This content originally appeared on Level Up Coding - Medium and was authored by Pius Oruko
When it comes to biometric security, facial authentication is a powerful tool, but it must be fortified against spoofing attempts to ensure its reliability. Liveness detection, which verifies that the face being scanned is from a live person rather than a photograph or video, is crucial. In this article, we’ll explore how to implement liveness detection and facial authentication in JavaScript using FACEIO.
What is Liveness Detection?
Liveness detection is a security feature used in facial recognition systems to determine if the face being scanned is that of a live person. It helps prevent spoofing attacks, such as using photographs, videos, or masks to fool the system. Various techniques are used for liveness detection, including:
Motion Analysis: Detects natural movements like blinking and head movements.
Texture Analysis: Differentiates between the texture of live skin and that of a photograph or screen.
Challenge-Response: Prompts the user to perform actions, like blinking or smiling, to prove liveness.
Implementing Liveness Detection with FACEIO
FACEIO provides an easy-to-integrate solution for facial authentication with built-in liveness detection. Below is a step-by-step guide to get started.

Step 1: Setting Up FACEIO
First, you need to sign up and create an account on the FACEIO Console. After creating an account, obtain your API key, which will be used to authenticate your requests.
Step 2: Integrating FACEIO SDK
Integrate the FACEIO JavaScript SDK into your project. Include the following script in your HTML file:
<script src="https://cdn.faceio.net/fio.js"></script>
Step 3: Initializing FACEIO
Initialize the FACEIO instance in your JavaScript code:
const faceio = new FACEIO("YOUR_API_KEY");
Replace YOUR_API_KEY with the API key obtained from the FACEIO console.
Step 4: Implementing liveness detection using Javascript
FACEIO’s liveness detection is built into its facial recognition workflow. Here’s how you can implement it:
- Triggering the Liveness Check: When you want to perform a liveness check, prompt the user to start the facial recognition process.
async function performLivenessCheck() {
try {
const response = await faceio.authenticate({
"payload": {
"action": "livenessCheck"
}
});
console.log('Liveness check successful:', response);
} catch (error) {
console.error('Liveness check failed:', error);
}
}
The “performLivenessCheck” function in JavaScript uses the FACEIO library to authenticate users by checking for liveness. It asynchronously calls “faceio.authenticate” with a liveness check payload and logs the response if successful, or an error message if it fails.
2. Handling the Response: The response will include a confidence score indicating the likelihood that the scanned face is live. Based on this score, you can take appropriate actions.
if (response.livenessScore > 0.9) {
// User is live
console.log('User authenticated successfully');
} else {
// Possible spoofing attempt
console.warn('Liveness check failed');
}
“response.livenessScore > 0.9”: This line checks if the liveness score from the FACEIO response is greater than 0.9. A high score indicates a high confidence that the user is live.
“Successful Authentication”: If the liveness score is greater than 0.9, a message is logged indicating that the user is authenticated successfully.
“Spoofing Attempt”: If the liveness score is 0.9 or less, a warning is logged indicating a possible spoofing attempt.
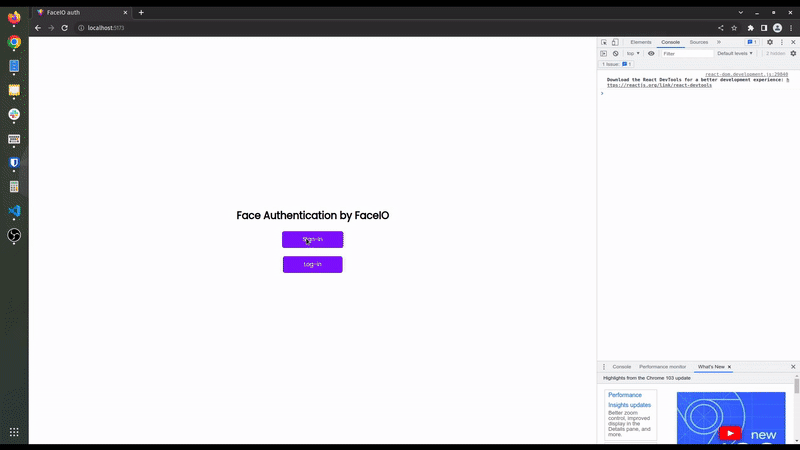
Sample Application
Below is a simple example of a web page that uses FACEIO to perform liveness detection and facial authentication:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Liveness Detection with FACEIO</title>
<script src="https://cdn.faceio.net/faceio.js"></script>
</head>
<body>
<h1>Liveness Detection with FACEIO</h1>
<button onclick="performLivenessCheck()">Start Liveness Check</button>
<script>
const faceio = new FACEIO("your-api-key");
async function performLivenessCheck() {
try {
const response = await faceio.authenticate({
"payload": {
"action": "livenessCheck"
}
});
if (response.livenessScore > 0.9) {
console.log('User authenticated successfully');
} else {
console.warn('Liveness check failed');
}
} catch (error) {
console.error('Liveness check failed:', error);
}
}
</script>
</body>
</html>
Advanced Features
FACEIO offers several advanced features to enhance your application’s security and user experience:
Multi-Factor Authentication (MFA): Combine facial recognition with other authentication methods for added security.
