This content originally appeared on DEV Community and was authored by Super Kai (Kazuya Ito)
tile() can repeat the zero or more elements of a 0D or more D tensor as shown below:
*Memos:
-
tile()
can be used with torch or a tensor. - The 1st argument(
tensor
ofint
,float
,complex
orbool
) withtorch
or using a tensor(tensor
ofint
,float
,complex
orbool
) isinput
(Required). - The 2nd argument(
tuple
) withtorch
or the 1st argument(tuple
) with a tensor isdims
(Required). *Memos:- If at least one dimension is
0
, an empty tensor is returned. - The 1st argument...(
int
,int
...) with a tensor is alsodims
. *dims=
mustn't be used.
- If at least one dimension is
import torch
my_tensor = torch.tensor([3, 5, 1])
torch.tile(input=my_tensor, dims=(1,))
my_tensor.tile(dims=(1,))
# tensor([3, 5, 1])
torch.tile(input=my_tensor, dims=(2,))
# tensor([3, 5, 1, 3, 5, 1])
torch.tile(input=my_tensor, dims=(3,))
# tensor([3, 5, 1, 3, 5, 1, 3, 5, 1])
etc.
torch.tile(input=my_tensor, dims=(1, 1))
# tensor([[3, 5, 1]])
torch.tile(input=my_tensor, dims=(1, 2))
# tensor([[3, 5, 1, 3, 5, 1]])
torch.tile(input=my_tensor, dims=(1, 3))
# tensor([[3, 5, 1, 3, 5, 1, 3, 5, 1]])
etc.
torch.tile(input=my_tensor, dims=(2, 1))
# tensor([[3, 5, 1],
# [3, 5, 1]])
torch.tile(input=my_tensor, dims=(2, 2))
# tensor([[3, 5, 1, 3, 5, 1],
# [3, 5, 1, 3, 5, 1]])
torch.tile(input=my_tensor, dims=(2, 3))
# tensor([[3, 5, 1, 3, 5, 1, 3, 5, 1],
# [3, 5, 1, 3, 5, 1, 3, 5, 1]])
etc.
torch.tile(input=my_tensor, dims=(3, 1))
# tensor([[3, 5, 1],
# [3, 5, 1],
# [3, 5, 1]])
etc.
torch.tile(input=my_tensor, dims=(1, 1, 1))
# tensor([[[3, 5, 1]]])
etc.
torch.tile(input=my_tensor, dims=(1, 0, 1))
# tensor([], size=(1, 0, 3), dtype=torch.int64)
my_tensor.tile(3, 2, 1)
# tensor([[[3, 5, 1], [3, 5, 1]],
# [[3, 5, 1], [3, 5, 1]],
# [[3, 5, 1], [3, 5, 1]]])
my_tensor = torch.tensor([3., 5., 1.])
torch.tile(input=my_tensor, dims=(2,))
# tensor([3., 5., 1., 3., 5., 1.])
my_tensor = torch.tensor([3.+0.j, 5.+0.j, 1.+0.j])
torch.tile(input=my_tensor, dims=(2,))
# tensor([3.+0.j, 5.+0.j, 1.+0.j, 3.+0.j, 5.+0.j, 1.+0.j])
my_tensor = torch.tensor([True, False, True])
torch.tile(input=my_tensor, dims=(2,))
# tensor([True, False, True, True, False, True])
my_tensor = torch.tensor([[3, 5, 1],
[6, 0, 5]])
torch.tile(input=my_tensor, dims=(1,))
# tensor([[3, 5, 1],
# [6, 0, 5]])
torch.tile(input=my_tensor, dims=(2,))
# tensor([[3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5]])
torch.tile(input=my_tensor, dims=(3,))
# tensor([[3, 5, 1, 3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5, 6, 0, 5]])
etc.
torch.tile(input=my_tensor, dims=(1, 1))
# tensor([[3, 5, 1],
# [6, 0, 5]])
torch.tile(input=my_tensor, dims=(1, 2))
# tensor([[3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5]])
torch.tile(input=my_tensor, dims=(1, 3))
# tensor([[3, 5, 1, 3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5, 6, 0, 5]])
etc.
torch.tile(input=my_tensor, dims=(2, 1))
# tensor([[3, 5, 1],
# [6, 0, 5],
# [3, 5, 1],
# [6, 0, 5]])
torch.tile(input=my_tensor, dims=(2, 2))
# tensor([[3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5],
# [3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5]])
torch.tile(input=my_tensor, dims=(2, 3))
# tensor([[3, 5, 1, 3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5, 6, 0, 5],
# [3, 5, 1, 3, 5, 1, 3, 5, 1],
# [6, 0, 5, 6, 0, 5, 6, 0, 5]])
etc.
torch.tile(input=my_tensor, dims=(3, 1))
# tensor([[3, 5, 1],
# [6, 0, 5],
# [3, 5, 1],
# [6, 0, 5],
# [3, 5, 1],
# [6, 0, 5]])
etc.
torch.tile(input=my_tensor, dims=(1, 1, 1))
# tensor([[[3, 5, 1],
# [6, 0, 5]]])
etc.
