This content originally appeared on Level Up Coding - Medium and was authored by CyCoderX
Boost Code Quality and Efficiency Effortlessly
Type hinting in Python is a way to indicate the expected data types of variables, function arguments, and return values. Introduced in PEP 484 and significantly enhanced in subsequent PEPs, type hinting has become an essential feature in Python, especially for large codebases and collaborative projects.
Importance in Modern Python Development
Type hinting improves code clarity and maintainability, making it easier for developers to understand and collaborate on projects. It also aids in the use of development tools and IDEs, providing real-time feedback and error checking, which speeds up the development process and reduces bugs.
Target Audience and Article Goals
This article is tailored for tech professionals, developers, and tech enthusiasts who are familiar with Python and looking to deepen their understanding of type hinting. The goal is to provide a comprehensive guide that not only explains the basics but also dives into advanced topics, ensuring that readers can effectively implement type hinting in their projects.
Did you know that you can clap up to 50 times per article? Well now you do! Please consider helping me out by clapping and following me! 😊
Interested in more Python or SQL content? Don’t worry I got you covered! Feel free to explore some of my lists on medium!
Database SQL Sagas By CyCoderX
What is Type Hinting?
Definition and Basic Concept
Type hinting is a feature in Python that allows developers to specify the expected data types of variables, function parameters, and return values. Unlike statically typed languages, Python is dynamically typed, meaning variable types are determined at runtime. Type hinting provides a way to enforce type constraints during development, enhancing code readability and reliability.
History and Evolution in Python
Type hinting was first introduced in Python 3.5 with PEP 484, which laid the groundwork for adding type annotations to Python code. Prior to this, Python developers often relied on comments and docstrings to indicate types, which were less formal and prone to errors. Since PEP 484, several other PEPs (Python Enhancement Proposals) have expanded and refined type hinting, including PEP 526 for variable annotations and PEP 563 for postponed evaluation of type annotations.
Comparison with Other Languages
Type hinting in Python is optional and more flexible compared to statically typed languages like Java or C#. While those languages enforce type constraints at compile time, Python’s type hints serve as guidelines that can be checked by static type checkers like mypy but are not enforced at runtime. This approach maintains Python’s dynamic nature while providing the benefits of static typing.
Master the Python sys Module: Essential Guide
Looking to boost your Python coding expertises and examples? Explore my articles on essential Python exercises and mastering the sys module!
Benefits of Type Hinting
Improved Code Readability
Type hints act as a form of documentation, making it immediately clear what types of arguments a function expects and what type it returns. This clarity helps both the original developer and others who may work on the code later, reducing the learning curve and the potential for misunderstandings.
For example:
def add_numbers(a: int, b: int) -> int:
return a + b
Without type hints, it might not be immediately obvious that a and b should be integers and that the function returns an integer.
Enhanced Development Tools and IDE Support
Type hinting significantly improves the capabilities of Integrated Development Environments (IDEs) and code editors. Tools like PyCharm, VSCode, and others use type hints to provide features like:
- Autocomplete: Suggesting possible completions based on type hints.
- Inline Documentation: Showing type information when hovering over variables or function signatures.
- Error Highlighting: Identifying type mismatches during development.
These features streamline the development process, making it faster and more efficient to write correct code.
Early Error Detection and Debugging
By specifying types, you enable static type checkers like mypy to analyze your code and catch type-related errors before the program is run. This pre-runtime error detection is especially valuable in large codebases, where tracking down runtime errors can be challenging and time-consuming.
For example, consider the following code without type hints:
def concatenate(a, b):
return a + b
result = concatenate("Hello, ", 42) # This will raise an error at runtime
With type hints and a static type checker, this error would be caught early:
def concatenate(a: str, b: str) -> str:
return a + b
result = concatenate("Hello, ", 42) # mypy will flag this as an error
Must know Python Built-in Functions!
Interested in elevating your Python coding skills? Explore my articles on essential Python built-in functions and mastering f-strings for cleaner code!
Elevating Python Code with f-strings: The Definitive Guide
Basic Syntax and Examples
Simple Type Hints for Variables and Functions
Type hints can be added to both variables and function signatures to specify expected types.
