This content originally appeared on DEV Community and was authored by Shaikh AJ
Asynchronous programming is a key aspect of JavaScript that allows developers to perform long network requests, file operations, and other time-consuming tasks without blocking the main thread. This ensures that applications remain responsive and user-friendly. JavaScript provides three main ways to handle asynchronous operations: Callbacks, Promises, and Async/Await. In this article, we will delve into each of these methods, exploring their syntax, usage, and differences through detailed examples.
Table of Contents
- Introduction to Asynchronous Programming
-
Callbacks
- Syntax and Example
- Advantages and Disadvantages
-
Promises
- Syntax and Example
- Chaining Promises
- Advantages and Disadvantages
-
Async/Await
- Syntax and Example
- Error Handling
- Advantages and Disadvantages
- Comparison and Best Practices
- Conclusion
Introduction to Asynchronous Programming
In JavaScript, operations that take time to complete, such as fetching data from a server, reading files, or performing computations, can be handled asynchronously. This means that while waiting for an operation to complete, the JavaScript engine can continue executing other tasks. This is crucial for creating efficient and smooth user experiences in web applications.
Callbacks
Syntax and Example
Callbacks are one of the earliest methods for handling asynchronous operations in JavaScript. A callback is simply a function passed as an argument to another function, which will be executed once an asynchronous operation completes.
function fetchData(callback) {
setTimeout(() => {
callback("Data fetched!");
}, 1000);
}
function displayData(data) {
console.log(data);
}
fetchData(displayData);
In the example above, fetchData
simulates an asynchronous operation using setTimeout
. After 1 second, it calls the displayData
function, passing the fetched data as an argument.
Advantages and Disadvantages
Advantages:
- Simple and straightforward for small tasks.
- Provides a way to execute code after an asynchronous operation completes.
Disadvantages:
- Can lead to "callback hell" when multiple asynchronous operations are nested, making the code difficult to read and maintain.
- Error handling is cumbersome, as errors need to be managed in each callback.
Promises
Syntax and Example
Promises were introduced in ES6 (ECMAScript 2015) to address the issues associated with callbacks. A Promise represents an operation that hasn't completed yet but is expected in the future. It can be in one of three states: pending, fulfilled, or rejected.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched!");
}, 1000);
});
}
fetchData()
.then(data => {
console.log(data);
})
.catch(error => {
console.error("Error:", error);
});
In this example, fetchData
returns a Promise that resolves with "Data fetched!" after 1 second. The then
method is used to handle the resolved value, and catch
is used to handle any errors.
Chaining Promises
Promises can be chained to perform a series of asynchronous operations in sequence.
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched!");
}, 1000);
});
}
function processData(data) {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve(`${data} Processed!`);
}, 1000);
});
}
fetchData()
.then(data => {
console.log(data);
return processData(data);
})
.then(processedData => {
console.log(processedData);
})
.catch(error => {
console.error("Error:", error);
});
In this example, processData
is called after fetchData
, and the results are handled in sequence using then
.
Advantages and Disadvantages
Advantages:
- Avoids callback hell by allowing chaining of asynchronous operations.
- Provides built-in error handling with
catch
. - Improves code readability and maintainability.
Disadvantages:
- Can still become complex with multiple nested
then
calls. - Error handling in a chain can be non-trivial.
Async/Await
Syntax and Example
Async/Await, introduced in ES2017, provides a more readable and concise way to write asynchronous code using Promises. The async
keyword is used to define an asynchronous function, and the await
keyword is used to pause execution until a Promise is resolved.
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data fetched!");
}, 1000);
});
}
async function displayData() {
const data = await fetchData();
console.log(data);
}
displayData();
In this example, displayData
is an asynchronous function that waits for fetchData
to complete before logging the data.
Error Handling
Error handling with Async/Await can be done using try/catch blocks.
async function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
reject("Failed to fetch data!");
}, 1000);
});
}
async function displayData() {
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error("Error:", error);
}
}
displayData();
Here, if fetchData
fails, the error is caught and logged by the catch
block.
Advantages and Disadvantages
Advantages:
- Simplifies asynchronous code, making it look more like synchronous code.
- Enhances code readability and maintainability.
- Simplifies error handling with try/catch blocks.
Disadvantages:
- Requires understanding of Promises since Async/Await is built on top of them.
- Still relatively new, so some environments may not fully support it.
Comparison and Best Practices
Comparison
- Callbacks: Suitable for simple, single-level asynchronous tasks but can lead to callback hell in complex scenarios.
-
Promises: Improve readability and manageability, allowing chaining of asynchronous operations. Built-in error handling with
catch
. - Async/Await: Provides the most readable and maintainable way to write asynchronous code. Simplifies error handling and looks like synchronous code.
