This content originally appeared on DEV Community and was authored by Iñigo Etxaniz
Introduction
When developing Rust applications, error handling is a crucial aspect that can significantly impact the debugging process and overall maintainability of your code. While Rust's error messages are generally informative about why an error occurred, they often fall short in providing insight into the program's flow leading up to that error. In complex applications with multiple layers of function calls, understanding the sequence of events that led to an error can be challenging.
To address this challenge, I have developed an approach that enhances Rust's error handling capabilities by incorporating program flow information directly into error messages. This method combines custom traits, macros, and the anyhow
crate to provide a more comprehensive error reporting system.
Here's a snippet that demonstrates how clean and straightforward the implementation can be:
pub fn run() -> Result<()> {
let input = debug_err!(parse_argument(), "Error parsing command-line argument")?;
let result = debug_err!(intermediate_function(input))?;
println!("Success: {}", result);
Ok(())
}
As you can see, this approach allows you to add debug information to your error handling with minimal impact on your code's readability. The debug_err!
macro seamlessly integrates with Rust's ?
operator, maintaining the concise style that Rust developers appreciate. It also provides flexibility by allowing optional custom error messages:
let result = debug_err!(some_operation())?; // Adds file and line information
let result = debug_err!(some_operation(), "Custom error message")?; // Adds custom message along with file and line
Let's look at how this solution works in practice with a few examples:
- Successful execution:
$ cargo run -- 12
Finished `dev` profile [unoptimized + debuginfo] target(s) in 0.00s
Running `target/debug/rust_error_handling 12`
Success: you guessed correctly
- Error in main operation:
$ cargo run -- 89
Finished `dev` profile [unoptimized + debuginfo] target(s) in 0.00s
Running `target/debug/rust_error_handling 89`
Error:
(at src/some_module/mod.rs:28): Error in intermediate_function
(at src/some_module/mod.rs:23): Invalid input: 89
- Error in parsing command-line argument:
$ cargo run -- io
Finished `dev` profile [unoptimized + debuginfo] target(s) in 0.00s
Running `target/debug/rust_error_handling io`
Error: Error parsing command-line argument
(at src/some_module/mod.rs:27): Failed to parse input as integer: invalid digit found in string
As you can see, in cases where an error occurs, our enhanced error messages provide the reason for the error along with the file and line number, offering a clear picture of how the program reached the error state. The custom messages, when provided, offer additional context to aid in debugging.
In the following sections, we'll dive into the implementation details of this error handling approach, explore its benefits and potential drawbacks, and discuss best practices for incorporating it into your Rust projects.
Implementation and Example
Let's walk through the implementation of our enhanced error handling approach and demonstrate its usage with a practical example. We'll start by creating a new Rust project and then build our error handling system step by step.
Begin by creating a new Rust project and adding the necessary dependency:
cargo new rust_error_handling_example
cd rust_error_handling_example
cargo add anyhow
This will create a new project and add anyhow
to your Cargo.toml
file. Now, let's set up our project structure. Create the following directories and files:
src/
|-- error_handling/
| |-- mod.rs
|-- some_module/
| |-- mod.rs
|-- main.rs
Now that our project is set up, let's implement our core error handling logic. Create src/error_handling/mod.rs
and add the following code:
use anyhow::{Context, Result};
pub trait AddDebugInfo<T> {
fn add_debug_info(self, context: Option<&str>, file: &str, line: u32) -> Result<T>;
}
impl<T> AddDebugInfo<T> for Result<T> {
fn add_debug_info(self, context: Option<&str>, file: &str, line: u32) -> Result<T> {
self.with_context(move || {
if let Some(ctx) = context {
format!("{}\n(at {}:{})", ctx, file, line)
} else {
format!("\n(at {}:{})", file, line)
}
})
}
}
#[macro_export]
macro_rules! debug_err {
($result:expr) => {
$result.add_debug_info(None, file!(), line!())
};
($result:expr, $msg:expr) => {
$result.add_debug_info(Some(&format!($msg)), file!(), line!())
};
}
This code defines the AddDebugInfo
trait and implements it for Result<T>
. The debug_err!
macro provides a convenient way to add debug information to errors, with or without custom messages.
