This content originally appeared on DEV Community and was authored by ahmed elboshi
Beginner's Guide to Using if
Statements in Rust
The if
statement in Rust allows you to execute code conditionally, depending on whether an expression evaluates to true
or false
. This is similar to how if
statements work in Python, but with some syntax differences.
Basic if
Statement
In Python, you might write an if
statement like this:
Python:
x = 5
if x > 3:
print("x is greater than 3")
In Rust, the syntax is slightly different but serves the same purpose:
Rust:
fn main() {
let x = 5;
if x > 3 {
println!("x is greater than 3");
}
}
Breaking Down the Code
- Define the Variable:
let x = 5;
This creates a variable x
with the value 5
.
- If Statement:
if x > 3 {
println!("x is greater than 3");
}
This checks if x
is greater than 3
. If the condition is true
, it executes the code inside the curly braces.
if-else
Statement
You can add an else
block to execute code when the condition is false
.
Python:
x = 2
if x > 3:
print("x is greater than 3")
else:
print("x is not greater than 3")
Rust:
fn main() {
let x = 2;
if x > 3 {
println!("x is greater than 3");
} else {
println!("x is not greater than 3");
}
}
Breaking Down the Code
- If-Else Statement:
if x > 3 {
println!("x is greater than 3");
} else {
println!("x is not greater than 3");
}
If the condition x > 3
is true
, it executes the first block. Otherwise, it executes the code in the else
block.
else if
Statement
For multiple conditions, you can use else if
.
Python:
x = 5
if x > 10:
print("x is greater than 10")
elif x > 5:
print("x is greater than 5 but less than or equal to 10")
else:
print("x is 5 or less")
Rust:
fn main() {
let x = 5;
if x > 10 {
println!("x is greater than 10");
} else if x > 5 {
println!("x is greater than 5 but less than or equal to 10");
} else {
println!("x is 5 or less");
}
}
Breaking Down the Code
- Else If Statement:
if x > 10 {
println!("x is greater than 10");
} else if x > 5 {
println!("x is greater than 5 but less than or equal to 10");
} else {
println!("x is 5 or less");
}
This checks multiple conditions in sequence. It executes the first block where the condition is true
.
Using if
in a let
Statement
In Rust, you can use if
expressions to assign values.
Python:
x = 10
y = "greater than 5" if x > 5 else "5 or less"
Rust:
fn main() {
let x = 10;
let y = if x > 5 {
"greater than 5"
} else {
"5 or less"
};
println!("y is {}", y);
}
Breaking Down the Code
- If Expression in Let Statement:
let y = if x > 5 {
"greater than 5"
} else {
"5 or less"
};
This uses an if
expression to assign a value to y
based on the condition.
Conclusion
The if
statement in Rust is a powerful tool for controlling the flow of your program based on conditions. It is similar to if
statements in Python but with slight syntax differences. You can use if
, else if
, and else
to handle multiple conditions and even use if
expressions to assign values. Happy coding!
Python:
x = 2
if x > 3:
print("x is greater than 3")
else:
print("x is not greater than 3")
Rust:
fn main() {
let x = 2;
if x > 3 {
println!("x is greater than 3");
} else {
println!("x is not greater than 3");
}
}
Breaking Down the Code
- If-Else Statement:
if x > 3 {
println!("x is greater than 3");
} else {
println!("x is not greater than 3");
}
If the condition x > 3
is true
, it executes the first block. Otherwise, it executes the code in the else
block.
else if
Statement
For multiple conditions, you can use else if
.
Python:
x = 5
if x > 10:
print("x is greater than 10")
elif x > 5:
print("x is greater than 5 but less than or equal to 10")
else:
print("x is 5 or less")
Rust:
fn main() {
let x = 5;
if x > 10 {
println!("x is greater than 10");
} else if x > 5 {
println!("x is greater than 5 but less than or equal to 10");
} else {
println!("x is 5 or less");
}
}
Breaking Down the Code
- Else If Statement:
if x > 10 {
println!("x is greater than 10");
} else if x > 5 {
println!("x is greater than 5 but less than or equal to 10");
} else {
println!("x is 5 or less");
}
This checks multiple conditions in sequence. It executes the first block where the condition is true
.
Using if
in a let
Statement
In Rust, you can use if
expressions to assign values.