For variables:
name: str = "Alice"
age: int = 30
is_active: bool = True
For functions:
def greet(name: str) -> str:
return f"Hello, {name}!"
Example Codes and Explanations
- Variables:
height: float = 5.9
is_student: bool = False
scores: list[int] = [85, 90, 78]
- height is expected to be a float.
- is_student is a boolean.
- scores is a list of integers.
2. Functions:
def add(a: int, b: int) -> int:
return a + b
- a and b are integers.
- The function returns an integer.
3. Function with multiple types:
from typing import Union
def process_data(data: Union[str, list[int]]) -> None:
if isinstance(data, str):
print(f"Processing string: {data}")
else:
print(f"Processing list of integers: {data}")
- data can be either a string or a list of integers.
- The function returns None.
Common Types and Their Annotations
Here are some commonly used types and their corresponding type annotations:
Basic Types:
- int
- float
- str
- bool
Collections:
- list[int]: List of integers
- tuple[str, int]: Tuple with a string and an integer
- dict[str, int]: Dictionary with string keys and integer values
- set[float]: Set of floats
Special Types:
- None: Represents the absence of a value
- Any: Represents any type
- Union: Represents multiple possible types
- Optional: Represents a value that could be of a specific type or None
Best Dataset Search Engines for Your Python Projects
Enjoying my article? I also have similar articles on various topics!
Master Python’s Enumerate for Efficient Coding
Advanced Type Hinting Techniques
Union, Optional, and Custom Types
- Union: The Union type allows a variable or function parameter to accept multiple types. It is useful when a value can be one of several types.
from typing import Union
def print_value(value: Union[int, str]) -> None:
print(f"The value is: {value}")
- Optional: Optional is a shorthand for Union with None. It indicates that a variable or parameter can be of a specified type or None.
from typing import Optional
def get_name(user_id: int) -> Optional[str]:
if user_id == 1:
return "Alice"
return None
- Custom Types: Custom types can be created using TypeVar for generics or TypedDict for more complex structures.
from typing import TypeVar, List
T = TypeVar('T')
def get_first_element(elements: List[T]) -> T:
return elements[0]
from typing import TypedDict
class User(TypedDict):
name: str
age: int
def print_user_info(user: User) -> None:
print(f"Name: {user['name']}, Age: {user['age']}")
Generics and Type Variables
Generics allow for more flexible and reusable code by parameterizing types. This is particularly useful for data structures like lists and dictionaries.
from typing import TypeVar, List
T = TypeVar('T')
def get_first_element(elements: List[T]) -> T:
return elements[0]
# Usage
print(get_first_element([1, 2, 3])) # Output: 1
print(get_first_element(["a", "b", "c"])) # Output: "a"
Callable, Any, and Other Complex Types
- Callable: The Callable type is used to specify the type of functions or callable objects.
from typing import Callable
def execute_function(func: Callable[[int, int], int], a: int, b: int) -> int:
return func(a, b)
# Usage
def add(x: int, y: int) -> int:
return x + y
print(execute_function(add, 2, 3)) # Output: 5
Any: The Any type is used when any type is acceptable. It effectively disables type checking for the annotated variable.
from typing import Any
def process_data(data: Any) -> None:
print(f"Processing data: {data}")
Other Complex Types: Python’s typing module includes other complex types like NewType, Literal, and Protocol, which can be used for more specific type constraints and validations.
5 Things I Wish I Knew Earlier in Python! 🐍
Interested in expanding your Python skills? Look no further! I have just the right articles for you!
Turbocharge Your Python with Lambda Functions
Type Hinting with Third-Party Libraries
Integrating with Popular Libraries
Many popular Python libraries now support type hinting, making it easier to use and integrate with them in type-annotated codebases. Here’s how type hinting can be used with some common libraries:
- NumPy: NumPy is a library for numerical computing in Python. To use type hints with NumPy arrays, the numpy.typing module provides useful types like NDArray.
import numpy as np
from numpy.typing import NDArray
def process_array(arr: NDArray[np.float64]) -> NDArray[np.float64]:
return arr * 2
my_array = np.array([1.0, 2.0, 3.0])
result = process_array(my_array)
2. Pandas: Pandas is a powerful data analysis and manipulation library. Type hinting with Pandas can be done using pd.DataFrame and pd.Series.