This content originally appeared on DEV Community and was authored by Super Kai (Kazuya Ito)
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
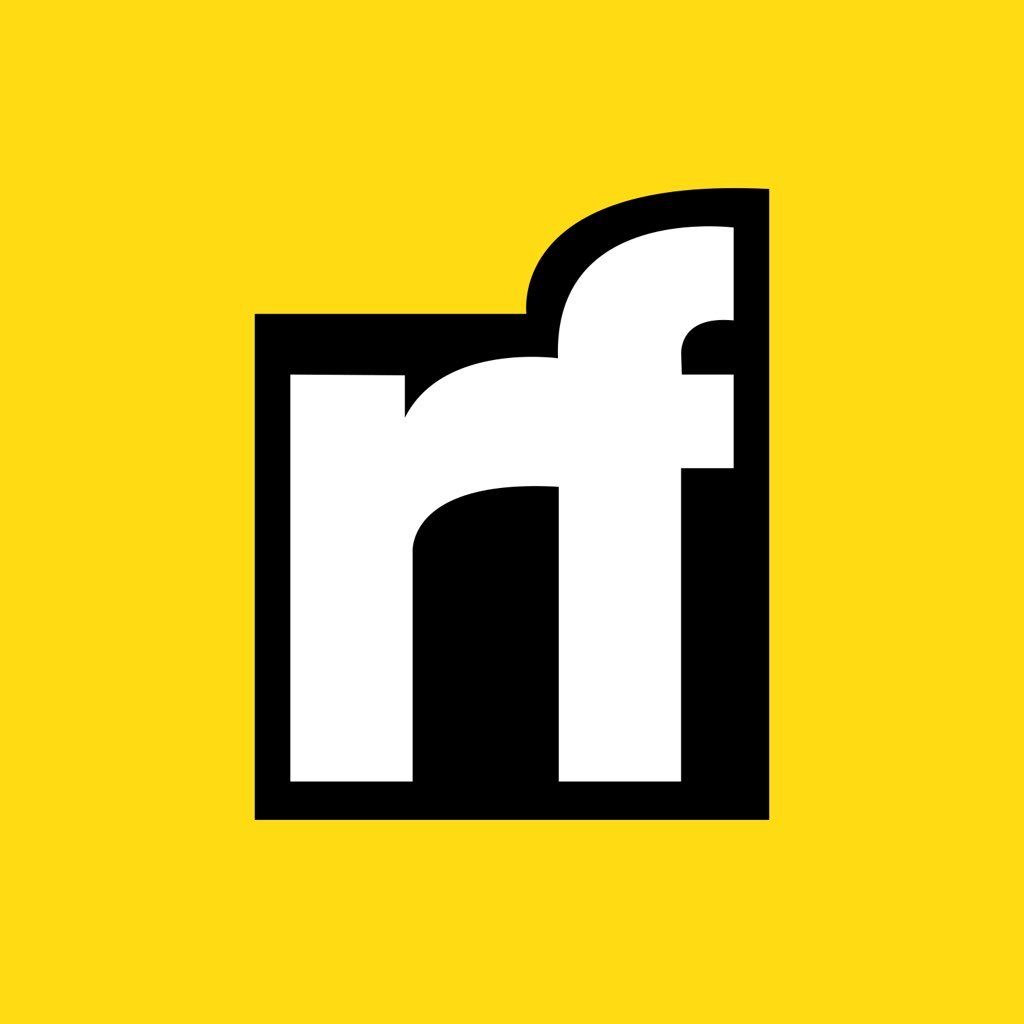
APA
MLA
Super Kai (Kazuya Ito) | Sciencx (2024-06-23T03:15:24+00:00) tile() in PyTorch. Retrieved from https://www.scien.cx/2024/06/23/tile-in-pytorch/
" » tile() in PyTorch." Super Kai (Kazuya Ito) | Sciencx - Sunday June 23, 2024, https://www.scien.cx/2024/06/23/tile-in-pytorch/
HARVARDSuper Kai (Kazuya Ito) | Sciencx Sunday June 23, 2024 » tile() in PyTorch., viewed ,<https://www.scien.cx/2024/06/23/tile-in-pytorch/>
VANCOUVERSuper Kai (Kazuya Ito) | Sciencx - » tile() in PyTorch. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2024/06/23/tile-in-pytorch/
CHICAGO" » tile() in PyTorch." Super Kai (Kazuya Ito) | Sciencx - Accessed . https://www.scien.cx/2024/06/23/tile-in-pytorch/
IEEE" » tile() in PyTorch." Super Kai (Kazuya Ito) | Sciencx [Online]. Available: https://www.scien.cx/2024/06/23/tile-in-pytorch/. [Accessed: ]
rf:citation » tile() in PyTorch | Super Kai (Kazuya Ito) | Sciencx | https://www.scien.cx/2024/06/23/tile-in-pytorch/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.