This content originally appeared on Level Up Coding - Medium and was authored by Martin Muller
In software development, ensuring that objects are copied correctly is critical for maintaining the integrity of your application. Many projects encounter bugs and unintended side effects because developers often overlook the differences between shallow and deep copies. This article delves into these differences, highlighting the drawbacks of shallow copies and the benefits of deep copies. We’ll explore techniques to create robust deep copies using copy constructors, comparing these methods in C# and Java. Whether you’re a beginner or a seasoned developer, understanding these concepts is essential for writing reliable and extensible code.
Before anyone intervenes, let’s be clear: shallow copies are not inherently bad! They can be perfectly fine when used knowingly. The issue arises when one is unaware of their actions. So, what exactly is a shallow copy?
Shallow Copy vs. Deep Copy
A shallow copy of an object is a new object that is a precise copy of the original object, but with references pointing to the same instances as the original. In contrast, a deep copy creates a new object along with copies of the objects referenced by the original. The difference is negligible with primitive data types and immutable classes. However, copying the reference of a mutable data type can lead to unexpected side effects.
Simplest Form Of A Shallow Copy
The simples way to create a shallow copy is an assignment of a mutable reference data type. Consider the following C# and Java examples:
public class Person
{
public required string FirstName { get; set; }
public required string LastName {get; set; }
public Person([NotNull] string firstName, [NotNull] string lastName)
{
FirstName = firstName ?? throw new ArgumentNullException(nameof(firstName));
LastName = lastName ?? throw new ArgumentNullException(nameof(lastName));
}
}
var person = new Person(firstName: "Joe", lastName: "Doe");
Person copy = person;
public class Person {
private String firstName;
private String lastName;
public Person(final @NonNull String firstName, final @NonNull String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(final @NonNull String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(final @NonNull String lastName) {
this.lastName = lastName;
}
}
String firstName, lastName;
var person = new Person(firstName = "Joe", lastName = "Doe");
Person copy = person;
Indeed, it takes a bit of imagination to see this as a shallow copy scenario, but that is indeed the case. In both examples, copy references the same Person instance as person. Since Person is a mutable class, any changes made to copy will reflect in person and vice versa:
copy.FirstName = "Jim";
Console.WriteLine(person.FirstName) // prints Jim
Console.WriteLine(copy.FirstName) // prints Jim
person.FirstName = "Jack";
Console.WriteLine(person.FirstName); // prints Jack
Console.WriteLine(copy.FirstName); // prints Jack
copy.setFirstName("Jim");
System.out.println(person.getFirstName()) // prints Jim
System.out.println(copy.getFirstName()) // prints Jim
person.setFirstName("Jack");
System.out.println(person.getFirstName()) // prints Jack
System.out.println(copy.getFirstName()) // prints Jack
Compound And Composite Objects
This effect just demonstrated remains, if we pass such an instance to compound class as composite objects. Suppose an e-commerce application, where we have a GoodsRecipient class, which consists of a Person and a (not shown) Address instance:
public sealed class GoodsRecipient
{
public required Person Person { get; }
public required Address Address { get; }
public GoodsRecipient([NotNull] Person person, [NotNull] Address address)
{
Person = person ?? throw new ArgumentNullException(nameof(person));
Address = address ?? throw new ArgumentNullException(nameof(address));
}
}
public final class GoodsRecipient {
private final Person person;
private final Address address;
public GoodsRecipient(final @NonNull Person person, final @NonNull Address address) {
this.person = person;
this.address = address;
}
public Person getPerson() {
return person;
}
public Address getAddress() {
return address;
}
}
GoodsRecipient is an immutable class. Once created, there is no way to change its person or address member data. Indeed? Observe the events that unfold:
The GoodsRecipient constructor assigns it’s two parameters to the corresponding class fields, thus creating a shallow copy of person and address. While it’s not possible to change a GoodsRecipient‘s person and address fields, for instance by assigning them new instances due to the missing C# property setter in conjunction with the required key word, or the missing Java setter method in conjunction with the final keyword on the fields, it is still possible to change the Person and Address instances.