Next, let's create a module that demonstrates our error handling approach. Create src/some_module/mod.rs
, with following code:
use anyhow::{Context, Result, anyhow};
use std::env;
use crate::error_handling::AddDebugInfo;
use crate::debug_err;
pub fn parse_argument() -> Result<i32> {
let args: Vec<String> = env::args().collect();
if args.len() != 2 {
return Err(anyhow!("Expected exactly one argument"));
}
args[1].parse::<i32>()
.context("Failed to parse input as integer")
}
pub fn some_fallible_operation(input: i32) -> Result<String> {
match input {
12 => Ok("you guessed correctly".to_string()),
_ => Err(anyhow!("Invalid input: {}", input)),
}
}
pub fn intermediate_function(input: i32) -> Result<String> {
debug_err!(some_fallible_operation(input), "Error in intermediate_function")
}
pub fn run() -> Result<()> {
let input = debug_err!(parse_argument(), "Error parsing command-line argument")?;
let result = debug_err!(intermediate_function(input))?;
println!("Success: {}", result);
Ok(())
}
This module demonstrates various ways to use our error handling pattern. In parse_argument()
, we use the standard context()
method from anyhow
to add a simple error message. In intermediate_function()
, we use debug_err!
with a custom message. The run()
function shows how to chain multiple operations using debug_err!
, adding contextual information at each step.
Finally, update src/main.rs
to use our new module:
mod error_handling;
mod some_module;
fn main() {
if let Err(e) = some_module::run() {
eprintln!("Error: {:#}", e);
std::process::exit(1);
}
}
Now you can run the example with different inputs to see how the error handling works:
cargo run -- 12
cargo run -- 89
cargo run -- io
When an error occurs, it will be displayed with the added context and location information, making it easier to trace and debug issues in your Rust applications. This pattern allows you to add contextual information at each step, creating a trail of debug information that helps trace the error's origin and path through the program.
Reflections on the Approach
Coming from a Go background, I often find myself struggling with error handling in Rust. Despite Rust's powerful type system and the Result
type, I frequently encounter situations where tracing the origin and path of errors through my codebase is more challenging than it should be. This struggle has led me to explore alternative approaches, ultimately resulting in the error handling pattern presented in this article.
The primary motivation behind developing this approach is to enhance the debugging process in my Rust applications. I want a method that can provide detailed context about where and how errors occur, significantly reducing the time and effort required to trace and resolve issues. After implementing this pattern, I have decided to change how I manage errors in my future Rust projects and potentially refactor existing codebases to incorporate this new approach.
One of the most compelling aspects of this method is the automatic inclusion of file and line information in error messages. I anticipate this feature will be particularly valuable when dealing with complex codebases, as it should immediately pinpoint the exact location where an error originates or is propagated. The flexibility to add optional custom messages also allows for the inclusion of rich, contextual information about the program's state at the time of the error.
The debug_err!
macro is a key component of this new approach. I have designed it to integrate seamlessly with Rust's ?
operator, allowing for concise error propagation while still maintaining detailed error context. My hope is that this will lead to more readable and maintainable code, as error handling becomes less intrusive and more informative.
However, I'm aware that this approach isn't without potential drawbacks. There might be a slight performance overhead associated with capturing and storing additional context information. While I expect this overhead to be negligible in most applications, it could become a consideration in performance-critical sections of code or in systems with extremely high throughput.
As I move forward with adopting this pattern, I plan to use it judiciously and in balance with other error handling techniques. For instance, I intend to use debug_err!
with custom messages at significant points in the program flow or when crossing module boundaries. For simpler, more localized error cases, I might stick with anyhow
's standard context
method or even Rust's built-in ?
operator without additional context.
When it comes to balancing detail and performance, I'm considering a strategy of using more detailed error messages in development and testing environments, while potentially scaling back the verbosity in production builds if performance becomes a concern. This could be achieved through conditional compilation or runtime flags.
Integrating this approach with existing error handling patterns in my projects will require careful consideration. In codebases that already have established error types or handling mechanisms, I plan to gradually introduce this method, starting with areas that would benefit most from enhanced debugging information.
While I haven't extensively used this error handling approach in production yet, I'm optimistic about its potential to improve my Rust development experience. I look forward to seeing how it performs in real-world scenarios and how it might evolve based on practical usage. As with any new technique, I expect there will be a learning curve and possibly some adjustments needed along the way. However, I believe the potential benefits in terms of improved debugging capabilities and code maintainability make it worth exploring and implementing in my future Rust projects.
Conclusion
The enhanced error handling approach I've presented in this article offers a solution to a challenge I've often encountered in Rust development. By incorporating program flow information directly into error messages, I believe I can significantly improve my debugging process and the overall maintainability of my Rust applications. This method provides detailed context by automatically including file and line information in error messages, while offering the flexibility of optional custom messages for rich, contextual information. I've designed it to integrate seamlessly with Rust's ?
operator and existing error handling patterns, ensuring that I can adopt this approach without disrupting my current coding practices. I anticipate this will result in a substantial reduction in the time and effort required to trace and resolve issues, especially in the complex codebases I work with. While I'm aware of potential minor performance considerations in certain scenarios, I believe the improvements in code readability, maintainability, and debugging efficiency will make this approach a valuable addition to my Rust developer toolkit. As I prepare to implement and refine this error handling pattern in my real-world projects, I look forward to gaining practical insights and making further optimizations. My goal is to make my Rust development more efficient and enjoyable, allowing me to focus on solving problems rather than tracking down elusive errors. By sharing this approach, I hope other Rust developers might find it useful and potentially benefit from it in their own work as well.
