This content originally appeared on Telerik Blogs and was authored by Assis Zang
In this blog post, we will check out 12 questions and their answers on essential topics for understanding the basic concepts of ASP.NET Core and web development with C#.
Understanding the basics of ASP.NET Core is essential for beginners, as it creates a solid foundation for developers who want to enter the world of web development with Microsoft’s .NET framework. These core concepts are the foundations upon which developers build their skills and knowledge, enabling them to create robust and scalable web applications.
By mastering the fundamentals, beginners gain a deeper understanding of modern web development principles, which include data models, object orientation and state management.
With a solid foundation in the basics of ASP.NET Core, beginners can quickly progress on their learning journey, exploring more advanced topics such as user authorization and authentication, automated testing and cloud deployment.
Additionally, a solid understanding of ASP.NET Core fundamentals allows developers to adapt to future updates and evolutions of the framework easily, ensuring they remain current and competitive in the web development market.
In this post, we will check out 12 questions and their respective answers and see practical examples and references for further studies. At the end of the post, you will know about the main topics involved in developing web applications with ASP.NET Core.
You can access the source code of the examples demonstrated in the post here: Source Code Examples.
1. What Is ASP.NET Core?
ASP.NET Core is a modern, high-performance, cross-platform web development framework for .NET.
It is an evolution of the ASP.NET platform, designed to be more modular and lightweight, and able to work on multiple platforms. ASP.NET Core allows developers to build web applications and services using the C# programming language.
Some key features of ASP.NET Core include:
Multiplatform: ASP.NET Core is compatible with Windows, macOS and Linux, allowing applications to be developed and deployed on multiple operating systems.
Modularity: The framework comprises separate modules (NuGet packages), allowing developers to include only the components necessary for their applications, which contributes to efficiency and reduces the final size of the application.
Performance: ASP.NET Core has been optimized to deliver superior performance compared to previous versions of ASP.NET. It uses techniques such as asynchronous processing to improve the scalability and responsiveness of applications.
Container support: ASP.NET Core is designed to run in containers, making it easier to deploy and scale applications in container-based environments like Docker.
Open source: ASP.NET Core is completely open source, which means that developers have access to the source code and can contribute to the development and improvement of the platform.
Visual Studio integration: Developers can use Visual Studio, Microsoft’s IDE, to efficiently develop ASP.NET Core applications, taking advantage of features such as debugging, monitoring tools and integrated source control.
References:
2. C# Is a Typed, Compiled and Managed Language—What Does That Mean?
The C# programming language is used to develop ASP.NET Core applications and has three main characteristics:
Typed
This means that the language requires variables to have a specified data type. For example, a variable can be of integer type (int
), floating point type (float
), character type (char
), among others. This helps ensure that operations performed on a variable are appropriate for the type of data it stores, reducing programming errors.
C# is a strongly typed language, which means that data types are strictly checked at compile time. This increases the security and robustness of the code, as many errors can be detected even before the program is executed, preventing simple errors such as decimal amount = "sample value";
from reaching testing and production environments.
Note that in the code above a variable called amount
is being declared, which is of decimal type (decimal numeric values), but a value of type text is being assigned to it. If this code is declared in C#, the application will not even be compiled until the error is corrected.
Compiled
This means that the source code written in C# is translated into machine language (executable code) by a program called a compiler. The source code is compiled into an executable file that can be run directly by the computer.
Compilation occurs in two phases:
Compilation to Common Intermediate Language (CIL), which is an intermediate language
Just-In-Time (JIT) compilation, which translates CIL into platform-specific machine code during execution of the program
Compilation has performance benefits because compiled code is generally faster than interpreted code.
Managed
This means that the execution of C# code is controlled by a runtime environment, such as the Common Language Runtime (CLR) for .NET Framework or .NET Core Runtime for .NET Core.
The runtime environment manages memory allocation and release, exception management, thread execution and other tasks related to program execution.
