This content originally appeared on DEV Community and was authored by Ayush Sharma
Hey there, JavaScript enthusiasts! Whether you're a seasoned developer or just starting out, this blog is dedicated to sharing quick tips and tricks that can make your JavaScript coding more efficient and enjoyable. Let's dive into some handy techniques and shortcuts that will help you write cleaner, more effective code.
Let's Start,
Trick #1: Destructuring Assignment
Destructuring assignment is a powerful feature introduced in ES6 that allows you to extract values from arrays or objects into distinct variables. It's great for simplifying your code and making it more readable.
Example:
// Destructuring an array
const numbers = [1, 2, 3, 4, 5];
const [first, second] = numbers;
console.log(first); // Output: 1
console.log(second); // Output: 2
// Destructuring an object
const person = {
name: 'John',
age: 30,
city: 'New York'
};
const { name, age } = person;
console.log(name); // Output: John
console.log(age); // Output: 30
Trick #2: Spread Syntax
The spread syntax (...) is another ES6 feature that is incredibly useful for manipulating arrays and objects. It allows you to expand elements where multiple arguments or elements are expected.
Example:
// Combining arrays
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
const combined = [...arr1, ...arr2];
console.log(combined); // Output: [1, 2, 3, 4, 5, 6]
// Copying arrays
const original = [1, 2, 3];
const copy = [...original];
console.log(copy); // Output: [1, 2, 3]
// Passing arguments to a function
function sum(x, y, z) {
return x + y + z;
}
const numbers = [1, 2, 3];
console.log(sum(...numbers)); // Output: 6
Trick #3: Template Literals
Template literals provide a more convenient way to concatenate strings and include variables or expressions in your strings.
Example:
const name = 'Alice';
const age = 25;
console.log(`Hello, my name is ${name} and I am ${age} years old.`);
// Output: Hello, my name is Alice and I am 25 years old.
Trick #4: Arrow Functions
Arrow functions provide a concise syntax for writing function expressions, especially useful for inline and anonymous functions.
Example:
// Regular function
function multiply(a, b) {
return a * b;
}
// Arrow function
const multiply = (a, b) => a * b;
console.log(multiply(3, 4)); // Output: 12
Trick #5: Optional Chaining
Optional chaining (?.) allows you to safely access deeply nested properties of an object without worrying about whether the property exists or not.
Example:
const person = {
name: 'Jane',
address: {
city: 'London'
}
};
console.log(person.address?.city); // Output: London
console.log(person.address?.country); // Output: undefined (no error thrown)
Stay tuned for more JavaScript tricks and tips coming your way! Remember, mastering these shortcuts can significantly enhance your coding productivity and clarity. Happy coding! 🚀
This content originally appeared on DEV Community and was authored by Ayush Sharma
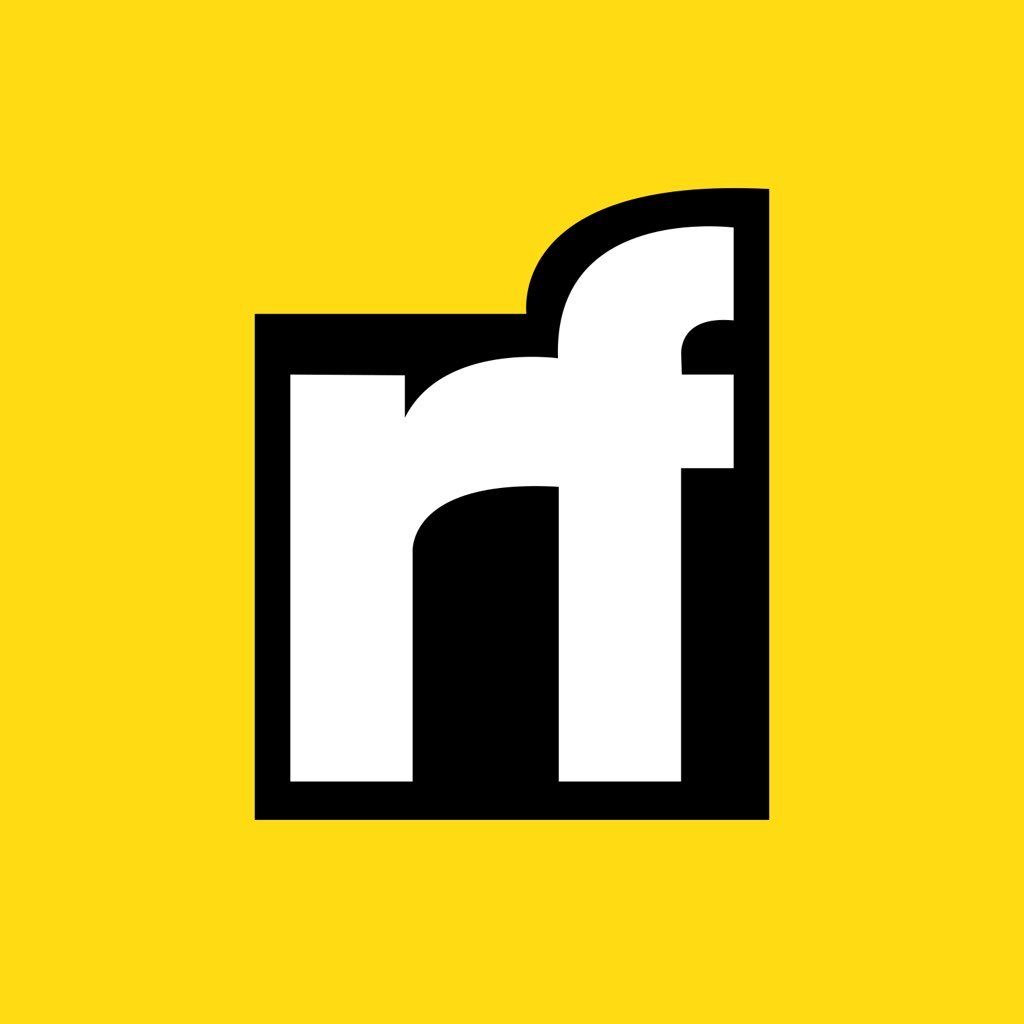
Ayush Sharma | Sciencx (2024-06-25T04:20:07+00:00) JavaScript Short Tricks Time. Retrieved from https://www.scien.cx/2024/06/25/javascript-short-tricks-time/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.