import pandas as pd
def summarize_data(data: pd.DataFrame) -> pd.Series:
return data.describe()
df = pd.DataFrame({
'A': [1, 2, 3],
'B': [4, 5, 6]
})
summary = summarize_data(df)
3. Requests: Requests is a library for making HTTP requests. While Requests does not have built-in type hints, you can still use standard types to annotate functions that work with it.
import requests
from typing import Dict
def fetch_data(url: str) -> Dict:
response = requests.get(url)
return response.json()
data = fetch_data('https://api.example.com/data')
Example Scenarios and Code Snippets
- Using Type Hints with Flask: Flask is a popular web framework. You can use type hints to improve the readability and maintainability of your route handlers.
from flask import Flask, request, jsonify
from typing import Dict
app = Flask(__name__)
@app.route('/add', methods=['POST'])
def add() -> Dict[str, int]:
data = request.json
result = data['a'] + data['b']
return jsonify({'result': result})
if __name__ == '__main__':
app.run()
2. Type Hints with SQLAlchemy: SQLAlchemy is an ORM for SQL databases. Use type hints to specify the types of your database models and query results.
from sqlalchemy import Column, Integer, String, create_engine
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from typing import List
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String)
engine = create_engine('sqlite:///:memory:')
Session = sessionmaker(bind=engine)
session = Session()
def get_all_users() -> List[User]:
return session.query(User).all()
# Example usage
Base.metadata.create_all(engine)
users = get_all_users()
Static Type Checkers
Introduction to mypy and Other Type Checkers
Static type checkers analyze your code to find type errors before runtime. mypy is the most widely used static type checker for Python, providing detailed feedback on type annotations.
mypy:
- Mypy checks your codebase against the specified type hints and reports any type inconsistencies.
- It’s simple to integrate into your development workflow, and it works with most popular IDEs.
Other static type checkers:
- Pyre: Developed by Facebook, Pyre is a fast type checker designed for large codebases.
- Pytype: Developed by Google, Pytype can infer types and check your code without requiring explicit type annotations.
How to Use Them in Your Development Workflow
- Installing mypy:
pip install mypy
- Running mypy: You can run mypy from the command line to check your Python files.
mypy your_script.py
- Configuring mypy: You can create a mypy.ini configuration file for more advanced settings.
[mypy]
python_version = 3.9
disallow_untyped_defs = True
ignore_missing_imports = True
- Integrating with IDEs:
VSCode: Install the Python extension, which supports mypy integration.
PyCharm: Configure mypy under the External Tools settings.
Examples and Best Practices
- Basic mypy Check:
def greet(name: str) -> str:
return f"Hello, {name}!"
print(greet("Alice"))
Run mypy to check for errors:
mypy your_script.py
2. Handling Complex Types:
from typing import List, Tuple
def process_data(data: List[Tuple[int, str]]) -> None:
for item in data:
print(item)
sample_data = [(1, 'apple'), (2, 'banana')]
process_data(sample_data)
3. Ignoring Specific Errors: Sometimes, you might need to ignore specific type errors.
from typing import Any
def risky_function() -> Any:
return "This might be anything"
value: int = risky_function() # type: ignore
- Incremental Typing: Start with type hints in critical parts of your codebase and gradually expand. Use # type: ignore to bypass errors temporarily.
- Using Type Comments: For backward compatibility with Python 2.x or for in-line type hints.
Interested in exploring NumPy for numerical operations and Pandas for data manipulation? Click the image above to boost your data science and computational skills!
Elevate Your Data Analysis Skills with Pandas
Common Pitfalls and Best Practices
Avoiding Common Mistakes
Incorrect Type Annotations: Ensure your type annotations correctly reflect the intended use of variables and functions. A mismatch between the annotated and actual types can lead to confusion and errors.
def add_numbers(a: int, b: int) -> str: # Incorrect return type
return a + b
Ignoring None in Optional Types: When using Optional, remember that None is a valid value. Always check for None before performing operations.
from typing import Optional
def greet(name: Optional[str]) -> str:
if name is None:
return "Hello, stranger!"
return f"Hello, {name}!"
