This content originally appeared on DEV Community and was authored by Subham Behera
JavaScript is a versatile and powerful language, but it comes with its own set of quirks. One of the most bizarre and often misunderstood aspects of JavaScript is type coercion. Type coercion is the process by which JavaScript automatically converts a value from one type to another. This can lead to some unexpected and downright weird behaviors.
Understanding Type Coercion
Type coercion in JavaScript can occur in various situations, such as during comparisons, arithmetic operations, and even in logical contexts. JavaScript has two types of equality operators: ==
(loose equality) and ===
(strict equality). The loose equality operator (==
) performs type coercion, while the strict equality operator (===
) does not.
Let's start with some simple examples to see how type coercion works:
console.log(1 == '1'); // true
console.log(1 === '1'); // false
In the first example, JavaScript converts the string '1'
to a number before making the comparison, resulting in true
. In the second example, no conversion occurs, so the comparison returns false
.
Type Coercion in Arithmetic Operations
Type coercion can lead to some unexpected results in arithmetic operations. Let's look at a few examples:
console.log('5' - 3); // 2
console.log('5' + 3); // '53'
console.log('5' * '2'); // 10
console.log('5' / '2'); // 2.5
In the first example, the string '5'
is converted to a number before the subtraction operation, resulting in 2
. However, in the second example, the +
operator concatenates the string '5'
and the number 3
, resulting in the string '53'
. The *
and /
operators also convert strings to numbers before performing the operations.
The Infamous NaN
and Type Coercion
NaN
stands for "Not-a-Number," but it is, in fact, a number type in JavaScript. Type coercion involving NaN
can lead to some peculiar results:
console.log(NaN == NaN); // false
console.log(NaN === NaN); // false
console.log(isNaN(NaN)); // true
console.log(isNaN('hello')); // true
NaN
is the only value in JavaScript that is not equal to itself, which is why NaN == NaN
and NaN === NaN
both return false
. The isNaN
function can be particularly confusing because it returns true
for non-numeric strings as well.
Coercion in Logical Contexts
Type coercion also occurs in logical contexts, such as if
statements and logical operators (&&
, ||
):
console.log(false == '0'); // true
console.log(false === '0'); // false
console.log(null == undefined); // true
console.log(null === undefined); // false
if ('hello') {
console.log('This is true!'); // This is true!
}
if ('') {
console.log('This is true!');
} else {
console.log('This is false!'); // This is false!
}
In logical contexts, JavaScript coerces values to booleans. Non-empty strings are truthy, while empty strings are falsy. Similarly, null
and undefined
are both falsy, but null == undefined
returns true
due to type coercion.
The Weirdness of Arrays and Objects
Type coercion can produce some truly bizarre results when dealing with arrays and objects:
console.log([] == ''); // true
console.log([] == 0); // true
console.log([1, 2, 3] == '1,2,3'); // true
console.log({} == '[object Object]'); // false
console.log({} + []); // '[object Object]'
console.log([] + {}); // '[object Object]'
In the first example, an empty array is coerced to an empty string, resulting in true
. In the second example, the empty array is coerced to 0
, also resulting in true
. The third example converts the array [1, 2, 3]
to the string '1,2,3'
. However, when comparing objects, the results can be less predictable, as seen in the fourth example.
The last two examples demonstrate the odd behavior of the +
operator with arrays and objects. When adding an empty array to an object, JavaScript converts both to strings and concatenates them.
Avoiding Type Coercion Pitfalls
To avoid the pitfalls of type coercion, it's important to use the strict equality operator (===
) whenever possible. This ensures that no type conversion occurs during comparisons:
console.log(1 === '1'); // false
console.log(false === '0'); // false
console.log(null === undefined); // false
Additionally, you can use explicit type conversion to make your intentions clear:
console.log(Number('5') - 3); // 2
console.log(String(5) + '3'); // '53'
console.log(Boolean('hello')); // true
console.log(Boolean('')); // false
Conclusion
JavaScript type coercion can lead to some surprising and sometimes confusing results. You can avoid many common pitfalls by understanding how type coercion works and using strict equality checks and explicit type conversions. Embrace the quirks of JavaScript, and you'll be better equipped to handle the bizarre world of type coercion with confidence.
