This content originally appeared on DEV Community and was authored by Harsh Mishra
Python Built-in Functions and Methods Guide
Numeric Functions
abs
- Returns the absolute value of a number.
-
Syntax:
abs(number)
num = -10
abs_num = abs(num)
print(abs_num) # Output: 10
divmod
- Returns the quotient and remainder of division.
-
Syntax:
divmod(x, y)
quotient, remainder = divmod(10, 3)
print(quotient, remainder) # Output: 3 1
max
- Returns the largest item in an iterable or among arguments.
-
Syntax:
max(iterable, *args, key=None)
numbers = [1, 5, 2, 8, 3]
max_num = max(numbers)
print(max_num) # Output: 8
min
- Returns the smallest item in an iterable or among arguments.
-
Syntax:
min(iterable, *args, key=None)
numbers = [1, 5, 2, 8, 3]
min_num = min(numbers)
print(min_num) # Output: 1
pow
- Returns x to the power of y.
-
Syntax:
pow(base, exp, mod=None)
result = pow(2, 3)
print(result) # Output: 8
round
- Rounds a number to a specified precision in decimal digits.
-
Syntax:
round(number[, ndigits])
num = 3.14159
rounded_num = round(num, 2)
print(rounded_num) # Output: 3.14
sum
- Returns the sum of elements in an iterable.
-
Syntax:
sum(iterable, start=0)
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) # Output: 15
String Methods
chr
- Returns the string representing a character whose Unicode code point is the integer.
-
Syntax:
chr(i)
char = chr(65)
print(char) # Output: 'A'
format
- Formats a specified value into a specified format.
-
Syntax:
format(value, format_spec)
formatted = format(123.456, ".2f")
print(formatted) # Output: '123.46'
ord
- Returns the Unicode code point for a given character.
-
Syntax:
ord(c)
unicode_value = ord('A')
print(unicode_value) # Output: 65
str
- Returns a string version of an object.
-
Syntax:
str(object='')
num_str = str(123)
print(num_str) # Output: '123'
Container Methods
dict
- Creates a new dictionary.
-
Syntax:
dict(**kwargs)
ordict(iterable, **kwargs)
my_dict = dict(name='John', age=30)
print(my_dict) # Output: {'name': 'John', 'age': 30}
frozenset
- Returns an immutable frozenset object.
-
Syntax:
frozenset([iterable])
my_frozenset = frozenset({1, 2, 3})
print(my_frozenset) # Output: frozenset({1, 2, 3})
list
- Returns a list object.
-
Syntax:
list(iterable=())
my_list = list(range(5))
print(my_list) # Output: [0, 1, 2, 3, 4]
set
- Returns a new set object.
-
Syntax:
set([iterable])
my_set = set([1, 2, 3])
print(my_set) # Output: {1, 2, 3}
tuple
- Returns a tuple object.
-
Syntax:
tuple([iterable])
my_tuple = tuple([1, 2, 3])
print(my_tuple) # Output: (1, 2, 3)
Type Conversion Functions
bool
- Returns the boolean value of an object.
-
Syntax:
bool([value])
bool_value = bool(0)
print(bool_value) # Output: False
float
- Returns a floating-point number from a number or string.
-
Syntax:
float([x])
float_num = float("3.14")
print(float_num) # Output: 3.14
hex
- Converts an integer to a lowercase hexadecimal string prefixed with '0x'.
-
Syntax:
hex(x)
hex_value = hex(255)
print(hex_value) # Output: '0xff'
int
- Returns an integer object from a number or string.
-
Syntax:
int([x])
int_num = int("123")
print(int_num) # Output: 123
oct
- Converts an integer to an octal string prefixed with '0o'.
-
Syntax:
oct(x)
oct_value = oct(8)
print(oct_value) # Output: '0o10'
Other Functions
all
- Returns True if all elements of an iterable are true.
-
Syntax:
all(iterable)
items = [True, True, False]
print(all(items)) # Output: False
any
- Returns True if any element of an iterable is true.
-
Syntax:
any(iterable)
items = [False, False, True]
print(any(items)) # Output: True
bin
- Converts an integer to a binary string prefixed with '0b'.
-
Syntax:
bin(x)
bin_value = bin(5)
print(bin_value) # Output: '0b101'
enumerate
- Returns an enumerate object that yields tuples of index and value.
-
Syntax:
enumerate(iterable, start=0)
letters = ['a', 'b', 'c']
for index, value in enumerate(letters):
print(index, value)
# Output:
# 0 a
# 1 b
# 2 c
filter
- Constructs an iterator from elements of an iterable for which a function returns true.
-
Syntax:
filter(function, iterable)
def is_even(num):
return num % 2 == 0
numbers = [1, 2, 3, 4, 5]
even_numbers = list(filter(is_even, numbers))
print(even_numbers) # Output: [2, 4]
map
- Applies a function to all items in an input iterable.
-
Syntax:
map(function, iterable, ...)
def square(x):
return x ** 2
numbers = [1, 2, 3, 4, 5]
squared_numbers = list(map(square, numbers))
print(squared_numbers) # Output: [1, 4, 9, 16, 25]
sorted
- Returns a new sorted list from the elements of any iterable.
-
Syntax:
sorted(iterable, *, key=None, reverse=False)
numbers = [5, 2, 3, 1, 4]
sorted_numbers = sorted(numbers)
print(sorted_numbers) # Output: [1, 2, 3, 4, 5]
super
- Returns a proxy object that delegates method calls to a parent or sibling class.
