This content originally appeared on Telerik Blogs and was authored by Claudio Bernasconi
Learn how to add context-sensitive page titles and add metadata to HTML pages using Blazor, which is helpful for SEO on public-facing websites.
With .NET 8 and the new Blazor Web App project template, server pre-rendering is enabled by default. It means that the server sends an HTML document to the client before the webpage has completely loaded.
This feature helps with search engine optimization (SEO) by improving the first-page load speed. The head section of an HTML document becomes important when building websites or applications that are publicly exposed.
What Goes into the HTML Head Section?
Let’s quickly take a step back and consider why we should care about the head section of the HTML document. What should we put in it?
- Page title: The most important information inside the head section is definitely the page title. In HTML, we simply use the
<title>
tag inside the head section to define the page title. Luckily, there is a very convenient built-in solution to define a page title for a Blazor page. - Linking CSS and JavaScript files: With isolated CSS and the
site.css
we have an infrastructure in Blazor that allows us to add CSS wherever we need it without linking additional CSS files in the head section. We also don’t need to link additional JavaScript files. - Metadata: Typically not displayed in the browser but used by browsers, metadata allows us to define information relevant to search engines. Some information needs to be the same for all pages, but we might want to change a few of them depending on what page the user is.
The Page Title
Blazor offers the PageTitle
component from the Microsoft.AspNetCore.Components.Web namespace that allows us to set the page title from inside a Blazor page.
Consider the following example:
@page "/about"
<PageTitle>Software Wizardry - About Us</PageTitle>
<h3>About Us</h3>
<p>We are a team of highly motivated software developers focused on delivering sustainable and modern software solutions.</p>
In this article page component, we use the PageTitle
component and provide a static string as its value.
We could also use properties or variables defined in the component as part of the PageTitle
content, for example, by using string interpolation.
PageTitle Inside a Child Component
Even though it’s best to define the PageTitle
directly inside a page component, we could also define it inside a child component.
What sounds strange also risks causing confusion about where and what page title is set. However, sometimes, we want to include context-sensitive information inside the page title that is not available on the page component.
This might be the case where putting the PageTitle
component inside a child component is a simple and clean way to solve the issue.
Consider the following example of an Article
page component:
@page "/article/{ArticleId:int}"
@using BlazorHead.Client.Components
<PageTitle>Software Wizardry</PageTitle>
<h1>Amazing Blog Post Title</h1>
<Author ArticleId=@ArticleId />
<p>Amazing Content</p>
@code {
[Parameter]
public int ArticleId { get; set; }
}
We receive the ArticleId
property as a parameter when navigating to the page. You can see the parameter definition in the @page
directive.
Hint: Learn more about component parameters and state management in another article of the Blazor Basics series.
Next, we set the PageTitle
to a context-insensitive, static title. However, notice the Author
component. Let’s take a closer look at it:
<p><i>Author: @Name</i></p>
<PageTitle>Software Wizardry - @Name</PageTitle>
@code {
[Parameter]
public int ArticleId { get; set; }
public string? Name { get; set; }
protected override void OnInitialized()
{
if (ArticleId == 16)
{
Name = "Claudio Bernasconi";
}
else
{
Name = "Unknown";
}
}
}
Once again, we accept the ArticleId
as the parameter of the component. In the OnInitialized
lifecycle method, we have a logic to load the author’s name.
In this example, we have a trivial if/else
logic to resolve my name for the article id 16, and Unknown for all other articles. In a real application, we might call an API to load the author’s information specific to this article.
In the template definition, we append the author’s name to the static part of the page title using the PageTitle
component.
Since Blazor renders top-down, the PageTitle
component inside the Author
component is rendered after the PageTitle
component inside the Article
component. Therefore, the page title will include the author.
Metadata
Metadata is important for search engine optimization (SEO) since the content of the description, title and sometimes author properties is displayed on the search result page.
The HeadContent
component allows us to add additional tags to the head section of an HTML document. Consider the following snippet added to the previously shown Article
page component:
<HeadContent>
<meta name="description" content="Amazing Content in a blog post." />
</HeadContent>
The HeadContent
component from the Microsoft.AspNetCore.Components.Web namespace provides a simple component that allows new metadata to be added to an HTML document.
In this case, we add a meta tag with the name description and provide a text as its content.
Remember the Author
child component of the Article
component shown above? Let’s also add the author as metadata to the HTML document.
Attention: The first idea is to add another HeadContent
component inside the Author component. However, similar to the PageTitle
component, top-down rendering results in the last HeadContent
component winning. Only its content will be added to the head section of the HTML document.
However, Blazor wouldn’t be Blazor if there wasn’t a simple solution to solve the issue we have here. We want to add the author’s information and not replace the description.
We change the implementation in the Article
component and use the SectionOutlet
introduced in .NET 8.
<HeadContent>
<meta name="description" content="Amazing Content in a blog post." />
<SectionOutlet SectionName="HeadContent" />
</HeadContent>
In addition to the description, we add the SectionOutlet
component and provide a section name.