Memory management includes the use of garbage collection, which automatically frees memory from unused objects, making development more convenient and safer for programmers without the need for manual memory management, although it may introduce some performance overhead.
Reference: A tour of the C# language.
3. What Does Intermediate Language (IL) Mean?
Intermediate language (IL) is the result of compiling code written in high-level .NET languages.
Like other .NET frameworks, ASP.NET Core compiles C# or VB.NET source code into IL. This IL is then just-in-time (JIT) compiled to the machine code specific to the platform it is running on.
IL code is an intermediate representation of source code that is independent of the specific hardware architecture. This allows code to run on different platforms and architectures, as long as there is a JIT compiler available to translate the IL code into executable machine code.
Therefore, when you develop an ASP.NET Core application, the C# code you write is compiled to IL and then executed on the target machine through the JIT compilation process. This provides flexibility and portability to .NET Core applications.
Reference: Managed Code.
4. What Is Object Oriented Programming (OOP)?
Object-oriented programming (OOP) is a programming paradigm that is based on the concept of “objects,” which can contain data in the form of fields (also known as attributes or properties) and code in the form of procedures (methods or functions). These objects can interact with each other.
OOP is comprised of four fundamental principles:
Abstraction: The process of identifying the essential characteristics of an object and ignoring less important details.
Encapsulation: The idea of grouping data and related methods into a single unit, which is the class, and restricting direct access to the data.
Inheritance: The ability of a class to inherit attributes and methods from another class, allowing code reuse and the creation of class hierarchies.
Polymorphism: The ability for objects from different classes to be treated similarly, allowing methods with the same signature to behave differently depending on the type of object that calls them.
In ASP.NET Core, object-oriented programming is widely used to create web applications, where classes are typically created to represent data models, services, controllers, views and other application components.
These classes interact with each other, following OOP principles, to create robust, scalable and easily maintained applications. For example, you can create classes to represent users, products, orders and services, and then use inheritance, encapsulation and polymorphism to organize and manage these objects effectively.
References:
5. What Are Built-in Types and What Are Some Examples?
In C#, “built-in types” refer to fundamental data types built directly into the language. These types are native to the language and do not require additional definitions to be used. They are used to declare variables, define method parameters and return method values.
Below are some examples of built-in types in C#:
- Integral numeric types:
- int: Represents 32-bit integers.
- long: Represents 64-bit integers.
- Numerical types of floating point:
- float: Represents single-precision floating-point numbers.
- double: Represents double precision floating point numbers.
- Character types:
- char: Represents a single Unicode character.
- Boolean type:
- bool: Represents true or false values.
- String type:
- string: Represents a sequence of Unicode characters.
- Object type:
- object: It is the root of the type hierarchy in C#; all types, including built-in types, are derived from objects.
Reference: C# built-in types.
6. What Are Namespaces and What Are They Used for?
In ASP.NET Core, a namespace is a way to logically organize and group related types, such as classes, structs, interfaces, enums and others.
They are used for the following purposes:
Code organization and structuring: Namespaces help organize code into a logical and hierarchical structure, making source code easier to understand and maintain, especially in large projects.
Preventing name conflicts: Namespaces allow types to have similar names as long as they belong to different namespaces. They help avoid name conflicts in projects that use third-party libraries or frameworks, or even in internal projects with many classes.
Encapsulation and hiding of implementation details: Namespaces allow you to hide the implementation of certain types, providing a layer of encapsulation protecting the internal details of classes and providing a clean interface for consumers of the code.
Facilitate code reuse: By grouping related types into namespaces, it becomes easier to identify and reuse classes and other types in different parts of the project or across different projects.
Facilitate code distribution: Using well-defined namespaces can facilitate the distribution of software components such as libraries or frameworks, as it allows consumers to easily access the provided types without ambiguity. For example, the .NET library has namespaces such as System, System.Collections and System.IO, among others, to organize different functionalities and related types. These namespaces help structure the library and make it easier for developers to understand and use its functionality.
Reference: C# Namespaces.