var person = new Person(firstName: "Joe", lastName: "Doe");
var address = new Address(street: "5, Dead End Avenue", zip: "4711", city: "In The Middle Of Nowhere");
var goodsRecipient = new GoodsRecipient(person: person, address: address);
Console.WriteLine(goodsRecipient.Person.FirstName); // prints Joe
Console.WriteLine(goodsRecipient.Person.LastName); // prints Doe
Console.WriteLine(goodsRecipient.Address.Zip); // prints 4711
Console.WriteLine(person.LastName); // prints Doe
person.FirstName = "Jim";
address.Zip = "5822";
goodsRecipient.Person.LastName = "Duffy";
Console.WriteLine(goodsRecipient.Person.FirstName); // prints Jim
Console.WriteLine(goodsRecipient.Person.LastName); // prints Duffy
Console.WriteLine(goodsRecipient.Address.Zip); // prints 5822
Console.WriteLine(person.FirstName); // prints Duffy
String firstName, lastName;
var person = new Person(firstName = "Joe", lastName = "Doe");
String street, zip, city;
var address = new Address(street = "5, Dead End Avenue", zip = "4711", city = "In The Middle Of Nowhere");
var goodsRecipient = new GoodsRecipient(person, address);
System.out.println(goodsRecipient.getPerson.getFirstName()) // prints Joe
System.out.println(goodsRecipient.getPerson.getLastName()) // prints Doe
System.out.println(goodsRecipient.getAddress.getZip()) // prints 4711
System.out.println(person.getLastName()) // prints Doe
person.setFirstName("Jim");
address.setZip("5822");
goodsRecipient.getPerson.setLastName("Duffy");
System.out.println(goodsRecipient.getPerson.getFirstName()) // prints Jim
System.out.println(goodsRecipient.getPerson.getLastName()) // prints Duffy
System.out.println(goodsRecipient.getAddress.getZip()) // prints 5822
System.out.println(person.getLastName()) // prints Duffy
Creating only a shallow copy introduces a backdoor that compromises the immutability of GoodsRecipient. While this is often described as practical and useful, such practice can lead to significant issues in applications expected to have a lifecycle beyond two to three years, which often have more complex business use cases and nested workflows as our sample above. To avoid this nightmare, implementing a robust way to create deep copies is worth the time.
Simplest Way To Create A Deep Copy
Note: This sample code is solely for demonstrating the underlying mechanics. I would not recommend implementing deep copies in this manner unless you lack access to the class’s source code, which does not offer a superior method. Even then, I would advise writing a wrapper class to confine this expedient and unsophisticated implementation to a single, distinct point within your codebase.
While it is the quickest & dirtiest way to create a deep copy, it is the simplest one:
var person = new Person(firstName: "Joe", lastName: "Doe");
var copy = new Person(firstName: person.FirstName, lastName: person.LastName);
copy.FirstName = "Jim";
Console.WriteLine(person.FirstName) // prints Joe
Console.WriteLine(copy.FirstName) // prints Jim
String firstName, lastName;
var person = new Person(firstName = "Joe", lastName = "Doe");
var copy = new Person(firstName = person.getFirstName(), lastName = person.getLastName());
copy.setFirstName("Jim");
System.out.println(person.getFirstName()) // prints Joe
System.out.println(copy.getFirstName()) // prints Jim
Already Part Of Your Language: Copy Constructor
The good news is that most programming languages already have a construct to manage the creation of a copy. In C#, Java, and other languages, this construct is known as the “Copy Constructor.” The signature of a copy constructor is consistent with other constructors, yet it is distinct in terms of its parameters. The classic copy constructor accepts an instance of the same class as its sole argument.
