This content originally appeared on DEV Community and was authored by El Morjani Mohamed
As developers, we often need to extract only a few items from a validated request. Instead of manually unsetting or filtering, Laravel provides a clean and efficient way to do this using the safe() method. Here’s how:
// Extract only specific fields
$validated = $request->safe()->only(['name', 'email']);
// Extract all fields except specified ones
$validated = $request->safe()->except(['name', 'email']);
// Extract all validated input
$validated = $request->safe()->all();
Example Usage:
use Illuminate\Http\Request;
public function store(Request $request)
{
// Validate the incoming request data
$validated = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email',
'password' => 'required|string|min:8|confirmed',
'role' => 'required|string',
]);
// Extract only the 'name' and 'email' fields
$nameAndEmail = $request->safe()->only(['name', 'email']);
// Extract all validated input except 'name' and 'email'
$exceptNameAndEmail = $request->safe()->except(['name', 'email']);
// Extract all validated input
$allValidated = $request->safe()->all();
// Use the extracted data as needed
// For example, creating a new user with only the 'name' and 'email'
$user = User::create($nameAndEmail);
// Continue with the rest of your logic
}
Using safe() makes your code cleaner and ensures you're only working with validated data, enhancing both security and code clarity.
This content originally appeared on DEV Community and was authored by El Morjani Mohamed
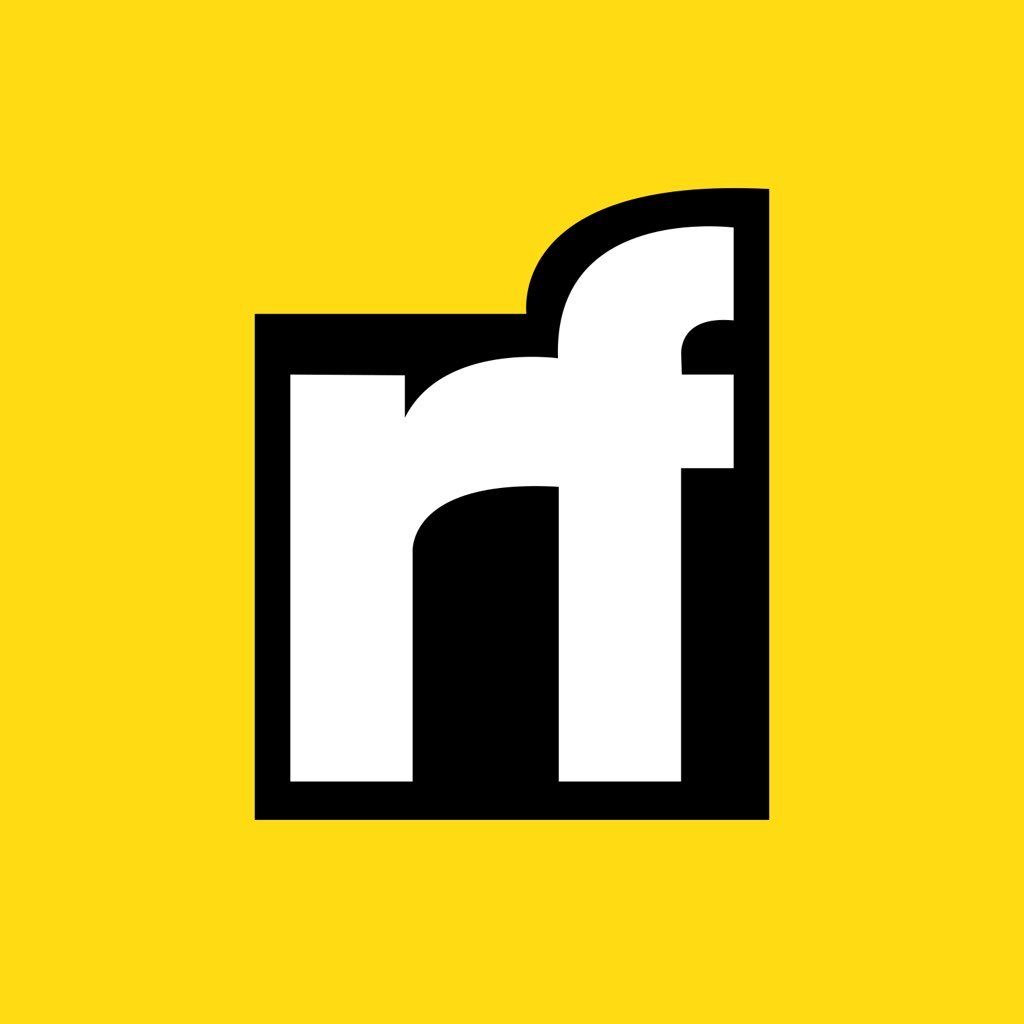
El Morjani Mohamed | Sciencx (2024-07-21T18:26:54+00:00) 🚀 Laravel Tip💡: Extract Validated Input Elegantly. Retrieved from https://www.scien.cx/2024/07/21/%f0%9f%9a%80-laravel-tip%f0%9f%92%a1-extract-validated-input-elegantly/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.