-
Syntax:
super([type[, object-or-type]])
class Parent:
def __init__(self, name):
self.name = name
class Child(Parent):
def __init__(self, name, age):
super().__init__(name)
self.age = age
child = Child("John", 10)
print(child.name, child.age) # Output: John 10
Input and Output Functions
input
- Reads a line from input, converts it to a string (stripping a trailing newline), and returns it.
-
Syntax:
input(prompt)
name = input("Enter your name: ")
print("Hello, " + name + "!")
print
- Prints objects to the text stream file, separated by sep and followed by end.
-
Syntax:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
print("Hello", "World", sep=", ", end="!\n")
# Output: Hello, World!
This content originally appeared on DEV Community and was authored by Harsh Mishra
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
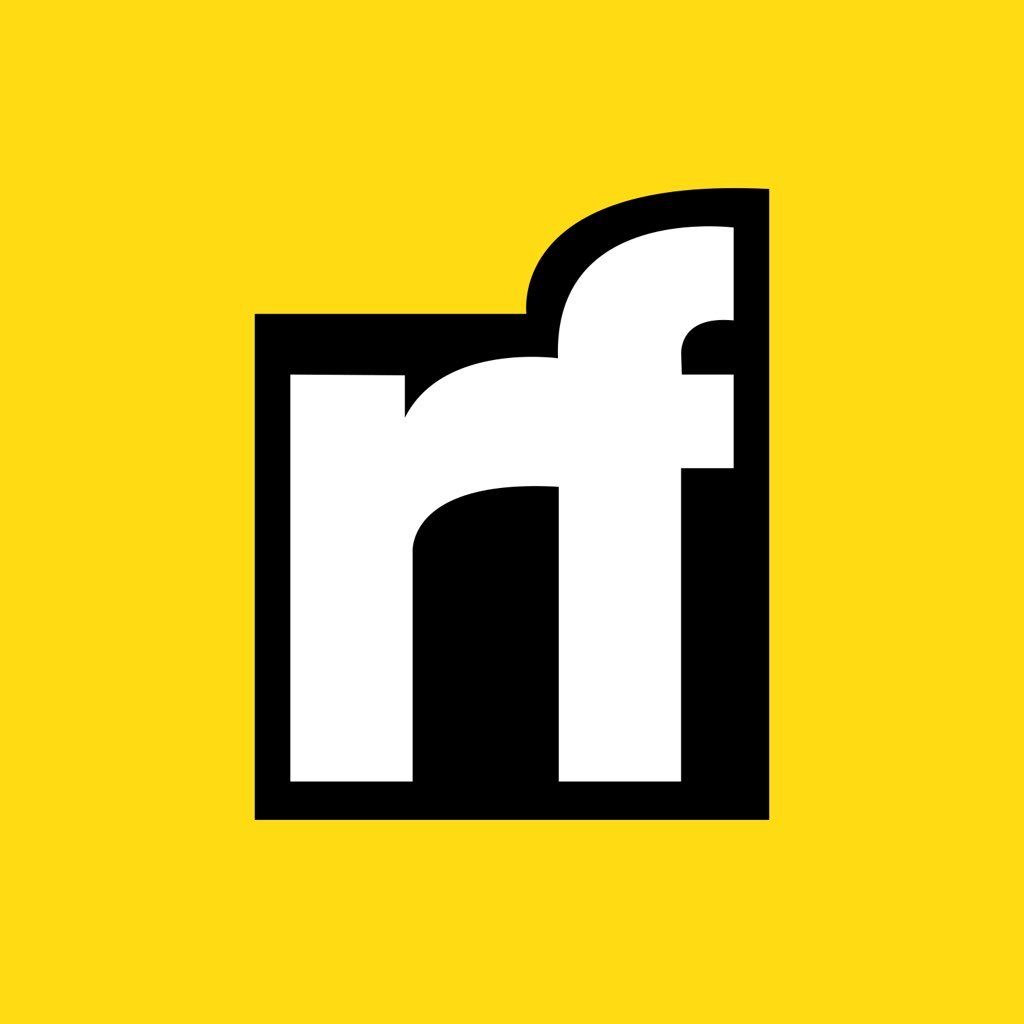
APA
MLA
Harsh Mishra | Sciencx (2024-06-27T11:59:54+00:00) Python Built-in Functions. Retrieved from https://www.scien.cx/2024/06/27/python-built-in-functions/
" » Python Built-in Functions." Harsh Mishra | Sciencx - Thursday June 27, 2024, https://www.scien.cx/2024/06/27/python-built-in-functions/
HARVARDHarsh Mishra | Sciencx Thursday June 27, 2024 » Python Built-in Functions., viewed ,<https://www.scien.cx/2024/06/27/python-built-in-functions/>
VANCOUVERHarsh Mishra | Sciencx - » Python Built-in Functions. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2024/06/27/python-built-in-functions/
CHICAGO" » Python Built-in Functions." Harsh Mishra | Sciencx - Accessed . https://www.scien.cx/2024/06/27/python-built-in-functions/
IEEE" » Python Built-in Functions." Harsh Mishra | Sciencx [Online]. Available: https://www.scien.cx/2024/06/27/python-built-in-functions/. [Accessed: ]
rf:citation » Python Built-in Functions | Harsh Mishra | Sciencx | https://www.scien.cx/2024/06/27/python-built-in-functions/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.