7. What Are Classes and Objects, and How Do They Relate to Each Other?
Classes and objects are fundamental concepts for implementing object-oriented systems.
Class is a blueprint or template for creating objects. It defines the properties (attributes) and behaviors (methods) that the objects of the class will have. We can say that the class is like the construction blueprint for a house, defining the characteristics and methods that operate on encapsulated data for the object.
Example of implementing a class:
public class User
{
public Guid Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public User()
{
}
}
Object is an instance of a class, representing a specific class instance with its unique data and state.
Objects are created based on the structure defined by the class, interacting with other objects and performing actions based on the methods defined by the class. This way we can say that if the class is the house plan, the object is the house already built.
Below is an example of an object created from the User
class:
var newUser = new User();
newUser.Id = Guid.NewGuid();
newUser.Name = "John Smith";
newUser.Age = 18;
Relationship Between the Two
Classes and objects work together to enable the creation and manipulation of data in an organized and efficient manner within object-oriented programming paradigms.
While classes define the structure and behavior of objects, objects are instances of classes, which are created based on the model provided by the class.
Reference: C# classes.
8. What Are Access Modifiers?
Access modifiers are keywords used to restrict or not access a member or type, which include classes, methods, variables and others. Below are six types of access modifiers in C#:
public
: Members marked as public are accessible from anywhere, both inside and outside the class in which they are defined.private
: Members marked as private are accessible only within the class in which they are defined. They cannot be accessed by code outside the class, including subclasses.protected
: Members marked as protected are accessible within the class in which they are defined and also in subclasses of that class. However, they are not accessible outside the inheritance hierarchy.internal
: Members marked as internal are accessible within the same assembly (compilation unit) in which they are defined. This means that other files in the same project can access these members, but they cannot be accessed from outside the assembly.protected internal
: It is a combination of the protected and internal modifiers. Internal protected members are accessible within the same assembly in which they are defined and also in subclasses, regardless of the assembly.private protected
: This modifier allows access to members only within the same assembly and within derived classes in the same assembly. In other words, it is more restrictive thanprotected internal
, as it does not allow access to subclasses outside the same assembly.
Reference: Access modifiers.
9. What Are Interfaces and Abstract Classes and What Are the Differences Between Them?
Interfaces and abstract classes are key concepts in object-oriented programming that allow you to define structures for classes and objects.
Interfaces
An interface is a structure that contains declarations of methods, properties, events or indexers that a class or struct can implement.
Interfaces are used to define common behaviors that different classes can share, regardless of inheritance.
Example:
public interface IShape
{
double GetArea();
double GetPerimeter();
}
public class Square : IShape
{
public double GetArea()
{
throw new NotImplementedException();
}
public double GetPerimeter()
{
throw new NotImplementedException();
}
}
Abstract Classes
An abstract class is a class that cannot be instantiated by itself. It needs to be derived by other classes.
It can contain abstract methods (methods without implementation) and concrete methods (with implementation).
Abstract classes are used to provide a common structure for their subclasses. They can contain common states and behaviors shared by their subclasses. Subclasses must implement all of the abstract methods of the abstract class or they must themselves be declared as abstract classes.
Differences
Interfaces cannot be instantiated, only implemented by classes, while abstract classes can contain constructors and therefore can have instances, but the abstract class itself cannot be instantiated directly.
Interfaces can only contain the signature of methods, without implementation, while abstract classes can contain abstract methods (without implementation) and concrete methods (with implementation).
Example:
abstract class Shape
{
public abstract int GetArea();
}
class NewSquare : Shape
{
private int _side;
public NewSquare(int n) => _side = n;
public override int GetArea() => _side * _side;
}
References:
10. What Is a Sealed Class and When Is It Used?
In C#, a sealed class is a class that cannot be inherited. It is designed to prevent other classes from inheriting from it.
Sealed classes are useful in several situations where it is desirable to ensure that a class is not inherited. Below are some scenarios where a sealed class is useful.
Completed implementation: When the implementation of a class is complete and is not expected to be extended or modified.