public class Person
{
public required string FirstName { get; set; }
public required string LastName {get; set; }
public Person([NotNull] string firstName, [NotNull] string lastName)
{
FirstName = firstName ?? throw new ArgumentNullException(nameof(firstName));
LastName = lastName ?? throw new ArgumentNullException(nameof(lastName));
}
/// <summary>
/// Copy constructor. Creates a deep copy of a <see cref="Person"/>
/// </summary>
public Person([NotNull] Person toCopy)
{
_ = toCopy ?? throw new ArgumentNullException(nameof(toCopy))
FirstName = toCopy.FirstName;
LastName = toCopy.LastName;
}
}
public class Person {
private String firstName;
private String lastName;
public Person(final @NonNull String firstName, final @NonNull String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
/**
* Copy constructor. Creates a deep copy of a {@link Person}.
*/
public Person(final @NonNull Person toCopy) {
this.firstName = toCopy.firstName;
this.lastName = toCopy.lastName;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(final @NonNull String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(final @NonNull String lastName) {
this.lastName = lastName;
}
}
Using the copy constructor, our code to create a deep copy now looks like this:
var person = new Person(firstName: "Joe", lastName: "Doe");
var copy = new Person(person);
copy.FirstName = "Jim";
Console.WriteLine(person.FirstName) // prints Joe
Console.WriteLine(copy.FirstName) // prints Jim
String firstName, lastName;
var person = new Person(firstName = "Joe", lastName = "Doe");
var copy = new Person(person);
copy.setFirstName("Jim");
System.out.println(person.getFirstName()) // prints Joe
System.out.println(copy.getFirstName()) // prints Jim
Indeed, this is much more straightforward to read and extend.
Working With Deep Copies And Compound Classes
As previously stated, primitive data types and immutable classes are not included in the current scope of the problem; however, mutable instances are. Considering that our Person and Address classes are mutable and we have implemented a copy constructor for deep copying, it is essential to contemplate the steps required to make the GoodsRecipient class both secure and robust.
Please take a moment to reflect on the requirements before moving on. Ready? Alright, you may continue.
When you again examine the code above, which modifies the GoodsRecipient instance through Person and Address instances, and the Person instance through the GoodsRecipient instance, you are able to derive the requirements.
The simplest approach, which I — believe it or not — have observed in various software projects, is to pass a deep copy to the GoodsRecipient constructor and generate a deep copy every time the property/getter is accessed. However, this method is murky. It’s the class’ obligation to protect its state against unintended modification. Therefore, a clean, robust, readable and modifiable solution might look like this:
public sealed class GoodsRecipient
{
public required Person Person
{
get { return new Person(_person); }
}
public required Address Address
{
get { return new Address(_address); }
}
public GoodsRecipient([NotNull] Person person, [NotNull] Address address)
{
_person = new Person(person);
_address = new Address(address);
}
private readonly Person _person;
private readonly Address _address;
}
public sealed class GoodsRecipient {
private final Person person;
private final Address address;
public GoodsRecipient(final @NonNull Person person, final @NonNull Address address) {
this.person = new Person(person);
this.address = new Address(address);
}
public Person getPerson() {
return new Person(person);
}
public Address getAddress() {
return new Address(address);
}
}
Copy Constructor Secrets And Pitfalls
A Copy Constructor Never Creates A Shallow Copy
Consumers of a copy constructor can rely on the contract that it will never produce a shallow copy. As a rule of thumb, iat is the responsibility of every copy constructor implementation to ensure the creation of a complete deep copy.
