This content originally appeared on Level Up Coding - Medium and was authored by Rahul Beniwal
First Step to understand Python float weird behavior.
The IEEE 754 double-precision format is a widely adopted standard for representing floating-point numbers in computing. This format provides a balance between range and precision, using 64 bits to represent a floating-point number. These 64 bits are divided into three parts: the sign bit, the exponent, and the fraction (mantissa). Let’s break down this format and understand it with an example.
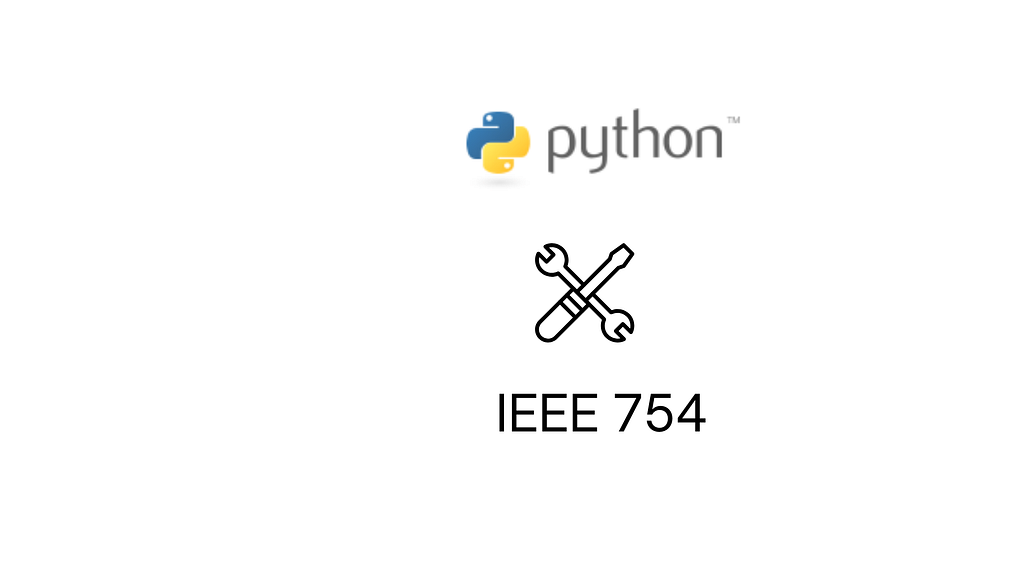
Components of the IEEE 754 Double-Precision Format
Sign Bit (1 bit)
- This bit determines whether the number is positive (0) or negative (1).
Exponent (11 bits)
- Encodes the power of two by which the mantissa is multiplied. The exponent is stored in a biased form, with a bias of 1023. This means the actual exponent is obtained by subtracting 1023 from the stored exponent value.
Fraction (Mantissa) (52 bits):
- Represents the significant digits of the number. The mantissa assumes an implicit leading 1 (unless the exponent is all zeros, indicating a de-normalized number).
Example: Representing 3.14 in IEEE 754 Double-Precision
Let’s represent the number 3.14 in IEEE 754 double-precision format.
Step 1: Convert to Binary
First, convert the number 3.14 to its binary representation:
- The integer part: 3 in binary is 11.
- The fractional part: 0.14 in binary is approximately 0.0010001111011 (repeating).
Combining these, we get:
3.14 (decimal) ≈ 11.0010001111011 (binary)
Step 2: Normalize the Binary Number
Normalize the binary number so it is in the form 1.xxxxx… * 2^n
11.0010001111011 (binary) = 1.10010001111011 * 2¹
Here, we’ve moved the binary point one position to the left, and we account for this by multiplying by 2¹.
Step 3: Determine the Sign, Exponent, and Mantissa
- Sign Bit: Since 3.14 is positive, the sign bit is
- Exponent: The exponent is 1 (from 2¹). To store this in the biased form:
Exponent (biased) = 1 + 1023 = 1024
The binary representation of 1024 is 10000000000.
- Mantissa: The mantissa is the fractional part of the normalized number (without the leading 1):
Mantissa = 10010001111011
To fit into 52 bits, we pad it with zeros:
Mantissa (52 bits) = 1001000111101100000000000000000000000000000000000000
Step 4: Combine the Components
Now, combine the sign bit, the exponent, and the mantissa:
- Sign Bit: 0
- Exponent: 10000000000
- Mantissa: 1001000111101100000000000000000000000000000000000000
Putting it all together, the IEEE 754 representation of 3.14 in double-precision floating-point format is:
0 10000000000 1001000111101100000000000000000000000000000000000000
Verifying the Normalized Form
To verify this representation, you can use Python to see the binary representation:
import struct
num = 3.14
binary_representation = struct.unpack('!Q', struct.pack('!d', num))[0]
binary_string = f"{binary_representation:064b}"
sign = binary_string[0]
exponent = binary_string[1:12]
mantissa = binary_string[12:]
print(f'Sign: {sign}')
print(f'Exponent: {exponent}')
print(f'Mantissa: {mantissa}')
Sign: 0
Exponent: 10000000000
Mantissa: 1001000111101011100001010001111010111000010100011111
Note that the mantissa here is slightly different from our manual calculation due to the approximation of the fractional part in binary.