Python:
x = 10
y = "greater than 5" if x > 5 else "5 or less"
Rust:
fn main() {
let x = 10;
let y = if x > 5 {
"greater than 5"
} else {
"5 or less"
};
println!("y is {}", y);
}
Breaking Down the Code
- If Expression in Let Statement:
let y = if x > 5 {
"greater than 5"
} else {
"5 or less"
};
This uses an if
expression to assign a value to y
based on the condition.
Conclusion
The if
statement in Rust is a powerful tool for controlling the flow of your program based on conditions. It is similar to if
statements in Python but with slight syntax differences. You can use if
, else if
, and else
to handle multiple conditions and even use if
expressions to assign values. Happy coding!
This content originally appeared on DEV Community and was authored by ahmed elboshi
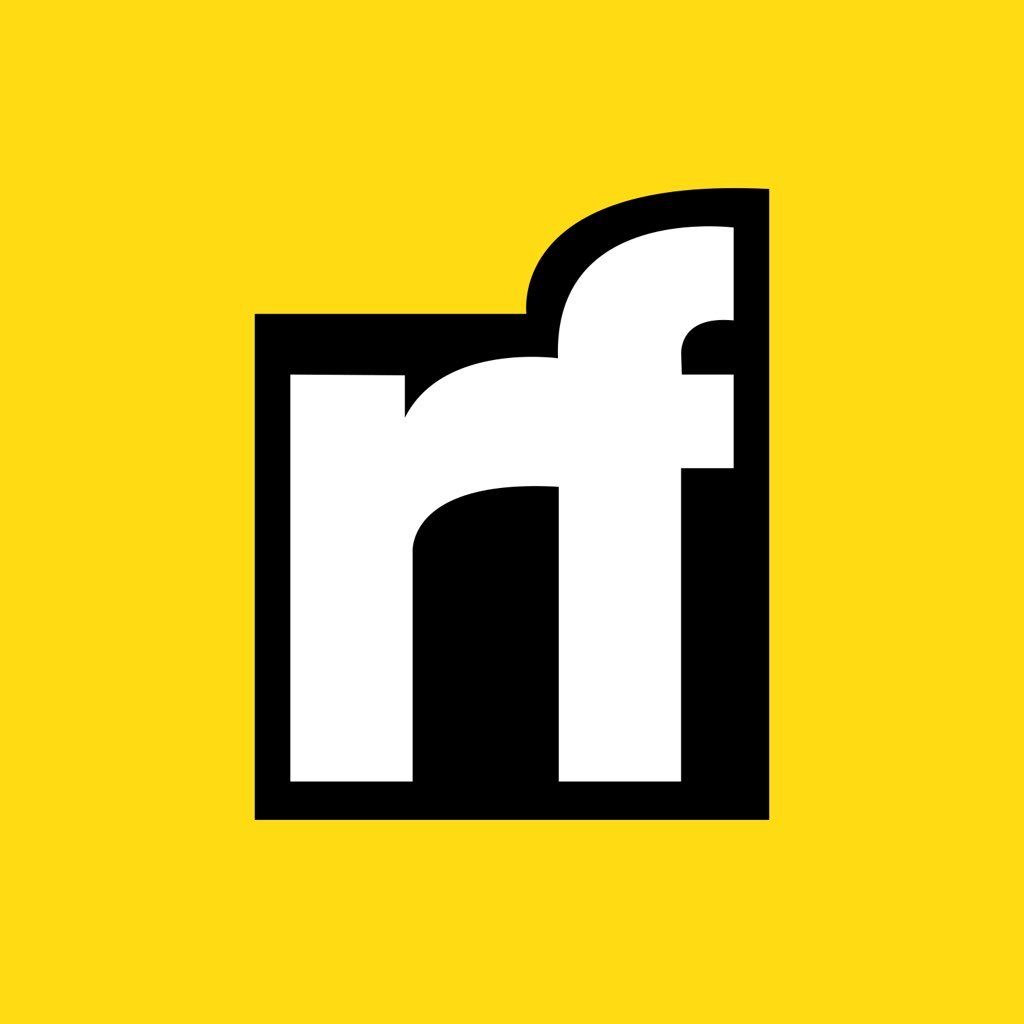
ahmed elboshi | Sciencx (2024-07-14T04:16:18+00:00) Rust tutorials for Python DEV: if statements. Retrieved from https://www.scien.cx/2024/07/14/rust-tutorials-for-python-dev-if-statements/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.