As mentioned before, if there is no access to the source code of a class to add a proper copy constructor, another approach might help. If the class has a default constructor only, but public C# getter/setter/initialization properties or Java getter/setter methods, a deep copy can be constructed as well using this technique:
public class Person
{
public required string FirstName { get; set; }
public required string LastName { get; set; }
}
public static foo(Person anExtraTerrestial)
{
var copy = new Person()
{
FirstName = anExtraTerrestial.FirstName,
LastName = anExtraTerrestial.LastName
};
}
@Getter
@Setter
public class Person {
private String firstName;
private String lastName;
}
public static foo(final Person anExtraTerrestial) {
var copy = new Person();
copy.setFirstName(anExtraTerrestial.getFirstName());
copy.setLastName(anExtraTerrestial.getLastName());
}
However, instead of repeating this code whenever you need a copy of Person, an extension method in C# or a wrapper class in Java might be useful:
puplic class PersonExtensions
{
public static Person CreateDeepCopy(this Person toCopy)
{
return new Person()
{
FirstName = anExtraTerrestial.FirstName,
LastName = anExtraTerrestial.LastName
};
}
}
public static foo(Person anExtraTerrestial)
{
var copy = anExtraTerrestial.CreateDeepCopy();
}
public class LegacyPersonWrapper {
private final Person person;
public LegacyPersonWrapper(final @NonNull Person person) {
this.person = person;
}
public LegacyPersonWrapper(final @NonNull LegacyPersonWrapper toCopy) {
this(new Person(toCopy.getFirstName(), toCopy.getLastName()));
}
public String getFirstName() {
return person.getFirstName();
}
public void setFirstName(final String firstName) {
person.setFirstName(firstName);
}
// LastName getter/setter omitted
}
public static foo(LegacyPersonWrapper anExtraTerrestial) {
var copy = new LegacyPersonWrapper(anExtraTerrestial);
}
Other solutions include creating a wrapper in C#, or utilizing a static “extension” method in Java that takes a Person as an argument. However, be mindful of the pitfalls of static hell.
Avoid Boilerplate Copy Constructor Code
Upon closer examination of the Person copy constructors above, it becomes apparent that there is similar code in both the constructor and the copy constructor:
public Person([NotNull] string firstName, [NotNull] string lastName)
{
FirstName = firstName ?? throw new ArgumentNullException(nameof(firstName));
LastName = lastName ?? throw new ArgumentNullException(nameof(lastName));
}
/// <summary>
/// Copy constructor. Creates a deep copy of a <see cref="Person"/>
/// </summary>
public Person([NotNull] Person toCopy)
{
_ = toCopy ?? throw new ArgumentNullException(nameof(toCopy))
FirstName = toCopy.FirstName;
LastName = toCopy.LastName;
}
public Person(final @NonNull String firstName, final @NonNull String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
/**
* Copy constructor. Creates a deep copy of a {@link Person}.
*/
public Person(final @NonNull Person toCopy) {
this.firstName = toCopy.firstName;
this.lastName = toCopy.lastName;
}
To eliminate the boilerplate code, we can simply invoke the all-argument constructor from within the copy constructor.
public Person([NotNull] string firstName, [NotNull] string lastName)
{
FirstName = firstName ?? throw new ArgumentNullException(nameof(firstName));
LastName = lastName ?? throw new ArgumentNullException(nameof(lastName));
}
/// <summary>
/// Copy constructor. Creates a deep copy of a <see cref="Person"/>
/// </summary>
public Person([NotNull] Person toCopy)
: this(toCopy?.FirstName ?? throw new ArgumentNullException(nameof(toCopy)),
toCopy?.LastName ?? throw new ArgumentNullException(nameof(toCopy)))
{}
public Person(final @NonNull String firstName, final @NonNull String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
/**
* Copy constructor. Creates a deep copy of a {@link Person}.
*/
public Person(final @NonNull Person toCopy) {
this(toCopy.firstName, toCopy.lastName);
}
Beyond the issue of boilerplate code, there’s a more significant problem with the former type of implementation: when a new property or field is added to the Person class, it’s often overlooked to update both the constructor and the copy constructor. Thus, circumventing this boilerplate code by calling the all-arguments constructor enhances robustness and simplifies extensibility. Adding a new field and updating only the all-arguments constructor will result in a compile-time error in the copy constructor.
