This content originally appeared on DEV Community and was authored by Viraj Lakshitha Bandara
Taming Deeply Nested Components: Advanced Prop Drilling in React
In the realm of React development, passing data seamlessly between components is paramount for building robust and maintainable applications. While prop drilling, the process of passing props down through multiple levels of nested components, is a fundamental concept, it can quickly become cumbersome and inefficient as applications scale in complexity. Deeply nested component trees can lead to prop drilling nightmares, hindering development speed and code readability.
This article delves into advanced prop drilling techniques in React, equipping you with the knowledge and tools to navigate the complexities of data flow in intricate component hierarchies. We will explore various strategies and best practices to streamline prop management and enhance the efficiency of your React projects.
Understanding the Basics: Prop Drilling in React
Before we delve into advanced techniques, let's briefly recap the fundamentals of prop drilling. At its core, prop drilling is the mechanism by which data is passed down from a parent component to its children through props. Each level in the hierarchy receives the data and passes it down until it reaches the intended recipient.
Consider a simple example of a component tree displaying user profile information:
// App.js
function App() {
const user = { name: 'John Doe', email: 'john.doe@example.com' };
return (
<div>
<Profile user={user} />
</div>
);
}
// Profile.js
function Profile({ user }) {
return (
<div>
<h2>{user.name}</h2>
<Email email={user.email} />
</div>
);
}
// Email.js
function Email({ email }) {
return <p>{email}</p>;
}
In this scenario, the user
object originates in the App
component and is passed down to the Profile
component. Subsequently, the email
property of the user
object is further passed down to the Email
component. This sequential passing of data through props is the essence of prop drilling.
When Prop Drilling Becomes a Challenge
While prop drilling is straightforward for simple component structures, it can quickly become problematic in complex applications with deeply nested component trees. As the number of levels in the hierarchy increases, so does the burden of passing props through each intermediary component. This can lead to:
- Verbose and repetitive code: Passing the same props through multiple levels results in redundant code, making it harder to maintain and understand.
- Reduced code readability: Deeply nested prop drilling obscures the flow of data, making it challenging to track how information is being passed between components.
- Increased risk of errors: With more components involved in prop passing, the likelihood of introducing errors or inconsistencies in the data flow rises.
Advanced Techniques for Efficient Prop Management
To overcome the challenges posed by prop drilling in large-scale React applications, developers have devised various advanced techniques for efficient prop management. Let's explore some of the most effective strategies:
1. Composition Over Inheritance
React's component-based architecture emphasizes composition over inheritance as a fundamental principle. Instead of creating deeply nested component hierarchies, consider breaking down your components into smaller, more reusable units. By composing these smaller components, you can effectively reduce the depth of your component tree and minimize the need for excessive prop drilling.
For instance, instead of passing the entire user
object down multiple levels, you could create separate components for displaying the user's name, email, and other profile details. These components can then be independently composed within the Profile
component, reducing the scope of prop drilling.
2. Context API for Global State Management
When data needs to be accessible by components situated in disparate branches of the component tree, prop drilling can become particularly cumbersome. React's Context API provides an elegant solution by allowing you to share data globally without the need for manual prop drilling through every intermediary level.
The Context API involves three key elements:
- Context: A container that holds the global state data.
- Provider: A component that makes the context value available to its descendants.
- Consumer: A component that accesses the context value.
By wrapping the relevant portion of your component tree with a Provider
, you can make the shared data accessible to all its children without explicitly passing it as props. Consumers within this subtree can then access and utilize the context value directly.
3. State Management Libraries (Redux, Zustand)
For large-scale applications with complex state management requirements, dedicated state management libraries such as Redux and Zustand offer powerful solutions. These libraries provide a centralized store for managing global application state, eliminating the need for prop drilling altogether.
Redux, a widely adopted library, employs a unidirectional data flow architecture. Actions are dispatched to update the store, and components subscribe to relevant parts of the store to receive state updates. Zustand, a more lightweight alternative, offers a simpler API while maintaining efficiency and scalability.