I am not an expert in Rust, but I've adapted principles I found useful in Go to the Rust ecosystem. I hope this method proves helpful to others facing similar challenges in debugging and maintaining Rust applications. If you have more experience in Rust or suggestions for improving this approach, I would greatly appreciate your feedback. Let's continue to evolve and refine our error handling techniques together.
This content originally appeared on DEV Community and was authored by Iñigo Etxaniz
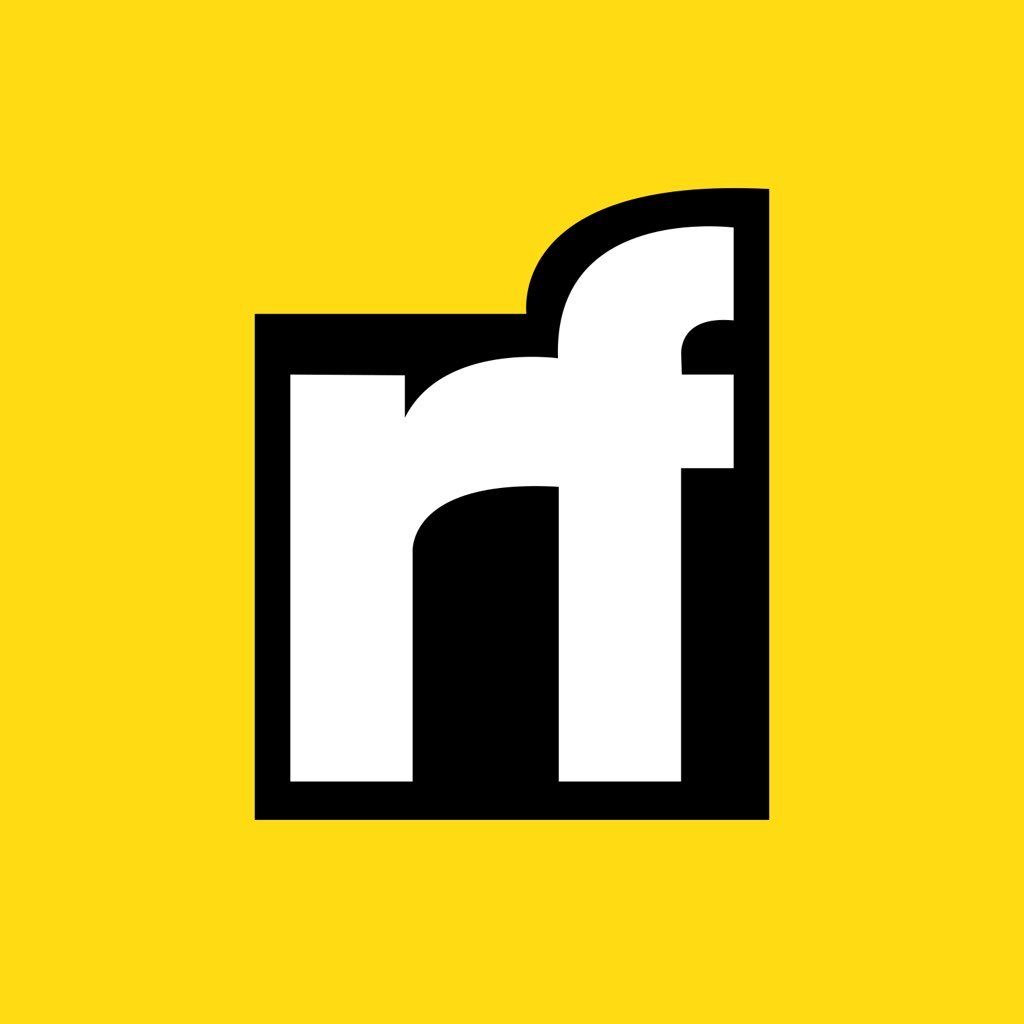
Iñigo Etxaniz | Sciencx (2024-07-21T09:01:28+00:00) Enhancing Rust Error Handling: Macro to add Program Flow Trace to your applications. Retrieved from https://www.scien.cx/2024/07/21/enhancing-rust-error-handling-macro-to-add-program-flow-trace-to-your-applications/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.