Copy Constructor Issues With Inheritance
Invoking the all-arguments constructor from the copy constructor, as previously mentioned, offers an additional benefit in class hierarchies: the superclass is not required to implement a copy constructor. Nevertheless, it is advisable to forgo the copy constructor in the superclass only if the superclass is abstract and, therefore, cannot be instantiated independently. But keep calling the all argument constructor in the derived class due to the reasons previously mentioned.
public class Manager : Person
{
public string Position { get; set; }
public Manager([NotNull] string firstName, [NotNull] string lastName, [NotNull] string position)
: base(firstName ?? throw new ArgumentNullException(nameof(firstName)),
lastName ?? throw new ArgumentNullException(nameof(lastName)))
{
Position = position ?? throw new ArgumentNullException(nameof(position));
}
public Manager([NotNull] Manager toCopy)
: this(toCopy?.FirstName ?? throw new ArgumentNullException(nameof(toCopy)),
toCopy.LastName, toCopy.Position)
{}
}
@Getter
@Setter
public class Manager extends Person {
private String position;
public Manager(final @NonNull String firstName, final @NonNull String lastName,
final @NonNull String position) {
super(firstName, lastName);
this.position = position;
}
public Manager(final @NonNull Manager manager) {
this(manager.getFirstName(), manager.getLastName(), manager.getPosition());
}
}
Collection Members
Special attention is needed when a class holds collections of components (one-to-many relations). Suppose our GoodsRecipient class above supports multiple Address instances, one for each AddressType. When handling mutable class components, as in our situation, it’s essential to not just create a new collection but also to perform a deep copy of the components. On the other hand, if the components belong to immutable classes, simply creating a new collection is adequate.
public enum AddressType
{
InvoiceAddress,
DeliveryAddress
}
public sealed class GoodsRecipient
{
public Person Person { get; init; }
public Dictionary<AddressType, Address> Addresses { get; init; }
public GoodsRecipient(Person person, Dictionary<AddressType, Address> addresses)
{
Person = new Person(person);
Addresses = new Dictionary<AddressType, Address>();
foreach (var entry in addresses)
{
Addresses[entry.Key] = new Address(entry.Value);
}
}
// Copy constructor
public GoodsRecipient(GoodsRecipient toCopy)
: this(toCopy?.Person ?? throw new ArgumentNullException(nameof(toCopy)),
toCopy.Addresses)
{}
}
public enum AddressType {
INVOICE_ADDRESS,
DELIVERY_ADDRESS
}
public final class GoodsRecipient {
private final Person person;
private final EnumMap<AddressType, Address> addresses;
public GoodsRecipient(final @NonNull Person person, final @NonNull EnumMap<AddressType, Address> addresses) {
this.person = new Person(person);
this.addresses = new EnumMap<>(AddressType.class);
for (Map.Entry<AddressType, Address> entry : addresses.entrySet()) {
this.addresses.put(entry.getKey(), new Address(entry.getValue()));
}
}
// Copy constructor
public GoodsRecipient(final @NonNull GoodsRecipient toCopy) {
this.person = new Person(toCopy.person);
this.addresses = new EnumMap<>(AddressType.class);
for (Map.Entry<AddressType, Address> entry : toCopy.addresses.entrySet()) {
this.addresses.put(entry.getKey(), new Address(entry.getValue()));
}
}
public Person getPerson() {
return new Person(person);
}
public EnumMap<AddressType, Address> getAddresses() {
EnumMap<AddressType, Address> copiedAddresses = new EnumMap<>(AddressType.class);
for (Map.Entry<AddressType, Address> entry : addresses.entrySet()) {
copiedAddresses.put(entry.getKey(), new Address(entry.getValue()));
}
return copiedAddresses;
}
}
Consider to return immutable collections in getters as an alternate, if the effort to copy the collection content is too high in your circumstance. In C#, this introduces the usage of the Immutable* variants of the collection library. In Java, I’d encourage to use Eclipse Collections over the dirty Collections.unmodifiable* variants in Java.Util. Nevertheless, the vast scope of this topic merits further exploration in a subsequent article.
