This content originally appeared on DEV Community and was authored by _Khojiakbar_
The code iterates through an array called userList
, and for each user object (el)
, it creates a card element using the createElement
function. This card contains the user's avatar, name, and email. The card is then appended to an element called servicesContent
.
// Iterate through each user object in the userList array
userList.forEach(el => {
// Create a 'card' div with user details using the createElement function
const card = createElement('div', 'card', `
<img src="${el.avatar}" alt="img" class="w-3">
<div class="p-2">
<h1>${el.last_name} ${el.first_name}</h1>
<p>${el.email}</p>
</div>
`);
// Append the created card to the servicesContent element
servicesContent.append(card);
});
Detailed Steps
-
Iterate through
userList
: The forEach method loops through each user object (el
) in theuserList
array. -
Create a Card: For each user object, a
div
element with the classcard
is created. This card contains: - An
img
element displaying the user's avatar. - A
div
with the user's name and email. -
Append the Card: The created card is appended to the
servicesContent
element.
Key Points
createElement Function:
This is used to create the card element. It takes three arguments: the tag name ('div'
), the class list ('card'
), and the inner HTML content (a template literal containing the user's details).
Template Literals: Template literals (enclosed in backticks `) allow embedding variables directly into the string using
${variable}` syntax.
Appending to the DOM: The append
method adds the created card element to the servicesContent element in the DOM.
This code dynamically creates and adds a user card to the webpage for each user in the userList array.
This content originally appeared on DEV Community and was authored by _Khojiakbar_
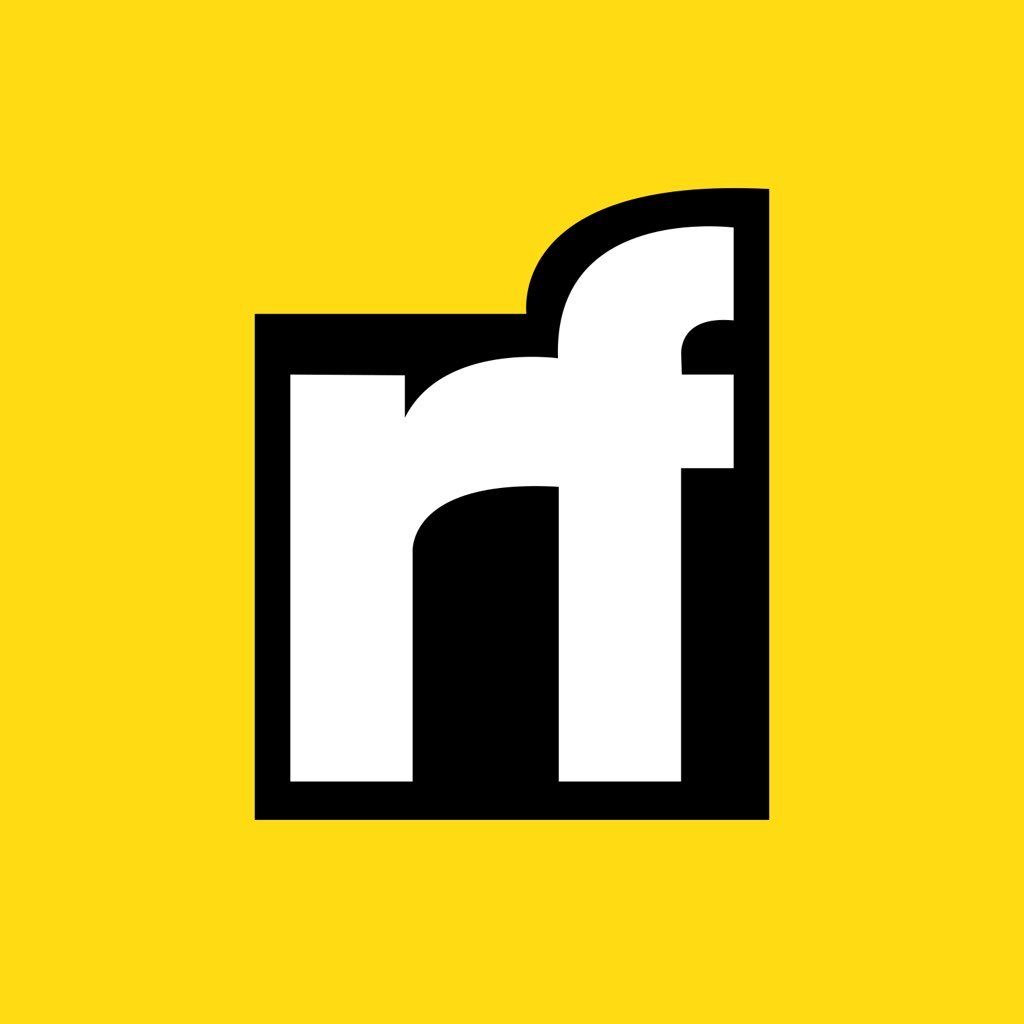
_Khojiakbar_ | Sciencx (2024-06-26T23:35:19+00:00) Dynamically Adding To The Webpage. Retrieved from https://www.scien.cx/2024/06/26/dynamically-adding-to-the-webpage/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.