Best Practices
- Use Async/Await for most scenarios where you need to handle asynchronous operations. It provides the best readability and simplicity.
- Use Promises when you need to perform multiple asynchronous operations in sequence or when using libraries that return Promises.
- Avoid Callbacks unless working with legacy code or when dealing with very simple asynchronous tasks.
Conclusion
Asynchronous programming in JavaScript is essential for building responsive and efficient applications. Understanding the differences between Callbacks, Promises, and Async/Await allows developers to choose the right tool for their specific use case. While Callbacks are the simplest form of handling asynchronous operations, they can lead to messy code. Promises offer a more structured approach, but Async/Await provides the most elegant and readable solution. By following best practices and understanding these tools, developers can write clean, maintainable, and efficient asynchronous code.
This content originally appeared on DEV Community and was authored by Shaikh AJ
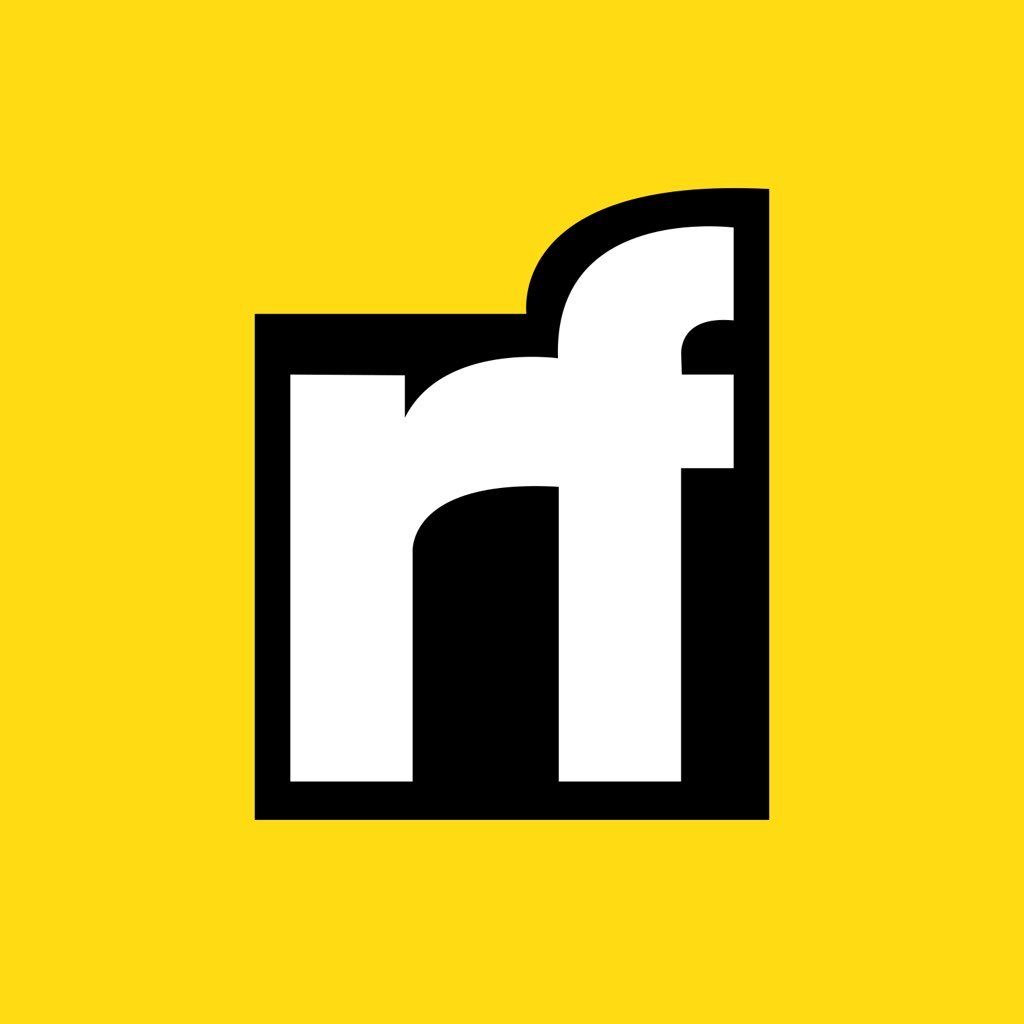
Shaikh AJ | Sciencx (2024-07-14T14:46:35+00:00) Asynchronous Programming in JavaScript: Callbacks vs Promises vs Async/Await. Retrieved from https://www.scien.cx/2024/07/14/asynchronous-programming-in-javascript-callbacks-vs-promises-vs-async-await/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.