Customizable User Prompts: Customize the instructions and prompts shown to users during the liveness check to improve usability and compliance.
Analytics and Reporting: Access detailed reports and analytics on authentication attempts, helping you monitor and improve your system’s performance.
Conclusion
In summary, integrating FACEIO for liveness detection and facial authentication in JavaScript enhances digital security significantly. With its robust API and user-friendly JavaScript library, developers can easily implement biometric authentication to prevent spoofing and unauthorized access. FACEIO offers advanced features like multi-factor authentication, customizable prompts, and detailed analytics, ensuring both security and user experience are prioritized. Whether for financial services, healthcare, or e-commerce, FACEIO provides a scalable solution to reinforce application security effectively. By choosing FACEIO, developers can confidently protect user data and meet stringent security standards, making it a valuable addition to any web application’s defense against modern threats.
Liveness Detection and Facial Authentication in JavaScript with FACEIO was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Pius Oruko
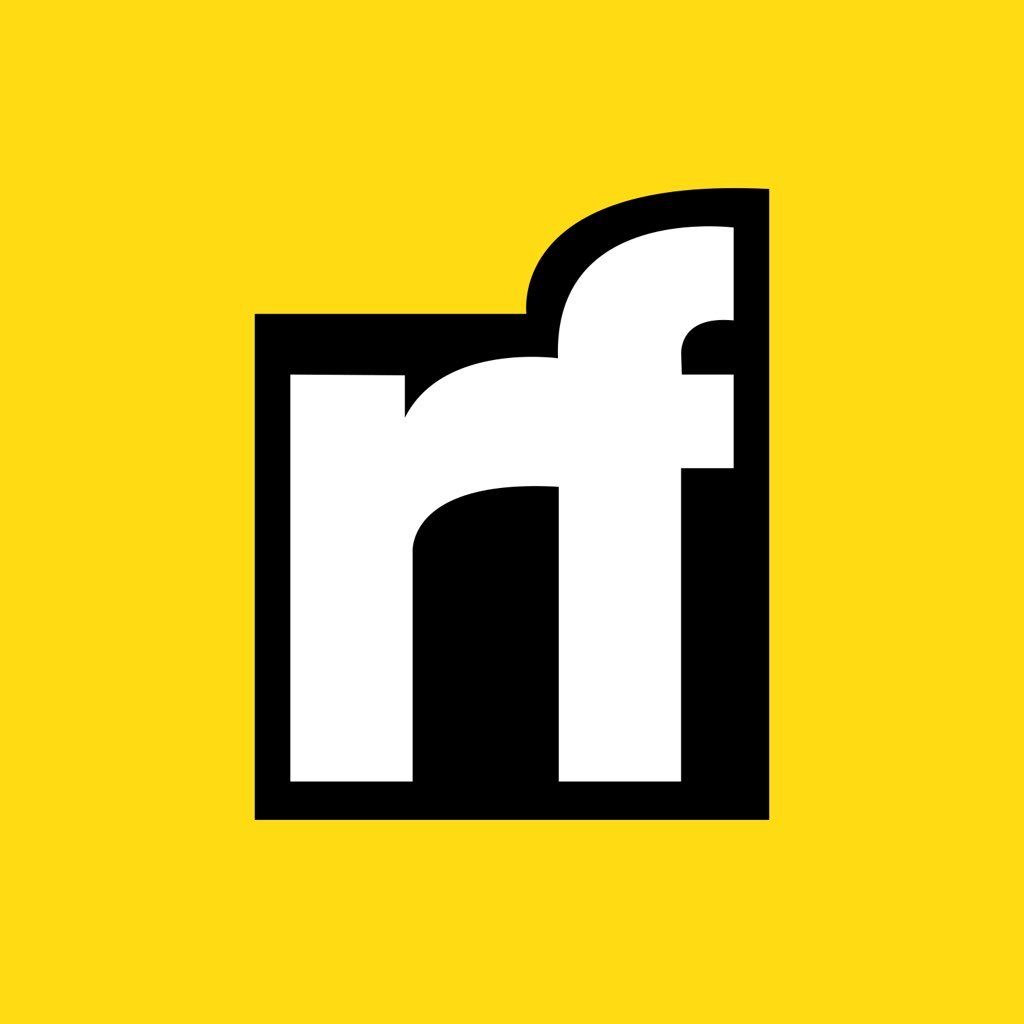
Pius Oruko | Sciencx (2024-07-02T22:54:59+00:00) Liveness Detection and Facial Authentication in JavaScript with FACEIO. Retrieved from https://www.scien.cx/2024/07/02/liveness-detection-and-facial-authentication-in-javascript-with-faceio/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.