighs the additional complexity introduced by memoization.
Real-World Use Cases:
The useMemo hook finds practical applications in various scenarios. Here’s a real-world use case that demonstrates its effectiveness:
Memoizing a Complex Data Transformation in a Data Visualization Component In a data visualization component that handles large datasets, performing complex data transformations can be resource-intensive. By memoizing the transformation logic using useMemo, we can optimize the performance of the component. Let’s explore a simplified example.
Example: Memoizing Data Transformation
import React, { useMemo } from 'react';
const DataVisualization = ({ data }) => {
const transformedData = useMemo(() => {
// Perform complex data transformation
// ...
return transformedData;
}, [data]);
// Render the data visualization using transformedData
// ...
return <div>{/* Rendered visualization */}</div>;
};
const App = () => {
const data = fetchData(); // Fetch data from an API
return <DataVisualization data={data} />;
};
export default App;
Explanation: In the above example, we have a DataVisualization component that receives data as a prop. Inside the component, we use the useMemo hook to memoize the result of a complex data transformation. The memoized transformedData is then used to render the data visualization.
The code flow in this example is as follows:
- The App component renders and fetches data from an API.
- The fetched data is passed as a prop to the DataVisualization component.
- The useMemo hook is called, and the complex data transformation is performed only when the data prop changes.
- The memoized transformedData is stored and used for rendering the data visualization.
By memoizing the data transformation, we avoid recalculating the transformation logic on each render, resulting in improved performance when handling large datasets.
Conclusion:
The useMemo hook in React is a powerful tool for optimizing performance by memoizing expensive computations, preventing unnecessary re-renders, and memoizing callback functions. By utilizing memoization techniques strategically, React developers can create faster and more efficient applications.
Continuous performance monitoring, benchmarking, and optimization are essential for delivering optimal user experiences. By leveraging the useMemo hook and other performance optimization techniques, you can create highly performant React applications that delight users with their speed and responsiveness. Remember to measure the impact of memoization and fine-tune its usage based on your specific application requirements.
Hope the above article gave a better understanding. If you have any questions regarding the areas I have discussed in this article, areas of improvement don’t hesitate to comment below.
Build React apps with reusable components, just like Lego

Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Creating a Developer Website with Bit components
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
- How to Reuse and Share React Components in 2023: A Step-by-Step Guide
- 5 Tools for Building React Component Libraries in 2023
[Disclosure: This article is a collaborative creation blending my own ideation with the assistance of ChatGPT for optimal articulation.]
useMemo Hook in React: Boosting Performance with Memoization was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Theodore John.S
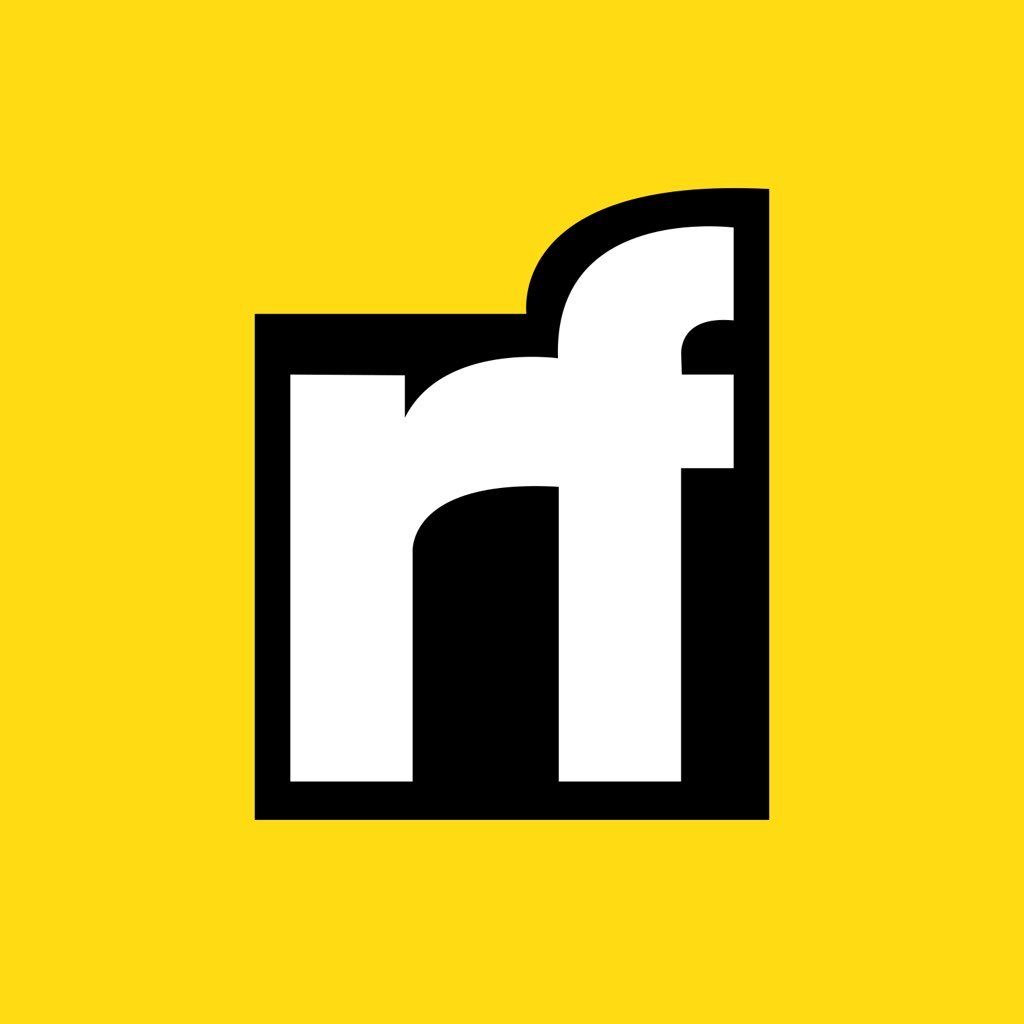
Theodore John.S | Sciencx (2023-06-05T18:23:54+00:00) useMemo Hook in React: Boosting Performance with Memoization. Retrieved from https://www.scien.cx/2023/06/05/usememo-hook-in-react-boosting-performance-with-memoization/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.