Using Any Excessively: While Any can be useful, overusing it defeats the purpose of type hinting. Use specific types whenever possible to maintain type safety.
from typing import Any
def process_data(data: Any) -> None:
print(data) # Avoid using Any unless absolutely necessary
Tips for Effective Type Hinting
- Start with Critical Parts: Begin by adding type hints to the most critical and complex parts of your codebase. Gradually expand to cover the entire codebase.
- Leverage IDE Features: Utilize the features provided by your IDE for type hinting. Autocompletion, inline documentation, and error highlighting can significantly enhance your productivity.
- Use Type Aliases: For complex types that are used repeatedly, define type aliases to simplify your code.
from typing import List, Tuple
Coordinates = List[Tuple[int, int]]
def calculate_area(coords: Coordinates) -> float:
pass
4. Document with Type Hints: Type hints serve as a form of documentation. Use them to make your code self-explanatory, reducing the need for additional comments.
def find_user(user_id: int) -> Optional[User]:
"""
Fetches a user by their ID.
"""
pass
5. Regularly Run Type Checkers: Integrate static type checkers like mypy into your CI/CD pipeline to catch type errors early. This ensures type consistency across your codebase.
6. Stay Updated: Python’s type hinting system is continually evolving. Keep up with the latest developments and PEPs to leverage new features and best practices.
Real-World Examples and Case Studies
- Django Type Hinting: Django, a popular web framework, can benefit from type hinting, especially in models and views. Type hinting can clarify the types of query sets and model instances.
from django.http import HttpRequest, HttpResponse
from django.shortcuts import get_object_or_404
from .models import BlogPost
def blog_post_detail(request: HttpRequest, post_id: int) -> HttpResponse:
post: BlogPost = get_object_or_404(BlogPost, id=post_id)
return HttpResponse(f"Title: {post.title}")
2. Large Codebases: In large projects, type hinting helps maintain code quality and consistency. Companies like Dropbox and Instagram have successfully integrated type hinting and static type checking to manage their extensive Python codebases.
By adhering to these best practices and avoiding common pitfalls, you can effectively implement type hinting in your Python projects, leading to more robust, readable, and maintainable code.
Mastering the Art of System Design: Road to Architect
Conclusion
Recap of Key Points
Type hinting in Python is a powerful tool that brings several advantages to the development process. By specifying the expected types of variables, function arguments, and return values, type hinting improves code readability, enhances the capabilities of development tools, and facilitates early error detection through static type checking.
Throughout this article, we’ve explored:
- The basics of type hinting: Understanding its purpose and how it has evolved in Python.
- Benefits: From improved code clarity to better debugging and tool support.
- Syntax and Examples: Simple and advanced type hinting techniques.
- Integration with Third-Party Libraries: How to effectively use type hints with popular libraries like NumPy, Pandas, and Flask.
- Static Type Checkers: Using tools like mypy to enforce type constraints and catch errors early.
- Best Practices: Avoiding common mistakes and leveraging type hinting for better code quality.
Encouragement to Adopt Type Hinting
For tech professionals, developers, and tech enthusiasts, adopting type hinting in Python can lead to more reliable and maintainable codebases. It bridges the gap between Python’s dynamic nature and the safety benefits of static typing, offering a balanced approach that suits a wide range of projects.
Further Resources and Reading Recommendations
To deepen your understanding of type hinting and its applications, consider exploring the following resources:
- PEP 484: The foundational proposal for type hinting in Python.
- Mypy Documentation: Comprehensive guide on using mypy for static type checking.
- Typing Module Documentation: Detailed explanations and examples of Python’s typing module.
- Python Type Hints Cheat Sheet: A quick reference for common type hints and patterns.
Additionally, engaging with the Python community through forums, blogs, and conferences can provide valuable insights and practical tips for leveraging type hinting in your projects.
By integrating type hinting into your workflow, you can enhance the quality and maintainability of your code, making development more efficient and enjoyable.
Final Words:
Thank you for taking the time to read my article.
This article was first published on medium by CyCoderX.
Hey There! I’m CyCoderX, a data engineer who loves crafting end-to-end solutions. I write articles about Python, SQL, AI, Data Engineering, lifestyle and more!
