This content originally appeared on DEV Community and was authored by Sharoz Tanveer🚀
When discussing the most underrated features in TypeScript, one that often flies under the radar is the as const
assertion. This feature is incredibly useful in various scenarios, providing significant benefits for developers.
Understanding "as const"
To start, let's define what as const
does. Imagine you have an object like this:
const routes = {
home: "/home",
profile: "/profile",
notifications: "/notification",
};
If you hover over the properties of routes
, you'll notice they are typed as string
. For instance, routes.home
is typed as string
, not "/home"
. This is because TypeScript assumes these properties might change, so it infers them as mutable strings.
Now, consider a function that should accept only these specific routes. You might define the types like this:
function changeRoute(route: "home" | "profile" | "notifications") {
// navigate to route
}
This approach works, but it's repetitive and error-prone. If you add a new route, you must update the function's type definition, which isn't ideal.
Making Objects Immutable
Here's where as const
comes into play. By using as const
, you can make the object immutable, and TypeScript will infer the literal types instead of just string
.
const routes = {
home: "/home",
profile: "/profile",
notifications: "/notification",
} as const;
Now, routes.home
is typed as "/home"
, routes.notifications
as "/notifications"
, and so on. This immutability ensures that these properties cannot be changed, and TypeScript recognizes their specific values.
Practical Use Case
Let's integrate as const into a function:
function changeRoute(route: typeof routes[keyof typeof routes]) {
// navigate to route
}
Here, typeof routes
gets the type of the routes
object, and keyof typeof routes
extracts the keys, resulting in the exact types of the route values. This makes the function adaptable to any changes in the routes
object without the need for repetitive updates.
Extracting Types
Another powerful aspect of as const is how it enables type extraction. For instance:
type Routes = (typeof routes)[keyof typeof routes];
This type of definition dynamically extracts the values of the routes
object, making the function more maintainable and reducing redundancy.
Conclusion
The as const
assertion in TypeScript is a versatile and powerful feature that can significantly improve type safety and reduce redundancy in your code. By making objects immutable and enabling precise type inference, it simplifies maintaining and extending codebases. Give it a try, and you'll see how it can enhance your TypeScript projects!
Happy Coding!
[Disclosure: This article is a collaborative effort, combining my own ideas with the assistance of ChatGPT for enhanced articulation.]
This content originally appeared on DEV Community and was authored by Sharoz Tanveer🚀
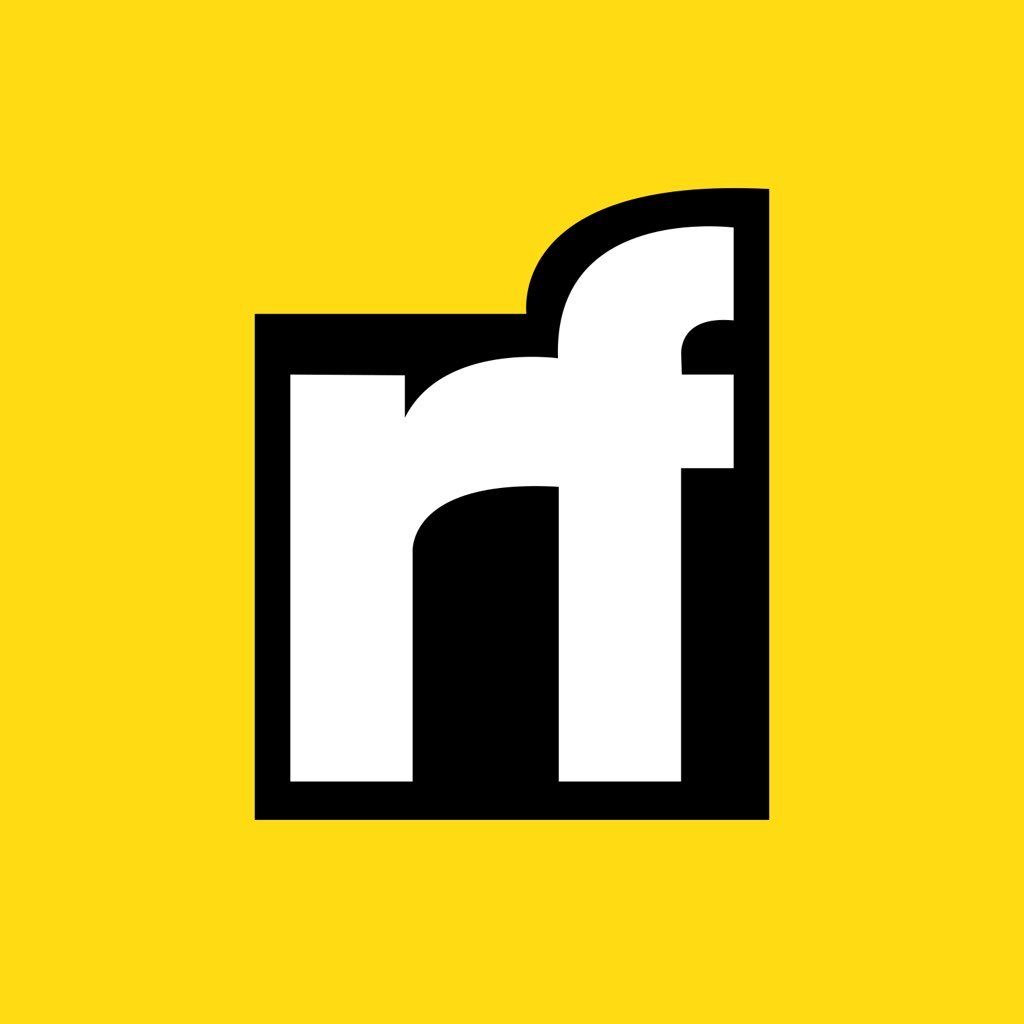
Sharoz Tanveer🚀 | Sciencx (2024-07-19T20:31:15+00:00) Unlocking the Power of TypeScript’s “as const”: The Underrated Feature You Need to Know. Retrieved from https://www.scien.cx/2024/07/19/unlocking-the-power-of-typescripts-as-const-the-underrated-feature-you-need-to-know/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.