This content originally appeared on DEV Community and was authored by Super Kai (Kazuya Ito)
*Memos:
- My post explains argmin() and argmax().
- My post explains aminmax(), amin() and amax().
- My post explains minimum(), maximum(). fmin() and fmax().
- My post explains kthvalue() and topk().
min() can get the 0D of the 1st one minimum element or the 0D or more D tensor of the 1st zero or more minimum elements and their indices from the one or two 0D or more D tensors of zero or more elements as shown below:
*Memos:
-
min()
can be used with torch or a tensor. - The 1st argument with
torch
or using a tensor isinput
(Required-Type:tensor
ofint
,float
orbool
). - The 2nd argument with
torch
or the 1st argument isdim
(Optional-Type:int
). *Settingdim
can get zero or more 1st minimum elements and their indices. - The 2nd argument with
torch
or the 1st argument isother
(Optional-Type:tensor
ofint
,float
orbool
). *Memos:- It can only be used with
input
. - This is the functionality of minimum().
- It can only be used with
- The 3rd argument with
torch
or the 2nd argument iskeepdim
(Optional-Type:bool
). *Memos:- It must be used with
dim
withoutother
. -
My post explains
keepdim
argument.
- It must be used with
- There is
out
argument withtorch
(Optional-Type:tensor
ortuple
(tensor
,tensor
): *Memos:- The type of
tensor
must be used withoutdim
andkeepdim
. - The type of
tuple
(tensor
,tensor
) must be used withdim
withoutother
. -
out=
must be used. -
My post explains
out
argument.
- The type of
- Empty 2D or more D
input
tensor withoutother
tensor doesn't work if not settingdim
. - Empty 1D
input
tesnor withoutother
tensor doesn't work even if settingdim
.
import torch
my_tensor = torch.tensor([[5, 4, 7, 7],
[6, 5, 3, 5],
[3, 8, 9, 3]])
torch.min(input=my_tensor)
my_tensor.min()
# tensor(3)
torch.min(input=my_tensor, dim=0)
torch.min(input=my_tensor, dim=-2)
# torch.return_types.min(
# values=tensor([3, 4, 3, 3]),
# indices=tensor([2, 0, 1, 2]))
torch.min(input=my_tensor, dim=1)
torch.min(input=my_tensor, dim=-1)
# torch.return_types.min(
# values=tensor([4, 3, 3]),
# indices=tensor([1, 2, 0]))
tensor1 = torch.tensor([5, 4, 7, 7])
tensor2 = torch.tensor([[6, 5, 3, 5],
[3, 8, 9, 3]])
torch.min(input=tensor1, other=tensor2)
# tensor([[5, 4, 3, 5],
# [3, 4, 7, 3]])
tensor1 = torch.tensor([5., 4., 7., 7.])
tensor2 = torch.tensor([[6., 5., 3., 5.],
[3., 8., 9., 3.]])
torch.min(input=tensor1, other=tensor2)
# tensor([[5., 4., 3., 5.],
# [3., 4., 7., 3.]])
tensor1 = torch.tensor([True, False, True, False])
tensor2 = torch.tensor([[True, False, True, False],
[False, True, False, True]])
torch.min(input=tensor1, other=tensor2)
# tensor([[True, False, True, False],
# [False, False, False, False]])
my_tensor = torch.tensor([])
my_tensor = torch.tensor([[]])
my_tensor = torch.tensor([[[]]])
torch.min(input=my_tensor) # Error
my_tensor = torch.tensor([])
torch.min(input=my_tensor, dim=0) # Error
my_tensor = torch.tensor([[]])
torch.min(input=my_tensor, dim=0)
# torch.return_types.min(
# values=tensor([]),
# indices=tensor([], dtype=torch.int64))
my_tensor = torch.tensor([[[]]])
torch.min(input=my_tensor, dim=0)
# torch.return_types.min(
# values=tensor([], size=(1, 0)),
# indices=tensor([], size=(1, 0), dtype=torch.int64))
max() can get the 0D of the 1st one maximum element or the 0D or more D tensor of the 1st zero or more maximum elements and their indices from the one or two 0D or more D tensors of zero or more elements as shown below:
-
max()
can be used withtorch
or a tensor. - The 1st argument with
torch
or using a tensor isinput
(Required-Type:tensor
ofint
,float
orbool
). - The 2nd argument with
torch
or the 1st argument isdim
(Optional-Type:int
). *Settingdim
can get zero or more 1st maximum elements and their indices. - The 2nd argument with
torch
or the 1st argument isother
(Optional-Type:tensor
ofint
,float
orbool
). *Memos:- It can only be used with
input
. - This is the functionality of maximum().
- It can only be used with
- The 3rd argument with
torch
or the 2nd argument iskeepdim
(Optional-Type:bool
). *Memos:- It must be used with
dim
withoutother
. -
My post explains
keepdim
argument.
- It must be used with
- There is
out
argument withtorch
(Optional-Type:tensor
ortuple
(tensor
,tensor
): *Memos:- The type of
tensor
must be used withoutdim
andkeepdim
. - The type of
tuple
(tensor
,tensor
) must be used withdim
withoutother
. -
out=
must be used. -
My post explains
out
argument.