Implementation safety: So that certain class members are not overridden in derived classes. By marking the class as sealed, it is possible to protect certain aspects of the class’s implementation.
Performance: In some situations, marking a class as sealed can allow performance optimizations by the compiler, as it knows that there is no need to consider the possibility of inheritance and member replacement.
Immutable data modeling: In some situations, there may be classes that represent immutable data, where it does not make sense to extend or modify the class. Marking these classes as sealed can help keep them immutable.
Example:
sealed class RestrictedUserService
{
private List<string> SearchRestrictedInformation(string userName)
{
// Rest of the code...
}
}
Reference: Sealed class.
11. What Are Relational Databases? What Are Some Well-known Examples?
Relational databases are database management systems (DBMSs) that structure and organize data into tables that are related to each other. These databases use the relational model, where data is stored in tables consisting of rows and columns. Primary and foreign keys define the relationships between these tables.
In the context of ASP.NET Core, we can identify some well-known examples of relational databases:
SQL Server: Developed by Microsoft, SQL Server is a very popular relational DBMS widely used in ASP.NET Core applications. It provides advanced data management, security and performance features.
MySQL: MySQL is a widely used open-source relational DBMS. It is known for its reliability, scalability and performance, making it a popular choice for ASP.NET Core applications.
PostgreSQL: PostgreSQL is another open-source relational DBMS with a strong reputation for being robust, reliable and highly extensible. It is often chosen for applications that require advanced data manipulation and transaction support capabilities.
SQLite: SQLite is a relational database library that operates without a separate database server. It is a popular choice for ASP.NET Core applications that require an embedded database, as it does not require separate server configuration and is easy to deploy. Due to its simplicity, it is widely used in mobile applications.
Oracle Database: Developed by Oracle Corporation, the Oracle Database is one of the oldest and most widely used DBMSs. It is known for its scalability, performance and advanced features, making it a common choice for large enterprise ASP.NET Core applications.
Reference: What is a relational database?
12. What Are Web APIs and What Resources Are Available in ASP.NET Core to Work with Them?
Web Application Programming Interfaces (APIs) are sets of protocols and tools for building software applications that interact with each other on the internet.
They allow different systems to communicate and share data efficiently and securely. Web APIs are widely used to integrate systems, provide services and enable communication between different applications and devices.
In the context of ASP.NET Core, there are several features available for working with Web APIs:
- ASP.NET Core MVC: ASP.NET Core MVC (Model-View-Controller) is a powerful framework for building web applications and APIs. It provides complete support for developing and consuming web APIs using RESTful standards. MVC controllers can be used to define API endpoints and handle HTTP requests.
- ASP.NET Core Web API: ASP.NET Core Web API is a specific framework for creating HTTP APIs. It offers built-in support for routing, JSON serialization, versioning, middleware and other features needed to build complete, high-quality RESTful APIs.
- Flexible routing: ASP.NET Core offers a flexible routing system that allows you to define custom route patterns to map HTTP requests to the appropriate control methods. This simplifies the configuration of API endpoints with meaningful, user-friendly URIs and provides capabilities for versioning.
- JSON serialization: ASP.NET Core has built-in support for serialization and deserialization of JSON objects. This facilitates communication between clients and servers that exchange data in JSON format, widely used in APIs and web systems.
- Middleware: The ASP.NET Core middleware pipeline provides a flexible way to add request and response processing functionality to an API. This includes middleware for authentication, authorization, logging and compression, among others.
- Swagger/OpenAPI: ASP.NET Core supports integration with Swagger/OpenAPI, which is a tool for documenting APIs in an automated way. It automatically generates interactive documentation for the APIs, which is extremely useful for developers consuming the API to understand how to use it correctly.
Reference: APIs with ASP.NET Core.
Conclusion
Investing time and effort into understanding the fundamentals of ASP.NET Core is essential for beginners who want to build a successful career as web developers.
In this post, we covered 12 questions and their answers on the main topics involving programming in systems developed in ASP.NET Core.
