This content originally appeared on DEV Community and was authored by MD ARIFUL HAQUE
350. Intersection of Two Arrays II
Easy
Given two integer arrays nums1
and nums2
, return an array of their intersection. Each element in the result must appear as many times as it shows in both arrays, and you may return the result in any order.
Example 1:
- Input: nums1 = [1,2,2,1], nums2 = [2,2]
- Output: [2,2]
Example 2:
- Input: nums1 = [4,9,5], nums2 = [9,4,9,8,4]
- Output: [4,9]
- Explanation: [9,4] is also accepted.
Constraints:
1 <= nums1.length, nums2.length <= 1000
0 <= nums1[i], nums2[i] <= 1000
Solution:
class Solution {
/**
* @param Integer[] $nums1
* @param Integer[] $nums2
* @return Integer[]
*/
function intersect($nums1, $nums2) {
// Count occurrences of each element in both arrays
$counts1 = array_count_values($nums1);
$counts2 = array_count_values($nums2);
$intersection = [];
// Iterate through the first count array
foreach ($counts1 as $num => $count) {
// Check if the element exists in the second array
if (isset($counts2[$num])) {
// Find the minimum occurrence
$minCount = min($count, $counts2[$num]);
// Add the element to the result array, repeated $minCount times
for ($i = 0; $i < $minCount; $i++) {
$intersection[] = $num;
}
}
}
return $intersection;
}
}
Contact Links
This content originally appeared on DEV Community and was authored by MD ARIFUL HAQUE
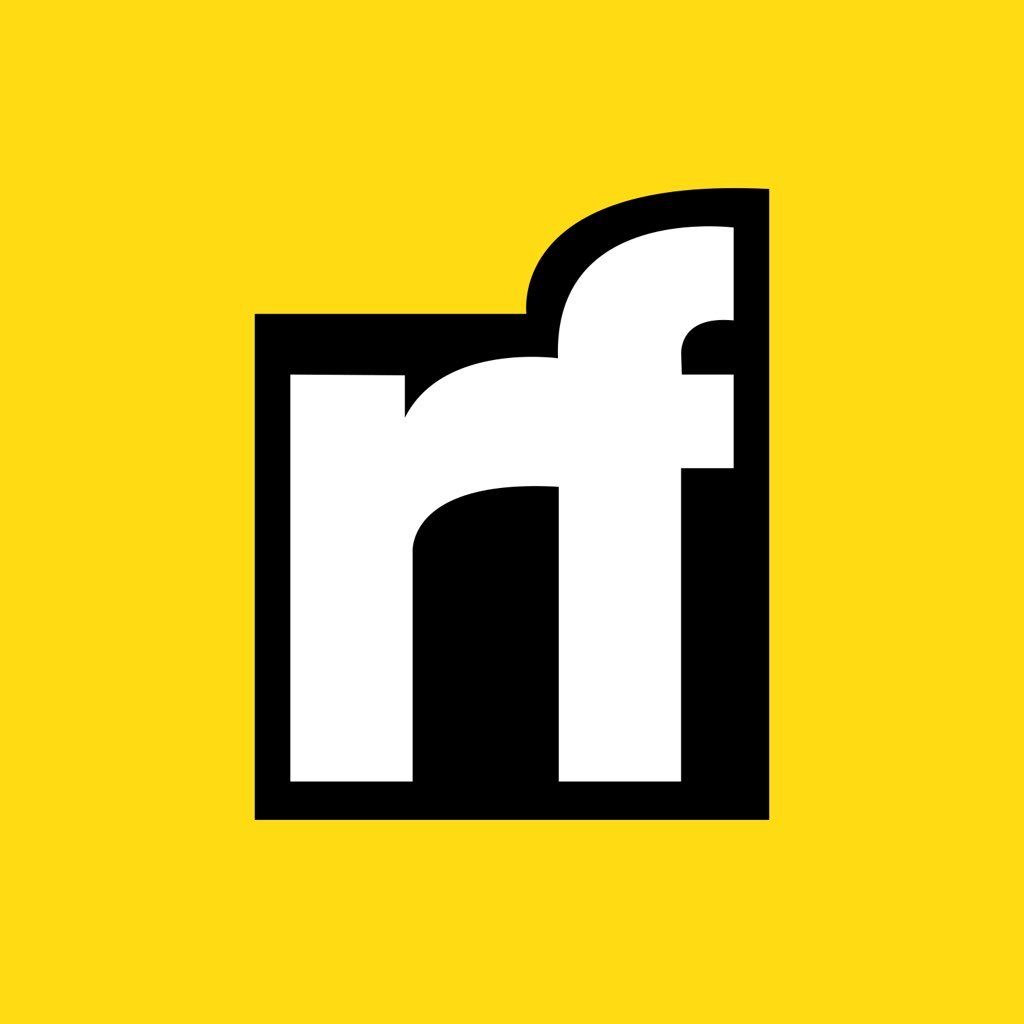
MD ARIFUL HAQUE | Sciencx (2024-07-02T15:48:03+00:00) 350. Intersection of Two Arrays II. Retrieved from https://www.scien.cx/2024/07/02/350-intersection-of-two-arrays-ii-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.