Join me as we explore the exciting world of tech, data and beyond!
For similar articles and updates, feel free to explore my Medium profile
If you enjoyed this article, consider following for future updates.
Interested in Python content and tips? Click here to check out my list on Medium.
Interested in more SQL, Databases and Data Engineering content? Click here to find out more!
Happy Coding!
What did you think about this article? If you found it helpful or have any feedback, I’d love to hear from you in the comments below!
Supercharge Your Python Code with Type Hinting was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by CyCoderX
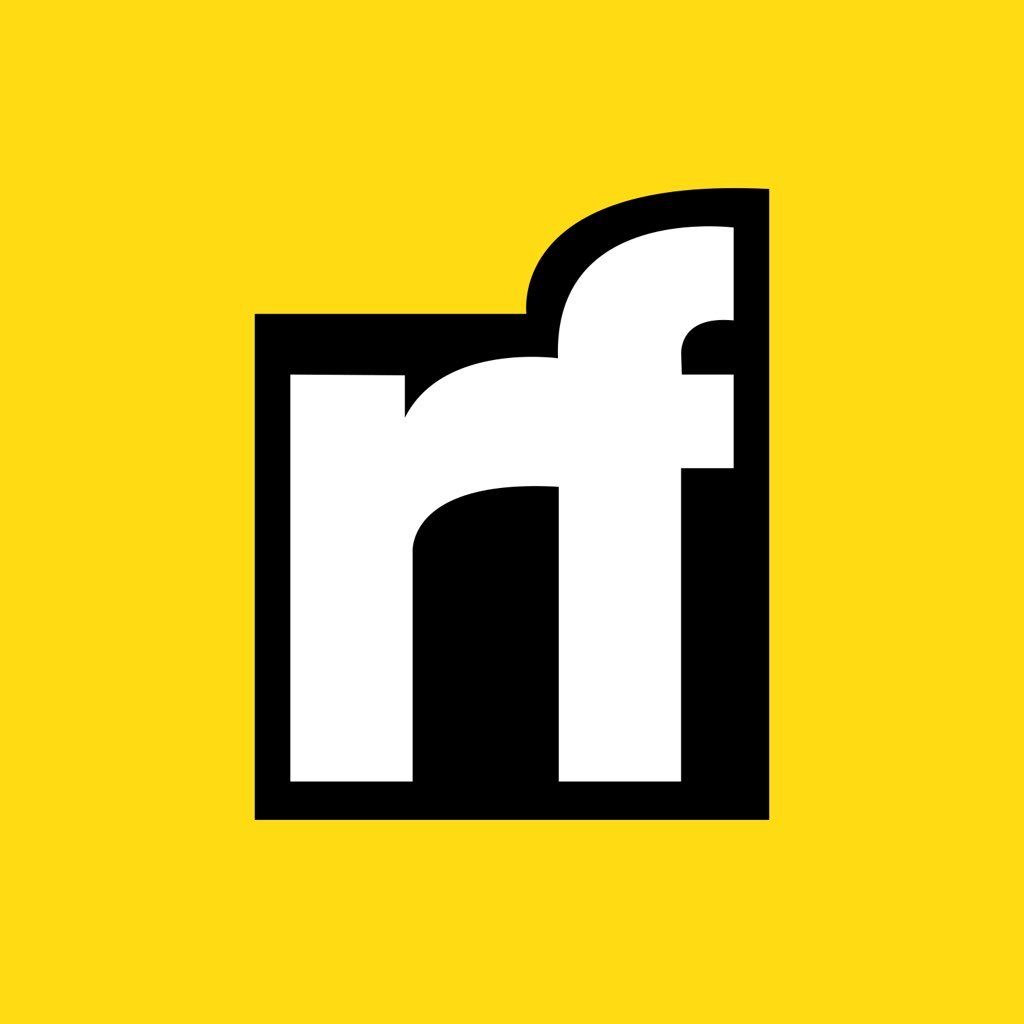
CyCoderX | Sciencx (2024-07-12T13:15:07+00:00) Supercharge Your Python Code with Type Hinting. Retrieved from https://www.scien.cx/2024/07/12/supercharge-your-python-code-with-type-hinting/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.