This content originally appeared on DEV Community and was authored by Urunna Glad Erondu
Creating users and groups manually particularly in a large environment can be time consuming and tedious. Automating this task with a bash script saves time and errors.
This script create_users.sh
streamline the process, automates the creation of users and groups, sets up home directories, generates random passwords, and logs all actions. This article explains each step of the script and provides insights into its implementation.
Script Overview
The script reads a input file containing usernames and group names, creates users and groups as specified, sets up home directories with appropriate permissions and ownership, generates random passwords, and logs all actions. Additionally, it stores the generated passwords securely.
Prerequisites
- Basic understanding of Linux CLI and Bash Scripting.
-
Ensure the script is run as root: Root privileges are necessary for creating users and groups. The script should be run using
sudo
. -
Provide an input file: The input file should contain usernames and group names separated by semicolons (
;
). Multiple group names should be comma-separated. Each user should be on a new line. For example:
glad;admin,devops
urunna;sysops
micah;architect,user
Step-by-Step Explanation:
1. Checking Root Privileges
The script start by checking if it is run with root privileges. If not, it exits with an error message.
if [ "$(id -u)" -ne 0 ]; then
echo "This script must be run as root" >&2
exit 1
fi
2. Validating Input File
The script checks if an input file is provided and if it exists. If not, it exits with an error message.
USER_LIST=$1
if [ -z "$USER_LIST" ]; then
echo "Usage: $0 <input_file>" >&2
exit 1
fi
if [ ! -f "$USER_LIST" ]; then
echo "Error: file not found: $USER_LIST" >&2
exit 1
fi
3. Setting Up Log and Password Files
The script checks for the existence of the log and password files and creates them if they do not exist. It also sets appropriate permissions for the password file to ensure security.
LOG_FILE="/var/log/user_management.log"
if [ ! -f "$LOG_FILE" ]; then
sudo touch "$LOG_FILE"
echo "$LOG_FILE has been created."
else
echo "Skipping creation of: $LOG_FILE (Already exists)"
fi
PASSWORD_FILE="/var/secure/user_passwords.txt"
if [ ! -d /var/secure ]; then
sudo mkdir -p /var/secure
sudo touch "$PASSWORD_FILE"
sudo chmod 700 /var/secure
fi
4. Generating a Random Password
This function generates a random password using OpenSSL.
generate_password() {
openssl rand -base64 12
}
5. Processing the Input File
This section reads the input file line by line, processes each username and group, creates the necessary groups, and adds the user to the specified groups. It also generates a random password for each user, sets up their home directory, and logs all actions.
while IFS=';' read -r username groups; do
username=$(echo "$username" | xargs)
groups=$(echo "$groups" | xargs)
echo "Processing user: $username" | tee -a $LOG_FILE
if ! getent group "$username" > /dev/null 2>&1; then
sudo groupadd "$username"
echo "Group $username created" | tee -a $LOG_FILE
else
echo "Group $username already exists" | tee -a $LOG_FILE
fi
group_array=()
for group in $(echo "$groups" | tr ',' ' '); do
group=$(echo "$group" | xargs)
if getent group "$group" > /dev/null 2>&1; then
group_array+=("$group")
else
echo "Invalid group name: $group" | tee -a $LOG_FILE
fi
done
more_groups=$(IFS=','; echo "${group_array[*]}")
if ! id -u "$username" > /dev/null 2>&1; then
sudo useradd -m -g "$username" -G "$more_groups" "$username" &>/dev/null
if [[ $? -eq 0 ]]; then
echo "User $username created and added to groups: $more_groups" | tee -a $LOG_FILE
password=$(generate_password)
echo "$username:$password" | sudo chpasswd
if [[ $? -eq 0 ]]; then
echo "Password for $username set" | tee -a $LOG_FILE
echo "$username,$password" >> $PASSWORD_FILE
else
echo "Failed to set password for user $username" | tee -a $LOG_FILE
fi
sudo chown "$username:$username" "/home/$username"
sudo chmod 700 "/home/$username"
echo "Home directory permissions set for $username" | tee -a $LOG_FILE
else
echo "Failed to create user $username" | tee -a $LOG_FILE
fi
else
echo "User $username already exists" | tee -a $LOG_FILE
fi
done < "$USER_LIST"
echo "User creation process completed. Logs can be found at $LOG_FILE.