4. Render Props for Data Sharing and Logic Reuse
Render props, a powerful pattern in React, allow you to pass functions as props, effectively sharing logic and data between components. This technique is particularly useful when you need to reuse a specific behavior or data retrieval logic across multiple parts of your application.
By passing a function as a render prop, a parent component can delegate rendering responsibility to its child while still providing access to its internal state or methods. This promotes code reusability and avoids unnecessary prop drilling.
5. Higher-Order Components (HOCs)
Similar to render props, Higher-Order Components (HOCs) provide a mechanism for code reuse and logic abstraction. An HOC is a function that takes a component as input and returns a new component with enhanced functionality.
By wrapping a component with an HOC, you can inject additional props, state, or lifecycle methods without modifying the original component's code. This is particularly beneficial for cross-cutting concerns such as authentication, data fetching, or logging.
Exploring Alternative Solutions
Beyond the realm of React, other front-end frameworks and libraries offer their own mechanisms for data binding and state management:
-
Vue.js: Vue's reactivity system automatically tracks dependencies between components, eliminating the need for manual prop drilling in many scenarios. Its
provide/inject
mechanism offers a similar functionality to React's Context API. - Angular: Angular's hierarchical dependency injection system provides a structured approach to sharing data and services across components. Components declare their dependencies, and the framework resolves them at runtime.
Conclusion
Efficiently managing data flow in React applications is crucial for maintainability and scalability. While prop drilling serves as the foundation for passing props between components, relying solely on this technique for complex applications can lead to code verbosity, reduced readability, and increased risk of errors.
By embracing advanced techniques such as component composition, the Context API, state management libraries, render props, and HOCs, you can streamline prop management and enhance the overall efficiency of your React projects. By choosing the right tool for the right job and carefully considering the trade-offs of each approach, you can write cleaner, more maintainable, and scalable React applications.
Advanced Use Case: Building a Collaborative Real-Time Editor with AWS
Let's imagine we're building a collaborative real-time editor, similar to Google Docs, using React and AWS. We need to handle real-time updates, user presence, and data persistence. Here's how we can leverage various AWS services and advanced prop drilling techniques:
1. WebSockets for Real-Time Communication
AWS API Gateway with WebSockets provides a scalable and managed solution for handling real-time, bidirectional communication between the client and the server. We can establish a WebSocket connection between the editor and API Gateway, allowing for instant propagation of changes.
2. DynamoDB for Data Persistence
Amazon DynamoDB, a fully managed NoSQL database, can store the document content. Its low latency and high throughput make it suitable for real-time updates. We can leverage DynamoDB Streams to capture data modifications and trigger subsequent actions.
3. AWS Lambda for Serverless Backend
Lambda functions can power the serverless backend, handling WebSocket events from API Gateway and interacting with DynamoDB to persist changes and retrieve the latest document state. Lambda's scalability and pay-as-you-go model make it cost-effective for real-time applications.
4. Shared Context for Real-Time Updates
Within our React application, we can use the Context API to create a shared context for the document content and user presence. This context will be updated whenever a WebSocket message is received from API Gateway, reflecting the latest document state and user activity.
5. Custom Hooks for Data Fetching and Synchronization
Custom React Hooks can encapsulate the logic for connecting to the WebSocket, subscribing to document updates, sending changes to the server, and updating the shared context. These hooks can be reused across different components, promoting code reusability and separation of concerns.
6. Optimistic Updates for Enhanced User Experience
To provide a seamless editing experience, we can implement optimistic updates. When a user makes a change, we can immediately update the local state and UI while sending the changes to the server asynchronously. If the server acknowledges the update, we can maintain consistency. If there's a conflict, we can implement conflict resolution mechanisms.
By combining these AWS services and advanced React techniques, we can build a robust, scalable, and performant collaborative real-time editor that provides a seamless user experience. The use of a shared context ensures that all components have access to the latest document state, while custom hooks encapsulate the complexity of WebSocket communication and data synchronization.
