This content originally appeared on DEV Community and was authored by Manish Thakurani
New Features in Java 8
Java 8 introduced several significant features:
- Lambda Expressions
- Syntax: Lambda expressions enable functional programming.
- Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println(name));
- Stream API
- Functional Operations: Stream API facilitates processing collections with functional-style operations.
- Example:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
long count = names.stream().filter(name -> name.startsWith("A")).count();
- Date and Time API
- Improved API: Provides a comprehensive set of classes for date and time manipulation.
- Example:
LocalDate today = LocalDate.now();
LocalDateTime dateTime = LocalDateTime.of(today, LocalTime.of(14, 30));
- Default Methods in Interfaces
- Interface Evolution: Allows adding methods to interfaces without breaking existing implementations.
- Example:
public interface MyInterface {
default void defaultMethod() {
System.out.println("Default method implementation");
}
}
- Optional Class
- Null Handling: Encourages explicit handling of nullable values to avoid
NullPointerException\
. - Example:
- Null Handling: Encourages explicit handling of nullable values to avoid
Optional<String> optionalName = Optional.ofNullable(name);
optionalName.ifPresent(System.out::println);
Java 8's features promote cleaner code, improved performance, and better support for modern programming paradigms.
This content originally appeared on DEV Community and was authored by Manish Thakurani
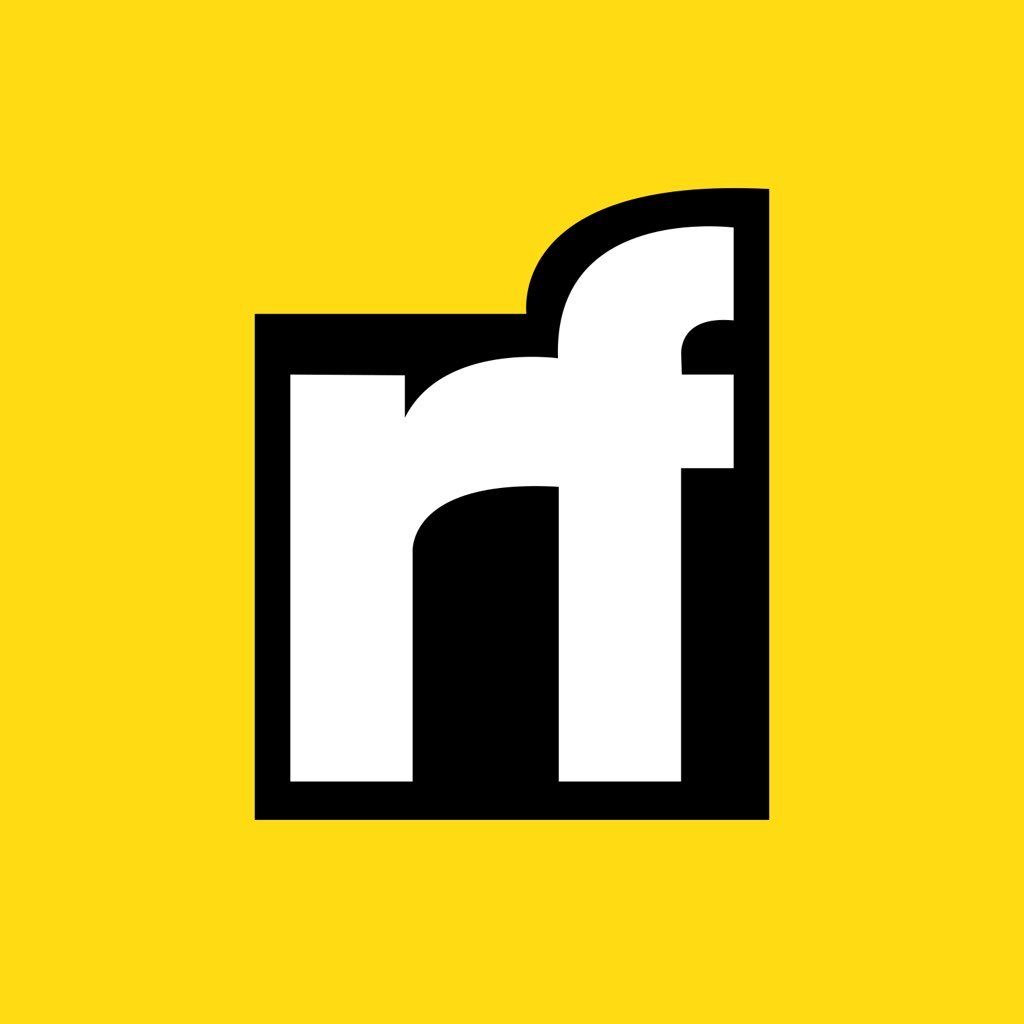
Manish Thakurani | Sciencx (2024-07-04T14:46:55+00:00) What are new features of Java 8 #interviewQuestion. Retrieved from https://www.scien.cx/2024/07/04/what-are-new-features-of-java-8-interviewquestion/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.