By understanding the concepts covered, you will be able to feel confident in creating scalable applications using object-oriented principles and the valuable features of C# and ASP.NET Core.
This content originally appeared on Telerik Blogs and was authored by Assis Zang
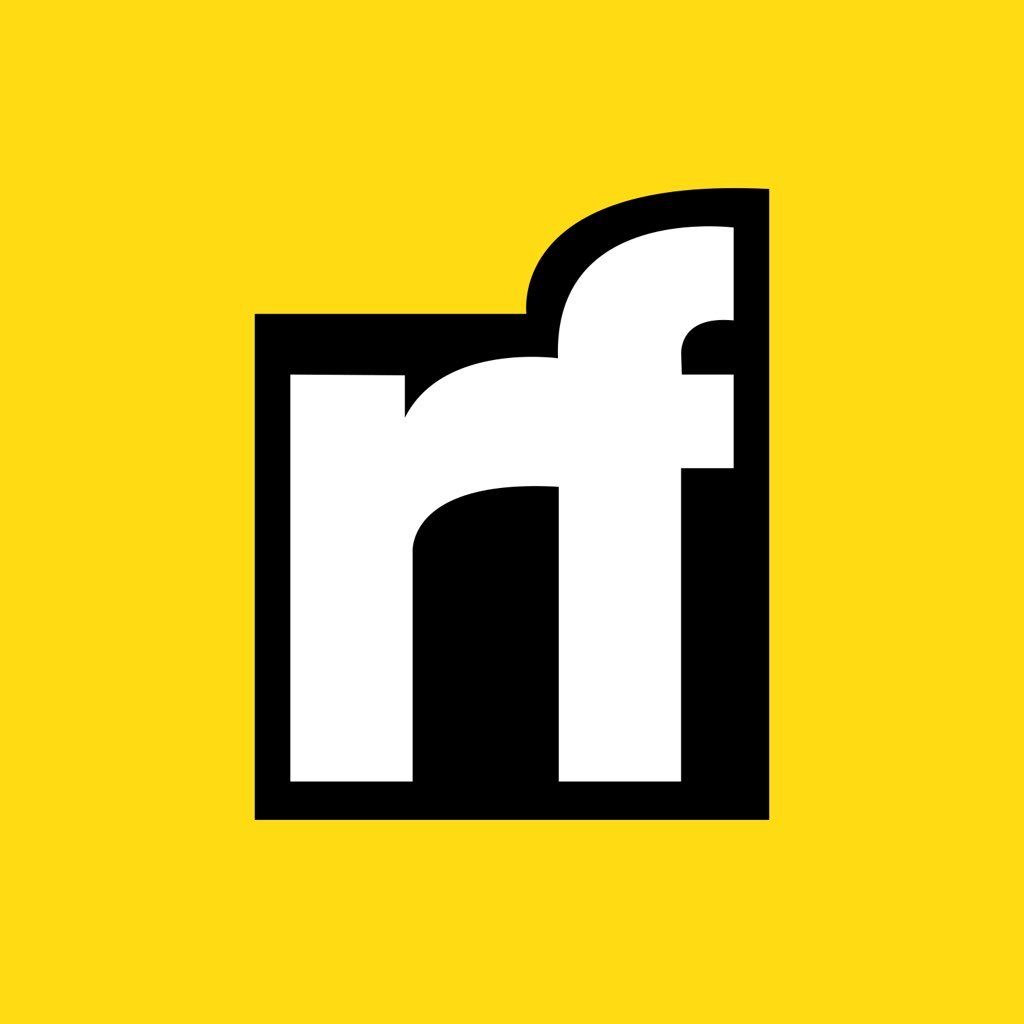
Assis Zang | Sciencx (2024-07-11T14:55:11+00:00) ASP.NET Core Basics: 12 Questions and Answers for Beginners. Retrieved from https://www.scien.cx/2024/07/11/asp-net-core-basics-12-questions-and-answers-for-beginners/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.