Making the Script Executable
Before running the script, make sure it is executable. You can do this with the following command:
chmod +x create_users.sh
Running the Script
Now you can run the script with sudo
:
sudo ./create_users.sh users.txt
This will ensure that the script reads from the provided user.txt
file and performs the necessary user and group management tasks, logging actions to /var/log/user_management.log
and storing passwords in /var/secure/user_passwords.txt.
This article is part of my participation task in HNG internship
For more information and to join this exciting program, check out the HNG Internship, visit HNG Internship website or learn how to hire top talent from HNG
This content originally appeared on DEV Community and was authored by Urunna Glad Erondu
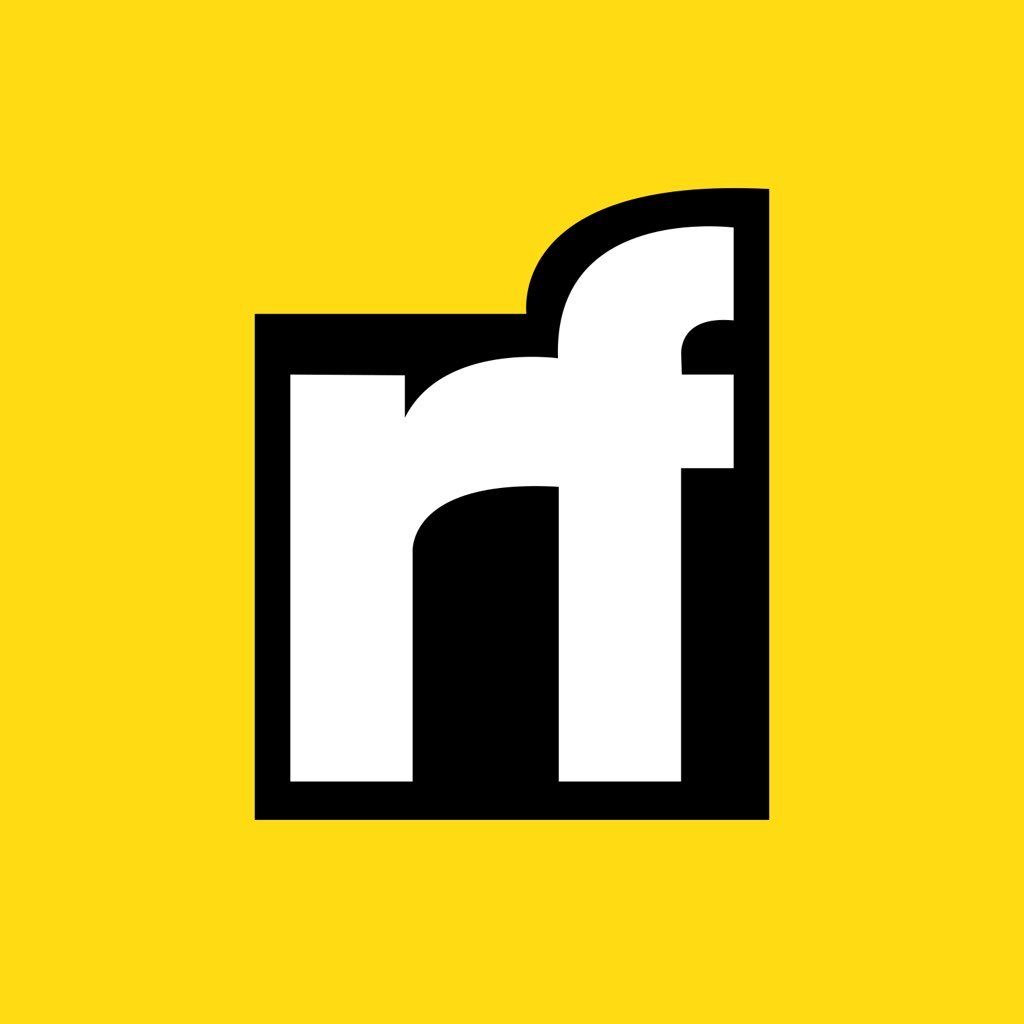
Urunna Glad Erondu | Sciencx (2024-07-06T08:14:46+00:00) Automating User and Group Management using Bash Script. Retrieved from https://www.scien.cx/2024/07/06/automating-user-and-group-management-using-bash-script-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.