This content originally appeared on DEV Community and was authored by Viraj Lakshitha Bandara
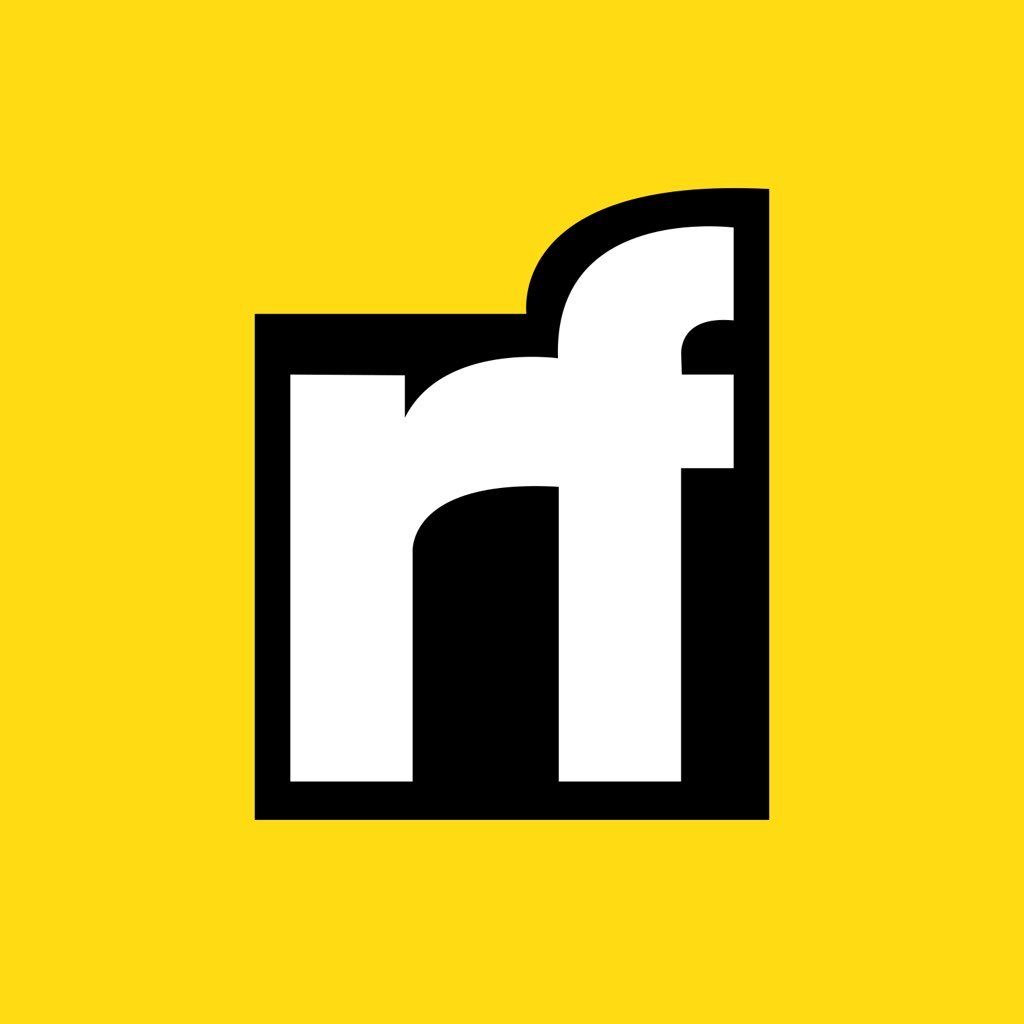
Viraj Lakshitha Bandara | Sciencx (2024-07-20T03:05:02+00:00) Taming Deeply Nested Components: Advanced Prop Drilling in React. Retrieved from https://www.scien.cx/2024/07/20/taming-deeply-nested-components-advanced-prop-drilling-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.