In the Author
component, instead of using the HeadContent
and causing the order problem during the rendering phase, we use the SectionContent
component using the same section name to define the author meta tag.
<SectionContent SectionName="HeadContent">
<meta name="author" content="@Name" />
</SectionContent>
Using this template code, we will have both the description and the author tag inside the resulting HTML document.
Consider the following screenshot from the developer tools when visiting the Article
page.
We can use the HeadContent
and the combination of the SectionOutlet
and SectionContent
components to add various other head tags, such as social media tags for Facebook, Twitter/X or LinkedIn.
Conclusion
Setting the page title and metadata is important when it comes to SEO for public-facing websites and web applications.
Blazor provides the PageTitle
component to conveniently set the page’s title, which is shown in the browser tab. Remember that the last call to the component in the rendering hierarchy will win, and its content will be shown.
The HeadContent
component, in combination with the SectionOutlet
and the SectionContent
components, provides a convenient way to add additional (context-sensitive) metadata to the HTML document.
You can access the code used in this example on GitHub.
If you want to learn more about Blazor development, you can watch my free Blazor Crash Course on YouTube. And stay tuned to the Telerik blog for more Blazor Basics.
This content originally appeared on Telerik Blogs and was authored by Claudio Bernasconi
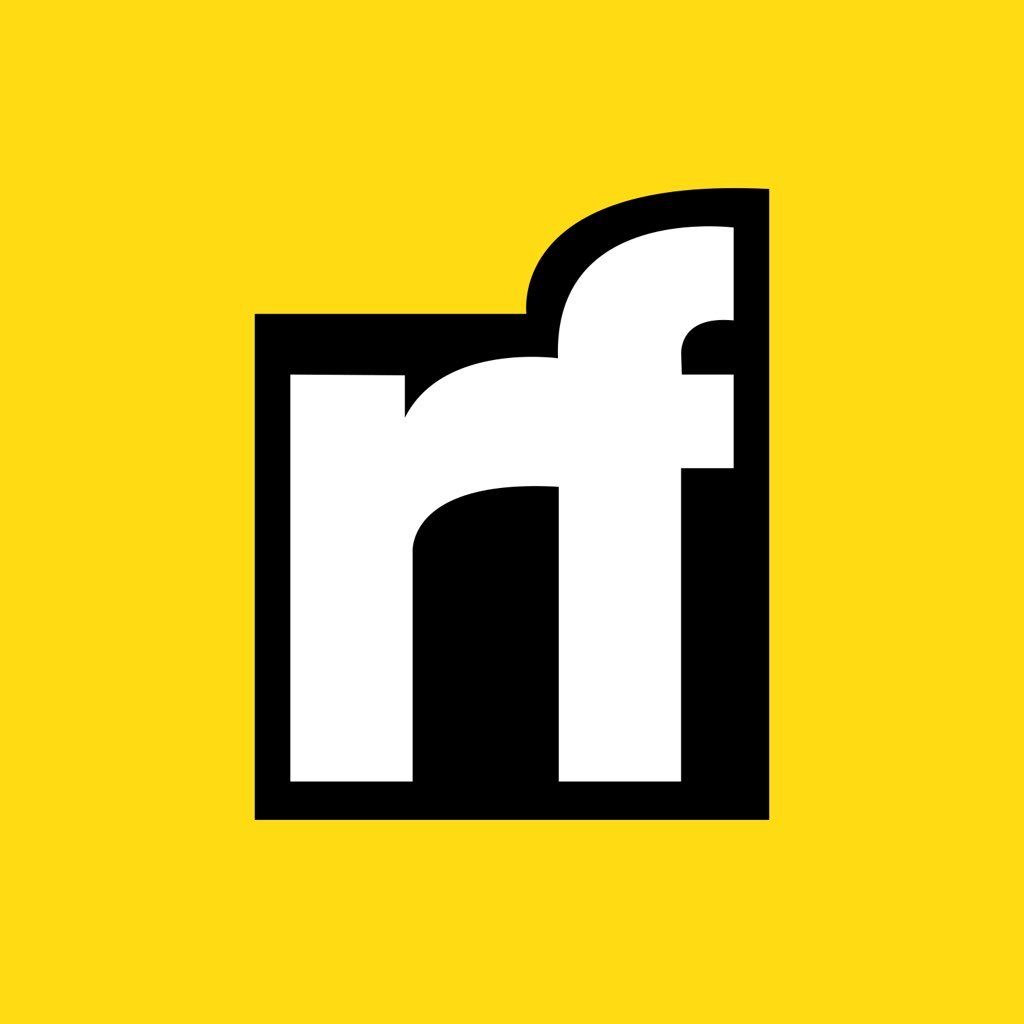
Claudio Bernasconi | Sciencx (2024-07-24T15:16:11+00:00) Blazor Basics: Controlling the HTML HEAD Section in Blazor. Retrieved from https://www.scien.cx/2024/07/24/blazor-basics-controlling-the-html-head-section-in-blazor/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.