Happy coding!
This content originally appeared on DEV Community and was authored by Subham Behera
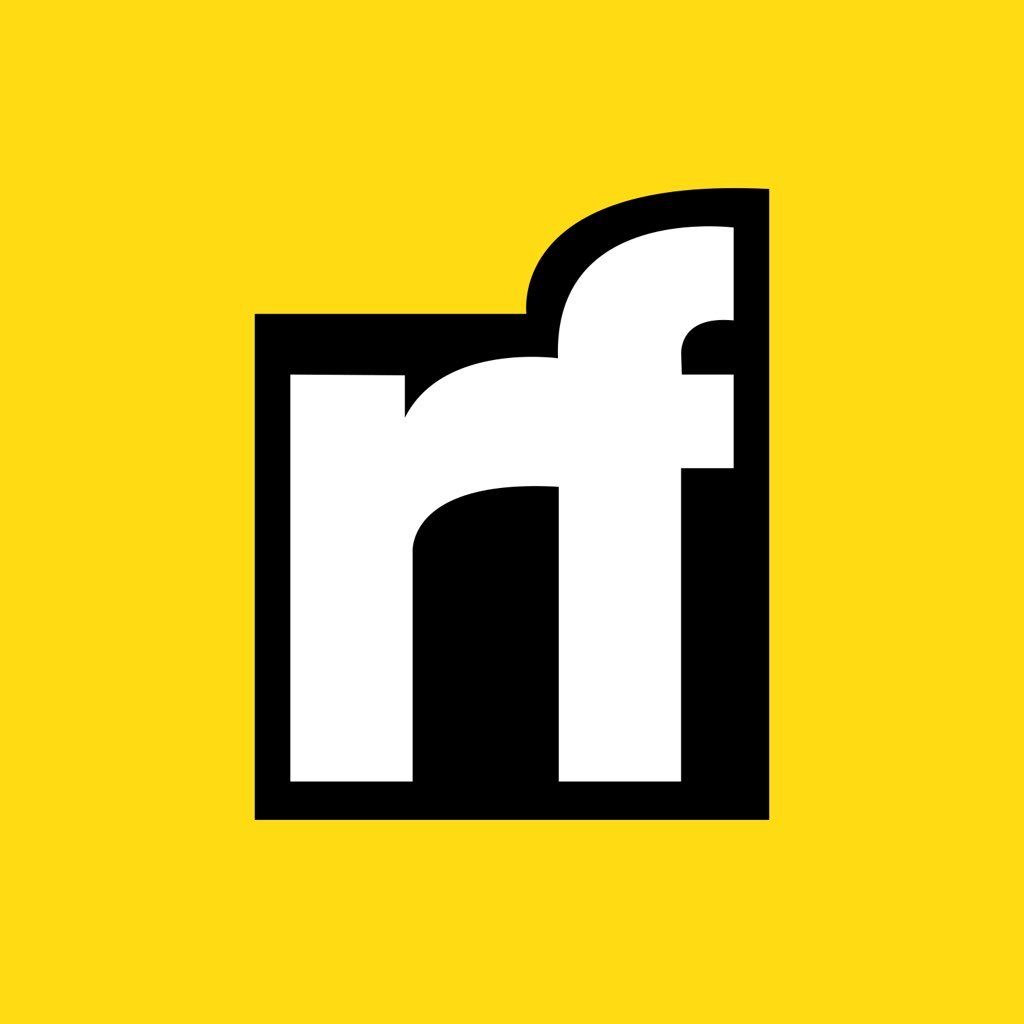
Subham Behera | Sciencx (2024-07-07T01:50:26+00:00) The Bizarre World of JavaScript Type Coercion. Retrieved from https://www.scien.cx/2024/07/07/the-bizarre-world-of-javascript-type-coercion/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.