Deep Copy Performance
Deep copying is inherently more resource-intensive than shallow copying because it involves duplicating every referenced object. This process can consume significant CPU and memory resources, especially for complex objects with deep or large object graphs.
Considerations:
- CPU Usage: The CPU overhead increases with the complexity and depth of the object graph.
- Memory Usage: Memory consumption grows as new copies of objects are created, leading to higher memory usage compared to shallow copying.
- Garbage Collection: More objects mean more work for the garbage collector, which can impact performance in managed languages like C# and Java.
Use Cases:
- Scenarios requiring true isolation between the original and copied objects.
- Implementing robust classes without side effects to fully adhere to the encapsulation requirements of OOP’s fundamental paradigms.
- Long-lived objects where unintended side effects can lead to hard-to-debug issues.
Copy Constructor Efficiency
Copy constructors provide a balance between control and performance. By explicitly defining how each field and reference should be copied, you can optimize the copying process for your specific use case. However, the performance depends on how the copy constructor is implemented.
Best Practices:
- Avoid unnecessary deep copies of immutable fields or value types.
- Use efficient algorithms for copying collections and nested objects.
Alternate Ways To Create A Deep Copy
While implementing a copy constructor offers full control over what exactly happens when a deep copy is created and can distinguish between mutable and immutable classes, the effort to implement it is shunned by many developers. Therefore, many alternate ways to create a deep copy exist and I don’t want to bypass them without a closer view.
JSON Serialization
Many JSON serializers exist in both worlds, .NET and Java. System.Text.Json.JsonSerializer or Newtonsoft.Json on one hand, Gson, Apache Commons Lang Serialization Utils, or Jackson on the other. This list is not complete, of course. Their original intent was to convert objects into a string representation and vice versa to send them as strings over service boundaries with REST interfaces. With this capability, they seem to fit perfectly to create deep copies of objects with nearly an effort striving towards zero. Let’s have a look using JsonSerializer for the C# samples and Jackson for Java. Common to both worlds is the need to implement a specific method whose name ideally has to be negotiated with the whole development team and must used consequently over the whole code base.
public Person DeepCopy()
{
string json = JsonSerializer.Serialize(this);
return JsonSerializer.Deserialize<Person>(json);
}
public Person deepCopy() throws Exception {
ObjectMapper mapper = new ObjectMapper();
String json = mapper.writeValueAsString(this);
return mapper.readValue(json, Person.class);
}
I must admit, that it is very easy to achieve deep copy capabilities with this technique. However, in my honest opinion, it’s a shortcut, because it comes with different disadvantages not visible when reading this simple code.
Firstly, the processes of serialization and deserialization can be quite time-consuming as they involve deep copying of immutable instances as well, leaving no room for optimization.
All those implementations are using reflection to inspect the properties and fields of objects at runtime to create the serialized string and to create new instances and set the properties and fields during deserialization. This allows it to dynamically access and convert the object's data into JSON format and vice versa.
Using reflection, however, has some implications:
- Performance: Reflection can be slower compared to direct field or property access because it involves additional metadata processing at runtime.
- Security: Reflection can potentially access private fields and methods, which might have security implications. However, serializers like JsonSerializer and Jackson are designed with security in mind and typically only access fields and properties that are intended to be serialized.
- Safety: A JVM can be configured to disable reflection at all for safety reasons, and in current times, where any known vulnerabilities are closed, it’s likely to meet such environment. Any Java application using reflection code will not run under such circumstances.