Conclusion
The IEEE 754 double-precision format uses 1 bit for the sign, 11 bits for the exponent (with a bias of 1023), and 52 bits for the mantissa. This format allows for a wide range of values but also introduces precision limitations, especially with very large or very small numbers. Understanding these components and their limitations is crucial for numerical computations in Python.
If you found this article helpful, Please leave a 👏 and follow Rahul Beniwal
Other similar articles by me.
- Database Sharding 101 With Python.
- Kickstart Your Open Source Journey with Django and DRF Setup
- Decoding Python Magic -> Private Scope In Python Classes.
Understanding the IEEE 754 Double-Precision Floating-Point Representation was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Rahul Beniwal
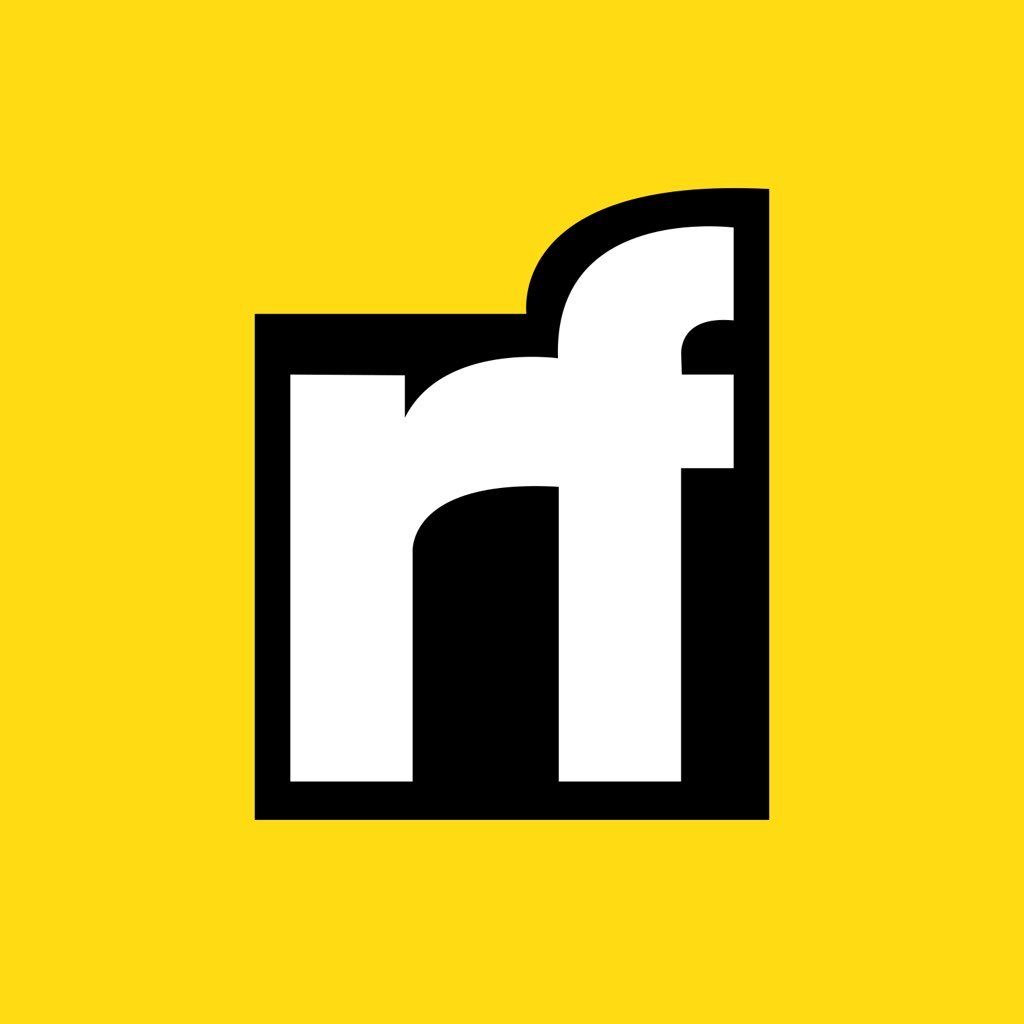
Rahul Beniwal | Sciencx (2024-07-16T16:31:52+00:00) Understanding the IEEE 754 Double-Precision Floating-Point Representation. Retrieved from https://www.scien.cx/2024/07/16/understanding-the-ieee-754-double-precision-floating-point-representation/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.