- The type of
- Empty 2D or more D
input
tensor withoutother
tensor doesn't work if not settingdim
. - Empty 1D
input
tesnor withoutother
tensor doesn't work even if settingdim
.
import torch
my_tensor = torch.tensor([[5, 4, 7, 7],
[6, 5, 3, 5],
[3, 8, 9, 3]])
torch.max(input=my_tensor)
my_tensor.max()
# tensor(9)
torch.max(input=my_tensor, dim=0)
torch.max(input=my_tensor, dim=-2)
# torch.return_types.max(
# values=tensor([6, 8, 9, 7]),
# indices=tensor([1, 2, 2, 0]))
torch.max(input=my_tensor, dim=1)
torch.max(input=my_tensor, dim=-1)
# torch.return_types.max(
# values=tensor([7, 6, 9]),
# indices=tensor([2, 0, 2]))
tensor1 = torch.tensor([5, 4, 7, 7])
tensor2 = torch.tensor([[6, 5, 3, 5],
[3, 8, 9, 3]])
torch.max(input=tensor1, other=tensor2)
# tensor([[6, 5, 7, 7],
# [5, 8, 9, 7]])
tensor1 = torch.tensor([5., 4., 7., 7.])
tensor2 = torch.tensor([[6., 5., 3., 5.],
[3., 8., 9., 3.]])
torch.max(input=tensor1, other=tensor2)
# tensor([[6., 5., 7., 7.],
# [5., 8., 9., 7.]])
tensor1 = torch.tensor([True, False, True, False])
tensor2 = torch.tensor([[True, False, True, False],
[False, True, False, True]])
torch.max(input=tensor1, other=tensor2)
# tensor([[True, False, True, False],
# [True, True, True, True]])
my_tensor = torch.tensor([])
my_tensor = torch.tensor([[]])
my_tensor = torch.tensor([[[]]])
torch.max(input=my_tensor) # Error
my_tensor = torch.tensor([])
torch.max(input=my_tensor, dim=0) # Error
my_tensor = torch.tensor([[]])
torch.max(input=my_tensor, dim=0)
# torch.return_types.max(
# values=tensor([]),
# indices=tensor([], dtype=torch.int64))
my_tensor = torch.tensor([[[]]])
torch.max(input=my_tensor, dim=0)
# torch.return_types.max(
# values=tensor([], size=(1, 0)),
# indices=tensor([], size=(1, 0), dtype=torch.int64))
This content originally appeared on DEV Community and was authored by Super Kai (Kazuya Ito)
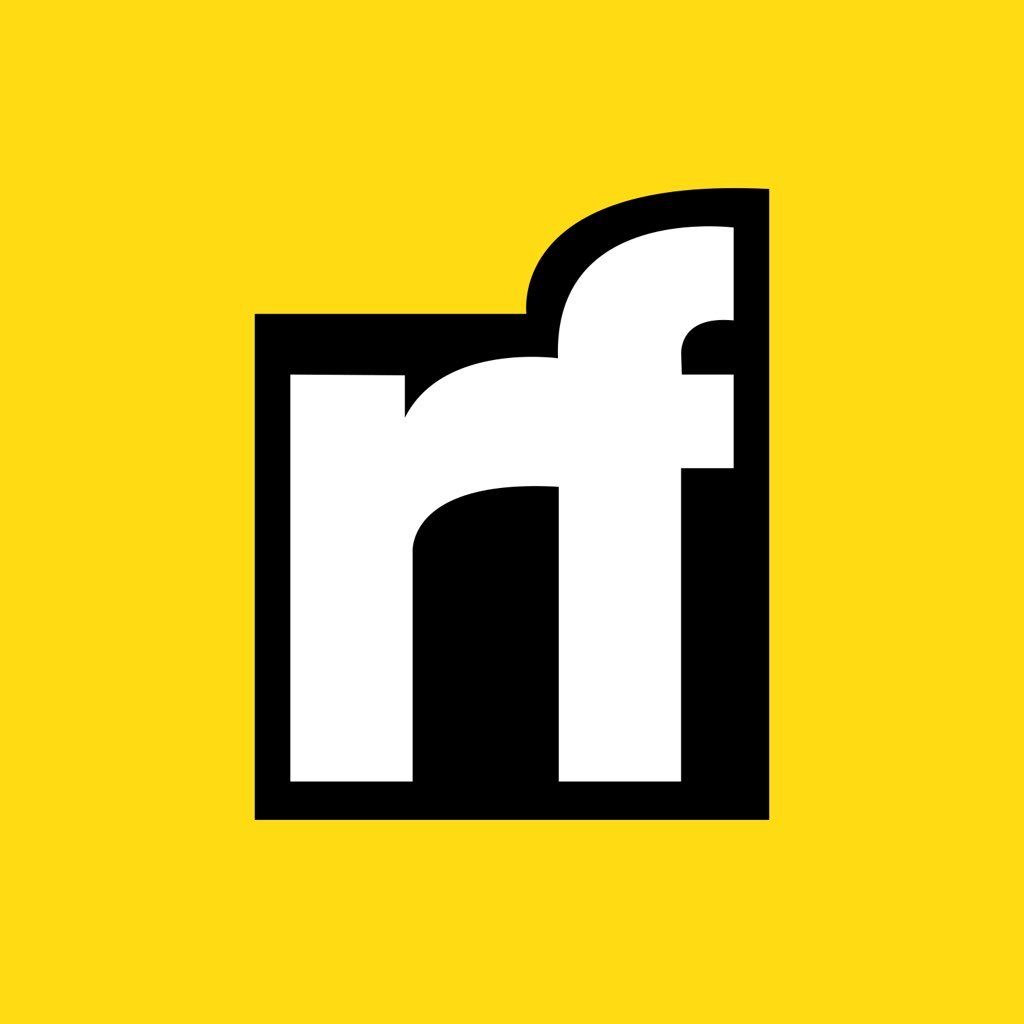
Super Kai (Kazuya Ito) | Sciencx (2024-07-04T17:25:11+00:00) min() and max() in PyTorch. Retrieved from https://www.scien.cx/2024/07/04/min-and-max-in-pytorch/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.