- Leaving the craftsmen’s path: Reflection makes the serialization and deserialization process simpler for developers because they don’t need to write custom code for each class they want to serialize. The serializer can handle a wide variety of classes without additional configuration. But it often requires special implementation of a class. Jackson for instance on the Java side requires each and every object to provide a default constructor, or an annotated all argument constructor or factory method. While a default constructor may be acceptable for a simple Data Transfer Object (DTO), offering one for a Plain Old Java Object (POJO) such as a domain entity or business object — solely to facilitate deep copying when the business case necessitates a different constructor — can be problematic. It may introduce unintended side effects, which deep copy implementations aim to avoid. Moreover, annotating POJOs with annotations defined in a 3rd-party library effectively transforms them into something beyond traditional POJOs.
Similar applies for JsonSerializer or Newtonsoft.Json it the C# world. In certain situations, writing a custom JSON converter is necessary. However, the advantages of creating a custom converter over using a simple copy constructor are not immediately clear to me.
Third-Party Libraries For Deep Copy
There are several libraries in C# which support creation of deep copies using extension methods on object. Force.DeepClone or FastDeepClone are two examples. However, since they all operate with reflection, the same mentioned above about reflection applies as well.
ICloneable / Cloneable Interface Implementation
ICloneable in C# and Cloneable in Java are interfaces to support the copy process of an object. Despite its simplicity and apparent usefulness, these interface have several significant drawbacks:
- Lack of Type Safety: The Clone/clone method returns an object/Object, which means you need to cast the result back to the appropriate type. This can lead to runtime errors if the cast fails.
- Undefined Semantics (Shallow vs. Deep Copy): The interfaces don’t specify whether Clone/clone should perform a shallow copy or a deep copy. This ambiguity can lead to confusion and bugs, as different classes might implement Clone/clone differently. In Java, Object::clone() performs a shallow copy. This can lead to unintended sharing of mutable objects between the original and the clone, if not carefully handled.
- Complexity and Fragility: Implementing a proper deep copy using Clone/clone() can be complex and error-prone, especially for classes with deep object graphs or complex inheritance hierarchies. Developers must manually ensure that all necessary objects are deeply cloned, which can be tedious and fragile. Using the presented copy constructor secrets above, this problem is much more virulent.
- Inconsistent Usage: (Java only) The Cloneable interface does not provide a clone method itself, leading to inconsistent usage and potential misunderstandings about what it means to implement Cloneable.
Conclusion
Understanding the distinction between shallow and deep copying is essential for developing robust and extendable software. Shallow copies, while efficient, can introduce unintended side effects when working with mutable objects, potentially leading to hard-to-diagnose bugs. On the other hand, deep copies ensure that objects are fully duplicated, providing complete isolation between the original and the copy. This article has explored various techniques to implement deep copying, emphasizing the use of copy constructors in C# and Java.
Implementing deep copies through copy constructors offers precise control over the copying process, ensuring that mutable references are properly duplicated. However, this method requires careful consideration and maintenance to avoid boilerplate code and ensure that new fields are correctly handled. Alternate methods, such as serialization or third-party libraries, provide convenience but come with performance and security trade-offs due to their reliance on reflection.
When choosing a copying technique, consider the specific requirements of your application. Shallow copies may suffice for immutable objects or performance-critical sections, while deep copies are necessary for ensuring object isolation in complex workflows. Performance profiling and testing are crucial to determine the impact of your chosen method on your application’s responsiveness and resource utilization.
Ultimately, the key to effective copying lies in understanding the implications of each method and applying best practices to ensure that your objects are copied correctly, maintaining the integrity and reliability of your software. By mastering these concepts, developers can avoid common pitfalls and create applications that are both robust and extensible.
About The Author
I’m a Swiss citizen and an experienced software craftsman for over 34 years with a wide variety of fields. I was involved in different branches during my software engineering carrier. I brought value to my clients as a developer (started with LISP, switched to C/C++, PHP, C#, and Java), as a manager, as a consultant, as well as a mentor and head of agile development teams. My main goal is delivering high quality for a feasible price because I learnt one thing for sure within the last three decades: If you must go fast, you must go well and make good decisions. Leadership skills complete my palmares. If you like my thoughts, follow me on X (@swcraftsman) or here on medium.
Deep Copy And The Secrets Of Copy Constructors was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Martin Muller
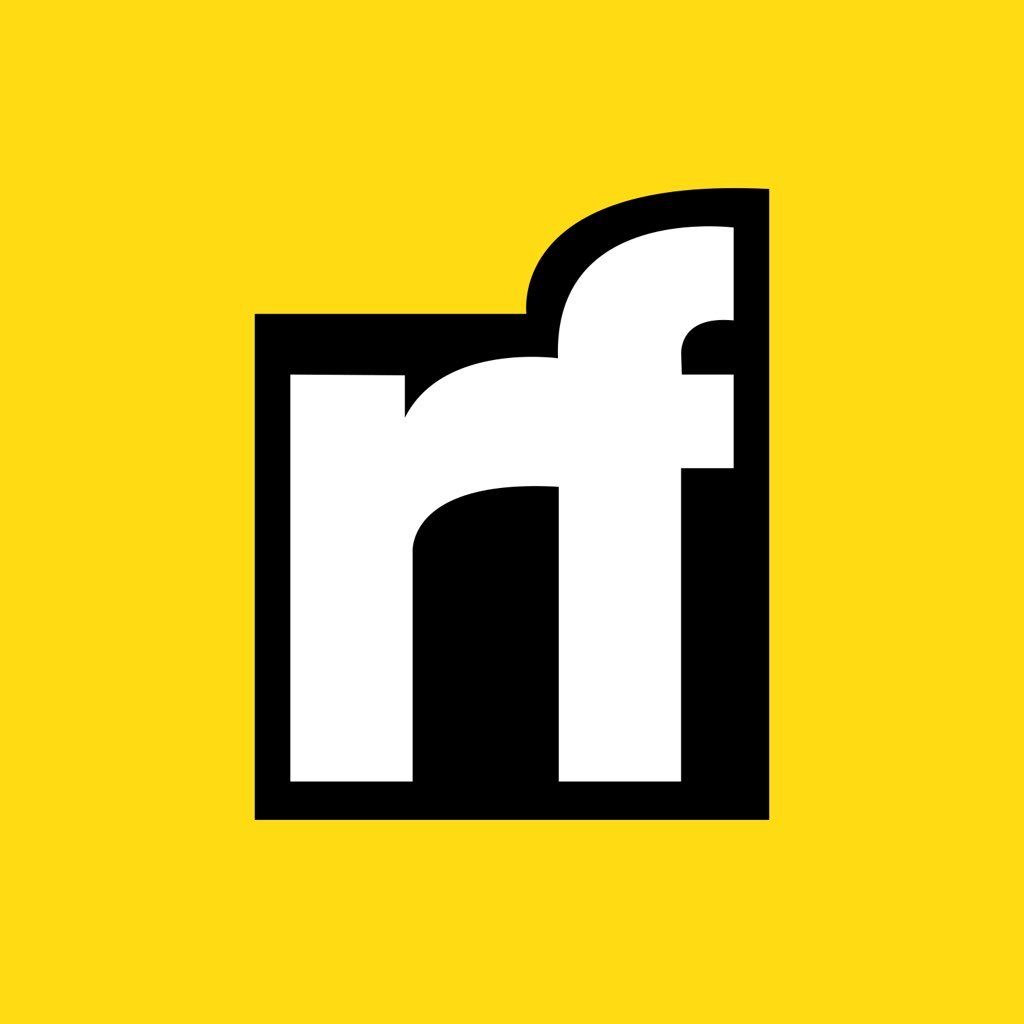
Martin Muller | Sciencx (2024-07-07T17:09:33+00:00) Deep Copy And The Secrets Of Copy Constructors. Retrieved from https://www.scien.cx/2024/07/07/deep-copy-and-the-secrets-of-copy-constructors/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.